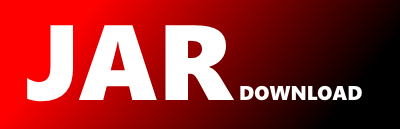
de.knightsoftnet.mtwidgets.client.ui.widget.AbstractPhoneNumberRestSuggestBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-mt-widgets-restygwt-jaxrs Show documentation
Show all versions of gwt-mt-widgets-restygwt-jaxrs Show documentation
A set of widgets and handlers using server calls for gwt applications using gwt-bean-validators-restygwt-jaxrs.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.mtwidgets.client.ui.widget;
import de.knightsoftnet.validators.client.rest.api.PhoneNumberServiceAsync;
import de.knightsoftnet.validators.client.rest.api.ServiceFactory;
import de.knightsoftnet.validators.client.rest.helper.FutureResult;
import de.knightsoftnet.validators.shared.data.ValueWithPos;
import de.knightsoftnet.validators.shared.data.ValueWithPosAndCountry;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import com.google.gwt.core.shared.GWT;
import com.google.gwt.i18n.client.LocaleInfo;
import com.google.gwt.user.client.TakesValue;
import com.google.gwt.user.client.ui.SuggestOracle;
import elemental.client.Browser;
import org.apache.commons.lang3.StringUtils;
import org.fusesource.restygwt.client.Method;
import org.fusesource.restygwt.client.MethodCallback;
import java.util.Objects;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
/**
* abstract phone number suggest widget with rest calls.
*
* @author Manfred Tremmel
*
*/
public abstract class AbstractPhoneNumberRestSuggestBox extends AbstractFormatingSuggestBox {
protected final PhoneNumberServiceAsync service;
protected final MethodCallback> callback;
protected TakesValue> countryCodeField;
private String valueBeforeFormat;
/**
* cache map.
*/
final LoadingCache, FutureResult>> cache;
/**
* default constructor.
*/
public AbstractPhoneNumberRestSuggestBox(final SuggestOracle poracle) {
super(poracle, new TextBoxWithFormating(Browser.getDocument().createInputElement(), "tel"));
((TextBoxWithFormating) getValueBox()).setFormating(this);
setAutocomplete("off");
service = ServiceFactory.getPhoneNumberService();
callback = new MethodCallback>() {
@Override
public void onFailure(final Method pmethod, final Throwable pexception) {
GWT.log(pexception.getMessage(), pexception);
}
@Override
public void onSuccess(final Method pmethod, final ValueWithPos presponse) {
if (presponse != null && StringUtils.isNotEmpty(presponse.getValue())
&& (presponse.getOriginalValue() == null
|| StringUtils.equals(presponse.getOriginalValue(), valueBeforeFormat))) {
AbstractPhoneNumberRestSuggestBox.this.setTextWithPos(presponse, true);
}
}
};
cache = CacheBuilder.newBuilder().maximumSize(10000).expireAfterWrite(10, TimeUnit.DAYS).build(
new CacheLoader, FutureResult>>() {
@Override
public FutureResult> load(
final ValueWithPosAndCountry pkey) {
final FutureResult> result = new FutureResult<>();
result.addCallback(callback);
try {
AbstractPhoneNumberRestSuggestBox.this.formatValue(pkey, result);
} catch (final ExecutionException e) {
GWT.log(e.getMessage(), e);
}
return result;
}
});
}
@Override
public void formatValue(final ValueWithPos pvalue, final boolean fireEvents) {
if (pvalue == null || StringUtils.isEmpty(pvalue.getValue())) {
this.setValue(StringUtils.EMPTY);
} else {
final ValueWithPosAndCountry unformatedEntry = new ValueWithPosAndCountry<>(
pvalue.getValue(), pvalue.getPos(), Objects.toString(countryCodeField.getValue()),
LocaleInfo.getCurrentLocale().getLocaleName());
valueBeforeFormat = pvalue.getValue();
try {
final FutureResult> result = cache.get(unformatedEntry);
if (result.isDone()) {
setTextWithPos(result.get(), fireEvents);
}
} catch (final ExecutionException e) {
GWT.log(e.getMessage(), e);
}
}
}
public abstract void formatValue(ValueWithPosAndCountry pkey,
FutureResult> presult) throws ExecutionException;
/**
* set reference to a field which contains the country code.
*
* @param pcountryCodeField field which contains the country code
*/
public final void setCountryCodeReferenceField(final TakesValue> pcountryCodeField) {
countryCodeField = pcountryCodeField;
}
@Override
public boolean isAllowedCharacter(final char pcharacter) {
return pcharacter >= '0' && pcharacter <= '9' || isFormatingCharacter(pcharacter);
}
@Override
public boolean isCharacterToReplace(final char pcharacter) {
return false;
}
@Override
public char replaceCharacter(final char pcharacter) {
return pcharacter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy