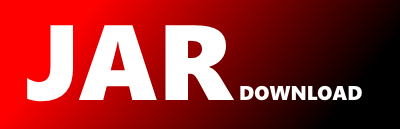
de.knightsoftnet.navigation.client.OwnPlaceManagerImpl Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.navigation.client;
import de.knightsoftnet.navigation.client.event.ChangePlaceEvent;
import de.knightsoftnet.navigation.client.ui.navigation.NavigationStructure;
import com.google.gwt.place.shared.PlaceHistoryHandler.Historian;
import com.google.web.bindery.event.shared.EventBus;
import com.gwtplatform.mvp.client.annotations.DefaultPlace;
import com.gwtplatform.mvp.client.annotations.ErrorPlace;
import com.gwtplatform.mvp.client.annotations.UnauthorizedPlace;
import com.gwtplatform.mvp.client.proxy.PlaceManagerImpl;
import com.gwtplatform.mvp.shared.proxy.PlaceRequest;
import com.gwtplatform.mvp.shared.proxy.TokenFormatter;
import org.apache.commons.lang3.StringUtils;
import java.util.Collections;
import javax.inject.Inject;
public class OwnPlaceManagerImpl extends PlaceManagerImpl {
private final PlaceRequest defaultPlaceRequest;
private final PlaceRequest errorPlaceRequest;
private final PlaceRequest unauthorizedPlaceRequest;
/**
* constructor injecting parameters.
*
* @param eventBus event bus
* @param tokenFormatter token formatter
* @param defaultPlaceNameToken default place name token
* @param errorPlaceNameToken error place name token
* @param unauthorizedPlaceNameToken unauthorized place name token
* @param historian historian
* @param navigationStructure navigation structure
*/
@Inject
public OwnPlaceManagerImpl(final EventBus eventBus, final TokenFormatter tokenFormatter,
@DefaultPlace final String defaultPlaceNameToken,
@ErrorPlace final String errorPlaceNameToken,
@UnauthorizedPlace final String unauthorizedPlaceNameToken, final Historian historian,
final NavigationStructure navigationStructure) {
super(eventBus, tokenFormatter, historian);
navigationStructure.setActiveNavigationEntryInterface(defaultPlaceNameToken);
if (StringUtils.contains(defaultPlaceNameToken, '{')) {
defaultPlaceRequest = new PlaceRequest.Builder().nameToken(defaultPlaceNameToken)
.with(Collections.emptyMap()).build();
} else {
defaultPlaceRequest = new PlaceRequest.Builder().nameToken(defaultPlaceNameToken).build();
}
if (StringUtils.contains(defaultPlaceNameToken, '{')) {
errorPlaceRequest = new PlaceRequest.Builder().nameToken(errorPlaceNameToken)
.with(Collections.emptyMap()).build();
} else {
errorPlaceRequest = new PlaceRequest.Builder().nameToken(errorPlaceNameToken).build();
}
if (StringUtils.contains(defaultPlaceNameToken, '{')) {
unauthorizedPlaceRequest = new PlaceRequest.Builder().nameToken(unauthorizedPlaceNameToken)
.with(Collections.emptyMap()).build();
} else {
unauthorizedPlaceRequest =
new PlaceRequest.Builder().nameToken(unauthorizedPlaceNameToken).build();
}
}
@Override
public void revealDefaultPlace() {
this.revealPlace(defaultPlaceRequest, false);
}
@Override
public void revealErrorPlace(final String invalidHistoryToken) {
this.revealPlace(errorPlaceRequest, false);
}
@Override
public void revealUnauthorizedPlace(final String unauthorizedHistoryToken) {
this.revealRelativePlace(unauthorizedPlaceRequest);
}
@Override
protected void doRevealPlace(final PlaceRequest request, final boolean updateBrowserUrl) {
super.doRevealPlace(request, updateBrowserUrl);
getEventBus().fireEvent(new ChangePlaceEvent(request));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy