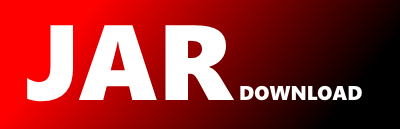
de.larsgrefer.sass.embedded.functions.HostFunctionFactory Maven / Gradle / Ivy
package de.larsgrefer.sass.embedded.functions;
import lombok.experimental.UtilityClass;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.function.BiFunction;
import java.util.function.Function;
/**
* @author Lars Grefer
*/
@UtilityClass
public class HostFunctionFactory {
public List allSassFunctions(@Nonnull T object) {
return allSassFunctions((Class) object.getClass(), object);
}
public List allSassFunctions(@Nonnull Class> clazz) {
return allSassFunctions(clazz, null);
}
public List allSassFunctions(@Nonnull Class clazz, @Nullable T object) {
List result = new ArrayList<>();
Method[] methods = clazz.getMethods();
for (Method method : methods) {
if (method.isAnnotationPresent(SassFunction.class)) {
result.add(ofMethod(method, object));
}
}
return result;
}
public HostFunction ofMethod(Method method) {
return ofMethod(method, null);
}
public HostFunction ofMethod(@Nonnull Method method, @Nullable Object targetObject) {
return new ReflectiveHostFunction(method, targetObject);
}
public HostFunction ofLambda(String name, Callable> lambda) {
return new CallableHostFunction(name, lambda);
}
public HostFunction ofLambda(String name, Class argType, Function lambda) {
return new FunctionHostFunction<>(name, argType, lambda);
}
public HostFunction ofLambda(String name, Class arg0Type, Class arg1Type, BiFunction lambda) {
return new BiFunctionHostFunction<>(name, arg0Type, arg1Type, lambda);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy