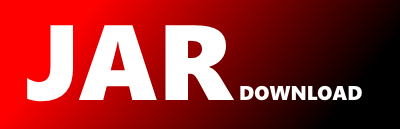
de.knightsoftnet.navigation.rebind.VersionInfoGenerator Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.navigation.rebind;
import com.google.gwt.core.ext.Generator;
import com.google.gwt.core.ext.GeneratorContext;
import com.google.gwt.core.ext.TreeLogger;
import com.google.gwt.core.ext.UnableToCompleteException;
import com.google.gwt.core.ext.typeinfo.JClassType;
import com.google.gwt.core.ext.typeinfo.NotFoundException;
import com.google.gwt.user.rebind.ClassSourceFileComposerFactory;
import com.google.gwt.user.rebind.SourceWriter;
import java.io.PrintWriter;
import java.util.ResourceBundle;
/**
* this class creates a implementation of the VersionInfoInterface with data from maven's pom.xml.
*
* @author Manfred Tremmel
*
*/
public class VersionInfoGenerator extends Generator {
private String simpleName;
@Override
public final String generate(final TreeLogger logger, final GeneratorContext context,
final String typeName) throws UnableToCompleteException {
try {
final JClassType classType = context.getTypeOracle().getType(typeName);
// Here you would retrieve the metadata based on typeName for this class
final SourceWriter src = getSourceWriter(classType, context, logger);
if (src != null) {
final ResourceBundle bundle = ResourceBundle.getBundle("Version");
final String version = bundle.getString("application.version");
final String buildTimeString = bundle.getString("build.timestamp");
final String copyright = bundle.getString("application.copyright");
final String author = bundle.getString("application.author");
src.println("public " + simpleName + "() {");
src.println(" super();");
src.println(" setCopyrightText(\"" + copyright + "\");");
src.println(" setVersionNumber(\"" + version + "\");");
src.println(" setVersionDate(\"" + buildTimeString + "\");");
src.println(" setAuthor(\"" + author + "\");");
src.println("}");
src.commit(logger);
System.out.println("Generating for: " + typeName);
}
return typeName + "Generated";
} catch (final NotFoundException e) {
e.printStackTrace();
}
return null;
}
/**
* get source writer for the generator.
*
* @param classType class type
* @param context generator context
* @param logger tree logger
* @return SourceWriter to write the generated sources to
*/
public final SourceWriter getSourceWriter(final JClassType classType,
final GeneratorContext context, final TreeLogger logger) {
final String packageName = classType.getPackage().getName();
simpleName = classType.getSimpleSourceName() + "Generated";
final ClassSourceFileComposerFactory composer =
new ClassSourceFileComposerFactory(packageName, simpleName);
composer.setSuperclass(classType.getName());
final PrintWriter printWriter = context.tryCreate(logger, packageName, simpleName);
if (printWriter == null) {
return null;
}
return composer.createSourceWriter(context, printWriter);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy