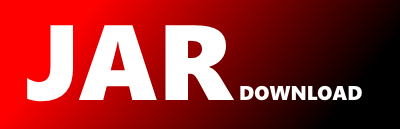
de.intarsys.tools.event.DeferredEventDispatcher Maven / Gradle / Ivy
Show all versions of isrt Show documentation
package de.intarsys.tools.event;
import java.util.ArrayList;
import java.util.List;
/**
* Pluggable helper object for management and dispatching of events. This
* utility is quite handsome when you
*
* - need to ensure event ordering in multithreaded scenarios
* - need to trigger events that you want to keep out of synchronized code
*
*
* Events are forwarded upon "flush" to all listeners in the thread of the
* caller.
*
*/
public class DeferredEventDispatcher implements INotificationListener,
INotificationSupport {
final private List events = new ArrayList();
final private EventDispatcher dispatcher;
final private Object lock = new Object();
public DeferredEventDispatcher(EventDispatcher dispatcher) {
this.dispatcher = dispatcher;
}
public DeferredEventDispatcher(Object owner) {
this.dispatcher = new EventDispatcher(owner);
}
public void addNotificationListener(EventType type,
INotificationListener listener) {
dispatcher.addNotificationListener(type, listener);
}
public void flush() {
List tempEvents;
synchronized (lock) {
tempEvents = new ArrayList(events);
events.clear();
}
for (Event event : tempEvents) {
dispatcher.handleEvent(event);
}
}
public void handleEvent(Event event) {
synchronized (lock) {
events.add(event);
}
}
public boolean hasListener() {
return dispatcher.hasListener();
}
public void removeNotificationListener(EventType type,
INotificationListener listener) {
dispatcher.removeNotificationListener(type, listener);
}
}