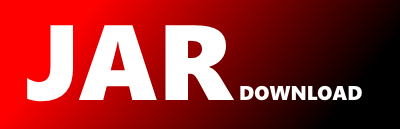
de.knightsoftnet.validators.shared.impl.BicValueValidator Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional information regarding
* copyright ownership. The ASF licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package de.knightsoftnet.validators.shared.impl;
import de.knightsoftnet.validators.shared.BicValue;
import de.knightsoftnet.validators.shared.data.BicMapSharedConstants;
import de.knightsoftnet.validators.shared.util.HasSetBicMapSharedConstants;
import de.knightsoftnet.validators.shared.util.IbanUtil;
import org.apache.commons.lang3.StringUtils;
import java.util.Objects;
import jakarta.validation.ConstraintValidator;
import jakarta.validation.ConstraintValidatorContext;
/**
* Check a string if it's a valid BIC.
*
* @author Manfred Tremmel
*
*/
public class BicValueValidator
implements ConstraintValidator, HasSetBicMapSharedConstants {
/**
* should whitespaces be ignored (true/false).
*/
private boolean ignoreWhitspaces;
/**
* provider for map of swift countries and the length of the ibans.
*/
private BicMapSharedConstants bicMapSharedConstants;
/**
* {@inheritDoc} initialize the validator.
*
* @see jakarta.validation.ConstraintValidator#initialize(java.lang.annotation.Annotation)
*/
@Override
public final void initialize(final BicValue constraintAnnotation) {
ignoreWhitspaces = constraintAnnotation.ignoreWhitspaces();
final IbanUtil ibanUtil = new IbanUtil();
ibanUtil.setBicMapSharedConstantsWhenAvailable(this);
}
@Override
public void setBicMapSharedConstants(final BicMapSharedConstants bicMapSharedConstants) {
this.bicMapSharedConstants = bicMapSharedConstants;
}
/**
* {@inheritDoc} check if given string is a valid BIC.
*
* @see jakarta.validation.ConstraintValidator#isValid(java.lang.Object,
* jakarta.validation.ConstraintValidatorContext)
*/
@Override
public final boolean isValid(final Object value, final ConstraintValidatorContext context) {
final String valueAsString;
if (ignoreWhitspaces) {
valueAsString =
Objects.toString(value, StringUtils.EMPTY).replaceAll("\\s+", StringUtils.EMPTY);
} else {
valueAsString = Objects.toString(value, null);
}
if (StringUtils.isEmpty(valueAsString)) {
// empty field is ok
return true;
}
if (valueAsString.length() != BicValidator.BIC_LENGTH_MIN
&& valueAsString.length() != BicValidator.BIC_LENGTH_MAX) {
// to short or to long, but it's handled by size validator!
return true;
}
if (!valueAsString.matches(BicValidator.BIC_REGEX)) {
// format is wrong!
return false;
}
return bicMapSharedConstants == null || bicMapSharedConstants.bics().containsKey(valueAsString)
|| bicMapSharedConstants.bics()
.containsKey(StringUtils.substring(valueAsString, 0, BicValidator.BIC_LENGTH_MIN));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy