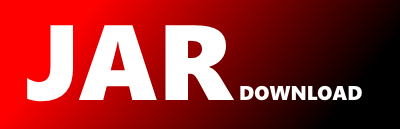
com.aliasi.xml.XHtmlWriter Maven / Gradle / Ivy
Show all versions of aliasi-lingpipe Show documentation
/*
* LingPipe v. 4.1.0
* Copyright (C) 2003-2011 Alias-i
*
* This program is licensed under the Alias-i Royalty Free License
* Version 1 WITHOUT ANY WARRANTY, without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the Alias-i
* Royalty Free License Version 1 for more details.
*
* You should have received a copy of the Alias-i Royalty Free License
* Version 1 along with this program; if not, visit
* http://alias-i.com/lingpipe/licenses/lingpipe-license-1.txt or contact
* Alias-i, Inc. at 181 North 11th Street, Suite 401, Brooklyn, NY 11211,
* +1 (718) 290-9170.
*/
package com.aliasi.xml;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
/**
* An XHtmlWriter
provides constants and convenience
* methods for generating strict XHTML 1.0 output. This class includes
* the Strict DTD specification:
*
*
*
* <!DOCTYPE html
* PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
* "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
*
*
*
* The XML version and decoding declaration will be included as in
* the parent class {@link SAXWriter}, as in:
*
*
*
* <?xml version="1.0" encoding="UTF-8"?>
*
*
*
* The parent class SAXWriter
is set to
* use XHTML mode (see {@link SAXWriter#SAXWriter(OutputStream,String,boolean)})
* output.
*
*
It is up to the application to supply an appropriate document
* structure and to validate any output. For instance, the top-level
* element must be html
, and it must specify the
* appropriate namespace and language, as in:
*
*
*
* <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
*
*
*
* A constant is provided for each legal XHTML element tag and each
* XHTML attribute. There are six convenience methods supplied to
* start each element. The first five methods allow from zero to four
* pairs of attributes and values to be supplied as strings. The
* sixth method accepts an arbitrary SAX {@link Attributes} object to
* supply the attributes. Elements must be closed using the
* underlying SAXWriter's end methods, such as {@link
* #endSimpleElement(String)}.
*
*
Like for SAXWriter, the underlying output stream must be closed
* by the calling application after the {@link #endDocument()} method
* is called.
*
*
Warning: No well-formedness checks are performed on the
* output. In particular, elements may not be balanced leading to
* invalid XML, or elements may not provide attributes matching the
* XHTML 1.0 specification. We suggest validating HTML using the W3C
* validator.
*
*
* - Official XHTML 1.0 Specification (from W3C)
*
- W3C XHTML Validator
* - W3 Schools XHTML Tutorial
(W3 Schools is not affiliated with the W3C -- they're
* a small Norwegian outfit)
*
*
* @author Bob Carpenter
* @version 2.3.1
* @since LingPipe2.3.1
*/
public class XHtmlWriter extends SAXWriter {
public XHtmlWriter(OutputStream out, String charsetName)
throws UnsupportedEncodingException {
super(out,charsetName,XHTML_MODE);
setDTDString(XHTML_1_0_STRICT_DTD);
}
public XHtmlWriter() {
super(XHTML_MODE);
setDTDString(XHTML_1_0_STRICT_DTD);
}
/**
* Constant for element a
.
*/
public static final String A = "a";
/**
* Start an a
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void a() throws SAXException {
a(EMPTY_ATTS);
}
/**
* Start an a
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void a(Attributes atts) throws SAXException {
startSimpleElement(A,atts);
}
/**
* Start an a
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void a(String att, String val) throws SAXException {
startSimpleElement(A,att,val);
}
/**
* Start an a
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void a(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(A,att1,val1,att2,val2);
}
/**
* Start an a
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void a(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(A,att1,val1,att2,val2,att3,val3);
}
/**
* Start an a
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void a(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(A,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element abbr
.
*/
public static final String ABBR = "abbr";
/**
* Start an abbr
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void abbr() throws SAXException {
abbr(EMPTY_ATTS);
}
/**
* Start an abbr
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void abbr(Attributes atts) throws SAXException {
startSimpleElement(ABBR,atts);
}
/**
* Start an abbr
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void abbr(String att, String val) throws SAXException {
startSimpleElement(ABBR,att,val);
}
/**
* Start an abbr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void abbr(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(ABBR,att1,val1,att2,val2);
}
/**
* Start an abbr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void abbr(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(ABBR,att1,val1,att2,val2,att3,val3);
}
/**
* Start an abbr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void abbr(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(ABBR,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element acronym
.
*/
public static final String ACRONYM = "acronym";
/**
* Start an acronym
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void acronym() throws SAXException {
acronym(EMPTY_ATTS);
}
/**
* Start an acronym
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void acronym(Attributes atts) throws SAXException {
startSimpleElement(ACRONYM,atts);
}
/**
* Start an acronym
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void acronym(String att, String val) throws SAXException {
startSimpleElement(ACRONYM,att,val);
}
/**
* Start an acronym
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void acronym(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(ACRONYM,att1,val1,att2,val2);
}
/**
* Start an acronym
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void acronym(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(ACRONYM,att1,val1,att2,val2,att3,val3);
}
/**
* Start an acronym
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void acronym(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(ACRONYM,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element address
.
*/
public static final String ADDRESS = "address";
/**
* Start an address
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void address() throws SAXException {
address(EMPTY_ATTS);
}
/**
* Start an address
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void address(Attributes atts) throws SAXException {
startSimpleElement(ADDRESS,atts);
}
/**
* Start an address
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void address(String att, String val) throws SAXException {
startSimpleElement(ADDRESS,att,val);
}
/**
* Start an address
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void address(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(ADDRESS,att1,val1,att2,val2);
}
/**
* Start an address
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void address(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(ADDRESS,att1,val1,att2,val2,att3,val3);
}
/**
* Start an address
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void address(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(ADDRESS,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element applet
.
*/
public static final String APPLET = "applet";
/**
* Start an applet
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void applet() throws SAXException {
applet(EMPTY_ATTS);
}
/**
* Start an applet
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void applet(Attributes atts) throws SAXException {
startSimpleElement(APPLET,atts);
}
/**
* Start an applet
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void applet(String att, String val) throws SAXException {
startSimpleElement(APPLET,att,val);
}
/**
* Start an applet
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void applet(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(APPLET,att1,val1,att2,val2);
}
/**
* Start an applet
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void applet(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(APPLET,att1,val1,att2,val2,att3,val3);
}
/**
* Start an applet
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void applet(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(APPLET,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element area
.
*/
public static final String AREA = "area";
/**
* Start an area
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void area() throws SAXException {
area(EMPTY_ATTS);
}
/**
* Start an area
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void area(Attributes atts) throws SAXException {
startSimpleElement(AREA,atts);
}
/**
* Start an area
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void area(String att, String val) throws SAXException {
startSimpleElement(AREA,att,val);
}
/**
* Start an area
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void area(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(AREA,att1,val1,att2,val2);
}
/**
* Start an area
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void area(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(AREA,att1,val1,att2,val2,att3,val3);
}
/**
* Start an area
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void area(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(AREA,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element b
.
*/
public static final String B = "b";
/**
* Start an b
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void b() throws SAXException {
b(EMPTY_ATTS);
}
/**
* Start an b
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void b(Attributes atts) throws SAXException {
startSimpleElement(B,atts);
}
/**
* Start an b
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void b(String att, String val) throws SAXException {
startSimpleElement(B,att,val);
}
/**
* Start an b
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void b(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(B,att1,val1,att2,val2);
}
/**
* Start an b
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void b(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(B,att1,val1,att2,val2,att3,val3);
}
/**
* Start an b
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void b(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(B,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element base
.
*/
public static final String BASE = "base";
/**
* Start an base
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void base() throws SAXException {
base(EMPTY_ATTS);
}
/**
* Start an base
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void base(Attributes atts) throws SAXException {
startSimpleElement(BASE,atts);
}
/**
* Start an base
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void base(String att, String val) throws SAXException {
startSimpleElement(BASE,att,val);
}
/**
* Start an base
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void base(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BASE,att1,val1,att2,val2);
}
/**
* Start an base
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void base(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BASE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an base
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void base(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BASE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element basefont
.
*/
public static final String BASEFONT = "basefont";
/**
* Start an basefont
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void basefont() throws SAXException {
basefont(EMPTY_ATTS);
}
/**
* Start an basefont
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void basefont(Attributes atts) throws SAXException {
startSimpleElement(BASEFONT,atts);
}
/**
* Start an basefont
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void basefont(String att, String val) throws SAXException {
startSimpleElement(BASEFONT,att,val);
}
/**
* Start an basefont
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void basefont(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BASEFONT,att1,val1,att2,val2);
}
/**
* Start an basefont
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void basefont(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BASEFONT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an basefont
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void basefont(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BASEFONT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element bdo
.
*/
public static final String BDO = "bdo";
/**
* Start an bdo
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void bdo() throws SAXException {
bdo(EMPTY_ATTS);
}
/**
* Start an bdo
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void bdo(Attributes atts) throws SAXException {
startSimpleElement(BDO,atts);
}
/**
* Start an bdo
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void bdo(String att, String val) throws SAXException {
startSimpleElement(BDO,att,val);
}
/**
* Start an bdo
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void bdo(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BDO,att1,val1,att2,val2);
}
/**
* Start an bdo
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void bdo(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BDO,att1,val1,att2,val2,att3,val3);
}
/**
* Start an bdo
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void bdo(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BDO,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element big
.
*/
public static final String BIG = "big";
/**
* Start an big
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void big() throws SAXException {
big(EMPTY_ATTS);
}
/**
* Start an big
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void big(Attributes atts) throws SAXException {
startSimpleElement(BIG,atts);
}
/**
* Start an big
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void big(String att, String val) throws SAXException {
startSimpleElement(BIG,att,val);
}
/**
* Start an big
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void big(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BIG,att1,val1,att2,val2);
}
/**
* Start an big
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void big(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BIG,att1,val1,att2,val2,att3,val3);
}
/**
* Start an big
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void big(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BIG,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element blockquote
.
*/
public static final String BLOCKQUOTE = "blockquote";
/**
* Start an blockquote
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void blockquote() throws SAXException {
blockquote(EMPTY_ATTS);
}
/**
* Start an blockquote
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void blockquote(Attributes atts) throws SAXException {
startSimpleElement(BLOCKQUOTE,atts);
}
/**
* Start an blockquote
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void blockquote(String att, String val) throws SAXException {
startSimpleElement(BLOCKQUOTE,att,val);
}
/**
* Start an blockquote
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void blockquote(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BLOCKQUOTE,att1,val1,att2,val2);
}
/**
* Start an blockquote
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void blockquote(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BLOCKQUOTE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an blockquote
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void blockquote(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BLOCKQUOTE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element body
.
*/
public static final String BODY = "body";
/**
* Start an body
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void body() throws SAXException {
body(EMPTY_ATTS);
}
/**
* Start an body
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void body(Attributes atts) throws SAXException {
startSimpleElement(BODY,atts);
}
/**
* Start an body
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void body(String att, String val) throws SAXException {
startSimpleElement(BODY,att,val);
}
/**
* Start an body
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void body(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BODY,att1,val1,att2,val2);
}
/**
* Start an body
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void body(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BODY,att1,val1,att2,val2,att3,val3);
}
/**
* Start an body
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void body(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BODY,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element br
.
*/
public static final String BR = "br";
/**
* Start an br
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void br() throws SAXException {
br(EMPTY_ATTS);
}
/**
* Start an br
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void br(Attributes atts) throws SAXException {
startSimpleElement(BR,atts);
}
/**
* Start an br
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void br(String att, String val) throws SAXException {
startSimpleElement(BR,att,val);
}
/**
* Start an br
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void br(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BR,att1,val1,att2,val2);
}
/**
* Start an br
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void br(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BR,att1,val1,att2,val2,att3,val3);
}
/**
* Start an br
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void br(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BR,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element button
.
*/
public static final String BUTTON = "button";
/**
* Start an button
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void button() throws SAXException {
button(EMPTY_ATTS);
}
/**
* Start an button
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void button(Attributes atts) throws SAXException {
startSimpleElement(BUTTON,atts);
}
/**
* Start an button
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void button(String att, String val) throws SAXException {
startSimpleElement(BUTTON,att,val);
}
/**
* Start an button
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void button(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(BUTTON,att1,val1,att2,val2);
}
/**
* Start an button
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void button(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(BUTTON,att1,val1,att2,val2,att3,val3);
}
/**
* Start an button
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void button(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(BUTTON,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element caption
.
*/
public static final String CAPTION = "caption";
/**
* Start an caption
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void caption() throws SAXException {
caption(EMPTY_ATTS);
}
/**
* Start an caption
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void caption(Attributes atts) throws SAXException {
startSimpleElement(CAPTION,atts);
}
/**
* Start an caption
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void caption(String att, String val) throws SAXException {
startSimpleElement(CAPTION,att,val);
}
/**
* Start an caption
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void caption(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(CAPTION,att1,val1,att2,val2);
}
/**
* Start an caption
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void caption(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(CAPTION,att1,val1,att2,val2,att3,val3);
}
/**
* Start an caption
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void caption(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(CAPTION,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element center
.
*/
public static final String CENTER = "center";
/**
* Start an center
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void center() throws SAXException {
center(EMPTY_ATTS);
}
/**
* Start an center
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void center(Attributes atts) throws SAXException {
startSimpleElement(CENTER,atts);
}
/**
* Start an center
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void center(String att, String val) throws SAXException {
startSimpleElement(CENTER,att,val);
}
/**
* Start an center
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void center(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(CENTER,att1,val1,att2,val2);
}
/**
* Start an center
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void center(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(CENTER,att1,val1,att2,val2,att3,val3);
}
/**
* Start an center
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void center(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(CENTER,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element cite
.
*/
public static final String CITE = "cite";
/**
* Start an cite
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void cite() throws SAXException {
cite(EMPTY_ATTS);
}
/**
* Start an cite
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void cite(Attributes atts) throws SAXException {
startSimpleElement(CITE,atts);
}
/**
* Start an cite
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void cite(String att, String val) throws SAXException {
startSimpleElement(CITE,att,val);
}
/**
* Start an cite
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void cite(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(CITE,att1,val1,att2,val2);
}
/**
* Start an cite
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void cite(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(CITE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an cite
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void cite(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(CITE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element code
.
*/
public static final String CODE = "code";
/**
* Start an code
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void code() throws SAXException {
code(EMPTY_ATTS);
}
/**
* Start an code
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void code(Attributes atts) throws SAXException {
startSimpleElement(CODE,atts);
}
/**
* Start an code
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void code(String att, String val) throws SAXException {
startSimpleElement(CODE,att,val);
}
/**
* Start an code
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void code(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(CODE,att1,val1,att2,val2);
}
/**
* Start an code
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void code(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(CODE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an code
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void code(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(CODE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element col
.
*/
public static final String COL = "col";
/**
* Start an col
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void col() throws SAXException {
col(EMPTY_ATTS);
}
/**
* Start an col
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void col(Attributes atts) throws SAXException {
startSimpleElement(COL,atts);
}
/**
* Start an col
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void col(String att, String val) throws SAXException {
startSimpleElement(COL,att,val);
}
/**
* Start an col
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void col(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(COL,att1,val1,att2,val2);
}
/**
* Start an col
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void col(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(COL,att1,val1,att2,val2,att3,val3);
}
/**
* Start an col
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void col(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(COL,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element colgroup
.
*/
public static final String COLGROUP = "colgroup";
/**
* Start an colgroup
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void colgroup() throws SAXException {
colgroup(EMPTY_ATTS);
}
/**
* Start an colgroup
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void colgroup(Attributes atts) throws SAXException {
startSimpleElement(COLGROUP,atts);
}
/**
* Start an colgroup
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void colgroup(String att, String val) throws SAXException {
startSimpleElement(COLGROUP,att,val);
}
/**
* Start an colgroup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void colgroup(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(COLGROUP,att1,val1,att2,val2);
}
/**
* Start an colgroup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void colgroup(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(COLGROUP,att1,val1,att2,val2,att3,val3);
}
/**
* Start an colgroup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void colgroup(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(COLGROUP,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element dd
.
*/
public static final String DD = "dd";
/**
* Start an dd
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void dd() throws SAXException {
dd(EMPTY_ATTS);
}
/**
* Start an dd
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dd(Attributes atts) throws SAXException {
startSimpleElement(DD,atts);
}
/**
* Start an dd
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dd(String att, String val) throws SAXException {
startSimpleElement(DD,att,val);
}
/**
* Start an dd
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dd(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(DD,att1,val1,att2,val2);
}
/**
* Start an dd
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dd(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(DD,att1,val1,att2,val2,att3,val3);
}
/**
* Start an dd
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dd(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(DD,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element del
.
*/
public static final String DEL = "del";
/**
* Start an del
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void del() throws SAXException {
del(EMPTY_ATTS);
}
/**
* Start an del
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void del(Attributes atts) throws SAXException {
startSimpleElement(DEL,atts);
}
/**
* Start an del
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void del(String att, String val) throws SAXException {
startSimpleElement(DEL,att,val);
}
/**
* Start an del
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void del(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(DEL,att1,val1,att2,val2);
}
/**
* Start an del
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void del(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(DEL,att1,val1,att2,val2,att3,val3);
}
/**
* Start an del
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void del(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(DEL,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element dfn
.
*/
public static final String DFN = "dfn";
/**
* Start an dfn
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void dfn() throws SAXException {
dfn(EMPTY_ATTS);
}
/**
* Start an dfn
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dfn(Attributes atts) throws SAXException {
startSimpleElement(DFN,atts);
}
/**
* Start an dfn
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dfn(String att, String val) throws SAXException {
startSimpleElement(DFN,att,val);
}
/**
* Start an dfn
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dfn(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(DFN,att1,val1,att2,val2);
}
/**
* Start an dfn
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dfn(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(DFN,att1,val1,att2,val2,att3,val3);
}
/**
* Start an dfn
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dfn(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(DFN,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element dir
.
*/
public static final String DIR = "dir";
/**
* Start an dir
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void dir() throws SAXException {
dir(EMPTY_ATTS);
}
/**
* Start an dir
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dir(Attributes atts) throws SAXException {
startSimpleElement(DIR,atts);
}
/**
* Start an dir
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dir(String att, String val) throws SAXException {
startSimpleElement(DIR,att,val);
}
/**
* Start an dir
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dir(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(DIR,att1,val1,att2,val2);
}
/**
* Start an dir
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dir(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(DIR,att1,val1,att2,val2,att3,val3);
}
/**
* Start an dir
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dir(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(DIR,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element div
.
*/
public static final String DIV = "div";
/**
* Start an div
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void div() throws SAXException {
div(EMPTY_ATTS);
}
/**
* Start an div
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void div(Attributes atts) throws SAXException {
startSimpleElement(DIV,atts);
}
/**
* Start an div
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void div(String att, String val) throws SAXException {
startSimpleElement(DIV,att,val);
}
/**
* Start an div
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void div(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(DIV,att1,val1,att2,val2);
}
/**
* Start an div
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void div(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(DIV,att1,val1,att2,val2,att3,val3);
}
/**
* Start an div
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void div(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(DIV,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element dl
.
*/
public static final String DL = "dl";
/**
* Start an dl
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void dl() throws SAXException {
dl(EMPTY_ATTS);
}
/**
* Start an dl
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dl(Attributes atts) throws SAXException {
startSimpleElement(DL,atts);
}
/**
* Start an dl
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dl(String att, String val) throws SAXException {
startSimpleElement(DL,att,val);
}
/**
* Start an dl
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dl(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(DL,att1,val1,att2,val2);
}
/**
* Start an dl
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dl(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(DL,att1,val1,att2,val2,att3,val3);
}
/**
* Start an dl
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dl(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(DL,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element dt
.
*/
public static final String DT = "dt";
/**
* Start an dt
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void dt() throws SAXException {
dt(EMPTY_ATTS);
}
/**
* Start an dt
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dt(Attributes atts) throws SAXException {
startSimpleElement(DT,atts);
}
/**
* Start an dt
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dt(String att, String val) throws SAXException {
startSimpleElement(DT,att,val);
}
/**
* Start an dt
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dt(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(DT,att1,val1,att2,val2);
}
/**
* Start an dt
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dt(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(DT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an dt
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void dt(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(DT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element em
.
*/
public static final String EM = "em";
/**
* Start an em
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void em() throws SAXException {
em(EMPTY_ATTS);
}
/**
* Start an em
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void em(Attributes atts) throws SAXException {
startSimpleElement(EM,atts);
}
/**
* Start an em
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void em(String att, String val) throws SAXException {
startSimpleElement(EM,att,val);
}
/**
* Start an em
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void em(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(EM,att1,val1,att2,val2);
}
/**
* Start an em
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void em(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(EM,att1,val1,att2,val2,att3,val3);
}
/**
* Start an em
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void em(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(EM,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element fieldset
.
*/
public static final String FIELDSET = "fieldset";
/**
* Start an fieldset
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void fieldset() throws SAXException {
fieldset(EMPTY_ATTS);
}
/**
* Start an fieldset
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void fieldset(Attributes atts) throws SAXException {
startSimpleElement(FIELDSET,atts);
}
/**
* Start an fieldset
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void fieldset(String att, String val) throws SAXException {
startSimpleElement(FIELDSET,att,val);
}
/**
* Start an fieldset
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void fieldset(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(FIELDSET,att1,val1,att2,val2);
}
/**
* Start an fieldset
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void fieldset(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(FIELDSET,att1,val1,att2,val2,att3,val3);
}
/**
* Start an fieldset
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void fieldset(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(FIELDSET,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element font
.
*/
public static final String FONT = "font";
/**
* Start an font
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void font() throws SAXException {
font(EMPTY_ATTS);
}
/**
* Start an font
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void font(Attributes atts) throws SAXException {
startSimpleElement(FONT,atts);
}
/**
* Start an font
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void font(String att, String val) throws SAXException {
startSimpleElement(FONT,att,val);
}
/**
* Start an font
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void font(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(FONT,att1,val1,att2,val2);
}
/**
* Start an font
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void font(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(FONT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an font
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void font(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(FONT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element form
.
*/
public static final String FORM = "form";
/**
* Start an form
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void form() throws SAXException {
form(EMPTY_ATTS);
}
/**
* Start an form
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void form(Attributes atts) throws SAXException {
startSimpleElement(FORM,atts);
}
/**
* Start an form
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void form(String att, String val) throws SAXException {
startSimpleElement(FORM,att,val);
}
/**
* Start an form
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void form(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(FORM,att1,val1,att2,val2);
}
/**
* Start an form
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void form(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(FORM,att1,val1,att2,val2,att3,val3);
}
/**
* Start an form
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void form(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(FORM,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element frame
.
*/
public static final String FRAME = "frame";
/**
* Start an frame
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void frame() throws SAXException {
frame(EMPTY_ATTS);
}
/**
* Start an frame
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frame(Attributes atts) throws SAXException {
startSimpleElement(FRAME,atts);
}
/**
* Start an frame
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frame(String att, String val) throws SAXException {
startSimpleElement(FRAME,att,val);
}
/**
* Start an frame
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frame(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(FRAME,att1,val1,att2,val2);
}
/**
* Start an frame
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frame(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(FRAME,att1,val1,att2,val2,att3,val3);
}
/**
* Start an frame
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frame(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(FRAME,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element frameset
.
*/
public static final String FRAMESET = "frameset";
/**
* Start an frameset
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void frameset() throws SAXException {
frameset(EMPTY_ATTS);
}
/**
* Start an frameset
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frameset(Attributes atts) throws SAXException {
startSimpleElement(FRAMESET,atts);
}
/**
* Start an frameset
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frameset(String att, String val) throws SAXException {
startSimpleElement(FRAMESET,att,val);
}
/**
* Start an frameset
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frameset(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(FRAMESET,att1,val1,att2,val2);
}
/**
* Start an frameset
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frameset(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(FRAMESET,att1,val1,att2,val2,att3,val3);
}
/**
* Start an frameset
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void frameset(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(FRAMESET,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element head
.
*/
public static final String HEAD = "head";
/**
* Start an head
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void head() throws SAXException {
head(EMPTY_ATTS);
}
/**
* Start an head
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void head(Attributes atts) throws SAXException {
startSimpleElement(HEAD,atts);
}
/**
* Start an head
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void head(String att, String val) throws SAXException {
startSimpleElement(HEAD,att,val);
}
/**
* Start an head
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void head(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(HEAD,att1,val1,att2,val2);
}
/**
* Start an head
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void head(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(HEAD,att1,val1,att2,val2,att3,val3);
}
/**
* Start an head
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void head(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(HEAD,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element h1
.
*/
public static final String H1 = "h1";
/**
* Start an h1
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void h1() throws SAXException {
h1(EMPTY_ATTS);
}
/**
* Start an h1
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h1(Attributes atts) throws SAXException {
startSimpleElement(H1,atts);
}
/**
* Start an h1
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h1(String att, String val) throws SAXException {
startSimpleElement(H1,att,val);
}
/**
* Start an h1
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h1(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(H1,att1,val1,att2,val2);
}
/**
* Start an h1
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h1(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(H1,att1,val1,att2,val2,att3,val3);
}
/**
* Start an h1
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h1(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(H1,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element h2
.
*/
public static final String H2 = "h2";
/**
* Start an h2
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void h2() throws SAXException {
h2(EMPTY_ATTS);
}
/**
* Start an h2
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h2(Attributes atts) throws SAXException {
startSimpleElement(H2,atts);
}
/**
* Start an h2
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h2(String att, String val) throws SAXException {
startSimpleElement(H2,att,val);
}
/**
* Start an h2
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h2(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(H2,att1,val1,att2,val2);
}
/**
* Start an h2
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h2(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(H2,att1,val1,att2,val2,att3,val3);
}
/**
* Start an h2
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h2(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(H2,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element h3
.
*/
public static final String H3 = "h3";
/**
* Start an h3
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void h3() throws SAXException {
h3(EMPTY_ATTS);
}
/**
* Start an h3
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h3(Attributes atts) throws SAXException {
startSimpleElement(H3,atts);
}
/**
* Start an h3
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h3(String att, String val) throws SAXException {
startSimpleElement(H3,att,val);
}
/**
* Start an h3
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h3(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(H3,att1,val1,att2,val2);
}
/**
* Start an h3
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h3(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(H3,att1,val1,att2,val2,att3,val3);
}
/**
* Start an h3
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h3(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(H3,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element h4
.
*/
public static final String H4 = "h4";
/**
* Start an h4
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void h4() throws SAXException {
h4(EMPTY_ATTS);
}
/**
* Start an h4
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h4(Attributes atts) throws SAXException {
startSimpleElement(H4,atts);
}
/**
* Start an h4
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h4(String att, String val) throws SAXException {
startSimpleElement(H4,att,val);
}
/**
* Start an h4
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h4(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(H4,att1,val1,att2,val2);
}
/**
* Start an h4
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h4(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(H4,att1,val1,att2,val2,att3,val3);
}
/**
* Start an h4
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h4(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(H4,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element h5
.
*/
public static final String H5 = "h5";
/**
* Start an h5
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void h5() throws SAXException {
h5(EMPTY_ATTS);
}
/**
* Start an h5
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h5(Attributes atts) throws SAXException {
startSimpleElement(H5,atts);
}
/**
* Start an h5
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h5(String att, String val) throws SAXException {
startSimpleElement(H5,att,val);
}
/**
* Start an h5
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h5(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(H5,att1,val1,att2,val2);
}
/**
* Start an h5
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h5(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(H5,att1,val1,att2,val2,att3,val3);
}
/**
* Start an h5
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h5(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(H5,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element h6
.
*/
public static final String H6 = "h6";
/**
* Start an h6
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void h6() throws SAXException {
h6(EMPTY_ATTS);
}
/**
* Start an h6
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h6(Attributes atts) throws SAXException {
startSimpleElement(H6,atts);
}
/**
* Start an h6
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h6(String att, String val) throws SAXException {
startSimpleElement(H6,att,val);
}
/**
* Start an h6
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h6(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(H6,att1,val1,att2,val2);
}
/**
* Start an h6
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h6(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(H6,att1,val1,att2,val2,att3,val3);
}
/**
* Start an h6
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void h6(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(H6,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element hr
.
*/
public static final String HR = "hr";
/**
* Start an hr
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void hr() throws SAXException {
hr(EMPTY_ATTS);
}
/**
* Start an hr
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void hr(Attributes atts) throws SAXException {
startSimpleElement(HR,atts);
}
/**
* Start an hr
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void hr(String att, String val) throws SAXException {
startSimpleElement(HR,att,val);
}
/**
* Start an hr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void hr(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(HR,att1,val1,att2,val2);
}
/**
* Start an hr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void hr(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(HR,att1,val1,att2,val2,att3,val3);
}
/**
* Start an hr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void hr(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(HR,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element html
.
*/
public static final String HTML = "html";
/**
* Start an html
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void html() throws SAXException {
html(EMPTY_ATTS);
}
/**
* Start an html
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void html(Attributes atts) throws SAXException {
startSimpleElement(HTML,atts);
}
/**
* Start an html
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void html(String att, String val) throws SAXException {
startSimpleElement(HTML,att,val);
}
/**
* Start an html
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void html(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(HTML,att1,val1,att2,val2);
}
/**
* Start an html
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void html(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(HTML,att1,val1,att2,val2,att3,val3);
}
/**
* Start an html
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void html(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(HTML,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element i
.
*/
public static final String I = "i";
/**
* Start an i
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void i() throws SAXException {
i(EMPTY_ATTS);
}
/**
* Start an i
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void i(Attributes atts) throws SAXException {
startSimpleElement(I,atts);
}
/**
* Start an i
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void i(String att, String val) throws SAXException {
startSimpleElement(I,att,val);
}
/**
* Start an i
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void i(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(I,att1,val1,att2,val2);
}
/**
* Start an i
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void i(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(I,att1,val1,att2,val2,att3,val3);
}
/**
* Start an i
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void i(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(I,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element iframe
.
*/
public static final String IFRAME = "iframe";
/**
* Start an iframe
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void iframe() throws SAXException {
iframe(EMPTY_ATTS);
}
/**
* Start an iframe
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void iframe(Attributes atts) throws SAXException {
startSimpleElement(IFRAME,atts);
}
/**
* Start an iframe
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void iframe(String att, String val) throws SAXException {
startSimpleElement(IFRAME,att,val);
}
/**
* Start an iframe
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void iframe(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(IFRAME,att1,val1,att2,val2);
}
/**
* Start an iframe
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void iframe(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(IFRAME,att1,val1,att2,val2,att3,val3);
}
/**
* Start an iframe
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void iframe(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(IFRAME,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element img
.
*/
public static final String IMG = "img";
/**
* Start an img
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void img() throws SAXException {
img(EMPTY_ATTS);
}
/**
* Start an img
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void img(Attributes atts) throws SAXException {
startSimpleElement(IMG,atts);
}
/**
* Start an img
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void img(String att, String val) throws SAXException {
startSimpleElement(IMG,att,val);
}
/**
* Start an img
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void img(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(IMG,att1,val1,att2,val2);
}
/**
* Start an img
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void img(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(IMG,att1,val1,att2,val2,att3,val3);
}
/**
* Start an img
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void img(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(IMG,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element input
.
*/
public static final String INPUT = "input";
/**
* Start an input
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void input() throws SAXException {
input(EMPTY_ATTS);
}
/**
* Start an input
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void input(Attributes atts) throws SAXException {
startSimpleElement(INPUT,atts);
}
/**
* Start an input
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void input(String att, String val) throws SAXException {
startSimpleElement(INPUT,att,val);
}
/**
* Start an input
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void input(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(INPUT,att1,val1,att2,val2);
}
/**
* Start an input
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void input(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(INPUT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an input
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void input(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(INPUT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element ins
.
*/
public static final String INS = "ins";
/**
* Start an ins
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void ins() throws SAXException {
ins(EMPTY_ATTS);
}
/**
* Start an ins
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ins(Attributes atts) throws SAXException {
startSimpleElement(INS,atts);
}
/**
* Start an ins
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ins(String att, String val) throws SAXException {
startSimpleElement(INS,att,val);
}
/**
* Start an ins
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ins(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(INS,att1,val1,att2,val2);
}
/**
* Start an ins
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ins(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(INS,att1,val1,att2,val2,att3,val3);
}
/**
* Start an ins
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ins(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(INS,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element kbd
.
*/
public static final String KBD = "kbd";
/**
* Start an kbd
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void kbd() throws SAXException {
kbd(EMPTY_ATTS);
}
/**
* Start an kbd
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void kbd(Attributes atts) throws SAXException {
startSimpleElement(KBD,atts);
}
/**
* Start an kbd
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void kbd(String att, String val) throws SAXException {
startSimpleElement(KBD,att,val);
}
/**
* Start an kbd
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void kbd(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(KBD,att1,val1,att2,val2);
}
/**
* Start an kbd
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void kbd(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(KBD,att1,val1,att2,val2,att3,val3);
}
/**
* Start an kbd
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void kbd(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(KBD,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element label
.
*/
public static final String LABEL = "label";
/**
* Start an label
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void label() throws SAXException {
label(EMPTY_ATTS);
}
/**
* Start an label
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void label(Attributes atts) throws SAXException {
startSimpleElement(LABEL,atts);
}
/**
* Start an label
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void label(String att, String val) throws SAXException {
startSimpleElement(LABEL,att,val);
}
/**
* Start an label
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void label(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(LABEL,att1,val1,att2,val2);
}
/**
* Start an label
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void label(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(LABEL,att1,val1,att2,val2,att3,val3);
}
/**
* Start an label
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void label(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(LABEL,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element legend
.
*/
public static final String LEGEND = "legend";
/**
* Start an legend
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void legend() throws SAXException {
legend(EMPTY_ATTS);
}
/**
* Start an legend
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void legend(Attributes atts) throws SAXException {
startSimpleElement(LEGEND,atts);
}
/**
* Start an legend
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void legend(String att, String val) throws SAXException {
startSimpleElement(LEGEND,att,val);
}
/**
* Start an legend
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void legend(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(LEGEND,att1,val1,att2,val2);
}
/**
* Start an legend
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void legend(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(LEGEND,att1,val1,att2,val2,att3,val3);
}
/**
* Start an legend
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void legend(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(LEGEND,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element li
.
*/
public static final String LI = "li";
/**
* Start an li
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void li() throws SAXException {
li(EMPTY_ATTS);
}
/**
* Start an li
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void li(Attributes atts) throws SAXException {
startSimpleElement(LI,atts);
}
/**
* Start an li
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void li(String att, String val) throws SAXException {
startSimpleElement(LI,att,val);
}
/**
* Start an li
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void li(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(LI,att1,val1,att2,val2);
}
/**
* Start an li
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void li(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(LI,att1,val1,att2,val2,att3,val3);
}
/**
* Start an li
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void li(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(LI,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element link
.
*/
public static final String LINK = "link";
/**
* Start an link
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void link() throws SAXException {
link(EMPTY_ATTS);
}
/**
* Start an link
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void link(Attributes atts) throws SAXException {
startSimpleElement(LINK,atts);
}
/**
* Start an link
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void link(String att, String val) throws SAXException {
startSimpleElement(LINK,att,val);
}
/**
* Start an link
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void link(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(LINK,att1,val1,att2,val2);
}
/**
* Start an link
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void link(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(LINK,att1,val1,att2,val2,att3,val3);
}
/**
* Start an link
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void link(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(LINK,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element map
.
*/
public static final String MAP = "map";
/**
* Start an map
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void map() throws SAXException {
map(EMPTY_ATTS);
}
/**
* Start an map
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void map(Attributes atts) throws SAXException {
startSimpleElement(MAP,atts);
}
/**
* Start an map
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void map(String att, String val) throws SAXException {
startSimpleElement(MAP,att,val);
}
/**
* Start an map
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void map(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(MAP,att1,val1,att2,val2);
}
/**
* Start an map
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void map(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(MAP,att1,val1,att2,val2,att3,val3);
}
/**
* Start an map
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void map(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(MAP,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element menu
.
*/
public static final String MENU = "menu";
/**
* Start an menu
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void menu() throws SAXException {
menu(EMPTY_ATTS);
}
/**
* Start an menu
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void menu(Attributes atts) throws SAXException {
startSimpleElement(MENU,atts);
}
/**
* Start an menu
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void menu(String att, String val) throws SAXException {
startSimpleElement(MENU,att,val);
}
/**
* Start an menu
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void menu(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(MENU,att1,val1,att2,val2);
}
/**
* Start an menu
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void menu(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(MENU,att1,val1,att2,val2,att3,val3);
}
/**
* Start an menu
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void menu(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(MENU,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element meta
.
*/
public static final String META = "meta";
/**
* Start an meta
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void meta() throws SAXException {
meta(EMPTY_ATTS);
}
/**
* Start an meta
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void meta(Attributes atts) throws SAXException {
startSimpleElement(META,atts);
}
/**
* Start an meta
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void meta(String att, String val) throws SAXException {
startSimpleElement(META,att,val);
}
/**
* Start an meta
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void meta(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(META,att1,val1,att2,val2);
}
/**
* Start an meta
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void meta(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(META,att1,val1,att2,val2,att3,val3);
}
/**
* Start an meta
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void meta(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(META,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element noframes
.
*/
public static final String NOFRAMES = "noframes";
/**
* Start an noframes
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void noframes() throws SAXException {
noframes(EMPTY_ATTS);
}
/**
* Start an noframes
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noframes(Attributes atts) throws SAXException {
startSimpleElement(NOFRAMES,atts);
}
/**
* Start an noframes
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noframes(String att, String val) throws SAXException {
startSimpleElement(NOFRAMES,att,val);
}
/**
* Start an noframes
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noframes(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(NOFRAMES,att1,val1,att2,val2);
}
/**
* Start an noframes
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noframes(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(NOFRAMES,att1,val1,att2,val2,att3,val3);
}
/**
* Start an noframes
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noframes(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(NOFRAMES,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element noscript
.
*/
public static final String NOSCRIPT = "noscript";
/**
* Start an noscript
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void noscript() throws SAXException {
noscript(EMPTY_ATTS);
}
/**
* Start an noscript
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noscript(Attributes atts) throws SAXException {
startSimpleElement(NOSCRIPT,atts);
}
/**
* Start an noscript
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noscript(String att, String val) throws SAXException {
startSimpleElement(NOSCRIPT,att,val);
}
/**
* Start an noscript
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noscript(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(NOSCRIPT,att1,val1,att2,val2);
}
/**
* Start an noscript
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noscript(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(NOSCRIPT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an noscript
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void noscript(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(NOSCRIPT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element object
.
*/
public static final String OBJECT = "object";
/**
* Start an object
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void object() throws SAXException {
object(EMPTY_ATTS);
}
/**
* Start an object
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void object(Attributes atts) throws SAXException {
startSimpleElement(OBJECT,atts);
}
/**
* Start an object
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void object(String att, String val) throws SAXException {
startSimpleElement(OBJECT,att,val);
}
/**
* Start an object
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void object(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(OBJECT,att1,val1,att2,val2);
}
/**
* Start an object
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void object(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(OBJECT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an object
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void object(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(OBJECT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element ol
.
*/
public static final String OL = "ol";
/**
* Start an ol
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void ol() throws SAXException {
ol(EMPTY_ATTS);
}
/**
* Start an ol
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ol(Attributes atts) throws SAXException {
startSimpleElement(OL,atts);
}
/**
* Start an ol
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ol(String att, String val) throws SAXException {
startSimpleElement(OL,att,val);
}
/**
* Start an ol
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ol(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(OL,att1,val1,att2,val2);
}
/**
* Start an ol
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ol(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(OL,att1,val1,att2,val2,att3,val3);
}
/**
* Start an ol
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ol(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(OL,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element optgroup
.
*/
public static final String OPTGROUP = "optgroup";
/**
* Start an optgroup
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void optgroup() throws SAXException {
optgroup(EMPTY_ATTS);
}
/**
* Start an optgroup
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void optgroup(Attributes atts) throws SAXException {
startSimpleElement(OPTGROUP,atts);
}
/**
* Start an optgroup
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void optgroup(String att, String val) throws SAXException {
startSimpleElement(OPTGROUP,att,val);
}
/**
* Start an optgroup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void optgroup(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(OPTGROUP,att1,val1,att2,val2);
}
/**
* Start an optgroup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void optgroup(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(OPTGROUP,att1,val1,att2,val2,att3,val3);
}
/**
* Start an optgroup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void optgroup(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(OPTGROUP,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element option
.
*/
public static final String OPTION = "option";
/**
* Start an option
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void option() throws SAXException {
option(EMPTY_ATTS);
}
/**
* Start an option
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void option(Attributes atts) throws SAXException {
startSimpleElement(OPTION,atts);
}
/**
* Start an option
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void option(String att, String val) throws SAXException {
startSimpleElement(OPTION,att,val);
}
/**
* Start an option
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void option(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(OPTION,att1,val1,att2,val2);
}
/**
* Start an option
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void option(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(OPTION,att1,val1,att2,val2,att3,val3);
}
/**
* Start an option
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void option(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(OPTION,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element p
.
*/
public static final String P = "p";
/**
* Start an p
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void p() throws SAXException {
p(EMPTY_ATTS);
}
/**
* Start an p
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void p(Attributes atts) throws SAXException {
startSimpleElement(P,atts);
}
/**
* Start an p
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void p(String att, String val) throws SAXException {
startSimpleElement(P,att,val);
}
/**
* Start an p
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void p(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(P,att1,val1,att2,val2);
}
/**
* Start an p
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void p(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(P,att1,val1,att2,val2,att3,val3);
}
/**
* Start an p
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void p(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(P,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element param
.
*/
public static final String PARAM = "param";
/**
* Start an param
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void param() throws SAXException {
param(EMPTY_ATTS);
}
/**
* Start an param
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void param(Attributes atts) throws SAXException {
startSimpleElement(PARAM,atts);
}
/**
* Start an param
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void param(String att, String val) throws SAXException {
startSimpleElement(PARAM,att,val);
}
/**
* Start an param
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void param(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(PARAM,att1,val1,att2,val2);
}
/**
* Start an param
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void param(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(PARAM,att1,val1,att2,val2,att3,val3);
}
/**
* Start an param
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void param(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(PARAM,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element pre
.
*/
public static final String PRE = "pre";
/**
* Start an pre
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void pre() throws SAXException {
pre(EMPTY_ATTS);
}
/**
* Start an pre
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void pre(Attributes atts) throws SAXException {
startSimpleElement(PRE,atts);
}
/**
* Start an pre
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void pre(String att, String val) throws SAXException {
startSimpleElement(PRE,att,val);
}
/**
* Start an pre
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void pre(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(PRE,att1,val1,att2,val2);
}
/**
* Start an pre
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void pre(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(PRE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an pre
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void pre(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(PRE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element q
.
*/
public static final String Q = "q";
/**
* Start an q
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void q() throws SAXException {
q(EMPTY_ATTS);
}
/**
* Start an q
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void q(Attributes atts) throws SAXException {
startSimpleElement(Q,atts);
}
/**
* Start an q
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void q(String att, String val) throws SAXException {
startSimpleElement(Q,att,val);
}
/**
* Start an q
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void q(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(Q,att1,val1,att2,val2);
}
/**
* Start an q
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void q(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(Q,att1,val1,att2,val2,att3,val3);
}
/**
* Start an q
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void q(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(Q,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element s
.
*/
public static final String S = "s";
/**
* Start an s
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void s() throws SAXException {
s(EMPTY_ATTS);
}
/**
* Start an s
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void s(Attributes atts) throws SAXException {
startSimpleElement(S,atts);
}
/**
* Start an s
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void s(String att, String val) throws SAXException {
startSimpleElement(S,att,val);
}
/**
* Start an s
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void s(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(S,att1,val1,att2,val2);
}
/**
* Start an s
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void s(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(S,att1,val1,att2,val2,att3,val3);
}
/**
* Start an s
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void s(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(S,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element samp
.
*/
public static final String SAMP = "samp";
/**
* Start an samp
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void samp() throws SAXException {
samp(EMPTY_ATTS);
}
/**
* Start an samp
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void samp(Attributes atts) throws SAXException {
startSimpleElement(SAMP,atts);
}
/**
* Start an samp
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void samp(String att, String val) throws SAXException {
startSimpleElement(SAMP,att,val);
}
/**
* Start an samp
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void samp(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(SAMP,att1,val1,att2,val2);
}
/**
* Start an samp
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void samp(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(SAMP,att1,val1,att2,val2,att3,val3);
}
/**
* Start an samp
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void samp(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(SAMP,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element script
.
*/
public static final String SCRIPT = "script";
/**
* Start an script
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void script() throws SAXException {
script(EMPTY_ATTS);
}
/**
* Start an script
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void script(Attributes atts) throws SAXException {
startSimpleElement(SCRIPT,atts);
}
/**
* Start an script
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void script(String att, String val) throws SAXException {
startSimpleElement(SCRIPT,att,val);
}
/**
* Start an script
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void script(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(SCRIPT,att1,val1,att2,val2);
}
/**
* Start an script
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void script(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(SCRIPT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an script
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void script(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(SCRIPT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element select
.
*/
public static final String SELECT = "select";
/**
* Start an select
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void select() throws SAXException {
select(EMPTY_ATTS);
}
/**
* Start an select
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void select(Attributes atts) throws SAXException {
startSimpleElement(SELECT,atts);
}
/**
* Start an select
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void select(String att, String val) throws SAXException {
startSimpleElement(SELECT,att,val);
}
/**
* Start an select
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void select(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(SELECT,att1,val1,att2,val2);
}
/**
* Start an select
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void select(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(SELECT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an select
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void select(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(SELECT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element small
.
*/
public static final String SMALL = "small";
/**
* Start an small
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void small() throws SAXException {
small(EMPTY_ATTS);
}
/**
* Start an small
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void small(Attributes atts) throws SAXException {
startSimpleElement(SMALL,atts);
}
/**
* Start an small
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void small(String att, String val) throws SAXException {
startSimpleElement(SMALL,att,val);
}
/**
* Start an small
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void small(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(SMALL,att1,val1,att2,val2);
}
/**
* Start an small
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void small(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(SMALL,att1,val1,att2,val2,att3,val3);
}
/**
* Start an small
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void small(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(SMALL,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element span
.
*/
public static final String SPAN = "span";
/**
* Start an span
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void span() throws SAXException {
span(EMPTY_ATTS);
}
/**
* Start an span
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void span(Attributes atts) throws SAXException {
startSimpleElement(SPAN,atts);
}
/**
* Start an span
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void span(String att, String val) throws SAXException {
startSimpleElement(SPAN,att,val);
}
/**
* Start an span
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void span(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(SPAN,att1,val1,att2,val2);
}
/**
* Start an span
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void span(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(SPAN,att1,val1,att2,val2,att3,val3);
}
/**
* Start an span
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void span(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(SPAN,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element strike
.
*/
public static final String STRIKE = "strike";
/**
* Start an strike
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void strike() throws SAXException {
strike(EMPTY_ATTS);
}
/**
* Start an strike
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strike(Attributes atts) throws SAXException {
startSimpleElement(STRIKE,atts);
}
/**
* Start an strike
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strike(String att, String val) throws SAXException {
startSimpleElement(STRIKE,att,val);
}
/**
* Start an strike
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strike(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(STRIKE,att1,val1,att2,val2);
}
/**
* Start an strike
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strike(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(STRIKE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an strike
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strike(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(STRIKE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element strong
.
*/
public static final String STRONG = "strong";
/**
* Start an strong
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void strong() throws SAXException {
strong(EMPTY_ATTS);
}
/**
* Start an strong
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strong(Attributes atts) throws SAXException {
startSimpleElement(STRONG,atts);
}
/**
* Start an strong
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strong(String att, String val) throws SAXException {
startSimpleElement(STRONG,att,val);
}
/**
* Start an strong
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strong(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(STRONG,att1,val1,att2,val2);
}
/**
* Start an strong
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strong(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(STRONG,att1,val1,att2,val2,att3,val3);
}
/**
* Start an strong
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void strong(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(STRONG,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element style
.
*/
public static final String STYLE = "style";
/**
* Start an style
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void style() throws SAXException {
style(EMPTY_ATTS);
}
/**
* Start an style
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void style(Attributes atts) throws SAXException {
startSimpleElement(STYLE,atts);
}
/**
* Start an style
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void style(String att, String val) throws SAXException {
startSimpleElement(STYLE,att,val);
}
/**
* Start an style
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void style(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(STYLE,att1,val1,att2,val2);
}
/**
* Start an style
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void style(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(STYLE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an style
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void style(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(STYLE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element sub
.
*/
public static final String SUB = "sub";
/**
* Start an sub
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void sub() throws SAXException {
sub(EMPTY_ATTS);
}
/**
* Start an sub
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sub(Attributes atts) throws SAXException {
startSimpleElement(SUB,atts);
}
/**
* Start an sub
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sub(String att, String val) throws SAXException {
startSimpleElement(SUB,att,val);
}
/**
* Start an sub
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sub(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(SUB,att1,val1,att2,val2);
}
/**
* Start an sub
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sub(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(SUB,att1,val1,att2,val2,att3,val3);
}
/**
* Start an sub
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sub(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(SUB,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element sup
.
*/
public static final String SUP = "sup";
/**
* Start an sup
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void sup() throws SAXException {
sup(EMPTY_ATTS);
}
/**
* Start an sup
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sup(Attributes atts) throws SAXException {
startSimpleElement(SUP,atts);
}
/**
* Start an sup
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sup(String att, String val) throws SAXException {
startSimpleElement(SUP,att,val);
}
/**
* Start an sup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sup(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(SUP,att1,val1,att2,val2);
}
/**
* Start an sup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sup(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(SUP,att1,val1,att2,val2,att3,val3);
}
/**
* Start an sup
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void sup(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(SUP,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element table
.
*/
public static final String TABLE = "table";
/**
* Start an table
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void table() throws SAXException {
table(EMPTY_ATTS);
}
/**
* Start an table
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void table(Attributes atts) throws SAXException {
startSimpleElement(TABLE,atts);
}
/**
* Start an table
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void table(String att, String val) throws SAXException {
startSimpleElement(TABLE,att,val);
}
/**
* Start an table
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void table(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TABLE,att1,val1,att2,val2);
}
/**
* Start an table
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void table(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TABLE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an table
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void table(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TABLE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element tbody
.
*/
public static final String TBODY = "tbody";
/**
* Start an tbody
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void tbody() throws SAXException {
tbody(EMPTY_ATTS);
}
/**
* Start an tbody
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tbody(Attributes atts) throws SAXException {
startSimpleElement(TBODY,atts);
}
/**
* Start an tbody
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tbody(String att, String val) throws SAXException {
startSimpleElement(TBODY,att,val);
}
/**
* Start an tbody
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tbody(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TBODY,att1,val1,att2,val2);
}
/**
* Start an tbody
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tbody(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TBODY,att1,val1,att2,val2,att3,val3);
}
/**
* Start an tbody
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tbody(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TBODY,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element td
.
*/
public static final String TD = "td";
/**
* Start an td
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void td() throws SAXException {
td(EMPTY_ATTS);
}
/**
* Start an td
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void td(Attributes atts) throws SAXException {
startSimpleElement(TD,atts);
}
/**
* Start an td
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void td(String att, String val) throws SAXException {
startSimpleElement(TD,att,val);
}
/**
* Start an td
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void td(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TD,att1,val1,att2,val2);
}
/**
* Start an td
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void td(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TD,att1,val1,att2,val2,att3,val3);
}
/**
* Start an td
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void td(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TD,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element textarea
.
*/
public static final String TEXTAREA = "textarea";
/**
* Start an textarea
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void textarea() throws SAXException {
textarea(EMPTY_ATTS);
}
/**
* Start an textarea
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void textarea(Attributes atts) throws SAXException {
startSimpleElement(TEXTAREA,atts);
}
/**
* Start an textarea
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void textarea(String att, String val) throws SAXException {
startSimpleElement(TEXTAREA,att,val);
}
/**
* Start an textarea
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void textarea(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TEXTAREA,att1,val1,att2,val2);
}
/**
* Start an textarea
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void textarea(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TEXTAREA,att1,val1,att2,val2,att3,val3);
}
/**
* Start an textarea
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void textarea(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TEXTAREA,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element tfoot
.
*/
public static final String TFOOT = "tfoot";
/**
* Start an tfoot
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void tfoot() throws SAXException {
tfoot(EMPTY_ATTS);
}
/**
* Start an tfoot
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tfoot(Attributes atts) throws SAXException {
startSimpleElement(TFOOT,atts);
}
/**
* Start an tfoot
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tfoot(String att, String val) throws SAXException {
startSimpleElement(TFOOT,att,val);
}
/**
* Start an tfoot
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tfoot(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TFOOT,att1,val1,att2,val2);
}
/**
* Start an tfoot
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tfoot(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TFOOT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an tfoot
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tfoot(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TFOOT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element th
.
*/
public static final String TH = "th";
/**
* Start an th
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void th() throws SAXException {
th(EMPTY_ATTS);
}
/**
* Start an th
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void th(Attributes atts) throws SAXException {
startSimpleElement(TH,atts);
}
/**
* Start an th
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void th(String att, String val) throws SAXException {
startSimpleElement(TH,att,val);
}
/**
* Start an th
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void th(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TH,att1,val1,att2,val2);
}
/**
* Start an th
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void th(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TH,att1,val1,att2,val2,att3,val3);
}
/**
* Start an th
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void th(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TH,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element thead
.
*/
public static final String THEAD = "thead";
/**
* Start an thead
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void thead() throws SAXException {
thead(EMPTY_ATTS);
}
/**
* Start an thead
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void thead(Attributes atts) throws SAXException {
startSimpleElement(THEAD,atts);
}
/**
* Start an thead
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void thead(String att, String val) throws SAXException {
startSimpleElement(THEAD,att,val);
}
/**
* Start an thead
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void thead(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(THEAD,att1,val1,att2,val2);
}
/**
* Start an thead
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void thead(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(THEAD,att1,val1,att2,val2,att3,val3);
}
/**
* Start an thead
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void thead(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(THEAD,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element title
.
*/
public static final String TITLE = "title";
/**
* Start an title
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void title() throws SAXException {
title(EMPTY_ATTS);
}
/**
* Start an title
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void title(Attributes atts) throws SAXException {
startSimpleElement(TITLE,atts);
}
/**
* Start an title
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void title(String att, String val) throws SAXException {
startSimpleElement(TITLE,att,val);
}
/**
* Start an title
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void title(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TITLE,att1,val1,att2,val2);
}
/**
* Start an title
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void title(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TITLE,att1,val1,att2,val2,att3,val3);
}
/**
* Start an title
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void title(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TITLE,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element tr
.
*/
public static final String TR = "tr";
/**
* Start an tr
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void tr() throws SAXException {
tr(EMPTY_ATTS);
}
/**
* Start an tr
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tr(Attributes atts) throws SAXException {
startSimpleElement(TR,atts);
}
/**
* Start an tr
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tr(String att, String val) throws SAXException {
startSimpleElement(TR,att,val);
}
/**
* Start an tr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tr(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TR,att1,val1,att2,val2);
}
/**
* Start an tr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tr(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TR,att1,val1,att2,val2,att3,val3);
}
/**
* Start an tr
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tr(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TR,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element tt
.
*/
public static final String TT = "tt";
/**
* Start an tt
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void tt() throws SAXException {
tt(EMPTY_ATTS);
}
/**
* Start an tt
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tt(Attributes atts) throws SAXException {
startSimpleElement(TT,atts);
}
/**
* Start an tt
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tt(String att, String val) throws SAXException {
startSimpleElement(TT,att,val);
}
/**
* Start an tt
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tt(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(TT,att1,val1,att2,val2);
}
/**
* Start an tt
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tt(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(TT,att1,val1,att2,val2,att3,val3);
}
/**
* Start an tt
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void tt(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(TT,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element u
.
*/
public static final String U = "u";
/**
* Start an u
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void u() throws SAXException {
u(EMPTY_ATTS);
}
/**
* Start an u
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void u(Attributes atts) throws SAXException {
startSimpleElement(U,atts);
}
/**
* Start an u
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void u(String att, String val) throws SAXException {
startSimpleElement(U,att,val);
}
/**
* Start an u
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void u(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(U,att1,val1,att2,val2);
}
/**
* Start an u
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void u(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(U,att1,val1,att2,val2,att3,val3);
}
/**
* Start an u
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void u(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(U,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element ul
.
*/
public static final String UL = "ul";
/**
* Start an ul
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void ul() throws SAXException {
ul(EMPTY_ATTS);
}
/**
* Start an ul
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ul(Attributes atts) throws SAXException {
startSimpleElement(UL,atts);
}
/**
* Start an ul
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ul(String att, String val) throws SAXException {
startSimpleElement(UL,att,val);
}
/**
* Start an ul
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ul(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(UL,att1,val1,att2,val2);
}
/**
* Start an ul
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ul(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(UL,att1,val1,att2,val2,att3,val3);
}
/**
* Start an ul
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void ul(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(UL,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* Constant for element var
.
*/
public static final String VAR = "var";
/**
* Start an var
element with no attributes.
*
* @throws SAXException If there is an underlying SAX exception.
*/
public void var() throws SAXException {
var(EMPTY_ATTS);
}
/**
* Start an var
element with the specified
* attributes.
*
* @param atts Attributes for element.
* @throws SAXException If there is an underlying SAX exception.
*/
public void var(Attributes atts) throws SAXException {
startSimpleElement(VAR,atts);
}
/**
* Start an var
element with the specified
* attribute and value.
*
* @param att Qualified name of attribute.
* @param val Value of attribute.
* @throws SAXException If there is an underlying SAX exception.
*/
public void var(String att, String val) throws SAXException {
startSimpleElement(VAR,att,val);
}
/**
* Start an var
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @throws SAXException If there is an underlying SAX exception.
*/
public void var(String att1, String val1,
String att2, String val2) throws SAXException {
startSimpleElement(VAR,att1,val1,att2,val2);
}
/**
* Start an var
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @throws SAXException If there is an underlying SAX exception.
*/
public void var(String att1, String val1,
String att2, String val2,
String att3, String val3) throws SAXException {
startSimpleElement(VAR,att1,val1,att2,val2,att3,val3);
}
/**
* Start an var
element with the specified
* attributes and values.
*
* @param att1 Qualified name of attribute one.
* @param val1 Value of attribute one.
* @param att2 Qualified name of attribute two.
* @param val2 Value of attribute two.
* @param att3 Qualified name of attribute three.
* @param val3 Value of attribute three.
* @param att4 Qualified name of attribute four.
* @param val4 Value of attribute four.
* @throws SAXException If there is an underlying SAX exception.
*/
public void var(String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4) throws SAXException {
startSimpleElement(VAR,att1,val1,att2,val2,att3,val3,att4,val4);
}
/**
* A constant for the abbr
attribute.
*/
public static final String ABBR_ATT = "abbr";
/**
* A constant for the accept
attribute.
*/
public static final String ACCEPT = "accept";
/**
* A constant for the accept-charset
attribute.
*/
public static final String ACCEPT_CHARSET = "accept-charset";
/**
* A constant for the accesskey
attribute.
*/
public static final String ACCESSKEY = "accesskey";
/**
* A constant for the action
attribute.
*/
public static final String ACTION = "action";
/**
* A constant for the align
attribute.
*/
public static final String ALIGN = "align";
/**
* A constant for the alt
attribute.
*/
public static final String ALT = "alt";
/**
* A constant for the archive
attribute.
*/
public static final String ARCHIVE = "archive";
/**
* A constant for the axis
attribute.
*/
public static final String AXIS = "axis";
/**
* A constant for the border
attribute.
*/
public static final String BORDER = "border";
/**
* A constant for the cellspacing
attribute.
*/
public static final String CELLSPACING = "cellspacing";
/**
* A constant for the cellpadding
attribute.
*/
public static final String CELLPADDING = "cellpadding";
/**
* A constant for the char
attribute.
*/
public static final String CHAR = "char";
/**
* A constant for the charoff
attribute.
*/
public static final String CHAROFF = "charoff";
/**
* A constant for the charset
attribute.
*/
public static final String CHARSET = "charset";
/**
* A constant for the checked
attribute.
*/
public static final String CHECKED = "checked";
/**
* A constant for the cite
attribute.
*/
public static final String CITE_ATT = "cite";
/**
* A constant for the class
attribute.
*/
public static final String CLASS = "class";
/**
* A constant for the classid
attribute.
*/
public static final String CLASSID = "classid";
/**
* A constant for the codebase
attribute.
*/
public static final String CODEBASE = "codebase";
/**
* A constant for the codetype
attribute.
*/
public static final String CODETYPE = "codetype";
/**
* A constant for the cols
attribute.
*/
public static final String COLS = "cols";
/**
* A constant for the colspan
attribute.
*/
public static final String COLSPAN = "colspan";
/**
* A constant for the content
attribute.
*/
public static final String CONTENT = "content";
/**
* A constant for the coords
attribute.
*/
public static final String COORDS = "coords";
/**
* A constant for the data
attribute.
*/
public static final String DATA = "data";
/**
* A constant for the datetime
attribute.
*/
public static final String DATETIME = "datetime";
/**
* A constant for the declare
attribute.
*/
public static final String DECLARE = "declare";
/**
* A constant for the defer
attribute.
*/
public static final String DEFER = "defer";
/**
* A constant for the dir
attribute.
*/
public static final String DIR_ATT = "dir";
/**
* A constant for the disabled
attribute.
*/
public static final String DISABLED = "disabled";
/**
* A constant for the enctype
attribute.
*/
public static final String ENCTYPE = "enctype";
/**
* A constant for the for
attribute.
*/
public static final String FOR = "for";
/**
* A constant for the frame
attribute.
*/
public static final String FRAME_ATT = "frame";
/**
* A constant for the headers
attribute.
*/
public static final String HEADERS = "headers";
/**
* A constant for the height
attribute.
*/
public static final String HEIGHT = "height";
/**
* A constant for the href
attribute.
*/
public static final String HREF = "href";
/**
* A constant for the hreflang
attribute.
*/
public static final String HREFLANG = "hreflang";
/**
* A constant for the http-equiv
attribute.
*/
public static final String HTTP_EQUIV = "http-equiv";
/**
* A constant for the id
attribute.
*/
public static final String ID = "id";
/**
* A constant for the ismap
attribute.
*/
public static final String ISMAP = "ismap";
/**
* A constant for the label
attribute.
*/
public static final String LABEL_ATT = "label";
/**
* A constant for the lang
attribute.
*/
public static final String LANG = "lang";
/**
* A constant for the longdesc
attribute.
*/
public static final String LONGDESC = "longdesc";
/**
* A constant for the maxlength
attribute.
*/
public static final String MAXLENGTH = "maxlength";
/**
* A constant for the media
attribute.
*/
public static final String MEDIA = "media";
/**
* A constant for the method
attribute.
*/
public static final String METHOD = "method";
/**
* A constant for the multiple
attribute.
*/
public static final String MULTIPLE = "multiple";
/**
* A constant for the name
attribute.
*/
public static final String NAME = "name";
/**
* A constant for the nohref
attribute.
*/
public static final String NOHREF = "nohref";
/**
* A constant for the onblur
attribute.
*/
public static final String ONBLUR = "onblur";
/**
* A constant for the onchange
attribute.
*/
public static final String ONCHANGE = "onchange";
/**
* A constant for the onclick
attribute.
*/
public static final String ONCLICK = "onclick";
/**
* A constant for the ondblclick
attribute.
*/
public static final String ONDBLCLICK = "ondblclick";
/**
* A constant for the onfocus
attribute.
*/
public static final String ONFOCUS = "onfocus";
/**
* A constant for the onkeydown
attribute.
*/
public static final String ONKEYDOWN = "onkeydown";
/**
* A constant for the onkeypress
attribute.
*/
public static final String ONKEYPRESS = "onkeypress";
/**
* A constant for the onkeyup
attribute.
*/
public static final String ONKEYUP = "onkeyup";
/**
* A constant for the onload
attribute.
*/
public static final String ONLOAD = "onload";
/**
* A constant for the onmousedown
attribute.
*/
public static final String ONMOUSEDOWN = "onmousedown";
/**
* A constant for the onmousemove
attribute.
*/
public static final String ONMOUSEMOVE = "onmousemove";
/**
* A constant for the onmouseout
attribute.
*/
public static final String ONMOUSEOUT = "onmouseout";
/**
* A constant for the onmouseover
attribute.
*/
public static final String ONMOUSEOVER = "onmouseover";
/**
* A constant for the onmouseup
attribute.
*/
public static final String ONMOUSEUP = "onmouseup";
/**
* A constant for the onreset
attribute.
*/
public static final String ONRESET = "onreset";
/**
* A constant for the onselect
attribute.
*/
public static final String ONSELECT = "onselect";
/**
* A constant for the onsubmit
attribute.
*/
public static final String ONSUBMIT = "onsubmit";
/**
* A constant for the onunload
attribute.
*/
public static final String ONUNLOAD = "onunload";
/**
* A constant for the profile
attribute.
*/
public static final String PROFILE = "profile";
/**
* A constant for the readonly
attribute.
*/
public static final String READONLY = "readonly";
/**
* A constant for the rel
attribute.
*/
public static final String REL = "rel";
/**
* A constant for the rev
attribute.
*/
public static final String REV = "rev";
/**
* A constant for the rows
attribute.
*/
public static final String ROWS = "rows";
/**
* A constant for the rowspan
attribute.
*/
public static final String ROWSPAN = "rowspan";
/**
* A constant for the rules
attribute.
*/
public static final String RULES = "rules";
/**
* A constant for the scheme
attribute.
*/
public static final String SCHEME = "scheme";
/**
* A constant for the scope
attribute.
*/
public static final String SCOPE = "scope";
/**
* A constant for the selected
attribute.
*/
public static final String SELECTED = "selected";
/**
* A constant for the shape
attribute.
*/
public static final String SHAPE = "shape";
/**
* A constant for the size
attribute.
*/
public static final String SIZE = "size";
/**
* A constant for the span
attribute.
*/
public static final String SPAN_ATT = "span";
/**
* A constant for the src
attribute.
*/
public static final String SRC = "src";
/**
* A constant for the standby
attribute.
*/
public static final String STANDBY = "standby";
/**
* A constant for the style
attribute.
*/
public static final String STYLE_ATT = "style";
/**
* A constant for the summary
attribute.
*/
public static final String SUMMARY = "summary";
/**
* A constant for the tabindex
attribute.
*/
public static final String TABINDEX = "tabindex";
/**
* A constant for the title
attribute.
*/
public static final String TITLE_ATT = "title";
/**
* A constant for the type
attribute.
*/
public static final String TYPE = "type";
/**
* A constant for the usemap
attribute.
*/
public static final String USEMAP = "usemap";
/**
* A constant for the valign
attribute.
*/
public static final String VALIGN = "valign";
/**
* A constant for the value
attribute.
*/
public static final String VALUE = "value";
/**
* A constant for the valuetype
attribute.
*/
public static final String VALUETYPE = "valuetype";
/**
* A constant for the width
attribute.
*/
public static final String WIDTH = "width";
/**
* A constant for the xml:lang
attribute.
*/
public static final String XML_LANG = "xml:lang";
/**
* A constant for the xml:space
attribute.
*/
public static final String XML_SPACE = "xml:space";
/**
* A constant for the xmlns
attribute.
*/
public static final String XMLNS = "xmlns";
private static final boolean XHTML_MODE = true;
private static final String XHTML_1_0_STRICT_DTD
= "";
private void startSimpleElement(String qName, String att1, String val1,
String att2, String val2,
String att3, String val3)
throws SAXException {
Attributes atts = createAttributes(att1,val1,att2,val2,att3,val3);
startSimpleElement(qName,atts);
}
private void startSimpleElement(String qName, String att1, String val1,
String att2, String val2,
String att3, String val3,
String att4, String val4)
throws SAXException {
Attributes atts = createAttributes(att1,val1,att2,val2,att3,val3,
att4,val4);
startSimpleElement(qName,atts);
}
}