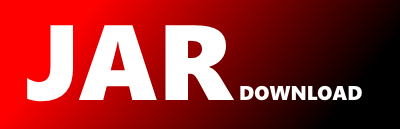
de.intarsys.pdf.postscript.Parser Maven / Gradle / Ivy
/*
* Copyright (c) 2007, intarsys consulting GmbH
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* - Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* - Neither the name of intarsys nor the names of its contributors may be used
* to endorse or promote products derived from this software without specific
* prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
/* Generated By:JavaCC: Do not edit this line. Parser.java */
package de.intarsys.pdf.postscript;
public class Parser implements ParserConstants {
static private int[] jj_la1_0;
static {
jj_la1_0();
}
private static void jj_la1_0() {
jj_la1_0 = new int[] { 0x3cf48, 0x3cf48, };
}
private java.util.Vector jj_expentries = new java.util.Vector();
private int[] jj_expentry;
private int jj_gen;
JavaCharStream jj_input_stream;
private int jj_kind = -1;
final private int[] jj_la1 = new int[2];
public Token jj_nt;
private int jj_ntk;
public Token token;
public ParserTokenManager token_source;
public Parser(java.io.InputStream stream) {
this(stream, null);
}
public Parser(java.io.InputStream stream, String encoding) {
try {
jj_input_stream = new JavaCharStream(stream, encoding, 1, 1);
} catch (java.io.UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
token_source = new ParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 2; i++)
jj_la1[i] = -1;
}
public Parser(java.io.Reader stream) {
jj_input_stream = new JavaCharStream(stream, 1, 1);
token_source = new ParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 2; i++)
jj_la1[i] = -1;
}
public Parser(ParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 2; i++)
jj_la1[i] = -1;
}
final public void disable_tracing() {
}
final public void enable_tracing() {
}
public ParseException generateParseException() {
jj_expentries.removeAllElements();
boolean[] la1tokens = new boolean[18];
for (int i = 0; i < 18; i++) {
la1tokens[i] = false;
}
if (jj_kind >= 0) {
la1tokens[jj_kind] = true;
jj_kind = -1;
}
for (int i = 0; i < 2; i++) {
if (jj_la1[i] == jj_gen) {
for (int j = 0; j < 32; j++) {
if ((jj_la1_0[i] & (1 << j)) != 0) {
la1tokens[j] = true;
}
}
}
}
for (int i = 0; i < 18; i++) {
if (la1tokens[i]) {
jj_expentry = new int[1];
jj_expentry[0] = i;
jj_expentries.addElement(jj_expentry);
}
}
int[][] exptokseq = new int[jj_expentries.size()][];
for (int i = 0; i < jj_expentries.size(); i++) {
exptokseq[i] = (int[]) jj_expentries.elementAt(i);
}
return new ParseException(token, exptokseq, tokenImage);
}
final public Token getNextToken() {
if (token.next != null) {
token = token.next;
} else {
token = token.next = token_source.getNextToken();
}
jj_ntk = -1;
jj_gen++;
return token;
}
final public Token getToken(int index) {
Token t = token;
for (int i = 0; i < index; i++) {
if (t.next != null) {
t = t.next;
} else {
t = t.next = token_source.getNextToken();
}
}
return t;
}
final private Token jj_consume_token(int kind) throws ParseException {
Token oldToken;
if ((oldToken = token).next != null) {
token = token.next;
} else {
token = token.next = token_source.getNextToken();
}
jj_ntk = -1;
if (token.kind == kind) {
jj_gen++;
return token;
}
token = oldToken;
jj_kind = kind;
throw generateParseException();
}
final private int jj_ntk() {
if ((jj_nt = token.next) == null) {
return (jj_ntk = (token.next = token_source.getNextToken()).kind);
} else {
return (jj_ntk = jj_nt.kind);
}
}
final public void parse(Handler handler) throws ParseException {
Token localToken;
label_1: while (true) {
switch ((jj_ntk == -1) ? jj_ntk() : jj_ntk) {
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case KEY_IDENTIFIER:
case IMMEDIATE_IDENTIFIER:
case LBRACE:
case RBRACE:
case LBRACKET:
case RBRACKET:
;
break;
default:
jj_la1[0] = jj_gen;
break label_1;
}
switch ((jj_ntk == -1) ? jj_ntk() : jj_ntk) {
case INTEGER_LITERAL:
localToken = jj_consume_token(INTEGER_LITERAL);
try {
handler.processLiteral(Integer.parseInt(localToken.image));
} catch (NumberFormatException e) {
if (true) {
throw new ParseException(e.toString());
}
}
break;
case FLOATING_POINT_LITERAL:
localToken = jj_consume_token(FLOATING_POINT_LITERAL);
try {
handler
.processLiteral(Double
.parseDouble(localToken.image));
} catch (NumberFormatException e) {
if (true) {
throw new ParseException(e.toString());
}
}
break;
case STRING_LITERAL:
localToken = jj_consume_token(STRING_LITERAL);
handler.processLiteral(localToken.image.substring(1,
localToken.image.length() - 1));
break;
case IDENTIFIER:
localToken = jj_consume_token(IDENTIFIER);
handler.processIdentifier(localToken.image);
break;
case KEY_IDENTIFIER:
localToken = jj_consume_token(KEY_IDENTIFIER);
handler.processKeyIdentifier(localToken.image.substring(1,
localToken.image.length()));
break;
case IMMEDIATE_IDENTIFIER:
localToken = jj_consume_token(IMMEDIATE_IDENTIFIER);
handler.processImmediateIdentifier(localToken.image.substring(
2, localToken.image.length()));
break;
case LBRACE:
jj_consume_token(LBRACE);
handler.processStartProcedure();
break;
case RBRACE:
jj_consume_token(RBRACE);
handler.processEndProcedure();
break;
case LBRACKET:
jj_consume_token(LBRACKET);
handler.processStartArray();
break;
case RBRACKET:
jj_consume_token(RBRACKET);
handler.processEndArray();
break;
default:
jj_la1[1] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
public void ReInit(java.io.InputStream stream) {
ReInit(stream, null);
}
public void ReInit(java.io.InputStream stream, String encoding) {
try {
jj_input_stream.ReInit(stream, encoding, 1, 1);
} catch (java.io.UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 2; i++)
jj_la1[i] = -1;
}
public void ReInit(java.io.Reader stream) {
jj_input_stream.ReInit(stream, 1, 1);
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 2; i++)
jj_la1[i] = -1;
}
public void ReInit(ParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 2; i++)
jj_la1[i] = -1;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy