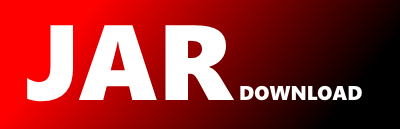
de.julielab.geneexpbase.ioc.BaseModule Maven / Gradle / Ivy
package de.julielab.geneexpbase.ioc;
import com.google.inject.AbstractModule;
import com.google.inject.Provides;
import de.julielab.geneexpbase.TermNormalizer;
import de.julielab.geneexpbase.configuration.Configuration;
import de.julielab.geneexpbase.data.DocumentLoader;
import de.julielab.geneexpbase.services.CacheService;
import de.julielab.geneexpbase.services.ShutdownRequiringExecutorService;
import javax.inject.Named;
import javax.inject.Singleton;
import java.io.File;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import static com.google.inject.matcher.Matchers.any;
public class BaseModule extends AbstractModule {
/**
* Constant for disambiguation of Guice services for the document level -> mention level gene ID inference
*/
public static final String ID_INFERENCE = "IdInference";
private final Configuration configuration;
public BaseModule(Configuration configuration) {
this.configuration = configuration;
}
@Override
protected void configure() {
super.configure();
bind(Configuration.class).toInstance(configuration);
bind(TermNormalizer.class);
bind(DocumentLoader.class);
bind(CacheService.class).in(Singleton.class);
// We want to pass the shutdownHub to the LifeCycleListener in order to automatically
// register ShutdownRequiring classes. Since we cannot inject services yet, we work with
// a specific instance instead.
ServicesShutdownHub shutdownHub = new ServicesShutdownHub();
bind(ServicesShutdownHub.class).toInstance(shutdownHub);
// This listener registers all services that are injected at any time of the application run
// that implement Closable. All those services are added to a list and
// Runtime.getRuntime().addShutdownHook(shutdownHook) is used to close all such services on application termination.
bindListener(any(), new LifeCycleListener(shutdownHub));
}
@Provides
@Named(de.julielab.geneexpbase.configuration.Configuration.GENE_ORTHOLOGS_PATH)
File provideGeneOrthologsFile(Configuration configuration) {
String orthologsPath = configuration.getProperty(de.julielab.geneexpbase.configuration.Configuration.GENE_ORTHOLOGS_PATH);
if (orthologsPath == null)
return null;
return new File(orthologsPath);
}
@Provides
@Singleton
ExecutorService provideExecutorService() {
int luceneConcurrencyLevel = Integer.parseInt((String) configuration.getOrDefault(Configuration.CONCURRENCY_LEVEL, "1"));
ShutdownRequiringExecutorService executorService = new ShutdownRequiringExecutorService(Executors.newFixedThreadPool(luceneConcurrencyLevel));
return executorService;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy