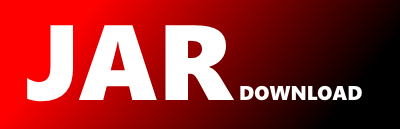
de.larsgrefer.sass.embedded.importer.ClasspathImporter Maven / Gradle / Ivy
package de.larsgrefer.sass.embedded.importer;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
/**
* @author Lars Grefer
*/
@Slf4j
@RequiredArgsConstructor
public class ClasspathImporter extends CustomUrlImporter {
private final ClassLoader classLoader;
public ClasspathImporter() {
this(ClasspathImporter.class.getClassLoader());
}
@Override
public URL canonicalizeUrl(String url) throws IOException {
Enumeration foundResources = classLoader.getResources(url);
List foundUrls = getNonDirectoryUrls(foundResources);
if (foundUrls.isEmpty()) {
return null;
}
else if (foundUrls.size() == 1) {
return foundUrls.get(0);
}
else {
throw new IllegalStateException(String.format("Import of '%s' found %d results.", url, foundUrls.size()));
}
}
@Nonnull
private List getNonDirectoryUrls(Enumeration foundResources) throws IOException {
List foundUrls = new ArrayList<>();
while (foundResources.hasMoreElements()) {
URL candidate = foundResources.nextElement();
if (isFile(candidate))
foundUrls.add(candidate);
}
return foundUrls;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy