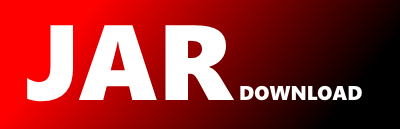
de.intarsys.tools.randomaccess.IRandomAccess Maven / Gradle / Ivy
Show all versions of isrt Show documentation
/*
* Copyright (c) 2007, intarsys consulting GmbH
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* - Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* - Neither the name of intarsys nor the names of its contributors may be used
* to endorse or promote products derived from this software without specific
* prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package de.intarsys.tools.randomaccess;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.RandomAccessFile;
/**
* An interface for an object that can randomly access bytes in a data stream.
*
* This is an abstraction of {@link RandomAccessFile} to support other data
* storage objects (like byte arrays and so on).
*/
public interface IRandomAccess {
/**
* A {@link InputStream} view on the data structure.
*
* @return A {@link InputStream} view on the data structure.
*/
public abstract InputStream asInputStream();
/**
* A {@link OutputStream} view on the data structure.
*
* @return A {@link OutputStream} view on the data structure.
*/
public abstract OutputStream asOutputStream();
/**
* Closes this random access data container and releases any system
* resources associated with the stream. A closed random access data
* container cannot perform input or output operations and cannot be
* reopened.
*
* @exception IOException
* if an I/O error occurs.
*/
public void close() throws IOException;
/**
* Force changes to be made persistent.
*
* @throws IOException
*/
public void flush() throws IOException;
/**
* Returns the length of this data container.
*
* @return the length of this data container, measured in bytes.
* @exception IOException
* if an I/O error occurs.
*/
public long getLength() throws IOException;
/**
* Returns the current offset in this data container.
*
* @return the offset from the beginning of the data container, in bytes, at
* which the next read or write occurs.
* @exception IOException
* if an I/O error occurs.
*/
public long getOffset() throws IOException;
/**
* true
if this is a read only data container.
*
* @return true
if this is a read only data container.
*/
public boolean isReadOnly();
/**
* Mark the current offset into the data in a stack like manner.
*/
public abstract void mark() throws IOException;
/**
* Reads a byte of data from this data container. The byte is returned as an
* integer in the range 0 to 255 (0x00-0x0ff
). This method
* blocks if no input is yet available.
*
* This method behaves in exactly the same way as the
* {@link InputStream#read()} method of InputStream
.
*
* @return the next byte of data, or -1
if the end of the data
* container has been reached.
* @exception IOException
* if an I/O error occurs. Not thrown if the end of the data
* container has been reached.
*/
public int read() throws IOException;
/**
* Reads up to buffer.length
bytes of data from this data
* container into an array of bytes. This method blocks until at least one
* byte of input is available.
*
* This method behaves in the exactly the same way as the
* {@link InputStream#read(byte[])} method of InputStream
.
*
* @param buffer
* the buffer into which the data is read.
* @return the total number of bytes read into the buffer, or
* -1
if there is no more data because the end of this
* data container has been reached.
* @exception IOException
* if an I/O error occurs.
*/
public int read(byte[] buffer) throws IOException;
/**
* Reads up to len
bytes of data from this data container into
* an array of bytes. This method blocks until at least one byte of input is
* available.
*
*
* @param buffer
* the buffer into which the data is read.
* @param start
* the start offset of the data.
* @param numBytes
* the maximum number of bytes read.
* @return the total number of bytes read into the buffer, or
* -1
if there is no more data because the end of the
* file has been reached.
* @exception IOException
* if an I/O error occurs.
*/
public int read(byte[] buffer, int start, int numBytes) throws IOException;
/**
* Reset to the last position on the mark-stack.
*/
public abstract void reset() throws IOException;
/**
* Sets the offset, measured from the beginning of the data container at
* which the next read or write occurs. The offset may be set beyond the end
* of the data container. Setting the offset beyond the end of the data
* container does not change the data container length. The length will
* change only by writing after the offset has been set beyond the end of
* the data container.
*
* @param offset
* the offset position, measured in bytes from the beginning of
* the data container
* @exception IOException
* if offset
is less than 0
or if
* an I/O error occurs.
*/
public void seek(long offset) throws IOException;
/**
* Sets the offset, measured from the current offset at which the next read
* or write occurs. The offset may be set beyond the end of the data
* container. Setting the offset beyond the end of the data container does
* not change the data container length. The length will change only by
* writing after the offset has been set beyond the end of the data
* container.
*
* @param delta
* the amount of bytes by which to change the current offset
* position
*
* @exception IOException
* if the resulting offset
is less than
* 0
or if an I/O error occurs.
*/
public void seekBy(long delta) throws IOException;
/**
* Assign the length. All bytes after length are truncated. If the real
* length is currently less than newLength, the data structure will be
* enlarged.
*
* @param newLength
* @throws IOException
*/
public void setLength(long newLength) throws IOException;
/**
* Writes b.length
bytes from the specified byte array,
* starting at the current offset.
*
* @param buffer
* the data.
* @exception IOException
* if an I/O error occurs.
*/
public void write(byte[] buffer) throws IOException;
/**
* Writes len
bytes from the specified byte array starting at
* start
.
*
* @param buffer
* the data.
* @param start
* the start offset in the data.
* @param numBytes
* the number of bytes to write.
* @exception IOException
* if an I/O error occurs.
*/
public abstract void write(byte[] buffer, int start, int numBytes)
throws IOException;
/**
* Writes the specified byte . The write starts at the current offset.
*
* @param b
* the byte
to be written.
* @exception IOException
* if an I/O error occurs.
*/
public void write(int b) throws IOException;
}