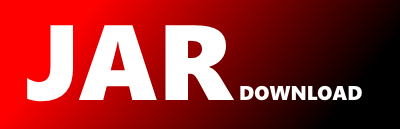
com.inet.sass.parser.ArgumentList Maven / Gradle / Ivy
/*
* Copyright 2023 i-net software
* Copyright 2000-2014 Vaadin Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.inet.sass.parser;
import java.util.ArrayList;
import java.util.List;
import com.inet.sass.ScssContext;
import com.inet.sass.tree.Node;
/**
* An ArgumentList is used for packing arguments into a list. There can be named
* and unnamed arguments, which are stored separately. ArgumentList is used for
* representing the arguments of a function or a mixin. ArgumentList is also
* used in ActualArgumentList to represent the unnamed (positional) and named
* parameters of an @include or a function call.
*/
public class ArgumentList extends SassList {
private List namedVariables = new ArrayList();
public ArgumentList(SassList list) {
super(list.getSeparator(), list.getItems());
}
public ArgumentList(Separator separator, List list,
List named) {
super(separator, list);
namedVariables = named;
}
public ArgumentList(Separator sep, SassListItem... items) {
super(sep, items);
}
public ArgumentList(Separator separator, List newParamValues) {
super(separator, newParamValues);
}
public List getNamedVariables() {
return namedVariables;
}
@Override
public ArgumentList evaluateFunctionsAndExpressions(ScssContext context,
boolean evaluateArithmetics) {
List list = new ArrayList();
for (SassListItem item : this) {
list.add(item.evaluateFunctionsAndExpressions(context,
evaluateArithmetics));
}
List named = new ArrayList();
for (Variable var : namedVariables) {
named.add(new Variable(var.getName(), var.getExpr()
.evaluateFunctionsAndExpressions(context,
evaluateArithmetics), var.isGuarded()));
}
return new ArgumentList(getSeparator(), list, named);
}
protected Separator getSeparator(SassListItem expr) {
if (expr instanceof SassList) {
SassList lastList = (SassList) expr;
if (lastList.size() > 1) {
return lastList.getSeparator();
}
}
return SassList.Separator.COMMA;
}
@Override
public String toString() {
String unnamed = buildString(Node.PRINT_STRATEGY);
String named = namedAsString();
if (unnamed.length() > 0 && named.length() > 0) {
unnamed += ", ";
}
return "ArgumentList [" + unnamed + named + "]";
}
private String namedAsString() {
String result = "";
for (int i = 0; i < namedVariables.size(); i++) {
Variable named = namedVariables.get(i);
String contents = named.getExpr() == null ? "null" : named
.getExpr().buildString(Node.PRINT_STRATEGY);
result += "$" + named.getName() + ": " + contents;
if (i < namedVariables.size() - 1) {
result += ", ";
}
}
return result;
}
@Override
public ArgumentList updateUrl(String prefix) {
SassList newUnnamedVariables = super.updateUrl(prefix);
ArrayList newNamedVariables = new ArrayList(
namedVariables.size());
for (Variable var : namedVariables) {
if (var.getExpr() != null) {
SassListItem newExpr = var.getExpr().updateUrl(prefix);
newNamedVariables.add(new Variable(var.getName(), newExpr, var
.isGuarded()));
} else {
newNamedVariables.add(var);
}
}
return new ArgumentList(getSeparator(), newUnnamedVariables.getItems(),
newNamedVariables);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy