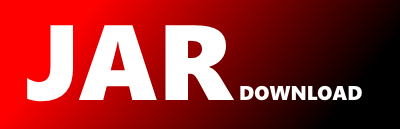
de.javagl.reflection.Constructors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reflection Show documentation
Show all versions of reflection Show documentation
Reflection utility classes
package de.javagl.reflection;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import de.javagl.reflection.InvokableParser.InvokableInfo;
/**
* Utility methods related to constructors.
*
*/
public class Constructors
{
/**
* Parse the constructor from the given string. The given string must be
* the string that is obtained from a constructor by calling
* {@link Constructor#toString()} or {@link Constructor#toGenericString()}.
*
* @param fullConstructorString The full constructor string
* @return The constructor
* @throws ReflectionException If the constructor can not be parsed
* for any reason. Either because the declaring class or any parameter
* class can not be found, or because the constructor is not found, or
* because the input string is otherwise invalid.
*/
public static Constructor> parseConstructorUnchecked(
String fullConstructorString)
{
InvokableInfo invokableInfo =
InvokableParser.parse(fullConstructorString);
// Fetch the fully qualified constructor name, which is of the form
// com.domain.ClassName
String className = invokableInfo.getFullyQualifiedName();
// Try to find the declaring class
Class> declaringClass = Types.parseTypeUnchecked(className);
// Finally, try to find the constructor
Class> parameterTypes[] = invokableInfo.getParameterTypes();
Constructor> result = getDeclaredConstructorUnchecked(
declaringClass, parameterTypes);
return result;
}
/**
* Delegates to {@link Constructor#newInstance(Object...)}.
*
* This call is wrapping all possible checked exceptions and
* SecurityExceptions into a {@link ReflectionException}
*
* @param The type of the constructor
* @param constructor The constructor
* @param arguments The arguments
* @return The new instance
* @throws ReflectionException if the call did not succeed
*/
public static T newInstanceUnchecked(
Constructor constructor, Object ... arguments)
{
try
{
T t = constructor.newInstance(arguments);
return t;
}
catch (IllegalArgumentException e)
{
throw new ReflectionException(e);
}
catch (InstantiationException e)
{
throw new ReflectionException(e);
}
catch (IllegalAccessException e)
{
throw new ReflectionException(e);
}
catch (InvocationTargetException e)
{
throw new ReflectionException(e);
}
catch (SecurityException e)
{
throw new ReflectionException(e);
}
}
/**
* Delegates to {@link Constructor#newInstance(Object...)}.
*
* Returns null
if the call did not succeed.
*
* @param The type of the constructor
* @param constructor The constructor
* @param arguments The arguments
* @return The new instance
* @throws ReflectionException if the call did not succeed
*/
public static T newInstanceOptional(
Constructor constructor, Object ... arguments)
{
try
{
T t = constructor.newInstance(arguments);
return t;
}
catch (IllegalArgumentException e)
{
return null;
}
catch (InstantiationException e)
{
return null;
}
catch (IllegalAccessException e)
{
return null;
}
catch (InvocationTargetException e)
{
return null;
}
catch (SecurityException e)
{
return null;
}
}
/**
* Delegates to {@link Constructor#newInstance(Object...)}.
*
* If the constructor is not accessible, then it will be made
* accessible for this call.
*
* This call is wrapping all possible checked exceptions and
* SecurityExceptions into a {@link ReflectionException}
*
* @param The type of the constructor
* @param constructor The constructor
* @param arguments The arguments
* @return The new instance
* @throws ReflectionException if the call did not succeed
*/
public static T newInstanceNonAccessibleUnchecked(
Constructor constructor, Object ... arguments)
{
boolean wasAccessible = constructor.isAccessible();
try
{
constructor.setAccessible(true);
T t = constructor.newInstance(arguments);
return t;
}
catch (IllegalArgumentException e)
{
throw new ReflectionException(e);
}
catch (InstantiationException e)
{
throw new ReflectionException(e);
}
catch (IllegalAccessException e)
{
throw new ReflectionException(e);
}
catch (InvocationTargetException e)
{
throw new ReflectionException(e);
}
catch (SecurityException e)
{
throw new ReflectionException(e);
}
finally
{
constructor.setAccessible(wasAccessible);
}
}
/**
* Delegates to {@link Constructor#newInstance(Object...)}.
*
* If the constructor is not accessible, then it will be made
* accessible for this call.
*
* Returns null
if the call did not succeed.
*
* @param The type of the constructor
* @param constructor The constructor
* @param arguments The arguments
* @return The new instance
*/
public static T newInstanceNonAccessibleOptional(
Constructor constructor, Object ... arguments)
{
boolean wasAccessible = constructor.isAccessible();
try
{
constructor.setAccessible(true);
T t = constructor.newInstance(arguments);
return t;
}
catch (IllegalArgumentException e)
{
return null;
}
catch (InstantiationException e)
{
return null;
}
catch (IllegalAccessException e)
{
return null;
}
catch (InvocationTargetException e)
{
return null;
}
catch (SecurityException e)
{
return null;
}
finally
{
constructor.setAccessible(wasAccessible);
}
}
/**
* Calls {@link Class#getConstructor(Class...)}.
*
* This call covers the following constructors:
*
* - public constructors
*
*
* This call is wrapping all possible checked exceptions and
* SecurityExceptions into a {@link ReflectionException}
*
* @param The type
* @param type The type
* @param parameterTypes The parameter types
* @return The constructor
* @throws ReflectionException if the call did not succeed
*/
public static Constructor getConstructorUnchecked(
Class type, Class>... parameterTypes)
{
try
{
return type.getConstructor(parameterTypes);
}
catch (SecurityException e)
{
throw new ReflectionException(e);
}
catch (NoSuchMethodException e)
{
throw new ReflectionException(e);
}
}
/**
* Calls {@link Class#getConstructor(Class...)}.
*
* This call covers the following constructors:
*
* - public constructors
*
*
* Returns null
if the call did not succeed.
*
* @param The type
* @param type The type
* @param parameterTypes The parameter types
* @return The constructor or null
*/
public static Constructor getConstructorOptional(
Class type, Class>... parameterTypes)
{
try
{
return type.getConstructor(parameterTypes);
}
catch (SecurityException e)
{
return null;
}
catch (NoSuchMethodException e)
{
return null;
}
}
/**
* Delegates to {@link Class#getConstructors()}, and returns the result
* as an unmodifiable list.
*
* This call covers the following constructors:
*
* - public constructors
*
*
* This call is wrapping all possible checked exceptions and
* SecurityExceptions into a {@link ReflectionException}
*
* @param type The type
* @return The constructors
* @throws ReflectionException if the call did not succeed
*/
public static List> getConstructorsUnchecked(Class> type)
{
try
{
return Collections.unmodifiableList(
Arrays.asList(type.getConstructors()));
}
catch (SecurityException e)
{
throw new ReflectionException(e);
}
}
/**
* Delegates to {@link Class#getConstructors()}, and returns the result
* as an unmodifiable list.
*
* This call covers the following constructors:
*
* - public constructors
*
*
* Returns an empty list if the underlying call did not succeed.
*
* @param type The type
* @return The constructors
*/
public static List> getConstructorsOptional(Class> type)
{
try
{
return Collections.unmodifiableList(
Arrays.asList(type.getConstructors()));
}
catch (SecurityException e)
{
return Collections.emptyList();
}
}
/**
* Calls {@link Class#getDeclaredConstructor(Class...)}.
*
* This call covers the following constructors:
*
* -
* public, protected, default,
* and private constructors
*
*
*
* This call is wrapping all possible checked exceptions and
* SecurityExceptions into a {@link ReflectionException}
*
* @param The type
* @param type The type
* @param parameterTypes The parameter types
* @return The constructor
* @throws ReflectionException if the call did not succeed
*/
public static Constructor getDeclaredConstructorUnchecked(
Class type, Class>... parameterTypes)
{
try
{
return type.getDeclaredConstructor(parameterTypes);
}
catch (SecurityException e)
{
throw new ReflectionException(e);
}
catch (NoSuchMethodException e)
{
throw new ReflectionException(e);
}
}
/**
* Calls {@link Class#getDeclaredConstructor(Class...)}.
*
* This call covers the following constructors:
*
* -
* public, protected, default,
* and private constructors
*
*
*
* Returns null
if the call did not succeed.
*
* @param The type
* @param type The type
* @param parameterTypes The parameter types
* @return The constructor or null
*/
public static Constructor getDeclaredConstructorOptional(
Class type, Class>... parameterTypes)
{
try
{
return type.getDeclaredConstructor(parameterTypes);
}
catch (SecurityException e)
{
return null;
}
catch (NoSuchMethodException e)
{
return null;
}
}
/**
* Delegates to {@link Class#getDeclaredConstructors()}, and returns the
* result as an unmodifiable list.
*
* This call covers the following constructors:
*
* -
* public, protected, default,
* and private constructors
*
*
*
* This call is wrapping all possible checked exceptions and
* SecurityExceptions into a {@link ReflectionException}
*
* @param type The type
* @return The constructors
* @throws ReflectionException if the call did not succeed
*/
public static List> getDeclaredConstructorsUnchecked(
Class> type)
{
try
{
return Collections.unmodifiableList(
Arrays.asList(type.getDeclaredConstructors()));
}
catch (SecurityException e)
{
throw new ReflectionException(e);
}
}
/**
* Delegates to {@link Class#getDeclaredConstructors()}, and returns the
* result as an unmodifiable list.
*
* This call covers the following constructors:
*
* -
* public, protected, default,
* and private constructors
*
*
*
* Returns an empty list if the underlying call did not succeed.
*
* @param type The type
* @return The constructors
*/
public static List> getDeclaredConstructorsOptional(
Class> type)
{
try
{
return Collections.unmodifiableList(
Arrays.asList(type.getDeclaredConstructors()));
}
catch (SecurityException e)
{
return Collections.emptyList();
}
}
/**
* Private constructor to prevent instantiation
*/
private Constructors()
{
// Private constructor to prevent instantiation
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy