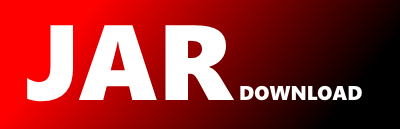
com.inet.sass.expression.ArithmeticExpressionEvaluator Maven / Gradle / Ivy
/*
* Copyright 2023 i-net software
* Copyright 2000-2014 Vaadin Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.inet.sass.expression;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_GE;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_GT;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_LE;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_LT;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_MINUS;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_MOD;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_MULTIPLY;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_PLUS;
import static com.inet.sass.parser.SCSSLexicalUnit.SAC_OPERATOR_SLASH;
import static com.inet.sass.parser.SCSSLexicalUnit.SCSS_OPERATOR_AND;
import static com.inet.sass.parser.SCSSLexicalUnit.SCSS_OPERATOR_EQUALS;
import static com.inet.sass.parser.SCSSLexicalUnit.SCSS_OPERATOR_NOT_EQUAL;
import static com.inet.sass.parser.SCSSLexicalUnit.SCSS_OPERATOR_OR;
import java.util.List;
import java.util.Stack;
import com.inet.sass.ScssContext;
import com.inet.sass.parser.LexicalUnitImpl;
import com.inet.sass.parser.ParseException;
import com.inet.sass.parser.SassList;
import com.inet.sass.parser.SassList.Separator;
import com.inet.sass.parser.SassListItem;
public class ArithmeticExpressionEvaluator {
private static void createNewOperand( BinaryOperator operator, Stack
© 2015 - 2025 Weber Informatics LLC | Privacy Policy