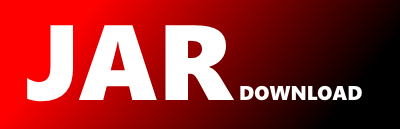
de.julielab.geneexpbase.genemodel.GeneOrthologs Maven / Gradle / Ivy
package de.julielab.geneexpbase.genemodel;
import com.google.common.collect.Sets;
import de.julielab.java.utilities.FileUtilities;
import de.julielab.java.utilities.cache.CacheAccess;
import de.julielab.java.utilities.cache.NoOpCacheAccess;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nullable;
import javax.inject.Inject;
import javax.inject.Named;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.Serializable;
import java.util.*;
import java.util.stream.Collectors;
import static de.julielab.geneexpbase.configuration.Configuration.GENE_ORTHOLOGS_PATH;
/**
* This class models the contents of the gene_orthologs file.
*
* @see ftp://ftp.ncbi.nih.gov/gene/DATA/
*/
public class GeneOrthologs {
private final static Logger log = LoggerFactory.getLogger(GeneOrthologs.class);
private static GeneOrthologs instance;
private Map> recordsByOtherGeneId;
private Map> recordsByGeneId;
private final CacheAccess> cache;
private File geneOrthologsFile;
@Inject
public GeneOrthologs(@Nullable @Named(GENE_ORTHOLOGS_PATH) File geneOrthologsFile) {
if (geneOrthologsFile != null) {
log.info("Reading gene orthologs from {}", geneOrthologsFile);
this.geneOrthologsFile = geneOrthologsFile;
} else {
log.warn("The path to the gene orthologs file was not specified. No ortholog information can be used.");
}
cache = new NoOpCacheAccess<>("", "");//CacheService.getInstance().getCacheAccess("Orthologs.db", "orthologs, ", CacheAccess.JAVA, CacheAccess.JAVA, mapSettings);
}
private synchronized void readOrthologsFile() {
if (recordsByGeneId == null) {
log.info("Reading gene orthologs from {}", geneOrthologsFile);
try (BufferedReader br = FileUtilities.getReaderFromFile(geneOrthologsFile)) {
List recordList = br.lines().filter(line -> !line.startsWith("#")).map(OrthologyRecord::new).collect(Collectors.toList());
recordsByGeneId = recordList.stream().collect(Collectors.groupingBy(OrthologyRecord::getGeneId, HashMap::new, Collectors.toSet()));
recordsByOtherGeneId = recordList.stream().collect(Collectors.groupingBy(OrthologyRecord::getOtherGeneId, HashMap::new, Collectors.toSet()));
} catch (IOException e) {
e.printStackTrace();
}
log.info("Done.");
}
}
public Set getOrthologs(String geneId) {
OrthologKey key = new OrthologKey(geneId);
Set orthologyRecords = cache.get(key);
if (orthologyRecords == null) {
// the method reads file only once
readOrthologsFile();
orthologyRecords = new HashSet<>(Sets.union(recordsByGeneId.getOrDefault(geneId, Collections.emptySet()), recordsByOtherGeneId.getOrDefault(geneId, Collections.emptySet())));
cache.put(key, orthologyRecords);
}
return orthologyRecords;
}
public Set getOrthologsInSpecies(String geneId, String targetTaxId) {
OrthologKey key = new OrthologKey(geneId, targetTaxId);
Set orthologyRecords = cache.get(key);
if (orthologyRecords == null) {
// the method reads the file only once
readOrthologsFile();
orthologyRecords = getOrthologs(geneId).stream().filter(r -> r.getTaxId().equals(targetTaxId) || r.getOtherTaxId().equals(targetTaxId)).collect(Collectors.toSet());
cache.put(key, orthologyRecords);
}
return orthologyRecords;
}
private static class OrthologKey implements Serializable {
private final String geneId;
private final String targetTaxId;
public OrthologKey(String geneId) {
this(geneId, null);
}
public OrthologKey(String geneId, String targetTaxId) {
this.geneId = geneId;
this.targetTaxId = targetTaxId;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
OrthologKey that = (OrthologKey) o;
return Objects.equals(geneId, that.geneId) &&
Objects.equals(targetTaxId, that.targetTaxId);
}
@Override
public int hashCode() {
return Objects.hash(geneId, targetTaxId);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy