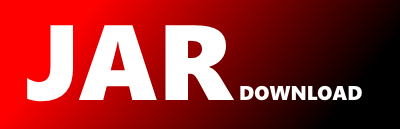
de.larsgrefer.sass.embedded.connection.StreamConnection Maven / Gradle / Ivy
package de.larsgrefer.sass.embedded.connection;
import com.google.protobuf.TextFormat;
import com.sass_lang.embedded_protocol.InboundMessage;
import com.sass_lang.embedded_protocol.OutboundMessage;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
/**
* {@link CompilerConnection} implementation based on an {@link InputStream} {@link OutputStream} pair.
*
* @author Lars Grefer
*/
@Slf4j
public abstract class StreamConnection implements CompilerConnection {
protected abstract InputStream getInputStream() throws IOException;
protected abstract OutputStream getOutputStream() throws IOException;
@Override
public synchronized void sendMessage(Packet packet) throws IOException {
if (log.isTraceEnabled()) {
log.trace("{} --> {}", packet.getCompilationId(), TextFormat.printer().shortDebugString(packet.getMessage()));
}
if (packet.getMessage().hasVersionRequest()) {
packet.setCompilationId(0);
}
OutputStream outputStream = getOutputStream();
packet.writeDelimitedTo(outputStream);
outputStream.flush();
}
@Override
public synchronized Packet readResponse() throws IOException {
Packet packet = Packet.parseDelimitedFrom(getInputStream(), OutboundMessage.parser());
if (log.isTraceEnabled()) {
log.trace("{} <-- {}", packet.getCompilationId(), TextFormat.printer().shortDebugString(packet.getMessage()));
}
return packet;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy