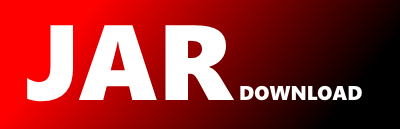
de.intarsys.tools.number.NumberParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of isrt Show documentation
Show all versions of isrt Show documentation
The basic runtime tools and interfaces for intarsys components.
/*
* Copyright (c) 2007, intarsys consulting GmbH
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* - Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* - Neither the name of intarsys nor the names of its contributors may be used
* to endorse or promote products derived from this software without specific
* prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package de.intarsys.tools.number;
import java.io.IOException;
import java.io.StringReader;
/**
* A parser able to read a definition of numbers.
*
* The parser supports single numbers, enumeration of numbers and intervals.
*
*
*
* S ::= NumberString
* NumberString ::= ( Number | Interval) [ ";" (Number | Interval) ]*
* Interval ::= Number "-" Number
* Number ::= a valid number literal
*
*
*/
public class NumberParser {
public static NumberWrapper parse(String numberstring) throws IOException {
if (numberstring == null) {
return null;
}
return new NumberParser(numberstring).parse();
}
private StringReader r;
protected NumberParser(String numberstring) {
super();
r = new StringReader(numberstring);
}
public int getChar() throws IOException {
int i = r.read();
return i;
}
private NumberWrapper makeWrapperObject(String numberValueInString) {
int trenner = numberValueInString.indexOf(NumberInterval.SEPARATOR, 1);
if (trenner == -1) {
// no 'intervaltrenner' means NumberInstance Object
double zahl = Double.parseDouble(numberValueInString);
NumberInstance instance = new NumberInstance(zahl);
return instance;
} else {
if (trenner == 0) {
// 'intervaltrenner' is first character in string
throw new NumberFormatException(
"Missing parameter FROM in interval");
} else {
if (trenner == (numberValueInString.length() - 1)) {
// 'intervaltrenner' is last character in string
throw new NumberFormatException(
"Missing parameter TO in interval");
} else {
// 'intervaltrenner' leads to NumberInterval Object
NumberInterval interval = new NumberInterval();
String stringFrom = numberValueInString.substring(0,
trenner);
String stringTo = numberValueInString
.substring(trenner + 1);
Number from = new Double(Double.parseDouble(stringFrom));
Number to = new Double(Double.parseDouble(stringTo));
interval.setFrom(from);
interval.setTo(to);
return interval;
}
}
}
}
private NumberWrapper parse() throws IOException {
NumberList numberList = new NumberList();
numberList = parseNumberString(numberList);
return numberList;
}
private NumberList parseNumberString(NumberList numberList)
throws IOException {
StringBuilder sb = new StringBuilder();
boolean spaceFoundInNumber = false;
for (int i = getChar(); i > -1; i = getChar()) {
char c = (char) i;
if (Character.isWhitespace(c)) {
if (sb.length() == 0) {
continue;
} else {
if (sb.charAt(sb.length() - 1) != '-') {
// space allowed after 'intervaltrenner'
spaceFoundInNumber = true;
}
continue;
}
} else {
if (c == NumberList.SEPARATOR) {
if (sb.length() == 0) {
// SEPARATOR is first sign in string
// throw new NumberFormatException("Missing parameter");
} else {
numberList.add(makeWrapperObject(sb.toString()));
sb.setLength(0);
spaceFoundInNumber = false;
}
} else {
if (spaceFoundInNumber == true) {
if (c != NumberInterval.SEPARATOR) {
throw new NumberFormatException(
"Space found in between number");
} else {
// space allowed before 'intervaltrenner'
sb.append(c);
spaceFoundInNumber = false;
}
} else {
sb.append(c);
}
}
}
}
if (sb.length() > 0) {
numberList.add(makeWrapperObject(sb.toString()));
} else {
// throw new IOException("Missing parameter");
}
return numberList;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy