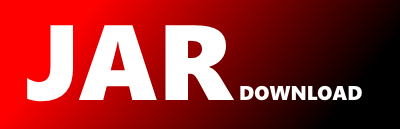
de.icongmbh.oss.maven.plugin.javassist.ClassnameExtractor Maven / Gradle / Ivy
Show all versions of javassist-maven-plugin Show documentation
/*
* Copyright 2013 https://github.com/barthel
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.icongmbh.oss.maven.plugin.javassist;
import static org.apache.commons.io.FilenameUtils.removeExtension;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
/**
* Provides methods for extract class name from file name.
*
* @author Uwe Barthel
*/
public class ClassnameExtractor {
private ClassnameExtractor() {
// private constructor for utility class
}
/**
* Remove passed parent directory from passed file name and replace
* directory separator with dots.
*
* e.g.:
*
*
* - parantDirectory:
/tmp/my/parant/src/
* - classFile:
/tmp/my/parent/src/foo/bar/MyApp.class
*
* returns: foo.bar.MyApp
*
* @param parentDirectory
* @param classFile
* @return class name extracted from file name or null
* @throws IOException
*/
public static String extractClassNameFromFile(final File parentDirectory,
final File classFile) throws IOException {
if (null == classFile) {
return null;
}
final String qualifiedFileName = parentDirectory != null ? classFile
.getCanonicalPath().substring(
parentDirectory.getCanonicalPath().length() + 1)
: classFile.getCanonicalPath();
return removeExtension(qualifiedFileName.replace(File.separator, "."));
}
/**
* @param parentDirectory
* @param classFiles
* @return iterator of full qualified class names based on passed classFiles
* @see #iterateClassnames(File, Iterator)
*/
public static Iterator iterateClassnames(
final File parentDirectory, final File... classFiles) {
return iterateClassnames(parentDirectory, Arrays.asList(classFiles)
.iterator());
}
/**
* Wrapping passed iterator (as reference) of class file names and extract full qualified class name on
* {@link Iterator#next()}.
*
* It is possible that {@link Iterator#hasNext()} returns true
* and {@link Iterator#next()} returns null
.
*
* @param parentDirectory
* @param classFiles
* @return iterator of full qualified class names based on passed classFiles
* or null
* @see #extractClassNameFromFile(File, File)
*/
// DANGEROUS call by reference
public static Iterator iterateClassnames(
final File parentDirectory, final Iterator classFileIterator) {
return new Iterator() {
// @Override
public boolean hasNext() {
return classFileIterator == null ? false : classFileIterator
.hasNext();
}
// @Override
public String next() {
final File classFile = classFileIterator.next();
try {
// possible returns null
return extractClassNameFromFile(parentDirectory, classFile);
} catch (final IOException e) {
throw new RuntimeException(e.getMessage());
}
}
// @Override
public void remove() {
classFileIterator.remove();
}
};
}
/**
* Wrapping passed list (as reference) of class file names and extract full qualified class name on
* {@link Iterator#next()}.
*
* It is possible that {@link Iterator#hasNext()} returns true
* and {@link Iterator#next()} returns null
.
*
* @param parentDirectory
* @param classFiles
* @return list of full qualified class names based on passed classFiles or
* null
* @throws IOException
* @see {@link #extractClassNameFromFile(File, File)}
*/
// DANGEROUS call by reference
public static List listClassnames(final File parentDirectory,
final List classFileList) throws IOException {
if (null == classFileList || classFileList.isEmpty()) {
return Collections.emptyList();
}
final List list = new ArrayList(classFileList.size());
for (final File file : classFileList) {
list.add(extractClassNameFromFile(parentDirectory, file));
}
return list;
}
/**
* @param parentDirectory
* @param classFiles
* @return list of full qualified class names based on passed classFiles or
* null
* @throws IOException
* @see {@link #extractClassNameFromFile(File, File)}
*/
public static List listClassnames(final File parentDirectory,
final String... classFileList) throws IOException {
if (null == classFileList || classFileList.length <= 0) {
return Collections.emptyList();
}
final List list = new ArrayList(classFileList.length);
for (final String file : classFileList) {
list.add(extractClassNameFromFile(parentDirectory, new File(
parentDirectory, file)));
}
return list;
}
}