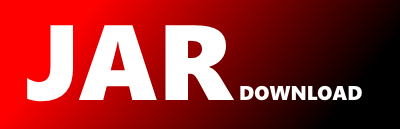
de.knightsoftnet.gwtp.spring.annotation.processor.BackofficePresenterCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwtp-spring-integration-client Show documentation
Show all versions of gwtp-spring-integration-client Show documentation
Basics to bring GWTP and Spring together.
package de.knightsoftnet.gwtp.spring.annotation.processor;
import de.knightsoftnet.gwtp.spring.client.annotation.BackofficeClientGenerator;
import de.knightsoftnet.gwtp.spring.client.annotation.SearchField;
import de.knightsoftnet.validators.shared.data.FieldTypeEnum;
import org.apache.commons.lang3.StringUtils;
import java.io.PrintWriter;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import javax.annotation.processing.ProcessingEnvironment;
import javax.lang.model.element.Element;
import javax.lang.model.element.TypeElement;
import javax.lang.model.type.DeclaredType;
/**
* Create gwtp presenter class.
*/
public class BackofficePresenterCreator
extends AbstractBackofficeCreator {
protected static final String CLASS_SUFFIX = "Presenter";
public BackofficePresenterCreator() {
super(CLASS_SUFFIX);
}
@Override
protected void addAdditionalImports(final String serverPackage, final Element element,
final BackofficeClientGenerator annotationInterface,
final ProcessingEnvironment processingEnv) {
addImports(//
"de.knightsoftnet.gwtp.spring.client.session.Session", //
"de.knightsoftnet.gwtp.spring.shared.search.SearchFieldDefinition", //
"de.knightsoftnet.gwtp.spring.shared.search.TableFieldDefinition", //
"de.knightsoftnet.mtwidgets.client.ui.page.admin.AbstractAdminPresenter", //
"de.knightsoftnet.mtwidgets.client.ui.request.DeleteRequestPresenter", //
"de.knightsoftnet.mtwidgets.client.ui.widget.helper.CountryMessages", //
"de.knightsoftnet.validators.shared.data.FieldTypeEnum", //
"com.google.web.bindery.event.shared.EventBus", //
"com.gwtplatform.dispatch.rest.delegates.client.ResourceDelegate", //
"com.gwtplatform.mvp.client.annotations.NameToken", //
"com.gwtplatform.mvp.client.annotations.ProxyCodeSplit", //
"com.gwtplatform.mvp.client.proxy.ProxyPlace", //
"org.apache.commons.lang3.StringUtils", //
"org.apache.commons.lang3.tuple.Pair", //
"java.util.Arrays", //
"java.util.Objects", //
"javax.inject.Inject");
final String entityName = getEntityNameOfElement(element);
addImport(element.asType());
addImport(serverPackage + "." + entityName + suffix + ".MyProxy");
addImport(serverPackage + "." + entityName + suffix + ".MyView");
addImports(annotationInterface.additionalPresenterImports());
}
@Override
protected void writeBody(final PrintWriter out, final String serverPackage, final Element element,
final BackofficeClientGenerator annotationInterface,
final ProcessingEnvironment processingEnv) {
final String entityName = getEntityNameOfElement(element);
out.print("public class ");
out.print(entityName);
out.print(suffix);
out.print(" extends AbstractAdminPresenter<");
out.print(entityName);
out.println(", MyProxy, MyView> {");
out.println();
out.print(" public interface MyView extends AbstractAdminPresenter.MyViewDef<");
out.print(entityName);
out.println(", MyProxy, MyView> {");
out.println(" }");
out.println();
out.println(" @ProxyCodeSplit");
out.print(" @NameToken({\"");
out.print(annotationInterface.baseToken());
out.print("\", \"");
out.print(annotationInterface.baseToken());
out.print("{" + de.knightsoftnet.validators.shared.Parameters.ID + "}");
out.println("\"})");
out.print(" public interface MyProxy extends ProxyPlace<");
out.print(entityName);
out.print(suffix);
out.println("> {");
out.println(" }");
out.println();
out.println(" @Inject");
out.print(" public ");
out.print(entityName);
out.print(suffix);
out.println("(final EventBus eventBus, final MyView view, final MyProxy proxy,");
out.print(" final ResourceDelegate<");
out.print(entityName);
out.print(BackofficeServiceCreator.CLASS_SUFFIX);
out.println("> service, final Session session,");
out.print(" final DeleteRequestPresenter deleteRequestPresenter, final ");
out.print(entityName);
out.print(BackofficeLocalInterfaceCreator.CLASS_SUFFIX);
out.print(" messages, final CountryMessages countryMessages");
for (final String param : annotationInterface.additionalPresenterConstructorPrarameters()) {
out.println(",");
out.print(" ");
out.print(param);
}
out.println(") {");
out.println(" super(eventBus, view, proxy, service, session, deleteRequestPresenter,");
out.println(" Arrays.asList(");
generateSearchFieldList(out, element, processingEnv);
out.println(" Arrays.asList(");
generateSearchResultList(out, annotationInterface, element, processingEnv);
out.println(" );");
out.println(" }");
out.println();
out.println(" @Override");
out.print(" protected ");
out.print(entityName);
out.println(" createNewEntry() {");
out.print(" return new ");
out.print(entityName);
out.println("();");
out.println(" }");
out.println("}");
}
private void generateSearchResultList(final PrintWriter out,
final BackofficeClientGenerator annotationInterface, final Element element,
final ProcessingEnvironment processingEnv) {
final Map widgets =
detectBackofficeWidgetsOfElementFlat(element, processingEnv).stream()
.collect(Collectors.toMap(BackofficeWidget::getName, widget -> widget));
final Iterator iterator = Stream.of(annotationInterface.searchResults())
.map(annotation -> searchResultEntry(annotation, annotationInterface, element, widgets))
.iterator();
while (iterator.hasNext()) {
out.print(" ");
out.print(iterator.next());
if (iterator.hasNext()) {
out.print(",");
} else {
out.print(")");
}
out.println();
}
}
private String searchResultEntry(final SearchField annotation,
final BackofficeClientGenerator annotationInterface, final Element element,
final Map widgets) {
final String fieldName = annotation.field();
final String cssClasses =
annotation.gridColumns() == 0 ? String.join(", ", annotation.styling())
: annotationInterface.javaGridClassesColumn()[annotation.gridColumns() - 1];
final String entityName = getEntityNameOfElement(element);
final StringBuilder sb = new StringBuilder();
sb.append("new TableFieldDefinition<");
sb.append(entityName);
sb.append(">(\"");
sb.append(fieldName);
if (widgets.get(fieldName).getFieldType() == FieldTypeEnum.STRING_LOCALIZED) {
sb.append(".localizedText");
}
sb.append("\", messages.");
sb.append(fieldName);
sb.append("(),\n");
sb.append(" ");
sb.append(generateToStringMethod(widgets.get(fieldName)));
sb.append(",\n");
sb.append(" ");
sb.append(cssClasses);
sb.append(")");
return sb.toString();
}
private String generateToStringMethod(final BackofficeWidget widget) {
// TODO incomplete implementation, handle additional stuff
final String getter = Stream.of(StringUtils.split(widget.getName(), '.'))
.map(name -> StringUtils.capitalize(name)).collect(Collectors.joining(".", "get", "()"));
switch (widget.getFieldType()) {
case DATE:
return "source -> source." + getter
+ ".format(java.time.format.DateTimeFormatter.ofLocalizedDate("
+ "java.time.format.FormatStyle.MEDIUM))";
case DATETIME:
if ("DateTimeLocalBoxLocalDateTime".equals(widget.getWidgetName())) {
return "source -> source." + getter
+ ".format(java.time.format.DateTimeFormatter.ofLocalizedDateTime("
+ "java.time.format.FormatStyle.MEDIUM))";
}
return "source -> new com.google.gwt.i18n.shared.DateTimeFormat("
+ "com.google.gwt.i18n.shared.DateTimeFormat.PredefinedFormat.DATE_TIME_MEDIUM)"
+ ".format(source." + getter + ")";
case TIME:
return "source -> source." + getter
+ ".format(java.time.format.DateTimeFormatter.ofLocalizedTime("
+ "java.time.format.FormatStyle.MEDIUM))";
case ENUM_FIXED:
if ("CountryListBox".equals(widget.getWidgetName())) {
return "source -> countryMessages.name(source." + getter + ")";
}
return "source -> messages." + widget.getName() + "(source." + getter + ")";
case STRING:
return "source -> source." + getter;
case STRING_LOCALIZED:
return "source -> source." + getter
+ ".getLocalizedText(com.google.gwt.i18n.client.LocaleInfo"
+ ".getCurrentLocale().getLocaleName())";
default:
return "source -> Objects.toString(source." + getter + ", StringUtils.EMPTY)";
}
}
private void generateSearchFieldList(final PrintWriter out, final Element element,
final ProcessingEnvironment processingEnv) {
final Iterator iterator =
detectBackofficeWidgetsOfElementFlat(element, processingEnv).stream()
.filter(widget -> widget.getFieldType() != FieldTypeEnum.EMBEDED
&& widget.getFieldType() != FieldTypeEnum.ONE_TO_MANY && !widget.isIgnore())
.map(widget -> elementToSearchOption(widget, processingEnv)).iterator();
while (iterator.hasNext()) {
out.print(" ");
out.print(iterator.next());
if (!iterator.hasNext()) {
out.print(")");
}
out.println(",");
}
}
private String elementToSearchOption(final BackofficeWidget widget,
final ProcessingEnvironment processingEnv) {
final StringBuilder sb = new StringBuilder();
sb.append("new SearchFieldDefinition(\"");
sb.append(widget.getName());
if (widget.getFieldType() == FieldTypeEnum.STRING_LOCALIZED) {
sb.append(".localizedText");
}
sb.append("\", messages.");
sb.append(fieldNameToCamelCase(widget.getName()));
sb.append("(), ");
sb.append("FieldTypeEnum.");
sb.append(widget.getFieldType().name());
if (widget.getFieldType() == FieldTypeEnum.ENUM_FIXED) {
sb.append(",\n");
sb.append(" Arrays.asList(\n");
final List enumValues = getEnumValues((TypeElement) ((DeclaredType) processingEnv
.getTypeUtils().asMemberOf(widget.getContaining(), widget.getField())).asElement());
final String messages =
"CountryListBox".equals(widget.getWidgetName()) ? "countryMessages" : "messages";
final String messageField = "CountryListBox".equals(widget.getWidgetName()) ? "name"
: fieldNameToCamelCase(widget.getName());
for (final String enumValue : enumValues) {
sb.append(" Pair.of(\"");
sb.append(enumValue);
sb.append("\", ");
sb.append(messages);
sb.append(".");
sb.append(messageField);
sb.append("(");
sb.append(widget.getField().asType().toString());
sb.append(".");
sb.append(enumValue);
sb.append("))");
if (!enumValues.get(enumValues.size() - 1).equals(enumValue)) {
sb.append(",");
}
sb.append("\n");
}
sb.append(" )");
}
sb.append(")");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy