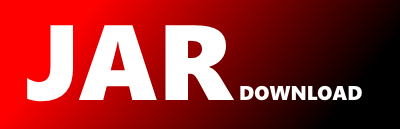
de.knightsoftnet.mtwidgets.client.ui.widget.MultiLanguageTextBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-mt-widgets-spring-gwtp Show documentation
Show all versions of gwt-mt-widgets-spring-gwtp Show documentation
A set of widgets and handlers using server calls for gwt applications using gwt-bean-validators-spring-gwtp.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.mtwidgets.client.ui.widget;
import de.knightsoftnet.gwtp.spring.shared.db.LocalizedEntity;
import de.knightsoftnet.mtwidgets.client.ui.widget.features.HasValidationMessageElement;
import de.knightsoftnet.mtwidgets.client.ui.widget.styling.WidgetResources;
import de.knightsoftnet.validators.client.decorators.ExtendedValueBoxEditor;
import de.knightsoftnet.validators.client.editor.ValueBoxEditor;
import de.knightsoftnet.validators.shared.util.LangFromPathUtil;
import com.google.gwt.core.shared.GWT;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.dom.client.DomEvent;
import com.google.gwt.event.dom.client.HasKeyPressHandlers;
import com.google.gwt.event.dom.client.HasKeyUpHandlers;
import com.google.gwt.event.dom.client.KeyPressEvent;
import com.google.gwt.event.dom.client.KeyPressHandler;
import com.google.gwt.event.dom.client.KeyUpEvent;
import com.google.gwt.event.dom.client.KeyUpHandler;
import com.google.gwt.event.logical.shared.ValueChangeEvent;
import com.google.gwt.event.logical.shared.ValueChangeHandler;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.i18n.client.LocaleInfo;
import com.google.gwt.safehtml.shared.SafeHtmlUtils;
import com.google.gwt.uibinder.client.UiBinder;
import com.google.gwt.uibinder.client.UiField;
import com.google.gwt.uibinder.client.UiHandler;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.FlowPanel;
import com.google.gwt.user.client.ui.HTML;
import com.google.gwt.user.client.ui.HTMLPanel;
import com.google.gwt.user.client.ui.HasValue;
import com.google.gwt.user.client.ui.Widget;
import org.gwtproject.editor.client.EditorError;
import org.gwtproject.editor.client.LeafValueEditor;
import org.gwtproject.editor.client.TakesValue;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import java.util.Set;
/**
* widget for multilingual input.
*
* @author Manfred Tremmel
*
*/
public class MultiLanguageTextBox extends Composite implements LeafValueEditor,
HasValue, TakesValue,
HasValidationMessageElement>, HasKeyUpHandlers,
HasKeyPressHandlers {
interface Binder extends UiBinder {
}
private static Binder uiBinder = GWT.create(Binder.class);
@UiField
WidgetResources resources;
@UiField
HTML showHide;
@UiField
TextBox localeLangInput;
@UiField
FlowPanel langValues;
private final ValueBoxEditor editor;
private Long id;
private final Map localizedText = new HashMap<>();
/**
* constructor.
*/
public MultiLanguageTextBox() {
super();
initWidget(MultiLanguageTextBox.uiBinder.createAndBindUi(this));
final String usedLang = LocaleInfo.getCurrentLocale().getLocaleName();
localizedText.put(usedLang, localeLangInput);
localeLangInput.addValueChangeHandler(event -> ValueChangeEvent.fire(this, getValue()));
localeLangInput
.addKeyPressHandler(event -> DomEvent.fireNativeEvent(event.getNativeEvent(), this));
localeLangInput
.addKeyUpHandler(event -> DomEvent.fireNativeEvent(event.getNativeEvent(), this));
for (final String lang : LocaleInfo.getAvailableLocaleNames()) {
if (!"default".equals(lang) && !usedLang.equals(lang)) {
langValues.add(createLangWidget(lang));
}
}
editor = new ExtendedValueBoxEditor<>(this, null);
}
private FlowPanel createLangWidget(final String plang) {
final FlowPanel langPanel = new FlowPanel();
final HTML langLabel = new HTML(SafeHtmlUtils.fromString(plang));
langPanel.add(langLabel);
final TextBox langInput = new TextBox();
langInput.addValueChangeHandler(event -> ValueChangeEvent.fire(this, getValue()));
langInput.addKeyPressHandler(event -> DomEvent.fireNativeEvent(event.getNativeEvent(), this));
langInput.addKeyUpHandler(event -> DomEvent.fireNativeEvent(event.getNativeEvent(), this));
langPanel.add(langInput);
localizedText.put(plang, langInput);
return langPanel;
}
@Override
public void setValue(final LocalizedEntity pvalue) {
setValue(pvalue, false);
}
@Override
public void setValue(final LocalizedEntity pvalue, final boolean pfireEvents) {
if (pvalue == null) {
id = null;
for (final Entry entry : localizedText.entrySet()) {
entry.getValue().setValue(null, pfireEvents);
}
} else {
id = pvalue.getId();
for (final Entry entry : localizedText.entrySet()) {
entry.getValue().setValue(pvalue.getLocalizedText(entry.getKey()), pfireEvents);
}
}
}
@Override
public LocalizedEntity getValue() {
final LocalizedEntity value = new LocalizedEntity(id);
for (final Entry entry : localizedText.entrySet()) {
value.getLocalizedText().put(entry.getKey(), entry.getValue().getValue());
}
return value;
}
@Override
public HandlerRegistration addValueChangeHandler(
final ValueChangeHandler phandler) {
return addHandler(phandler, ValueChangeEvent.getType());
}
@Override
public HandlerRegistration addKeyPressHandler(final KeyPressHandler handler) {
return addHandler(handler, KeyPressEvent.getType());
}
@Override
public HandlerRegistration addKeyUpHandler(final KeyUpHandler handler) {
return addHandler(handler, KeyUpEvent.getType());
}
@UiHandler("showHide")
public void switchShowHide(final ClickEvent event) {
this.setStyleName(resources.multiLanguageTextBoxStyle().multLangOpen(),
!this.getStyleName().contains(resources.multiLanguageTextBoxStyle().multLangOpen()));
}
@Override
protected void onEnsureDebugId(final String pbaseId) {
localizedText.entrySet()
.forEach(entry -> entry.getValue().ensureDebugId(pbaseId + "_" + entry.getKey()));
}
@Override
public void setValidationMessageElement(final HTMLPanel pelement) {
localizedText.values().forEach(textBox -> textBox.setValidationMessageElement(pelement));
}
@Override
public HTMLPanel getValidationMessageElement() {
return localeLangInput.getValidationMessageElement();
}
@Override
public void setCustomValidity(final String message) {
// ignore
}
@Override
public void showErrors(final List perrors) {
final Map> messages = new HashMap<>();
perrors.stream().filter(error -> editorErrorMatches(error)).forEach(error -> {
final String language = LangFromPathUtil.extract(error.getAbsolutePath());
if (language != null) {
if (!messages.containsKey(language)) {
messages.put(language, new HashSet<>());
}
messages.get(language).add(error.getMessage());
}
});
localizedText.entrySet().forEach(textInput -> {
if (messages.containsKey(textInput.getKey())) {
textInput.getValue().showErrors(messages.get(textInput.getKey()));
if (!Objects.equals(textInput.getValue(), localeLangInput)) {
this.setStyleName(resources.multiLanguageTextBoxStyle().multLangOpen(), true);
}
} else {
textInput.getValue().showErrors(Collections.emptySet());
}
});
}
@Override
public ValueBoxEditor asEditor() {
return editor;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy