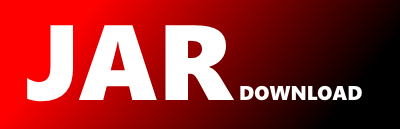
de.ipk_gatersleben.bit.bi.isa4j.components.Material Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of isa4J Show documentation
Show all versions of isa4J Show documentation
Fast and Scalable Java Library for Writing ISA-Tab Files
The newest version!
/**
* Copyright (c) 2020 Leibniz Institute of Plant Genetics and Crop Plant Research (IPK), Gatersleben, Germany.
* All rights reserved. This program and the accompanying materials are made available under the terms of the MIT License (https://spdx.org/licenses/MIT.html)
*
* Contributors:
* Leibniz Institute of Plant Genetics and Crop Plant Research (IPK), Gatersleben, Germany
*/
package de.ipk_gatersleben.bit.bi.isa4j.components;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import de.ipk_gatersleben.bit.bi.isa4j.util.StringUtil;
public class Material extends Source {
private String type;
public Material(String type, String name) {
super(name);
this.setType(type);
}
public Material(String type, String name, List characteristics) {
super(name, characteristics);
this.setType(type);
}
Map getFields() {
HashMap fields = new HashMap();
fields.put(this.type, new String[]{this.name});
fields.putAll(this.getFieldsForCharacteristics());
return fields;
}
LinkedHashMap getHeaders() {
LinkedHashMap headers = new LinkedHashMap();
headers.put(this.type, new String[]{this.type});
headers.putAll(this.getHeadersForCharacteristics());
return headers;
}
/**
* @return the type
*/
public String getType() {
return type;
}
/**
* @param type the type to set
*/
public void setType(String type) {
this.type = StringUtil.sanitize(Objects.requireNonNull(type, "Type cannot be null"));
}
@Override
public String toString() {
return " '" + this.name + "'";
}
}