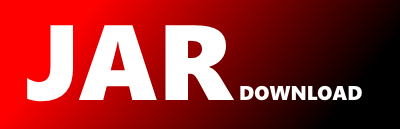
de.is24.javaparser.ImportDeclarations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deadcode4j-maven-plugin Show documentation
Show all versions of deadcode4j-maven-plugin Show documentation
Finds unused classes of a project
package de.is24.javaparser;
import com.google.common.base.Predicate;
import japa.parser.ast.ImportDeclaration;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import static de.is24.deadcode4j.Utils.checkNotNull;
/**
* Provides convenience methods for dealing with {@link japa.parser.ast.ImportDeclaration}s.
*
* @since 2.0.0
*/
public final class ImportDeclarations {
private ImportDeclarations() {}
/**
* Returns a Predicate
that evaluates to true
if the ImportDeclaration
being
* tested is an asterisk import.
*
* @since 2.0.0
*/
@Nonnull
public static Predicate super ImportDeclaration> isAsterisk() {
return new Predicate() {
@Override
@SuppressWarnings("ConstantConditions")
public boolean apply(@Nullable ImportDeclaration input) {
return checkNotNull(input).isAsterisk();
}
};
}
/**
* Returns a Predicate
that evaluates to true
if the ImportDeclaration
being
* tested is a static import.
*
* @since 2.0.0
*/
@Nonnull
public static Predicate super ImportDeclaration> isStatic() {
return new Predicate() {
@Override
@SuppressWarnings("ConstantConditions")
public boolean apply(@Nullable ImportDeclaration input) {
return checkNotNull(input).isStatic();
}
};
}
/**
* Returns a Predicate
that evaluates to true
if the last qualifier of the
* ImportDeclaration
being tested matches the given String.
*
* @since 2.0.0
*/
@Nonnull
public static Predicate super ImportDeclaration> refersTo(@Nonnull final String lastQualifier) {
return new Predicate() {
@Override
@SuppressWarnings("ConstantConditions")
public boolean apply(@Nullable ImportDeclaration input) {
return lastQualifier.equals(checkNotNull(input).getName().getName());
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy