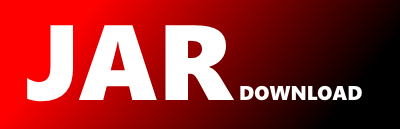
de.knightsoftnet.mtwidgets.client.ui.widget.AdminNavigationWidget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-mt-widgets-spring-gwtp Show documentation
Show all versions of gwt-mt-widgets-spring-gwtp Show documentation
A set of widgets and handlers using server calls for gwt applications using gwt-bean-validators-spring-gwtp.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.mtwidgets.client.ui.widget;
import de.knightsoftnet.gwtp.spring.shared.data.AdminNavigation;
import de.knightsoftnet.gwtp.spring.shared.search.SearchRequest;
import de.knightsoftnet.mtwidgets.client.ui.page.admin.AbstractAdminPresenter;
import de.knightsoftnet.mtwidgets.client.ui.widget.features.HandlesSelectedEntry;
import de.knightsoftnet.validators.shared.Parameters;
import com.google.gwt.dom.client.Style.Display;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.dom.client.KeyCodes;
import com.google.gwt.event.dom.client.KeyPressEvent;
import com.google.gwt.event.logical.shared.ValueChangeEvent;
import com.google.gwt.event.logical.shared.ValueChangeHandler;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.place.shared.PlaceHistoryHandler.Historian;
import com.google.gwt.uibinder.client.UiBinder;
import com.google.gwt.uibinder.client.UiFactory;
import com.google.gwt.uibinder.client.UiField;
import com.google.gwt.uibinder.client.UiHandler;
import com.google.gwt.user.client.ui.Button;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.HasText;
import com.google.gwt.user.client.ui.HasValue;
import com.google.gwt.user.client.ui.InlineHyperlink;
import com.google.gwt.user.client.ui.Widget;
import com.google.inject.Provider;
import com.gwtplatform.mvp.client.proxy.PlaceManager;
import com.gwtplatform.mvp.shared.proxy.PlaceRequest;
import com.gwtplatform.mvp.shared.proxy.PlaceRequest.Builder;
import org.gwtproject.editor.client.EditorDelegate;
import org.gwtproject.editor.client.ValueAwareEditor;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Persistable;
import java.util.Objects;
import javax.inject.Inject;
/**
* widget with error handling, to navigate and manage database entries.
*
* @author Manfred Tremmel
*
*/
public class AdminNavigationWidget> extends Composite
implements ValueAwareEditor>, HasValue>,
HandlesSelectedEntry {
interface Binder extends UiBinder> {
}
@UiField
Button newEntry;
@UiField
Button saveEntry;
@UiField
Button deleteEntry;
@UiField
InlineHyperlink firstEntry;
@UiField
InlineHyperlink previousEntry;
@UiField
LongBoxWithoutSeparator id;
@UiField
InlineHyperlink nextEntry;
@UiField
InlineHyperlink lastEntry;
@UiField
AdminNavigationSearch searchForm;
@UiField
PageableList searchResultList;
@UiField
HasText logMessages;
private final Provider> searchResultListProvider;
private final Provider adminNavigationSearchProvider;
private final PlaceManager placeManager;
private final Historian historian;
private AdminNavigation value;
private String link;
private String linkWithParameter;
private AbstractAdminPresenter presenter;
private boolean allowNew;
private boolean allowSave;
private boolean allowDelete;
private Display displaySearch;
/**
* Constructor for AdminNavigationWidget.
*/
@Inject
public AdminNavigationWidget(final Binder binder, final PlaceManager placeManager,
final Historian historian, final Provider> searchResultListProvider,
final Provider adminNavigationSearchProvider) {
super();
this.placeManager = placeManager;
this.historian = historian;
this.searchResultListProvider = searchResultListProvider;
this.adminNavigationSearchProvider = adminNavigationSearchProvider;
allowNew = true;
allowSave = true;
allowDelete = true;
initWidget(binder.createAndBindUi(this));
searchResultList.setParent(this);
displaySearch = Display.NONE;
searchForm.getElement().getStyle().setDisplay(displaySearch);
searchResultList.getElement().getStyle().setDisplay(displaySearch);
}
@Ignore
@UiFactory
public PageableList buildSearchResultList() {
return searchResultListProvider.get();
}
@Ignore
@UiFactory
public AdminNavigationSearch buildAdminNavigationSearch() {
return adminNavigationSearchProvider.get();
}
@Override
public void setValue(final AdminNavigation value) {
setValue(value, false);
}
@Override
public void setValue(final AdminNavigation value, final boolean fireEvents) {
final AdminNavigation oldValue = getValue();
this.value = value;
setLink(firstEntry, this.value.getFirstId());
setLink(previousEntry, this.value.getPreviousId());
setLink(nextEntry, this.value.getNextId());
setLink(lastEntry, this.value.getLastId());
deleteEntry.setEnabled(allowDelete && this.value.getCurrentId() != null);
id.setValue(this.value.getCurrentId());
switchHistory(this.value.getCurrentId());
if (fireEvents) {
ValueChangeEvent.fireIfNotEqual(this, oldValue, this.value);
}
}
@UiHandler("switchToId")
public void switchToEntry(final ClickEvent event) {
switchToEntry(id.getValue());
}
private void switchToEntry(final Long idToSwitchTo) {
if (idToSwitchTo != null) {
placeManager.revealPlace(generateRequestForId(idToSwitchTo), true);
}
}
private void switchHistory(final Long idToSwitchTo) {
final String oldToken = historian.getToken();
final String newToken = placeManager.buildHistoryToken(generateRequestForId(idToSwitchTo));
if (!Objects.equals(oldToken, newToken)) {
historian.newItem(newToken, false);
}
}
private PlaceRequest generateRequestForId(final Long entityId) {
final Builder placeRequestBuilder;
if (entityId == null) {
placeRequestBuilder = new PlaceRequest.Builder().nameToken(link);
} else {
placeRequestBuilder = new PlaceRequest.Builder().nameToken(linkWithParameter);
placeRequestBuilder.with(Parameters.ID, entityId.toString());
}
return placeRequestBuilder.build();
}
/**
* switch to typed in entry when pressing return/enter.
*
* @param event key press event
*/
@UiHandler("id")
public void switchToEntryOnReturn(final KeyPressEvent event) {
if (event.getCharCode() == KeyCodes.KEY_ENTER
|| event.getNativeEvent().getKeyCode() == KeyCodes.KEY_ENTER) {
switchToEntry(id.getValue());
}
}
/**
* pressed the new entry button.
*
* @param event click event
*/
@UiHandler("newEntry")
public void newEntry(final ClickEvent event) {
if (allowNew) {
presenter.newEntry();
}
}
/**
* delete the current entry.
*
* @param event click event
*/
@UiHandler("deleteEntry")
public void deleteEntry(final ClickEvent event) {
if (allowDelete) {
presenter.deleteEntry(value.getCurrentId());
}
}
/**
* toggle visibility of search form.
*
* @param event click event
*/
@UiHandler("searchEntry")
public void searchEntry(final ClickEvent event) {
displaySearch = displaySearch == Display.NONE ? Display.INITIAL : Display.NONE;
searchForm.getElement().getStyle().setDisplay(displaySearch);
searchResultList.getElement().getStyle()
.setDisplay(searchResultList.hasEntries() ? displaySearch : Display.NONE);
}
/**
* trigger search.
*
* @param event value change event
*/
@UiHandler("searchForm")
public void searchStart(final ValueChangeEvent event) {
presenter.search(event.getValue().toString());
}
/**
* pageable changed.
*
* @param event value change handler
*/
@UiHandler("searchResultList")
public void searchResultListChanged(final ValueChangeEvent event) {
presenter.search(searchForm.getValue().toString(), event.getValue());
}
/**
* set presenter.
*
* @param presenter of the administration page
*/
public void setPresenter(final AbstractAdminPresenter presenter) {
this.presenter = presenter;
searchResultList.setTableFieldDefinitions(this.presenter.getSearchResultDefinitions());
searchForm.fillSearchFieldDefinition(this.presenter.getSearchFieldDefinitions());
}
private void setLink(final InlineHyperlink inlineHyperlink, final Long entityId) {
inlineHyperlink.getElement().getStyle()
.setDisplay(entityId == null ? Display.NONE : Display.INLINE_BLOCK);
inlineHyperlink
.setTargetHistoryToken(placeManager.buildHistoryToken(generateRequestForId(entityId)));
}
public void setLink(final String link) {
this.link = link;
}
public void setLinkWithParameter(final String linkWithParameter) {
this.linkWithParameter = linkWithParameter;
}
@Override
public AdminNavigation getValue() {
return value;
}
@Override
public HandlerRegistration addValueChangeHandler(
final ValueChangeHandler> handler) {
return addHandler(handler, ValueChangeEvent.getType());
}
@Override
public void setDelegate(final EditorDelegate> delegate) {
delegate.subscribe();
}
@Override
public void onPropertyChange(final String... paths) {
// nothing to do
}
@Override
public void flush() {
// nothing to do
}
public Button getSaveEntry() {
return saveEntry;
}
public boolean isAllowNew() {
return allowNew;
}
public void setAllowNew(final boolean allowNew) {
this.allowNew = allowNew;
newEntry.setEnabled(this.allowNew);
}
public boolean isAllowSave() {
return allowSave;
}
public void setAllowSave(final boolean allowSave) {
this.allowSave = allowSave;
saveEntry.setEnabled(this.allowSave);
}
public boolean isAllowDelete() {
return allowDelete;
}
public void setAllowDelete(final boolean allowDelete) {
this.allowDelete = allowDelete;
deleteEntry.setEnabled(this.allowDelete);
}
/**
* display search results.
*
* @param result page object with search results
*/
public void displaySearchResult(final Page result) {
displaySearchResult(result, false);
}
/**
* display search results.
*
* @param result page object with search results
*/
public void displaySearchResult(final Page result, final boolean resetSorting) {
if (resetSorting) {
searchResultList.clearSort();
}
searchResultList.setValue(result);
searchResultList.getElement().getStyle()
.setDisplay(searchResultList.hasEntries() ? Display.INITIAL : Display.NONE);
}
@Override
public void handleSelectedEntry(final T entry) {
presenter.readEntry(entry.getId());
}
public void showMessage(final String message) {
logMessages.setText(message);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy