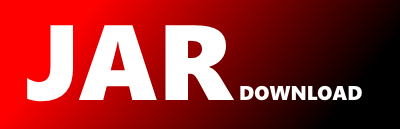
de.prob.prolog.term.IntegerPrologTerm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of prologlib Show documentation
Show all versions of prologlib Show documentation
Part of the ProB Parser library
/*
* (c) 2009-2022 Lehrstuhl fuer Softwaretechnik und Programmiersprachen, Heinrich
* Heine Universitaet Duesseldorf This software is licenced under EPL 1.0
* (http://www.eclipse.org/org/documents/epl-v10.html)
* */
package de.prob.prolog.term;
import java.math.BigInteger;
import de.prob.prolog.output.IPrologTermOutput;
/**
* Represents a Prolog integer.
*/
public final class IntegerPrologTerm extends AIntegerPrologTerm {
private final BigInteger value;
private final long ivalue; // holds the integer if value==null
// ideally we should create two instance classes, one for long and one for BigInteger
public IntegerPrologTerm(final BigInteger value) {
// super(value.toString());
// TODO: we could check longValueExact and catch ArithmeticException
this.value = value;
this.ivalue = -1;
}
public IntegerPrologTerm(final long value) {
//this(BigInteger.valueOf(value));
this.value = null; // BigInteger is not required
this.ivalue = value;
}
public IntegerPrologTerm(final byte[] arr) {
// super(new BigInteger(arr).toString());
this.value = new BigInteger(arr);
this.ivalue = -1;
}
@Override
public String getFunctor() {
if (value==null)
return Long.toString(ivalue);
else
return value.toString();
}
@Override
public BigInteger getValue() {
if (value==null)
return BigInteger.valueOf(ivalue);
else
return value;
}
@Override
public long longValueExact() {
if (value == null) {
return this.ivalue;
} else {
return this.value.longValueExact();
}
}
@Override
public int intValueExact() {
if (value == null) {
if (this.ivalue > Integer.MAX_VALUE || this.ivalue < Integer.MIN_VALUE) {
throw new ArithmeticException("IntegerPrologTerm value out of int range");
}
return (int)this.ivalue;
} else {
return this.value.intValueExact();
}
}
@Override
public void toTermOutput(final IPrologTermOutput pto) {
if (value==null)
pto.printNumber(ivalue);
else
pto.printNumber(value);
}
@Override
public int hashCode() {
if (value==null)
return Long.hashCode(ivalue) * 11 + 4;
else
return value.hashCode() * 11 + 4;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy