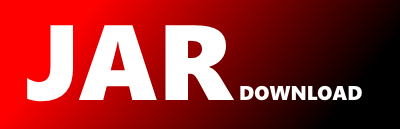
de.julielab.geneexpbase.configuration.Configuration Maven / Gradle / Ivy
package de.julielab.geneexpbase.configuration;
import de.julielab.java.utilities.FileUtilities;
import java.io.File;
import java.io.IOException;
import java.util.*;
/**
*
* A simple configuration class used to specify global static configuration values.
*
* Those are mostly file system paths to resources.
* Algorithmical parameters are represented by the {@link Parameters} class.
*/
public class Configuration extends Properties {
public static final String EHCACHE_CONFIG = "cache.ehcache.config";
/**
* Short for "machine learning". A special path element specifying the training and usage of a machine learning model.
* The idea is that the {@link #ML} element indicates the existence of a machine learning configuration. The directly
* succeeding path element should than represent the root of the configuration parameters for the model.
* E.g. candidate_retrieval.ml.approxmatches
* would be the root for the re-ranking ML model for approximate candidate matches for a gene mention.
*/
public static final String ML = "ml";
public static final String PARAM_ALGORITHM = "algorithm";
/**
* Configuration path element to denote the training instances creates for a machine learning approach.
* Should be located directly at the root of a machine learning configuration.
*
* @see {@link #ML}
*/
public static final String TRAINING_INSTANCES = "training_instances";
/**
* Instance field: The concrete classifier to employ for disambiguation.
*/
public static final String KEY_CLASSIFIER = "classifier";
/**
* Instance field: The concrete ranger to employ for disambiguation.
*/
public static final String KEY_RANKER = "ranker";
public static final String PARAM_STANDARDIZE_FEATURES = "standardize_features";
public static final String PARAM_MINMAX_SCALE_FEATURES = "minmax_scale_features";
public static final String KEY_STANDARDIZATION_VALUES = "standardization_values";
public static final String KEY_MINMAX_SCALING_VALUES = "minmax_scaling_values";
public static final String KEY_DATA_ALPHABET = "data_alphabet";
public static final String KEY_TARGET_ALPHABET = "target_alphabet";
public static final String VALUE_LTR = "ltr";
public static final String VALUE_LUCENE = "lucene";
public static final String VALUE_MAXENT = "maxent";
public static final String VALUE_SVM = "svm";
public static final String VALUE_TRANSFORMER = "transformer";
public static final String PARAM_SVM_TYPE = "svm.type";
public static final String PARAM_SVM_C = "svm.c";
public static final String PARAM_SVM_KERNEL_TYPE = "svm.kerneltype";
public static final String PARAM_SVM_GAMMA = "svm.gamma";
public static final String PARAM_SVM_COEF0 = "svm.coef0";
public static final String PARAM_SVM_DEGREE = "svm.degree";
public static final String PARAM_LTR_METRIC = "ltr.metric";
public static final String PARAM_LTR_K = "ltr.k";
public static final String PARAM_LTR_ALGORITHM = "ltr.algorithm";
public static final String PARAM_PYTHON_EXECUTABLE = "python_executable";
public static final String PYTHON_PROCESS_LIMIT = "python_process_limit";
public static final String CONCURRENCY_LEVEL = "concurrency_level";
/**
* For SMAC optimization with ML approaches: Whether to use the training data from all corpora or only the
* corpus the current instance is from.
*/
public static final String PARAM_ML_USE_ALL_CORPUS_TRAINSPLITS = "use_all_corpus_trainsplits";
// Mention and semantic score scaling to [0,1]. This is supposed to help with finding good rejection thresholds
// when the score is not a probability by nature.
public static final String PARAM_SCALE_RESULT_SCORE = "scale_result_score";
public static final String KEY_MAX_RESULT_SCORE = "max_result_score";
public static final String KEY_MIN_RESULT_SCORE = "min_result_score";
// Model paths
public static final String PARAM_MODEL = "model";
public static final String GENE_ORTHOLOGS_PATH = "gene_orthologs";
public Configuration() {
}
public Configuration(String... parameters) {
if (parameters != null) {
if (parameters.length % 2 == 1)
throw new IllegalArgumentException("Uneven number parameter-value items.");
for (int i = 0; i < parameters.length - 1; i++) {
String parameter = parameters[i];
String value = parameters[i + 1];
setProperty(parameter, value);
}
}
}
public Configuration(File configurationFile) throws IOException {
this.load(FileUtilities.getInputStreamFromFile(configurationFile));
}
/**
* Adds all properties in baseConfiguration to this configuration.
* @param baseConfiguration The configuration to copy.
*/
public Configuration(de.julielab.geneexpbase.configuration.Configuration baseConfiguration) {
for (String key : baseConfiguration.stringPropertyNames())
setProperty(key, baseConfiguration.getProperty(key));
}
public static String dot(String... inputs) {
return String.join(".", inputs);
}
public Optional getBoolean(String parameter) {
String property = getProperty(parameter.trim());
if (property != null)
return Optional.of(Boolean.parseBoolean(property.trim()));
return Optional.empty();
}
public OptionalInt getInteger(String parameter) {
String property = getProperty(parameter.trim());
if (property != null)
return OptionalInt.of(Integer.parseInt(property));
return OptionalInt.empty();
}
public OptionalDouble getDouble(String parameter) {
String property = getProperty(parameter.trim());
if (property != null)
return OptionalDouble.of(Float.parseFloat(property));
return OptionalDouble.empty();
}
public OptionalLong getLong(String parameter) {
String property = getProperty(parameter.trim());
if (property != null)
return OptionalLong.of(Long.parseLong(property));
return OptionalLong.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy