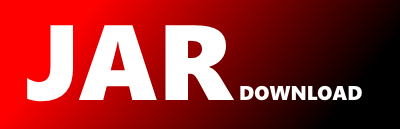
de.jball.sonar.hybris.java.checks.AvoidJalo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-hybris Show documentation
Show all versions of sonar-hybris Show documentation
SonarQube Rules for Hybris
package de.jball.sonar.hybris.java.checks;
import org.apache.commons.collections4.CollectionUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.sonar.check.Rule;
import org.sonar.plugins.java.api.IssuableSubscriptionVisitor;
import org.sonar.plugins.java.api.semantic.Symbol;
import org.sonar.plugins.java.api.semantic.Type;
import org.sonar.plugins.java.api.tree.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.EnumSet;
import java.util.List;
import java.util.Set;
@Rule(key = "AvoidJalo")
public class AvoidJalo extends IssuableSubscriptionVisitor {
private static final Set NODES_TO_VISIT = EnumSet.of(
Tree.Kind.METHOD,
Tree.Kind.METHOD_INVOCATION,
Tree.Kind.VARIABLE);
@Override
public List nodesToVisit() {
return Collections.unmodifiableList(new ArrayList<>(NODES_TO_VISIT));
}
@Override
public void visitNode(final Tree tree) {
switch (tree.kind()) {
case METHOD:
visitMethod((MethodTree) tree);
break;
case VARIABLE:
visitVariable((VariableTree) tree);
break;
case METHOD_INVOCATION:
visitMethodInvocation((MethodInvocationTree) tree);
break;
default:
throw new UnsupportedOperationException("Unknown tree kind: " + tree.kind().name());
}
}
private void visitVariable(final VariableTree variable) {
if (isJaloType(variable.type().symbolType())) {
reportJalo(variable);
}
}
private void visitMethod(final MethodTree method) {
// Arguments are handled by #visitVariable
if (methodHasJaloReturnType(method.symbol())) {
reportJalo(method);
}
}
private void visitMethodInvocation(MethodInvocationTree methodInvocation) {
if (!methodInvocation.symbol().isMethodSymbol() || methodInvocation.symbol().isUnknown()) {
return;
}
if (methodHasJaloReturnType((Symbol.MethodSymbol) methodInvocation.symbol()) //
|| methodHasJaloParameter((Symbol.MethodSymbol) methodInvocation.symbol())) {
reportJalo(methodInvocation.methodSelect());
} else if (methodInvocationWithJaloArg(methodInvocation)) {
reportJalo(methodInvocation);
} else if (methodReturnAssignedToJalo(methodInvocation)) {
reportJalo(methodInvocation);
}
}
private boolean methodHasJaloReturnType(final Symbol.MethodSymbol methodSymbol) {
return isJaloType(methodSymbol.returnType().type());
}
private boolean methodHasJaloParameter(final Symbol.MethodSymbol methodSymbol) {
return methodSymbol.parameterTypes().stream() //
.anyMatch(this::isJaloType);
}
private boolean methodInvocationWithJaloArg(MethodInvocationTree methodInvocation) {
return CollectionUtils.emptyIfNull(methodInvocation.arguments()).stream()
.map(ExpressionTree::symbolType)
.anyMatch(this::isJaloType);
}
private boolean methodReturnAssignedToJalo(MethodInvocationTree methodInvocation) {
return isJaloType(methodInvocation.symbolType());
}
private boolean isJaloType(final Type type) {
return type.isSubtypeOf("de.hybris.platform.jalo.Item");
}
private void reportJalo(final Tree tree) {
reportIssue(tree, "Avoid using jalo classes");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy