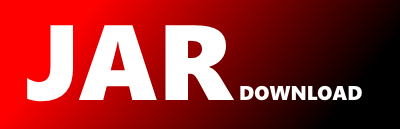
de.id4i.api.model.Route Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of id4i-api-client Show documentation
Show all versions of id4i-api-client Show documentation
The ID4i API is an HTTP API that allows developer to implement applications on top of http://id4i.de. This provides for automating GUID creation and object registration, working with Collections of GUIDs and Routing.
The newest version!
/*
* ID4i API
* ID4i HTTP API
*
* OpenAPI spec version: 0.9.7
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package de.id4i.api.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Route
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-11-21T14:03:07.356Z")
public class Route {
@SerializedName("params")
private Map params = new HashMap<>();
@SerializedName("priority")
private Integer priority = null;
@SerializedName("public")
private Boolean _public = null;
@SerializedName("type")
private String type = null;
@SerializedName("validUntil")
private Long validUntil = null;
public Route params(Map params) {
this.params = params;
return this;
}
public Route putParamsItem(String key, String paramsItem) {
this.params.put(key, paramsItem);
return this;
}
/**
* Get params
* @return params
**/
@ApiModelProperty(required = true, value = "")
public Map getParams() {
return params;
}
public void setParams(Map params) {
this.params = params;
}
public Route priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* Get priority
* @return priority
**/
@ApiModelProperty(value = "")
public Integer getPriority() {
return priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
}
public Route _public(Boolean _public) {
this._public = _public;
return this;
}
/**
* Get _public
* @return _public
**/
@ApiModelProperty(required = true, value = "")
public Boolean isPublic() {
return _public;
}
public void setPublic(Boolean _public) {
this._public = _public;
}
public Route type(String type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(required = true, value = "")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Route validUntil(Long validUntil) {
this.validUntil = validUntil;
return this;
}
/**
* Get validUntil
* @return validUntil
**/
@ApiModelProperty(value = "")
public Long getValidUntil() {
return validUntil;
}
public void setValidUntil(Long validUntil) {
this.validUntil = validUntil;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Route route = (Route) o;
return Objects.equals(this.params, route.params) &&
Objects.equals(this.priority, route.priority) &&
Objects.equals(this._public, route._public) &&
Objects.equals(this.type, route.type) &&
Objects.equals(this.validUntil, route.validUntil);
}
@Override
public int hashCode() {
return Objects.hash(params, priority, _public, type, validUntil);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Route {\n");
sb.append(" params: ").append(toIndentedString(params)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" _public: ").append(toIndentedString(_public)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" validUntil: ").append(toIndentedString(validUntil)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy