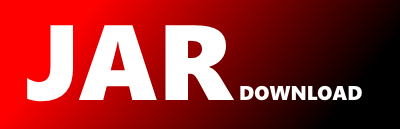
de.id4i.api.HistoryApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of id4i-api-client Show documentation
Show all versions of id4i-api-client Show documentation
The ID4i API is an HTTP API that allows developer to implement applications on top of http://id4i.de. This provides for automating GUID creation and object registration, working with Collections of GUIDs and Routing.
The newest version!
/*
* ID4i API
* ID4i HTTP API
*
* OpenAPI spec version: 0.9.7
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package de.id4i.api;
import de.id4i.ApiCallback;
import de.id4i.ApiClient;
import de.id4i.ApiException;
import de.id4i.ApiResponse;
import de.id4i.Configuration;
import de.id4i.Pair;
import de.id4i.ProgressRequestBody;
import de.id4i.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import de.id4i.api.model.ApiError;
import de.id4i.api.model.HistoryItem;
import de.id4i.api.model.HistoryItemUpdate;
import java.time.LocalDateTime;
import de.id4i.api.model.PaginatedResponseOfHistoryItem;
import de.id4i.api.model.Visibility;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class HistoryApi {
private ApiClient apiClient;
public HistoryApi() {
this(Configuration.getDefaultApiClient());
}
public HistoryApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for addItem
* @param id4n GUID to retrieve the history for (required)
* @param historyItem The history item to publish (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addItemCall(String id4n, HistoryItem historyItem, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = historyItem;
// create path and map variables
String localVarPath = "/api/v1/history/{id4n}"
.replaceAll("\\{" + "id4n" + "\\}", apiClient.escapeString(id4n.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/xml", "application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "Authorization" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addItemValidateBeforeCall(String id4n, HistoryItem historyItem, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id4n' is set
if (id4n == null) {
throw new ApiException("Missing the required parameter 'id4n' when calling addItem(Async)");
}
// verify the required parameter 'historyItem' is set
if (historyItem == null) {
throw new ApiException("Missing the required parameter 'historyItem' when calling addItem(Async)");
}
com.squareup.okhttp.Call call = addItemCall(id4n, historyItem, progressListener, progressRequestListener);
return call;
}
/**
* Add history item
* Add a new history item
* @param id4n GUID to retrieve the history for (required)
* @param historyItem The history item to publish (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void addItem(String id4n, HistoryItem historyItem) throws ApiException {
addItemWithHttpInfo(id4n, historyItem);
}
/**
* Add history item
* Add a new history item
* @param id4n GUID to retrieve the history for (required)
* @param historyItem The history item to publish (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addItemWithHttpInfo(String id4n, HistoryItem historyItem) throws ApiException {
com.squareup.okhttp.Call call = addItemValidateBeforeCall(id4n, historyItem, null, null);
return apiClient.execute(call);
}
/**
* Add history item (asynchronously)
* Add a new history item
* @param id4n GUID to retrieve the history for (required)
* @param historyItem The history item to publish (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addItemAsync(String id4n, HistoryItem historyItem, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addItemValidateBeforeCall(id4n, historyItem, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for filteredList
* @param id4n GUID to retrieve the history for (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param organization Show only entries created by one of the given organizations. This parameter can be used multiple times. (optional)
* @param type Show only entries matching one of the given history item types. This parameter can be used multiple times. (optional)
* @param qualifier Show only entries matching one of the given history item qualifiers (additional property de.id4i.history.item.qualifier). This parameter can be used multiple times. (optional)
* @param fromDate From date time as UTC Date-Time format (optional)
* @param toDate To date time as UTC Date-Time format (optional)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call filteredListCall(String id4n, Boolean includePrivate, String organization, List type, List qualifier, LocalDateTime fromDate, LocalDateTime toDate, Integer offset, Integer limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/history/{id4n}"
.replaceAll("\\{" + "id4n" + "\\}", apiClient.escapeString(id4n.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (includePrivate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("includePrivate", includePrivate));
if (organization != null)
localVarQueryParams.addAll(apiClient.parameterToPair("organization", organization));
if (type != null)
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("multi", "type", type));
if (qualifier != null)
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("multi", "qualifier", qualifier));
if (fromDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("fromDate", fromDate));
if (toDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("toDate", toDate));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/xml", "application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "Authorization" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call filteredListValidateBeforeCall(String id4n, Boolean includePrivate, String organization, List type, List qualifier, LocalDateTime fromDate, LocalDateTime toDate, Integer offset, Integer limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id4n' is set
if (id4n == null) {
throw new ApiException("Missing the required parameter 'id4n' when calling filteredList(Async)");
}
com.squareup.okhttp.Call call = filteredListCall(id4n, includePrivate, organization, type, qualifier, fromDate, toDate, offset, limit, progressListener, progressRequestListener);
return call;
}
/**
* List history
* Lists the history of a GUID
* @param id4n GUID to retrieve the history for (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param organization Show only entries created by one of the given organizations. This parameter can be used multiple times. (optional)
* @param type Show only entries matching one of the given history item types. This parameter can be used multiple times. (optional)
* @param qualifier Show only entries matching one of the given history item qualifiers (additional property de.id4i.history.item.qualifier). This parameter can be used multiple times. (optional)
* @param fromDate From date time as UTC Date-Time format (optional)
* @param toDate To date time as UTC Date-Time format (optional)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @return PaginatedResponseOfHistoryItem
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public PaginatedResponseOfHistoryItem filteredList(String id4n, Boolean includePrivate, String organization, List type, List qualifier, LocalDateTime fromDate, LocalDateTime toDate, Integer offset, Integer limit) throws ApiException {
ApiResponse resp = filteredListWithHttpInfo(id4n, includePrivate, organization, type, qualifier, fromDate, toDate, offset, limit);
return resp.getData();
}
/**
* List history
* Lists the history of a GUID
* @param id4n GUID to retrieve the history for (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param organization Show only entries created by one of the given organizations. This parameter can be used multiple times. (optional)
* @param type Show only entries matching one of the given history item types. This parameter can be used multiple times. (optional)
* @param qualifier Show only entries matching one of the given history item qualifiers (additional property de.id4i.history.item.qualifier). This parameter can be used multiple times. (optional)
* @param fromDate From date time as UTC Date-Time format (optional)
* @param toDate To date time as UTC Date-Time format (optional)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @return ApiResponse<PaginatedResponseOfHistoryItem>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse filteredListWithHttpInfo(String id4n, Boolean includePrivate, String organization, List type, List qualifier, LocalDateTime fromDate, LocalDateTime toDate, Integer offset, Integer limit) throws ApiException {
com.squareup.okhttp.Call call = filteredListValidateBeforeCall(id4n, includePrivate, organization, type, qualifier, fromDate, toDate, offset, limit, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* List history (asynchronously)
* Lists the history of a GUID
* @param id4n GUID to retrieve the history for (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param organization Show only entries created by one of the given organizations. This parameter can be used multiple times. (optional)
* @param type Show only entries matching one of the given history item types. This parameter can be used multiple times. (optional)
* @param qualifier Show only entries matching one of the given history item qualifiers (additional property de.id4i.history.item.qualifier). This parameter can be used multiple times. (optional)
* @param fromDate From date time as UTC Date-Time format (optional)
* @param toDate To date time as UTC Date-Time format (optional)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call filteredListAsync(String id4n, Boolean includePrivate, String organization, List type, List qualifier, LocalDateTime fromDate, LocalDateTime toDate, Integer offset, Integer limit, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = filteredListValidateBeforeCall(id4n, includePrivate, organization, type, qualifier, fromDate, toDate, offset, limit, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for list
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @deprecated
*/
@Deprecated
public com.squareup.okhttp.Call listCall(String id4n, String organizationId, Boolean includePrivate, Integer offset, Integer limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/history/{id4n}/{organizationId}"
.replaceAll("\\{" + "id4n" + "\\}", apiClient.escapeString(id4n.toString()))
.replaceAll("\\{" + "organizationId" + "\\}", apiClient.escapeString(organizationId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (includePrivate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("includePrivate", includePrivate));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/xml", "application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "Authorization" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@Deprecated
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call listValidateBeforeCall(String id4n, String organizationId, Boolean includePrivate, Integer offset, Integer limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id4n' is set
if (id4n == null) {
throw new ApiException("Missing the required parameter 'id4n' when calling list(Async)");
}
// verify the required parameter 'organizationId' is set
if (organizationId == null) {
throw new ApiException("Missing the required parameter 'organizationId' when calling list(Async)");
}
com.squareup.okhttp.Call call = listCall(id4n, organizationId, includePrivate, offset, limit, progressListener, progressRequestListener);
return call;
}
/**
* DEPRECATED - List history
* DEPRECATED - please use filteredList with organization parameter to achieve the same functionality
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @return PaginatedResponseOfHistoryItem
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @deprecated
*/
@Deprecated
public PaginatedResponseOfHistoryItem list(String id4n, String organizationId, Boolean includePrivate, Integer offset, Integer limit) throws ApiException {
ApiResponse resp = listWithHttpInfo(id4n, organizationId, includePrivate, offset, limit);
return resp.getData();
}
/**
* DEPRECATED - List history
* DEPRECATED - please use filteredList with organization parameter to achieve the same functionality
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @return ApiResponse<PaginatedResponseOfHistoryItem>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @deprecated
*/
@Deprecated
public ApiResponse listWithHttpInfo(String id4n, String organizationId, Boolean includePrivate, Integer offset, Integer limit) throws ApiException {
com.squareup.okhttp.Call call = listValidateBeforeCall(id4n, organizationId, includePrivate, offset, limit, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* DEPRECATED - List history (asynchronously)
* DEPRECATED - please use filteredList with organization parameter to achieve the same functionality
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param includePrivate Also return private history entries (optional, default to true)
* @param offset Start with the n-th element (optional)
* @param limit The maximum count of returned elements (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @deprecated
*/
@Deprecated
public com.squareup.okhttp.Call listAsync(String id4n, String organizationId, Boolean includePrivate, Integer offset, Integer limit, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = listValidateBeforeCall(id4n, organizationId, includePrivate, offset, limit, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for retrieveItem
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call retrieveItemCall(String id4n, String organizationId, Integer sequenceId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v1/history/{id4n}/{organizationId}/{sequenceId}"
.replaceAll("\\{" + "id4n" + "\\}", apiClient.escapeString(id4n.toString()))
.replaceAll("\\{" + "organizationId" + "\\}", apiClient.escapeString(organizationId.toString()))
.replaceAll("\\{" + "sequenceId" + "\\}", apiClient.escapeString(sequenceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/xml", "application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "Authorization" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call retrieveItemValidateBeforeCall(String id4n, String organizationId, Integer sequenceId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id4n' is set
if (id4n == null) {
throw new ApiException("Missing the required parameter 'id4n' when calling retrieveItem(Async)");
}
// verify the required parameter 'organizationId' is set
if (organizationId == null) {
throw new ApiException("Missing the required parameter 'organizationId' when calling retrieveItem(Async)");
}
// verify the required parameter 'sequenceId' is set
if (sequenceId == null) {
throw new ApiException("Missing the required parameter 'sequenceId' when calling retrieveItem(Async)");
}
com.squareup.okhttp.Call call = retrieveItemCall(id4n, organizationId, sequenceId, progressListener, progressRequestListener);
return call;
}
/**
* Get history item
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @return HistoryItem
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public HistoryItem retrieveItem(String id4n, String organizationId, Integer sequenceId) throws ApiException {
ApiResponse resp = retrieveItemWithHttpInfo(id4n, organizationId, sequenceId);
return resp.getData();
}
/**
* Get history item
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @return ApiResponse<HistoryItem>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse retrieveItemWithHttpInfo(String id4n, String organizationId, Integer sequenceId) throws ApiException {
com.squareup.okhttp.Call call = retrieveItemValidateBeforeCall(id4n, organizationId, sequenceId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get history item (asynchronously)
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call retrieveItemAsync(String id4n, String organizationId, Integer sequenceId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = retrieveItemValidateBeforeCall(id4n, organizationId, sequenceId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updateItem
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param update update (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call updateItemCall(String id4n, String organizationId, Integer sequenceId, HistoryItemUpdate update, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = update;
// create path and map variables
String localVarPath = "/api/v1/history/{id4n}/{organizationId}/{sequenceId}"
.replaceAll("\\{" + "id4n" + "\\}", apiClient.escapeString(id4n.toString()))
.replaceAll("\\{" + "organizationId" + "\\}", apiClient.escapeString(organizationId.toString()))
.replaceAll("\\{" + "sequenceId" + "\\}", apiClient.escapeString(sequenceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/xml", "application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "Authorization" };
return apiClient.buildCall(localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateItemValidateBeforeCall(String id4n, String organizationId, Integer sequenceId, HistoryItemUpdate update, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id4n' is set
if (id4n == null) {
throw new ApiException("Missing the required parameter 'id4n' when calling updateItem(Async)");
}
// verify the required parameter 'organizationId' is set
if (organizationId == null) {
throw new ApiException("Missing the required parameter 'organizationId' when calling updateItem(Async)");
}
// verify the required parameter 'sequenceId' is set
if (sequenceId == null) {
throw new ApiException("Missing the required parameter 'sequenceId' when calling updateItem(Async)");
}
// verify the required parameter 'update' is set
if (update == null) {
throw new ApiException("Missing the required parameter 'update' when calling updateItem(Async)");
}
com.squareup.okhttp.Call call = updateItemCall(id4n, organizationId, sequenceId, update, progressListener, progressRequestListener);
return call;
}
/**
* Update history item
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param update update (required)
* @return HistoryItem
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public HistoryItem updateItem(String id4n, String organizationId, Integer sequenceId, HistoryItemUpdate update) throws ApiException {
ApiResponse resp = updateItemWithHttpInfo(id4n, organizationId, sequenceId, update);
return resp.getData();
}
/**
* Update history item
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param update update (required)
* @return ApiResponse<HistoryItem>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateItemWithHttpInfo(String id4n, String organizationId, Integer sequenceId, HistoryItemUpdate update) throws ApiException {
com.squareup.okhttp.Call call = updateItemValidateBeforeCall(id4n, organizationId, sequenceId, update, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update history item (asynchronously)
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param update update (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateItemAsync(String id4n, String organizationId, Integer sequenceId, HistoryItemUpdate update, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateItemValidateBeforeCall(id4n, organizationId, sequenceId, update, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updateItemVisibility
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param visibility History item visibility restrictions (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call updateItemVisibilityCall(String id4n, String organizationId, Integer sequenceId, Visibility visibility, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = visibility;
// create path and map variables
String localVarPath = "/api/v1/history/{id4n}/{organizationId}/{sequenceId}/visibility"
.replaceAll("\\{" + "id4n" + "\\}", apiClient.escapeString(id4n.toString()))
.replaceAll("\\{" + "organizationId" + "\\}", apiClient.escapeString(organizationId.toString()))
.replaceAll("\\{" + "sequenceId" + "\\}", apiClient.escapeString(sequenceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/xml", "application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "Authorization" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateItemVisibilityValidateBeforeCall(String id4n, String organizationId, Integer sequenceId, Visibility visibility, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id4n' is set
if (id4n == null) {
throw new ApiException("Missing the required parameter 'id4n' when calling updateItemVisibility(Async)");
}
// verify the required parameter 'organizationId' is set
if (organizationId == null) {
throw new ApiException("Missing the required parameter 'organizationId' when calling updateItemVisibility(Async)");
}
// verify the required parameter 'sequenceId' is set
if (sequenceId == null) {
throw new ApiException("Missing the required parameter 'sequenceId' when calling updateItemVisibility(Async)");
}
// verify the required parameter 'visibility' is set
if (visibility == null) {
throw new ApiException("Missing the required parameter 'visibility' when calling updateItemVisibility(Async)");
}
com.squareup.okhttp.Call call = updateItemVisibilityCall(id4n, organizationId, sequenceId, visibility, progressListener, progressRequestListener);
return call;
}
/**
* Set history item visibility
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param visibility History item visibility restrictions (required)
* @return HistoryItem
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public HistoryItem updateItemVisibility(String id4n, String organizationId, Integer sequenceId, Visibility visibility) throws ApiException {
ApiResponse resp = updateItemVisibilityWithHttpInfo(id4n, organizationId, sequenceId, visibility);
return resp.getData();
}
/**
* Set history item visibility
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param visibility History item visibility restrictions (required)
* @return ApiResponse<HistoryItem>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateItemVisibilityWithHttpInfo(String id4n, String organizationId, Integer sequenceId, Visibility visibility) throws ApiException {
com.squareup.okhttp.Call call = updateItemVisibilityValidateBeforeCall(id4n, organizationId, sequenceId, visibility, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Set history item visibility (asynchronously)
*
* @param id4n GUID to retrieve the history for (required)
* @param organizationId organizationId (required)
* @param sequenceId sequenceId (required)
* @param visibility History item visibility restrictions (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateItemVisibilityAsync(String id4n, String organizationId, Integer sequenceId, Visibility visibility, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateItemVisibilityValidateBeforeCall(id4n, organizationId, sequenceId, visibility, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy