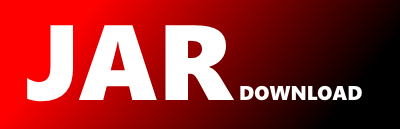
de.knightsoftnet.validators.shared.data.CreatePhoneCountryConstantsClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mt-bean-validators Show documentation
Show all versions of mt-bean-validators Show documentation
The MT Bean Validators is a collection of JSR-303/JSR-349/JSR-380 bean validators. It's extracted from
GWT Bean Validators and contains no dependencies to GWT.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.validators.shared.data;
import de.knightsoftnet.validators.shared.annotation.ReadProperties;
import java.util.Collections;
import java.util.Locale;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Read gwt constants from properties file on server side.
*
* @author Manfred Tremmel
*
*/
public class CreatePhoneCountryConstantsClass {
@ReadProperties(propertyName = "PhoneCountryNameConstants")
public interface PhoneCountryNameConstantsProperties extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneCountryNameConstants", locale = "de")
public interface PhoneCountryNameConstantsDeProperties extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneCountryCodeConstants")
public interface PhoneCountryCodeConstantsProperties extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneCountryTrunkAndExitCodesConstants")
public interface PhoneCountryTrunkAndExitCodesConstantsProperties extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode1Constants")
public interface PhoneRegionCode1Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode20Constants")
public interface PhoneRegionCode20Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode211Constants")
public interface PhoneRegionCode211Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode212Constants")
public interface PhoneRegionCode212Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode213Constants")
public interface PhoneRegionCode213Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode216Constants")
public interface PhoneRegionCode216Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode218Constants")
public interface PhoneRegionCode218Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode220Constants")
public interface PhoneRegionCode220Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode221Constants")
public interface PhoneRegionCode221Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode222Constants")
public interface PhoneRegionCode222Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode223Constants")
public interface PhoneRegionCode223Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode224Constants")
public interface PhoneRegionCode224Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode225Constants")
public interface PhoneRegionCode225Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode226Constants")
public interface PhoneRegionCode226Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode228Constants")
public interface PhoneRegionCode228Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode229Constants")
public interface PhoneRegionCode229Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode230Constants")
public interface PhoneRegionCode230Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode232Constants")
public interface PhoneRegionCode232Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode233Constants")
public interface PhoneRegionCode233Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode234Constants")
public interface PhoneRegionCode234Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode235Constants")
public interface PhoneRegionCode235Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode236Constants")
public interface PhoneRegionCode236Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode237Constants")
public interface PhoneRegionCode237Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode238Constants")
public interface PhoneRegionCode238Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode239Constants")
public interface PhoneRegionCode239Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode240Constants")
public interface PhoneRegionCode240Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode241Constants")
public interface PhoneRegionCode241Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode242Constants")
public interface PhoneRegionCode242Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode243Constants")
public interface PhoneRegionCode243Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode244Constants")
public interface PhoneRegionCode244Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode245Constants")
public interface PhoneRegionCode245Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode247Constants")
public interface PhoneRegionCode247Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode248Constants")
public interface PhoneRegionCode248Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode249Constants")
public interface PhoneRegionCode249Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode250Constants")
public interface PhoneRegionCode250Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode251Constants")
public interface PhoneRegionCode251Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode252Constants")
public interface PhoneRegionCode252Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode253Constants")
public interface PhoneRegionCode253Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode254Constants")
public interface PhoneRegionCode254Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode255Constants")
public interface PhoneRegionCode255Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode256Constants")
public interface PhoneRegionCode256Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode257Constants")
public interface PhoneRegionCode257Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode258Constants")
public interface PhoneRegionCode258Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode260Constants")
public interface PhoneRegionCode260Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode261Constants")
public interface PhoneRegionCode261Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode262Constants")
public interface PhoneRegionCode262Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode263Constants")
public interface PhoneRegionCode263Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode264Constants")
public interface PhoneRegionCode264Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode265Constants")
public interface PhoneRegionCode265Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode266Constants")
public interface PhoneRegionCode266Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode267Constants")
public interface PhoneRegionCode267Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode268Constants")
public interface PhoneRegionCode268Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode269Constants")
public interface PhoneRegionCode269Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode27Constants")
public interface PhoneRegionCode27Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode291Constants")
public interface PhoneRegionCode291Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode297Constants")
public interface PhoneRegionCode297Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode299Constants")
public interface PhoneRegionCode299Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode30Constants")
public interface PhoneRegionCode30Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode31Constants")
public interface PhoneRegionCode31Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode32Constants")
public interface PhoneRegionCode32Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode33Constants")
public interface PhoneRegionCode33Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode34Constants")
public interface PhoneRegionCode34Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode350Constants")
public interface PhoneRegionCode350Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode351Constants")
public interface PhoneRegionCode351Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode352Constants")
public interface PhoneRegionCode352Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode353Constants")
public interface PhoneRegionCode353Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode354Constants")
public interface PhoneRegionCode354Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode355Constants")
public interface PhoneRegionCode355Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode356Constants")
public interface PhoneRegionCode356Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode357Constants")
public interface PhoneRegionCode357Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode358Constants")
public interface PhoneRegionCode358Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode359Constants")
public interface PhoneRegionCode359Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode36Constants")
public interface PhoneRegionCode36Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode370Constants")
public interface PhoneRegionCode370Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode371Constants")
public interface PhoneRegionCode371Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode372Constants")
public interface PhoneRegionCode372Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode373Constants")
public interface PhoneRegionCode373Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode374Constants")
public interface PhoneRegionCode374Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode375Constants")
public interface PhoneRegionCode375Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode376Constants")
public interface PhoneRegionCode376Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode377Constants")
public interface PhoneRegionCode377Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode378Constants")
public interface PhoneRegionCode378Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode380Constants")
public interface PhoneRegionCode380Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode381Constants")
public interface PhoneRegionCode381Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode382Constants")
public interface PhoneRegionCode382Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode383Constants")
public interface PhoneRegionCode383Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode385Constants")
public interface PhoneRegionCode385Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode386Constants")
public interface PhoneRegionCode386Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode387Constants")
public interface PhoneRegionCode387Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode39Constants")
public interface PhoneRegionCode39Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode40Constants")
public interface PhoneRegionCode40Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode41Constants")
public interface PhoneRegionCode41Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode420Constants")
public interface PhoneRegionCode420Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode421Constants")
public interface PhoneRegionCode421Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode423Constants")
public interface PhoneRegionCode423Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode43Constants")
public interface PhoneRegionCode43Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode44Constants")
public interface PhoneRegionCode44Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode45Constants")
public interface PhoneRegionCode45Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode46Constants")
public interface PhoneRegionCode46Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode47Constants")
public interface PhoneRegionCode47Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode48Constants")
public interface PhoneRegionCode48Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode49Constants")
public interface PhoneRegionCode49Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode501Constants")
public interface PhoneRegionCode501Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode502Constants")
public interface PhoneRegionCode502Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode503Constants")
public interface PhoneRegionCode503Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode504Constants")
public interface PhoneRegionCode504Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode505Constants")
public interface PhoneRegionCode505Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode506Constants")
public interface PhoneRegionCode506Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode507Constants")
public interface PhoneRegionCode507Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode508Constants")
public interface PhoneRegionCode508Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode509Constants")
public interface PhoneRegionCode509Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode51Constants")
public interface PhoneRegionCode51Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode52Constants")
public interface PhoneRegionCode52Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode53Constants")
public interface PhoneRegionCode53Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode54Constants")
public interface PhoneRegionCode54Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode55Constants")
public interface PhoneRegionCode55Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode56Constants")
public interface PhoneRegionCode56Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode57Constants")
public interface PhoneRegionCode57Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode58Constants")
public interface PhoneRegionCode58Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode590Constants")
public interface PhoneRegionCode590Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode591Constants")
public interface PhoneRegionCode591Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode592Constants")
public interface PhoneRegionCode592Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode593Constants")
public interface PhoneRegionCode593Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode594Constants")
public interface PhoneRegionCode594Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode595Constants")
public interface PhoneRegionCode595Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode596Constants")
public interface PhoneRegionCode596Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode597Constants")
public interface PhoneRegionCode597Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode598Constants")
public interface PhoneRegionCode598Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode599Constants")
public interface PhoneRegionCode599Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode60Constants")
public interface PhoneRegionCode60Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode61Constants")
public interface PhoneRegionCode61Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode62Constants")
public interface PhoneRegionCode62Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode63Constants")
public interface PhoneRegionCode63Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode64Constants")
public interface PhoneRegionCode64Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode65Constants")
public interface PhoneRegionCode65Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode66Constants")
public interface PhoneRegionCode66Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode670Constants")
public interface PhoneRegionCode670Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode672Constants")
public interface PhoneRegionCode672Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode673Constants")
public interface PhoneRegionCode673Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode674Constants")
public interface PhoneRegionCode674Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode675Constants")
public interface PhoneRegionCode675Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode676Constants")
public interface PhoneRegionCode676Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode677Constants")
public interface PhoneRegionCode677Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode678Constants")
public interface PhoneRegionCode678Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode679Constants")
public interface PhoneRegionCode679Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode680Constants")
public interface PhoneRegionCode680Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode681Constants")
public interface PhoneRegionCode681Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode682Constants")
public interface PhoneRegionCode682Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode683Constants")
public interface PhoneRegionCode683Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode685Constants")
public interface PhoneRegionCode685Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode686Constants")
public interface PhoneRegionCode686Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode687Constants")
public interface PhoneRegionCode687Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode688Constants")
public interface PhoneRegionCode688Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode689Constants")
public interface PhoneRegionCode689Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode690Constants")
public interface PhoneRegionCode690Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode691Constants")
public interface PhoneRegionCode691Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode692Constants")
public interface PhoneRegionCode692Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode7Constants")
public interface PhoneRegionCode7Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode81Constants")
public interface PhoneRegionCode81Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode82Constants")
public interface PhoneRegionCode82Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode84Constants")
public interface PhoneRegionCode84Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode850Constants")
public interface PhoneRegionCode850Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode852Constants")
public interface PhoneRegionCode852Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode853Constants")
public interface PhoneRegionCode853Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode855Constants")
public interface PhoneRegionCode855Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode86Constants")
public interface PhoneRegionCode86Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode878Constants")
public interface PhoneRegionCode878Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode880Constants")
public interface PhoneRegionCode880Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode881Constants")
public interface PhoneRegionCode881Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode882Constants")
public interface PhoneRegionCode882Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode886Constants")
public interface PhoneRegionCode886Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode90Constants")
public interface PhoneRegionCode90Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode91Constants")
public interface PhoneRegionCode91Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode92Constants")
public interface PhoneRegionCode92Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode93Constants")
public interface PhoneRegionCode93Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode94Constants")
public interface PhoneRegionCode94Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode95Constants")
public interface PhoneRegionCode95Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode960Constants")
public interface PhoneRegionCode960Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode961Constants")
public interface PhoneRegionCode961Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode962Constants")
public interface PhoneRegionCode962Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode963Constants")
public interface PhoneRegionCode963Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode964Constants")
public interface PhoneRegionCode964Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode965Constants")
public interface PhoneRegionCode965Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode966Constants")
public interface PhoneRegionCode966Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode967Constants")
public interface PhoneRegionCode967Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode968Constants")
public interface PhoneRegionCode968Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode970Constants")
public interface PhoneRegionCode970Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode971Constants")
public interface PhoneRegionCode971Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode972Constants")
public interface PhoneRegionCode972Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode973Constants")
public interface PhoneRegionCode973Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode974Constants")
public interface PhoneRegionCode974Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode975Constants")
public interface PhoneRegionCode975Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode976Constants")
public interface PhoneRegionCode976Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode977Constants")
public interface PhoneRegionCode977Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode98Constants")
public interface PhoneRegionCode98Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode992Constants")
public interface PhoneRegionCode992Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode993Constants")
public interface PhoneRegionCode993Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode994Constants")
public interface PhoneRegionCode994Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode995Constants")
public interface PhoneRegionCode995Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode996Constants")
public interface PhoneRegionCode996Constants extends PropertiesMapConstants {
}
@ReadProperties(propertyName = "PhoneRegionCode998Constants")
public interface PhoneRegionCode998Constants extends PropertiesMapConstants {
}
private static volatile Map phoneCountryConstants =
new ConcurrentHashMap<>();
private static Map PHONE_REGION_CODE_MAP =
Stream.of(new Object[][] {
{"1", new PhoneRegionCode1ConstantsImpl()}, {"20", new PhoneRegionCode20ConstantsImpl()},
{"211", new PhoneRegionCode211ConstantsImpl()},
{"212", new PhoneRegionCode212ConstantsImpl()},
{"213", new PhoneRegionCode213ConstantsImpl()},
{"216", new PhoneRegionCode216ConstantsImpl()},
{"218", new PhoneRegionCode218ConstantsImpl()},
{"220", new PhoneRegionCode220ConstantsImpl()},
{"221", new PhoneRegionCode221ConstantsImpl()},
{"222", new PhoneRegionCode222ConstantsImpl()},
{"223", new PhoneRegionCode223ConstantsImpl()},
{"224", new PhoneRegionCode224ConstantsImpl()},
{"225", new PhoneRegionCode225ConstantsImpl()},
{"226", new PhoneRegionCode226ConstantsImpl()},
{"228", new PhoneRegionCode228ConstantsImpl()},
{"229", new PhoneRegionCode229ConstantsImpl()},
{"230", new PhoneRegionCode230ConstantsImpl()},
{"232", new PhoneRegionCode232ConstantsImpl()},
{"233", new PhoneRegionCode233ConstantsImpl()},
{"234", new PhoneRegionCode234ConstantsImpl()},
{"235", new PhoneRegionCode235ConstantsImpl()},
{"236", new PhoneRegionCode236ConstantsImpl()},
{"237", new PhoneRegionCode237ConstantsImpl()},
{"238", new PhoneRegionCode238ConstantsImpl()},
{"239", new PhoneRegionCode239ConstantsImpl()},
{"240", new PhoneRegionCode240ConstantsImpl()},
{"241", new PhoneRegionCode241ConstantsImpl()},
{"242", new PhoneRegionCode242ConstantsImpl()},
{"243", new PhoneRegionCode243ConstantsImpl()},
{"244", new PhoneRegionCode244ConstantsImpl()},
{"245", new PhoneRegionCode245ConstantsImpl()},
{"247", new PhoneRegionCode247ConstantsImpl()},
{"248", new PhoneRegionCode248ConstantsImpl()},
{"249", new PhoneRegionCode249ConstantsImpl()},
{"250", new PhoneRegionCode250ConstantsImpl()},
{"251", new PhoneRegionCode251ConstantsImpl()},
{"252", new PhoneRegionCode252ConstantsImpl()},
{"253", new PhoneRegionCode253ConstantsImpl()},
{"254", new PhoneRegionCode254ConstantsImpl()},
{"255", new PhoneRegionCode255ConstantsImpl()},
{"256", new PhoneRegionCode256ConstantsImpl()},
{"257", new PhoneRegionCode257ConstantsImpl()},
{"258", new PhoneRegionCode258ConstantsImpl()},
{"260", new PhoneRegionCode260ConstantsImpl()},
{"261", new PhoneRegionCode261ConstantsImpl()},
{"262", new PhoneRegionCode262ConstantsImpl()},
{"263", new PhoneRegionCode263ConstantsImpl()},
{"264", new PhoneRegionCode264ConstantsImpl()},
{"265", new PhoneRegionCode265ConstantsImpl()},
{"266", new PhoneRegionCode266ConstantsImpl()},
{"267", new PhoneRegionCode267ConstantsImpl()},
{"268", new PhoneRegionCode268ConstantsImpl()},
{"269", new PhoneRegionCode269ConstantsImpl()},
{"27", new PhoneRegionCode27ConstantsImpl()},
{"291", new PhoneRegionCode291ConstantsImpl()},
{"287", new PhoneRegionCode297ConstantsImpl()},
{"299", new PhoneRegionCode299ConstantsImpl()},
{"30", new PhoneRegionCode30ConstantsImpl()},
{"31", new PhoneRegionCode31ConstantsImpl()},
{"32", new PhoneRegionCode32ConstantsImpl()},
{"33", new PhoneRegionCode33ConstantsImpl()},
{"34", new PhoneRegionCode34ConstantsImpl()},
{"350", new PhoneRegionCode350ConstantsImpl()},
{"351", new PhoneRegionCode351ConstantsImpl()},
{"352", new PhoneRegionCode352ConstantsImpl()},
{"353", new PhoneRegionCode353ConstantsImpl()},
{"354", new PhoneRegionCode354ConstantsImpl()},
{"355", new PhoneRegionCode355ConstantsImpl()},
{"356", new PhoneRegionCode356ConstantsImpl()},
{"357", new PhoneRegionCode357ConstantsImpl()},
{"358", new PhoneRegionCode358ConstantsImpl()},
{"359", new PhoneRegionCode359ConstantsImpl()},
{"36", new PhoneRegionCode36ConstantsImpl()},
{"370", new PhoneRegionCode370ConstantsImpl()},
{"371", new PhoneRegionCode371ConstantsImpl()},
{"372", new PhoneRegionCode372ConstantsImpl()},
{"373", new PhoneRegionCode373ConstantsImpl()},
{"374", new PhoneRegionCode374ConstantsImpl()},
{"375", new PhoneRegionCode375ConstantsImpl()},
{"376", new PhoneRegionCode376ConstantsImpl()},
{"377", new PhoneRegionCode377ConstantsImpl()},
{"378", new PhoneRegionCode378ConstantsImpl()},
{"380", new PhoneRegionCode380ConstantsImpl()},
{"381", new PhoneRegionCode381ConstantsImpl()},
{"382", new PhoneRegionCode382ConstantsImpl()},
{"383", new PhoneRegionCode383ConstantsImpl()},
{"385", new PhoneRegionCode385ConstantsImpl()},
{"386", new PhoneRegionCode386ConstantsImpl()},
{"387", new PhoneRegionCode387ConstantsImpl()},
{"39", new PhoneRegionCode39ConstantsImpl()},
{"40", new PhoneRegionCode40ConstantsImpl()},
{"41", new PhoneRegionCode41ConstantsImpl()},
{"420", new PhoneRegionCode420ConstantsImpl()},
{"421", new PhoneRegionCode421ConstantsImpl()},
{"423", new PhoneRegionCode423ConstantsImpl()},
{"43", new PhoneRegionCode43ConstantsImpl()},
{"44", new PhoneRegionCode44ConstantsImpl()},
{"45", new PhoneRegionCode45ConstantsImpl()},
{"46", new PhoneRegionCode46ConstantsImpl()},
{"47", new PhoneRegionCode47ConstantsImpl()},
{"48", new PhoneRegionCode48ConstantsImpl()},
{"49", new PhoneRegionCode49ConstantsImpl()},
{"501", new PhoneRegionCode501ConstantsImpl()},
{"502", new PhoneRegionCode502ConstantsImpl()},
{"503", new PhoneRegionCode503ConstantsImpl()},
{"504", new PhoneRegionCode504ConstantsImpl()},
{"505", new PhoneRegionCode505ConstantsImpl()},
{"506", new PhoneRegionCode506ConstantsImpl()},
{"507", new PhoneRegionCode507ConstantsImpl()},
{"508", new PhoneRegionCode508ConstantsImpl()},
{"509", new PhoneRegionCode509ConstantsImpl()},
{"51", new PhoneRegionCode51ConstantsImpl()},
{"52", new PhoneRegionCode52ConstantsImpl()},
{"53", new PhoneRegionCode53ConstantsImpl()},
{"54", new PhoneRegionCode54ConstantsImpl()},
{"55", new PhoneRegionCode55ConstantsImpl()},
{"56", new PhoneRegionCode56ConstantsImpl()},
{"57", new PhoneRegionCode57ConstantsImpl()},
{"58", new PhoneRegionCode58ConstantsImpl()},
{"590", new PhoneRegionCode590ConstantsImpl()},
{"591", new PhoneRegionCode591ConstantsImpl()},
{"592", new PhoneRegionCode592ConstantsImpl()},
{"593", new PhoneRegionCode593ConstantsImpl()},
{"594", new PhoneRegionCode594ConstantsImpl()},
{"595", new PhoneRegionCode595ConstantsImpl()},
{"596", new PhoneRegionCode596ConstantsImpl()},
{"597", new PhoneRegionCode597ConstantsImpl()},
{"598", new PhoneRegionCode598ConstantsImpl()},
{"599", new PhoneRegionCode599ConstantsImpl()},
{"60", new PhoneRegionCode60ConstantsImpl()},
{"61", new PhoneRegionCode61ConstantsImpl()},
{"62", new PhoneRegionCode62ConstantsImpl()},
{"63", new PhoneRegionCode63ConstantsImpl()},
{"64", new PhoneRegionCode64ConstantsImpl()},
{"65", new PhoneRegionCode65ConstantsImpl()},
{"66", new PhoneRegionCode66ConstantsImpl()},
{"670", new PhoneRegionCode670ConstantsImpl()},
{"672", new PhoneRegionCode672ConstantsImpl()},
{"673", new PhoneRegionCode673ConstantsImpl()},
{"674", new PhoneRegionCode674ConstantsImpl()},
{"675", new PhoneRegionCode675ConstantsImpl()},
{"676", new PhoneRegionCode676ConstantsImpl()},
{"677", new PhoneRegionCode677ConstantsImpl()},
{"678", new PhoneRegionCode678ConstantsImpl()},
{"679", new PhoneRegionCode679ConstantsImpl()},
{"680", new PhoneRegionCode680ConstantsImpl()},
{"681", new PhoneRegionCode681ConstantsImpl()},
{"682", new PhoneRegionCode682ConstantsImpl()},
{"683", new PhoneRegionCode683ConstantsImpl()},
{"685", new PhoneRegionCode685ConstantsImpl()},
{"686", new PhoneRegionCode686ConstantsImpl()},
{"687", new PhoneRegionCode687ConstantsImpl()},
{"688", new PhoneRegionCode688ConstantsImpl()},
{"689", new PhoneRegionCode689ConstantsImpl()},
{"690", new PhoneRegionCode690ConstantsImpl()},
{"691", new PhoneRegionCode691ConstantsImpl()},
{"692", new PhoneRegionCode692ConstantsImpl()},
{"7", new PhoneRegionCode7ConstantsImpl()}, {"81", new PhoneRegionCode81ConstantsImpl()},
{"82", new PhoneRegionCode82ConstantsImpl()},
{"84", new PhoneRegionCode84ConstantsImpl()},
{"850", new PhoneRegionCode850ConstantsImpl()},
{"852", new PhoneRegionCode852ConstantsImpl()},
{"853", new PhoneRegionCode853ConstantsImpl()},
{"855", new PhoneRegionCode855ConstantsImpl()},
{"86", new PhoneRegionCode86ConstantsImpl()},
{"878", new PhoneRegionCode878ConstantsImpl()},
{"880", new PhoneRegionCode880ConstantsImpl()},
{"881", new PhoneRegionCode881ConstantsImpl()},
{"882", new PhoneRegionCode882ConstantsImpl()},
{"886", new PhoneRegionCode886ConstantsImpl()},
{"90", new PhoneRegionCode90ConstantsImpl()},
{"91", new PhoneRegionCode91ConstantsImpl()},
{"92", new PhoneRegionCode92ConstantsImpl()},
{"93", new PhoneRegionCode93ConstantsImpl()},
{"94", new PhoneRegionCode94ConstantsImpl()},
{"95", new PhoneRegionCode95ConstantsImpl()},
{"960", new PhoneRegionCode960ConstantsImpl()},
{"961", new PhoneRegionCode961ConstantsImpl()},
{"962", new PhoneRegionCode962ConstantsImpl()},
{"963", new PhoneRegionCode963ConstantsImpl()},
{"964", new PhoneRegionCode964ConstantsImpl()},
{"965", new PhoneRegionCode965ConstantsImpl()},
{"966", new PhoneRegionCode966ConstantsImpl()},
{"967", new PhoneRegionCode967ConstantsImpl()},
{"968", new PhoneRegionCode968ConstantsImpl()},
{"970", new PhoneRegionCode970ConstantsImpl()},
{"971", new PhoneRegionCode971ConstantsImpl()},
{"972", new PhoneRegionCode972ConstantsImpl()},
{"973", new PhoneRegionCode973ConstantsImpl()},
{"974", new PhoneRegionCode974ConstantsImpl()},
{"975", new PhoneRegionCode975ConstantsImpl()},
{"976", new PhoneRegionCode976ConstantsImpl()},
{"977", new PhoneRegionCode977ConstantsImpl()},
{"98", new PhoneRegionCode98ConstantsImpl()},
{"992", new PhoneRegionCode992ConstantsImpl()},
{"993", new PhoneRegionCode993ConstantsImpl()},
{"994", new PhoneRegionCode994ConstantsImpl()},
{"995", new PhoneRegionCode995ConstantsImpl()},
{"996", new PhoneRegionCode996ConstantsImpl()},
{"998", new PhoneRegionCode998ConstantsImpl()}
}).collect(
Collectors.toMap(data -> (String) data[0], data -> (PropertiesMapConstants) data[1]));
/**
* Instantiates a class via deferred binding.
*
* @return the new instance, which must be cast to the requested class
*/
public static PhoneCountrySharedConstants create() {
return create(Locale.ROOT);
}
/**
* Instantiates a class via deferred binding.
*
* @param locale language to create data for
* @return the new instance, which must be cast to the requested class
*/
public static PhoneCountrySharedConstants create(final Locale locale) {
if (!phoneCountryConstants.containsKey(locale)) { // NOPMD
synchronized (PhoneCountryConstantsImpl.class) {
if (!phoneCountryConstants.containsKey(locale)) {
phoneCountryConstants.put(locale,
AbstractCreateClass.createPhoneCountryConstants(locale));
}
}
}
return phoneCountryConstants.get(locale);
}
/**
* read phone country names.
*
* @param locale language to create data for
* @return map of phone country code and name
*/
public static Map readPhoneCountryNames(final Locale locale) {
final PropertiesMapConstants provider = Objects.toString(locale, "").startsWith("de")
? new PhoneCountryNameConstantsDePropertiesImpl()
: new PhoneCountryNameConstantsPropertiesImpl();
return provider.properties();
}
/**
* read phone country codes and country iso codes.
*
* @param locale language to create data for
* @return map of phone country code and country iso code
*/
public static Map readPhoneCountryCodes(final Locale locale) {
final PhoneCountryCodeConstantsProperties provider =
new PhoneCountryCodeConstantsPropertiesImpl();
return provider.properties();
}
/**
* read phone region codes for one country.
*
* @param phoneCountryCode country code of the phone number
* @param locale language to create data for
* @return map of area code and area name
*/
public static Map readPhoneRegionCodes(final String phoneCountryCode,
final Locale locale) {
if (PHONE_REGION_CODE_MAP.containsKey(phoneCountryCode)) {
return PHONE_REGION_CODE_MAP.get(phoneCountryCode).properties();
}
return Collections.emptyMap();
}
/**
* read phone trunk an exit code map from property file.
*
* @param locale language to create data for
* @return map of country code and combined string of trunk an exit code
*/
public static Map readPhoneTrunkAndExitCodes(final Locale locale) {
final PhoneCountryTrunkAndExitCodesConstantsProperties provider =
new PhoneCountryTrunkAndExitCodesConstantsPropertiesImpl();
return provider.properties();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy