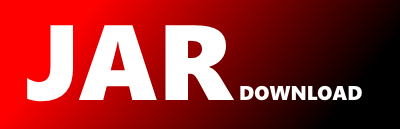
de.julielab.geneexpbase.genemodel.SpeciesCandidates Maven / Gradle / Ivy
package de.julielab.geneexpbase.genemodel;
import de.julielab.java.utilities.spanutils.OffsetMap;
import org.apache.commons.lang3.Range;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
public class SpeciesCandidates implements Cloneable {
private OffsetMap titleCandidates;
private Set meshCandidates;
private OffsetMap textCandidates;
public SpeciesCandidates(OffsetMap titleCandidates, Set meshCandidates,
OffsetMap textCandidates) {
this.titleCandidates = titleCandidates;
this.meshCandidates = meshCandidates;
this.textCandidates = textCandidates;
}
/**
* If the textCandidates include species mentions for the title,
* this constructor will automatically derive the title species IDs.
*
* @param titleBegin
* @param titleEnd
* @param meshCandidates
* @param textCandidates
*/
public SpeciesCandidates(int titleBegin, int titleEnd, Set meshCandidates,
OffsetMap textCandidates) {
this.textCandidates = textCandidates;
if (null == textCandidates)
this.textCandidates = OffsetMap.emptyOffsetMap();
this.titleCandidates = new OffsetMap(this.textCandidates.restrictTo(Range.between(titleBegin, titleEnd)));
this.meshCandidates = meshCandidates;
}
@Override
protected SpeciesCandidates clone() {
OffsetMap titleCandidates = this.titleCandidates != null ? new OffsetMap<>(this.titleCandidates) : OffsetMap.emptyOffsetMap();
Set meshCandidates = this.meshCandidates != null ? new HashSet<>(this.meshCandidates) : Collections.emptySet();
OffsetMap textCandidates = this.textCandidates != null ? new OffsetMap<>(this.textCandidates) : OffsetMap.emptyOffsetMap();
return new SpeciesCandidates(titleCandidates, meshCandidates, textCandidates);
}
public OffsetMap getAllMentionCandidates() {
OffsetMap allMentionCandidates = new OffsetMap<>();
if (titleCandidates != null)
allMentionCandidates.putAll(titleCandidates);
if (textCandidates != null)
allMentionCandidates.putAll(textCandidates);
return allMentionCandidates;
}
public OffsetMap getTitleCandidates() {
return titleCandidates;
}
public void setTitleCandidates(OffsetMap titleCandidates) {
this.titleCandidates = titleCandidates;
}
public Set getMeshCandidates() {
return meshCandidates;
}
public void setMeshCandidates(Set meshCandidates) {
this.meshCandidates = meshCandidates;
}
public OffsetMap getTextCandidates() {
return textCandidates;
}
public void setTextCandidates(OffsetMap textCandidates) {
this.textCandidates = textCandidates;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy