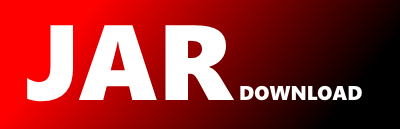
de.jsone_studios.wrapper.spotify.services.TracksSpotifyService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotify-web-api-java Show documentation
Show all versions of spotify-web-api-java Show documentation
A Java wrapper for Spotify's Web API using Retrofit
The newest version!
package de.jsone_studios.wrapper.spotify.services;
import de.jsone_studios.wrapper.spotify.models.*;
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Path;
import retrofit2.http.Query;
import retrofit2.http.QueryMap;
import java.util.Map;
public interface TracksSpotifyService
{
/**
* Get a detailed audio analysis for a single track identified by its unique Spotify ID.
*
* @param id The Spotify ID for the track.
* @return Audio analysis object
* @see Get Audio Analysis for a Track
*/
@GET("audio-analysis/{id}")
Call getTrackAudioAnalysis(@Path("id") String id);
/**
* Get audio feature information for a single track identified by its unique Spotify ID.
*
* @param id The Spotify ID for the track.
* @return Audio features object
* @see Get Audio Features for a Track
*/
@GET("audio-features/{id}")
Call getTrackAudioFeatures(@Path("id") String id);
/**
* Get audio features for multiple tracks based on their Spotify IDs.
*
* @param ids A comma-separated list of the Spotify IDs for the tracks. Maximum: 100 IDs
* @return An object whose key is "audio_features" and whose value is an array of audio features objects.
* @see Get Audio Features for Several Tracks
*/
@GET("audio-features")
Call getTracksAudioFeatures(@Query("ids") String ids);
/**
* Get Spotify catalog information for a single track identified by their unique Spotify ID.
*
* @param trackId The Spotify ID for the track.
* @return Requested track information
* @see Get a Track
*/
@GET("tracks/{id}")
Call
© 2015 - 2025 Weber Informatics LLC | Privacy Policy