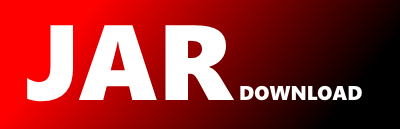
de.jsone_studios.wrapper.spotify.services.LibrarySpotifyService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotify-web-api-java Show documentation
Show all versions of spotify-web-api-java Show documentation
A Java wrapper for Spotify's Web API using Retrofit
The newest version!
package de.jsone_studios.wrapper.spotify.services;
import de.jsone_studios.wrapper.spotify.models.SavedAlbum;
import de.jsone_studios.wrapper.spotify.models.Pager;
import de.jsone_studios.wrapper.spotify.models.Result;
import de.jsone_studios.wrapper.spotify.models.SavedTrack;
import retrofit2.Call;
import retrofit2.http.*;
import java.util.Map;
public interface LibrarySpotifyService
{
/**
* Check if one or more albums is already saved in the current Spotify user’s “Your Music” library.
*
* @param ids A comma-separated list of the Spotify IDs for the albums. Maximum: 50 IDs.
* @return An array with boolean values that indicate whether the albums are in the current Spotify user’s “Your Music” library.
* @see Check User’s Saved Albums
*/
@GET("me/albums/contains")
Call containsMySavedAlbums(@Query("ids") String ids);
/**
* Check if one or more tracks is already saved in the current Spotify user’s “Your Music” library.
*
* @param ids A comma-separated list of the Spotify IDs for the tracks. Maximum: 50 IDs.
* @return An array with boolean values that indicate whether the tracks are in the current Spotify user’s “Your Music” library.
* @see Check User’s Saved Tracks
*/
@GET("me/tracks/contains")
Call containsMySavedTracks(@Query("ids") String ids);
/**
* Get a list of the albums saved in the current Spotify user’s “Your Music” library.
*
* @return A paginated list of saved albums
* @see Get a User’s Saved Albums
*/
@GET("me/albums")
Call> getMySavedAlbums();
/**
* Get a list of the albums saved in the current Spotify user’s “Your Music” library.
*
* @param options Optional parameters. For list of supported parameters see
* endpoint documentation
* @return A paginated list of saved albums
* @see Get a User’s Saved Albums
*/
@GET("me/albums")
Call> getMySavedAlbums(@QueryMap Map options);
/**
* Get a list of the songs saved in the current Spotify user’s “Your Music” library.
*
* @return A paginated list of saved tracks
* @see Get a User’s Saved Tracks
*/
@GET("me/tracks")
Call> getMySavedTracks();
/**
* Get a list of the songs saved in the current Spotify user’s “Your Music” library.
*
* @param options Optional parameters. For list of supported parameters see
* endpoint documentation
* @return A paginated list of saved tracks
* @see Get a User’s Saved Tracks
*/
@GET("me/tracks")
Call> getMySavedTracks(@QueryMap Map options);
/**
* Remove one or more albums from the current user’s “Your Music” library.
*
* @param ids A comma-separated list of the Spotify IDs for the albums
* @return An empty result
* @see Remove User’s Saved Albums
*/
@DELETE("me/albums")
Call removeFromMySavedAlbums(@Query("ids") String ids);
/**
* Remove one or more albums from the current user’s “Your Music” library.
*
* @param ids A list of the Spotify IDs for the albums
* @return An empty result
* @see Remove User’s Saved Albums
*/
@DELETE("me/albums")
Call removeFromMySavedAlbums(@Body String[] ids);
/**
* Remove one or more tracks from the current user’s “Your Music” library.
*
* @param ids A comma-separated list of the Spotify IDs for the tracks
* @return An empty result
* @see Remove User’s Saved Tracks
*/
@DELETE("me/tracks")
Call removeFromMySavedTracks(@Query("ids") String ids);
/**
* Remove one or more tracks from the current user’s “Your Music” library.
*
* @param ids A list of the Spotify IDs for the tracks
* @return An empty result
* @see Remove User’s Saved Tracks
*/
@DELETE("me/tracks")
Call removeFromMySavedTracks(@Body String[] ids);
/**
* Save one or more albums to the current user’s “Your Music” library.
*
* @param ids A comma-separated list of the Spotify IDs for the albums
* @return An empty result
* @see Save Albums for User
*/
@PUT("me/albums")
Call addToMySavedAlbums(@Query("ids") String ids);
/**
* Save one or more albums to the current user’s “Your Music” library.
*
* @param ids A list of the Spotify IDs for the albums
* @return An empty result
* @see Save Albums for User
*/
@PUT("me/albums")
Call addToMySavedAlbums(@Body String[] ids);
/**
* Save one or more tracks to the current user’s “Your Music” library.
*
* @param ids A comma-separated list of the Spotify IDs for the tracks
* @return An empty result
* @see Save Tracks for User
*/
@PUT("me/tracks")
Call addToMySavedTracks(@Query("ids") String ids);
/**
* Save one or more tracks to the current user’s “Your Music” library.
*
* @param ids A list of the Spotify IDs for the tracks
* @return An empty result
* @see Save Tracks for User
*/
@PUT("me/tracks")
Call addToMySavedTracks(@Body String[] ids);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy