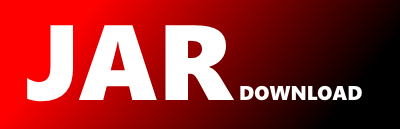
de.jakobjarosch.rethinkdb.orm.dao.GenericDAO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rethinkdb-orm Show documentation
Show all versions of rethinkdb-orm Show documentation
A lightweight OR mapper for RethinkDB, written in Java.
package de.jakobjarosch.rethinkdb.orm.dao;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.Joiner;
import com.rethinkdb.RethinkDB;
import com.rethinkdb.gen.ast.*;
import com.rethinkdb.gen.exc.ReqlDriverError;
import com.rethinkdb.net.Connection;
import com.rethinkdb.net.Cursor;
import de.jakobjarosch.rethinkdb.orm.model.ChangeFeedElement;
import de.jakobjarosch.rethinkdb.orm.model.IndexModel;
import rx.Observable;
import javax.inject.Provider;
import java.util.*;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
public class GenericDAO {
private static final RethinkDB R = RethinkDB.r;
private static final ObjectMapper MAPPER = new ObjectMapper();
private final Provider connection;
private final Class clazz;
private final String tableName;
private final String primaryKey;
private final Set indices = new HashSet<>();
public GenericDAO(Provider connection, Class clazz, String tableName, String primaryKey) {
this.connection = connection;
this.clazz = clazz;
this.tableName = tableName;
this.primaryKey = primaryKey;
}
public void addIndex(boolean geo, String fields) {
this.indices.add(new IndexModel(geo, fields.split(",")));
}
public void initTable() {
if (!hasTable(tableName)) {
R.tableCreate(tableName).optArg("primary_key", primaryKey).run(connection.get());
}
for (IndexModel index : indices) {
String indexName = Joiner.on("_").join(index.getFields());
if (!hasIndex(indexName)) {
IndexCreate indexCreate = R.table(tableName)
.indexCreate(indexName, row -> indexFieldsToReQL(row, index.getFields()));
if (index.isGeo()) {
indexCreate = indexCreate.optArg("geo", true);
}
indexCreate.run(connection.get());
}
}
}
public void create(T model) {
Map map = MAPPER.convertValue(model, Map.class);
R.table(tableName).insert(map).run(connection.get());
}
public List read() {
List