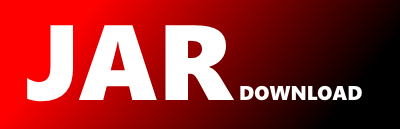
de.is24.guava.SequentialLoadingCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deadcode4j-maven-plugin Show documentation
Show all versions of deadcode4j-maven-plugin Show documentation
Finds unused classes of a project
package de.is24.guava;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.cache.AbstractLoadingCache;
import com.google.common.collect.Maps;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.ExecutionException;
/**
* The SequentialLoadingCache
is a LoadingCache
that is intended for single-threaded access.
* It is based on a {@link java.util.HashMap} and caches null
values.
*
* @param the keys' type
* @param the values' type
* @since 2.0.0
*/
public class SequentialLoadingCache extends AbstractLoadingCache> {
@Nonnull
private final Map> cache;
@Nonnull
private final Function> cacheLoader;
/**
* Creates a SequentialLoadingCache
that uses the given function to load the values.
*
* @since 2.0.0
*/
protected SequentialLoadingCache(@Nonnull Map> cache, @Nonnull Function> cacheLoader) {
this.cache = cache;
this.cacheLoader = cacheLoader;
}
/**
* Creates a SequentialLoadingCache
that uses the given function to load the values.
*
* @since 2.0.0
*/
public SequentialLoadingCache(@Nonnull Function> cacheLoader) {
this(Maps.>newHashMap(), cacheLoader);
}
/**
* Creates a SequentialLoadingCache
that only caches one value.
*
* @see #SequentialLoadingCache(com.google.common.base.Function)
* @since 2.0.0
*/
public static SequentialLoadingCache createSingleValueCache(@Nonnull Function> cacheLoader) {
return new SequentialLoadingCache(new HashMap>() {
@Override
public Optional put(K key, Optional value) {
super.clear();
return super.put(key, value);
}
}, cacheLoader);
}
@Nonnull
@Override
public Optional get(@Nullable K key) throws ExecutionException {
Optional value = this.cache.get(key);
if (value == null) {
value = cacheLoader.apply(key);
if (value == null) {
value = Optional.absent();
}
this.cache.put(key, value);
}
return value;
}
@Nonnull
@Override
@SuppressWarnings("SuspiciousMethodCalls")
public Optional getIfPresent(@Nonnull Object key) {
final Optional value = this.cache.get(key);
return value == null ? Optional.absent() : value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy