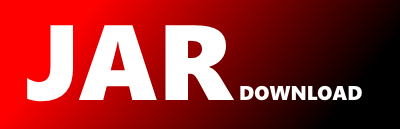
de.intarsys.tools.transaction.TransactionTools Maven / Gradle / Ivy
Show all versions of isrt Show documentation
package de.intarsys.tools.transaction;
/**
* Tools for handling transactions.
*
* Currently this lso implements the missing link between transactions and
* Resources.
*
*/
public class TransactionTools {
static public void delist(IResource resource) throws TransactionException {
Transaction transaction = (Transaction) TransactionManager.get()
.getTransaction();
if (transaction == null) {
throw new NoTransactionException();
}
transaction.delist(resource);
}
static public void enlist(IResource resource) throws TransactionException {
Transaction transaction = (Transaction) TransactionManager.get()
.getTransaction();
if (transaction == null) {
throw new NoTransactionException();
}
transaction.enlist(resource);
}
static public T getResource(IResourceType type,
boolean create) throws TransactionException {
Transaction transaction = (Transaction) TransactionManager.get()
.getTransaction();
if (transaction == null) {
throw new NoTransactionException();
}
return getResource(type, transaction, create);
}
static protected T getResource(IResourceType type,
Transaction transaction, boolean create)
throws TransactionException {
T tempResource = transaction.getResource(type);
if (tempResource == null) {
if (create) {
T parentResource = null;
Transaction parentTransaction = (Transaction) transaction
.getParent();
if (parentTransaction != null) {
parentResource = getResource(type, parentTransaction,
create);
}
tempResource = type.createResource(parentResource);
transaction.enlist(tempResource);
} else {
throw new TransactionException("no resource found");
}
}
return tempResource;
}
}