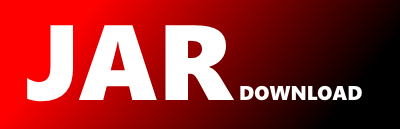
de.is24.deadcode4j.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deadcode4j-maven-plugin Show documentation
Show all versions of deadcode4j-maven-plugin Show documentation
Finds unused classes of a project
package de.is24.deadcode4j;
import com.google.common.base.Function;
import com.google.common.collect.FluentIterable;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.project.MavenProject;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import static com.google.common.collect.Sets.newHashSet;
/**
* Provides convenience methods.
*
* @since 1.2.0
*/
public final class Utils {
private Utils() {}
/**
* Returns groupId:artifactId for the specified artifact.
*
* @since 2.0.0
*/
@Nonnull
public static String getKeyFor(@Nonnull Artifact artifact) {
return artifact.getGroupId() + ":" + artifact.getArtifactId();
}
/**
* Returns groupId:artifactId:version for the specified artifact.
*
* @since 2.0.0
*/
@Nonnull
public static String getVersionedKeyFor(@Nonnull Artifact artifact) {
return getKeyFor(artifact) + ":" + artifact.getVersion();
}
/**
* Returns groupId:artifactId for the specified project.
*
* @since 1.2.0
*/
@Nonnull
public static String getKeyFor(@Nonnull MavenProject project) {
return project.getGroupId() + ":" + project.getArtifactId();
}
/**
* Returns a Function
transforming a MavenProject
into it's
* {@link #getKeyFor(org.apache.maven.project.MavenProject) key representation}.
*
* @see #getKeyFor(org.apache.maven.project.MavenProject)
* @since 1.4
*/
@Nonnull
public static Function toKey() {
return new Function() {
@Override
public String apply(@Nullable MavenProject input) {
return input == null ? null : getKeyFor(input);
}
};
}
/**
* Returns {@code true} if the given collection is {@code null} or empty.
*
* @since 2.0.0
*/
public static boolean isEmpty(Collection> collection) {
return collection == null || collection.isEmpty();
}
/**
* Adds the given element to a collection if the element is not null
.
*
* @since 1.2.0
*/
public static boolean addIfNonNull(@Nonnull Collection collection, @Nullable E element) {
return element != null && collection.add(element);
}
/**
* Returns a map's value for the specified key or the given default value if the value is null
.
*
* @since 1.2.0
*/
public static V getValueOrDefault(Map map, K key, V defaultValue) {
V value = map.get(key);
return value != null ? value : defaultValue;
}
/**
* Retrieves an existing Set
being mapped by the specified key or puts a new one into the map.
*
* @since 1.4
*/
public static Set getOrAddMappedSet(Map> map, K key) {
Set values = map.get(key);
if (values == null) {
values = newHashSet();
map.put(key, values);
}
return values;
}
/**
* Returns the given Iterable
or an empty list if it is null
as a {@link FluentIterable}.
*
* @since 2.0.0
*/
@Nonnull
public static FluentIterable emptyIfNull(@Nullable Iterable iterable) {
return FluentIterable.from(iterable == null ? Collections.emptyList() : iterable);
}
/**
* Returns {@code null} if the given String is {@code null} or empty; or the argument otherwise.
*
* @since 2.0.0
*/
@Nullable
public static String nullIfEmpty(@Nullable String string) {
return string == null || "".equals(string.trim()) ? null : string;
}
/**
* A properly annotated implementation of {@link com.google.common.base.Preconditions#checkNotNull(Object)}.
*
* @since 2.0.0
*/
@Nonnull
@SuppressFBWarnings(value = "NP_PARAMETER_MUST_BE_NONNULL_BUT_MARKED_AS_NULLABLE")
public static T checkNotNull(@Nullable T reference) {
if (reference == null) {
throw new NullPointerException();
}
return reference;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy