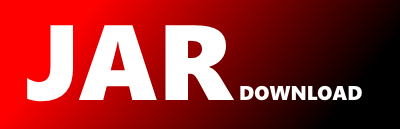
de.knightsoftnet.validators.server.controller.PhoneNumberServiceController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-bean-validators-spring-gwtp Show documentation
Show all versions of gwt-bean-validators-spring-gwtp Show documentation
The GWT Bean Validators is a collection of JSR-303/JSR-349/JSR 380 bean validators. It can be used on server
and with the help of GWT even on client side. This packages contains rest services and validator
for phone numbers based on gwtp-rest dispatcher and spring to reduce client side code.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.validators.server.controller;
import de.knightsoftnet.validators.shared.Parameters;
import de.knightsoftnet.validators.shared.ResourcePaths.PhoneNumber;
import de.knightsoftnet.validators.shared.data.PhoneNumberData;
import de.knightsoftnet.validators.shared.data.PhoneNumberDataWithFormats;
import de.knightsoftnet.validators.shared.data.ValueWithPos;
import de.knightsoftnet.validators.shared.data.ValueWithPosAndCountry;
import de.knightsoftnet.validators.shared.util.LocaleUtil;
import de.knightsoftnet.validators.shared.util.PhoneNumberUtil;
import org.apache.commons.lang3.BooleanUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
import javax.annotation.security.PermitAll;
/**
* phone number web service brings phone number util functions to client.
*
* @author Manfred Tremmel
*/
@RestController
@RequestMapping(PhoneNumber.ROOT)
public class PhoneNumberServiceController {
/**
* phone number utils to test phone numbers.
*/
private final PhoneNumberUtil phoneNumberUtil = new PhoneNumberUtil();
@RequestMapping(value = PhoneNumber.PARSE_PHONE_NUMBER, method = RequestMethod.GET)
@PermitAll
public PhoneNumberData parsePhoneNumber(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.parsePhoneNumber(pphoneNumber, pcountry,
LocaleUtil.convertLanguageToLocale(planguage));
}
@RequestMapping(value = PhoneNumber.PARSE_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos parsePhoneNumber(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.phoneNumberUtil.parsePhoneNumber(pphoneNumber, pphoneNumber.getCountry());
}
/**
* parse and reformat the phone number in all available formats.
*
* @param planguage language to use
* @param pcountry default country
* @param pphoneNumber phone number to format
* @return PhoneNumberDataWithFormats
*/
@RequestMapping(value = PhoneNumber.PARSE_AND_FORMAT, method = RequestMethod.GET)
@PermitAll
public PhoneNumberDataWithFormats parseAndFormatPhoneNumber(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
final PhoneNumberDataWithFormats result = new PhoneNumberDataWithFormats(this.phoneNumberUtil
.parsePhoneNumber(pphoneNumber, pcountry, LocaleUtil.convertLanguageToLocale(planguage)));
result.setCommonInternational(this.phoneNumberUtil.formatCommonInternational(result));
result.setCommonNational(this.phoneNumberUtil.formatCommonNational(result));
result.setDin5008International(this.phoneNumberUtil.formatDin5008International(result));
result.setDin5008National(this.phoneNumberUtil.formatDin5008National(result));
result.setE123International(this.phoneNumberUtil.formatE123International(result));
result.setE123National(this.phoneNumberUtil.formatE123National(result));
result.setMs(this.phoneNumberUtil.formatMs(result));
result.setUrl(this.phoneNumberUtil.formatUrl(result));
return result;
}
@RequestMapping(value = PhoneNumber.FORMAT_E123, method = RequestMethod.GET)
@PermitAll
public String formatE123(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatE123(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_E123_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatE123WithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatE123WithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_E123_INTERNATIONAL, method = RequestMethod.GET)
@PermitAll
public String formatE123International(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatE123International(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_E123_INTERNATIONAL_WITH_POS,
method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatE123InternationalWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatE123InternationalWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_E123_NATIONAL, method = RequestMethod.GET)
@PermitAll
public String formatE123National(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatE123National(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_E123_NATIONAL_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatE123NationalWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatE123NationalWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_DIN5008, method = RequestMethod.GET)
@PermitAll
public String formatDin5008(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatDin5008(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_DIN5008_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatDin5008WithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatDin5008WithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_DIN5008_INTERNATIONAL, method = RequestMethod.GET)
@PermitAll
public String formatDin5008International(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatDin5008International(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_DIN5008_INTERNATIONAL_WITH_POS,
method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatDin5008InternationalWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatDin5008InternationalWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_DIN5008_NATIONAL, method = RequestMethod.GET)
@PermitAll
public String formatDin5008National(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatDin5008National(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_DIN5008_NATIONAL_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatDin5008NationalWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatDin5008NationalWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_RFC3966, method = RequestMethod.GET)
@PermitAll
public String formatRfc3966(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatRfc3966(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_RFC3966_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatRfc3966WithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatRfc3966WithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_MS, method = RequestMethod.GET)
@PermitAll
public String formatMs(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatMs(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_MS_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatMsWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatMsWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_URL, method = RequestMethod.GET)
@PermitAll
public String formatUrl(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatUrl(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_URL_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatUrlWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatUrlWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_COMMON, method = RequestMethod.GET)
@PermitAll
public String formatCommon(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatCommon(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_COMMON_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatCommonWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatCommonWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_COMMON_INTERNATIONAL, method = RequestMethod.GET)
@PermitAll
public String formatCommonInternational(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatCommonInternational(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_COMMON_INTERNATIONAL_WITH_POS,
method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatCommonInternationalWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatCommonInternationalWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
@RequestMapping(value = PhoneNumber.FORMAT_COMMON_NATIONAL, method = RequestMethod.GET)
@PermitAll
public String formatCommonNational(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber) {
return this.phoneNumberUtil.formatCommonNational(pphoneNumber, pcountry);
}
@RequestMapping(value = PhoneNumber.FORMAT_COMMON_NATIONAL_WITH_POS, method = RequestMethod.PUT)
@ResponseStatus(HttpStatus.OK)
@PermitAll
@ResponseBody
public ValueWithPos formatCommonNationalWithPos(
@RequestBody final ValueWithPosAndCountry pphoneNumber) {
return this.valueWithPosDefaults(this.phoneNumberUtil.formatCommonNationalWithPos( //
pphoneNumber, pphoneNumber.getCountry()), pphoneNumber);
}
private ValueWithPos valueWithPosDefaults(final ValueWithPos pformatValueWithPos,
final ValueWithPosAndCountry pdefaultNumber) {
if (StringUtils.isEmpty(pformatValueWithPos.getValue()) //
|| StringUtils.startsWith(pdefaultNumber.getValue(), pformatValueWithPos.getValue())
&& !Character.isDigit(pdefaultNumber.getValue()
.charAt(StringUtils.length(pdefaultNumber.getValue()) - 1))) {
pformatValueWithPos.setValue(pdefaultNumber.getValue());
pformatValueWithPos.setPos(pdefaultNumber.getPos());
}
return pformatValueWithPos;
}
@RequestMapping(value = PhoneNumber.GET_SUGGESTIONS, method = RequestMethod.GET)
@PermitAll
public List getSuggestions(
@RequestParam(value = Parameters.LANGUAGE, required = true) final String planguage,
@RequestParam(value = Parameters.SEARCH, required = true) final String psearch,
@RequestParam(value = Parameters.LIMIT, required = true) final int plimit) {
return this.phoneNumberUtil.getSuggstions(psearch, plimit,
LocaleUtil.convertLanguageToLocale(planguage));
}
/**
* validate a phone number.
*
* @param pcountry default country
* @param pphoneNumber phone number to check
* @param pdin5008 set to true if DIN 5008 format is allowed
* @param pe123 set to true if E123 format is allowed
* @param puri set to true if URI format is allowed
* @param pms set to true if Microsoft format is allowed
* @param pcommon set to true if common format is allowed
* @return true if number is valid
*/
@RequestMapping(value = PhoneNumber.VALIDATE, method = RequestMethod.GET)
@PermitAll
public Boolean validate(
@RequestParam(value = Parameters.COUNTRY, required = true) final String pcountry,
@RequestParam(value = Parameters.PHONE_NUMBER, required = true) final String pphoneNumber,
@RequestParam(value = Parameters.DIN_5008, required = false) final Boolean pdin5008,
@RequestParam(value = Parameters.E123, required = false) final Boolean pe123,
@RequestParam(value = Parameters.URI, required = false) final Boolean puri,
@RequestParam(value = Parameters.MS, required = false) final Boolean pms,
@RequestParam(value = Parameters.COMMON, required = false) final Boolean pcommon) {
final PhoneNumberData parsedNumber =
this.phoneNumberUtil.parsePhoneNumber(pphoneNumber, pcountry);
if (parsedNumber.isValid()) {
if (BooleanUtils.isTrue(pdin5008) && (StringUtils.equals(pphoneNumber,
this.phoneNumberUtil.formatDin5008National(parsedNumber))
|| StringUtils.equals(pphoneNumber,
this.phoneNumberUtil.formatDin5008International(parsedNumber)))) {
return Boolean.TRUE;
}
if (BooleanUtils.isTrue(pe123) && (StringUtils.equals(pphoneNumber,
this.phoneNumberUtil.formatE123National(parsedNumber))
|| StringUtils.equals(pphoneNumber,
this.phoneNumberUtil.formatE123International(parsedNumber)))) {
return Boolean.TRUE;
}
if (BooleanUtils.isTrue(puri)
&& StringUtils.equals(pphoneNumber, this.phoneNumberUtil.formatUrl(parsedNumber))) {
return Boolean.TRUE;
}
if (BooleanUtils.isTrue(pms)
&& StringUtils.equals(pphoneNumber, this.phoneNumberUtil.formatMs(parsedNumber))) {
return Boolean.TRUE;
}
if (BooleanUtils.isTrue(pcommon) && (StringUtils.equals(pphoneNumber,
this.phoneNumberUtil.formatCommonNational(parsedNumber))
|| StringUtils.equals(pphoneNumber,
this.phoneNumberUtil.formatCommonInternational(parsedNumber)))) {
return Boolean.TRUE;
}
}
return Boolean.FALSE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy