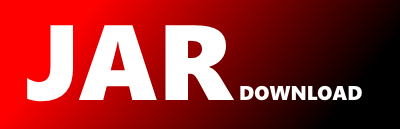
com.sass_lang.embedded_protocol.InboundMessage Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: sass-embedded-protocol-3.1.0/spec/embedded_sass.proto
// Protobuf Java Version: 4.28.2
package com.sass_lang.embedded_protocol;
/**
*
* The wrapper type for all messages sent from the host to the compiler. This
* provides a `oneof` that makes it possible to determine the type of each
* inbound message.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage}
*/
public final class InboundMessage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage)
InboundMessageOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
InboundMessage.class.getName());
}
// Use InboundMessage.newBuilder() to construct.
private InboundMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private InboundMessage() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.class, com.sass_lang.embedded_protocol.InboundMessage.Builder.class);
}
public interface VersionRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.VersionRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* This version request's id.
*
*
* uint32 id = 1;
* @return The id.
*/
int getId();
}
/**
*
* A request for information about the version of the embedded compiler. The
* host can use this to provide diagnostic information to the user, to check
* which features the compiler supports, or to ensure that it's compatible
* with the same protocol version the compiler supports.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.VersionRequest}
*/
public static final class VersionRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.VersionRequest)
VersionRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
VersionRequest.class.getName());
}
// Use VersionRequest.newBuilder() to construct.
private VersionRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private VersionRequest() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_VersionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_VersionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.class, com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private int id_ = 0;
/**
*
* This version request's id.
*
*
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0) {
output.writeUInt32(1, id_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.VersionRequest)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest other = (com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) obj;
if (getId()
!= other.getId()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.VersionRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A request for information about the version of the embedded compiler. The
* host can use this to provide diagnostic information to the user, to check
* which features the compiler supports, or to ensure that it's compatible
* with the same protocol version the compiler supports.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.VersionRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.VersionRequest)
com.sass_lang.embedded_protocol.InboundMessage.VersionRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_VersionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_VersionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.class, com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_VersionRequest_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequest getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequest build() {
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequest buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest result = new com.sass_lang.embedded_protocol.InboundMessage.VersionRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.VersionRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.VersionRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.VersionRequest other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance()) return this;
if (other.getId() != 0) {
setId(other.getId());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
id_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int id_ ;
/**
*
* This version request's id.
*
*
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
*
* This version request's id.
*
*
* uint32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* This version request's id.
*
*
* uint32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.VersionRequest)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.VersionRequest)
private static final com.sass_lang.embedded_protocol.InboundMessage.VersionRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.VersionRequest();
}
public static com.sass_lang.embedded_protocol.InboundMessage.VersionRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VersionRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompileRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.CompileRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
* @return Whether the string field is set.
*/
boolean hasString();
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
* @return The string.
*/
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput getString();
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInputOrBuilder getStringOrBuilder();
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return Whether the path field is set.
*/
boolean hasPath();
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return The path.
*/
java.lang.String getPath();
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @return The enum numeric value on the wire for style.
*/
int getStyleValue();
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @return The style.
*/
com.sass_lang.embedded_protocol.OutputStyle getStyle();
/**
*
* Whether to generate a source map. Note that this will *not* add a source
* map comment to the stylesheet; that's up to the host or its users.
*
*
* bool source_map = 5;
* @return The sourceMap.
*/
boolean getSourceMap();
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
java.util.List
getImportersList();
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getImporters(int index);
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
int getImportersCount();
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
java.util.List extends com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder>
getImportersOrBuilderList();
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder getImportersOrBuilder(
int index);
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @return A list containing the globalFunctions.
*/
java.util.List
getGlobalFunctionsList();
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @return The count of globalFunctions.
*/
int getGlobalFunctionsCount();
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param index The index of the element to return.
* @return The globalFunctions at the given index.
*/
java.lang.String getGlobalFunctions(int index);
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param index The index of the value to return.
* @return The bytes of the globalFunctions at the given index.
*/
com.google.protobuf.ByteString
getGlobalFunctionsBytes(int index);
/**
*
* Whether to use terminal colors in the formatted message of errors and
* logs.
*
*
* bool alert_color = 8;
* @return The alertColor.
*/
boolean getAlertColor();
/**
*
* Whether to encode the formatted message of errors and logs in ASCII.
*
*
* bool alert_ascii = 9;
* @return The alertAscii.
*/
boolean getAlertAscii();
/**
*
* Whether to report all deprecation warnings or only the first few ones.
* If this is `false`, the compiler may choose not to send events for
* repeated deprecation warnings. If this is `true`, the compiler must emit
* an event for every deprecation warning it encounters.
*
*
* bool verbose = 10;
* @return The verbose.
*/
boolean getVerbose();
/**
*
* Whether to omit events for deprecation warnings coming from dependencies
* (files loaded from a different importer than the input).
*
*
* bool quiet_deps = 11;
* @return The quietDeps.
*/
boolean getQuietDeps();
/**
*
* Whether to include sources in the generated sourcemap
*
*
* bool source_map_include_sources = 12;
* @return The sourceMapIncludeSources.
*/
boolean getSourceMapIncludeSources();
/**
*
* Whether to emit a `@charset`/BOM for non-ASCII stylesheets.
*
*
* bool charset = 13;
* @return The charset.
*/
boolean getCharset();
/**
*
* Whether to silently suppresses all `LogEvent`s.
*
*
* bool silent = 14;
* @return The silent.
*/
boolean getSilent();
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @return A list containing the fatalDeprecation.
*/
java.util.List
getFatalDeprecationList();
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @return The count of fatalDeprecation.
*/
int getFatalDeprecationCount();
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param index The index of the element to return.
* @return The fatalDeprecation at the given index.
*/
java.lang.String getFatalDeprecation(int index);
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param index The index of the value to return.
* @return The bytes of the fatalDeprecation at the given index.
*/
com.google.protobuf.ByteString
getFatalDeprecationBytes(int index);
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @return A list containing the silenceDeprecation.
*/
java.util.List
getSilenceDeprecationList();
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @return The count of silenceDeprecation.
*/
int getSilenceDeprecationCount();
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param index The index of the element to return.
* @return The silenceDeprecation at the given index.
*/
java.lang.String getSilenceDeprecation(int index);
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param index The index of the value to return.
* @return The bytes of the silenceDeprecation at the given index.
*/
com.google.protobuf.ByteString
getSilenceDeprecationBytes(int index);
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @return A list containing the futureDeprecation.
*/
java.util.List
getFutureDeprecationList();
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @return The count of futureDeprecation.
*/
int getFutureDeprecationCount();
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param index The index of the element to return.
* @return The futureDeprecation at the given index.
*/
java.lang.String getFutureDeprecation(int index);
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param index The index of the value to return.
* @return The bytes of the futureDeprecation at the given index.
*/
com.google.protobuf.ByteString
getFutureDeprecationBytes(int index);
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.InputCase getInputCase();
}
/**
*
* A request that compiles an entrypoint to CSS.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CompileRequest}
*/
public static final class CompileRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.CompileRequest)
CompileRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
CompileRequest.class.getName());
}
// Use CompileRequest.newBuilder() to construct.
private CompileRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CompileRequest() {
style_ = 0;
importers_ = java.util.Collections.emptyList();
globalFunctions_ =
com.google.protobuf.LazyStringArrayList.emptyList();
fatalDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
silenceDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
futureDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.class, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Builder.class);
}
public interface StringInputOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.CompileRequest.StringInput)
com.google.protobuf.MessageOrBuilder {
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @return The source.
*/
java.lang.String getSource();
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @return The bytes for source.
*/
com.google.protobuf.ByteString
getSourceBytes();
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @return The url.
*/
java.lang.String getUrl();
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @return The bytes for url.
*/
com.google.protobuf.ByteString
getUrlBytes();
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @return The enum numeric value on the wire for syntax.
*/
int getSyntaxValue();
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @return The syntax.
*/
com.sass_lang.embedded_protocol.Syntax getSyntax();
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
* @return Whether the importer field is set.
*/
boolean hasImporter();
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
* @return The importer.
*/
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getImporter();
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder getImporterOrBuilder();
}
/**
*
* An input stylesheet provided as plain text, rather than loaded from the
* filesystem.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CompileRequest.StringInput}
*/
public static final class StringInput extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.CompileRequest.StringInput)
StringInputOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
StringInput.class.getName());
}
// Use StringInput.newBuilder() to construct.
private StringInput(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private StringInput() {
source_ = "";
url_ = "";
syntax_ = 0;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_StringInput_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_StringInput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.class, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.Builder.class);
}
private int bitField0_;
public static final int SOURCE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object source_ = "";
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @return The source.
*/
@java.lang.Override
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
}
}
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @return The bytes for source.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int URL_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object url_ = "";
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @return The url.
*/
@java.lang.Override
public java.lang.String getUrl() {
java.lang.Object ref = url_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
url_ = s;
return s;
}
}
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @return The bytes for url.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = url_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
url_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SYNTAX_FIELD_NUMBER = 3;
private int syntax_ = 0;
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @return The enum numeric value on the wire for syntax.
*/
@java.lang.Override public int getSyntaxValue() {
return syntax_;
}
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @return The syntax.
*/
@java.lang.Override public com.sass_lang.embedded_protocol.Syntax getSyntax() {
com.sass_lang.embedded_protocol.Syntax result = com.sass_lang.embedded_protocol.Syntax.forNumber(syntax_);
return result == null ? com.sass_lang.embedded_protocol.Syntax.UNRECOGNIZED : result;
}
public static final int IMPORTER_FIELD_NUMBER = 4;
private com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer importer_;
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
* @return Whether the importer field is set.
*/
@java.lang.Override
public boolean hasImporter() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
* @return The importer.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getImporter() {
return importer_ == null ? com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance() : importer_;
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder getImporterOrBuilder() {
return importer_ == null ? com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance() : importer_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(source_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, source_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(url_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, url_);
}
if (syntax_ != com.sass_lang.embedded_protocol.Syntax.SCSS.getNumber()) {
output.writeEnum(3, syntax_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(4, getImporter());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(source_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, source_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(url_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, url_);
}
if (syntax_ != com.sass_lang.embedded_protocol.Syntax.SCSS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, syntax_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getImporter());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput other = (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) obj;
if (!getSource()
.equals(other.getSource())) return false;
if (!getUrl()
.equals(other.getUrl())) return false;
if (syntax_ != other.syntax_) return false;
if (hasImporter() != other.hasImporter()) return false;
if (hasImporter()) {
if (!getImporter()
.equals(other.getImporter())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SOURCE_FIELD_NUMBER;
hash = (53 * hash) + getSource().hashCode();
hash = (37 * hash) + URL_FIELD_NUMBER;
hash = (53 * hash) + getUrl().hashCode();
hash = (37 * hash) + SYNTAX_FIELD_NUMBER;
hash = (53 * hash) + syntax_;
if (hasImporter()) {
hash = (37 * hash) + IMPORTER_FIELD_NUMBER;
hash = (53 * hash) + getImporter().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An input stylesheet provided as plain text, rather than loaded from the
* filesystem.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CompileRequest.StringInput}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.CompileRequest.StringInput)
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInputOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_StringInput_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_StringInput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.class, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getImporterFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
source_ = "";
url_ = "";
syntax_ = 0;
importer_ = null;
if (importerBuilder_ != null) {
importerBuilder_.dispose();
importerBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_StringInput_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput build() {
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput result = new com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.source_ = source_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.url_ = url_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.syntax_ = syntax_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.importer_ = importerBuilder_ == null
? importer_
: importerBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance()) return this;
if (!other.getSource().isEmpty()) {
source_ = other.source_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getUrl().isEmpty()) {
url_ = other.url_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.syntax_ != 0) {
setSyntaxValue(other.getSyntaxValue());
}
if (other.hasImporter()) {
mergeImporter(other.getImporter());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
source_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
url_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
syntax_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
input.readMessage(
getImporterFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object source_ = "";
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @return The source.
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @return The bytes for source.
*/
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @param value The source to set.
* @return This builder for chaining.
*/
public Builder setSource(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
source_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @return This builder for chaining.
*/
public Builder clearSource() {
source_ = getDefaultInstance().getSource();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The contents of the stylesheet.
*
*
* string source = 1;
* @param value The bytes for source to set.
* @return This builder for chaining.
*/
public Builder setSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
source_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object url_ = "";
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @return The url.
*/
public java.lang.String getUrl() {
java.lang.Object ref = url_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
url_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @return The bytes for url.
*/
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = url_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
url_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @param value The url to set.
* @return This builder for chaining.
*/
public Builder setUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
url_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @return This builder for chaining.
*/
public Builder clearUrl() {
url_ = getDefaultInstance().getUrl();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The location from which `source` was loaded. If this is empty, it
* indicates that the URL is unknown.
*
* This must be a canonical URL recognized by `importer`, if it's passed.
*
*
* string url = 2;
* @param value The bytes for url to set.
* @return This builder for chaining.
*/
public Builder setUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
url_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private int syntax_ = 0;
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @return The enum numeric value on the wire for syntax.
*/
@java.lang.Override public int getSyntaxValue() {
return syntax_;
}
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @param value The enum numeric value on the wire for syntax to set.
* @return This builder for chaining.
*/
public Builder setSyntaxValue(int value) {
syntax_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @return The syntax.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.Syntax getSyntax() {
com.sass_lang.embedded_protocol.Syntax result = com.sass_lang.embedded_protocol.Syntax.forNumber(syntax_);
return result == null ? com.sass_lang.embedded_protocol.Syntax.UNRECOGNIZED : result;
}
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @param value The syntax to set.
* @return This builder for chaining.
*/
public Builder setSyntax(com.sass_lang.embedded_protocol.Syntax value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
syntax_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The syntax to use to parse `source`.
*
*
* .sass.embedded_protocol.Syntax syntax = 3;
* @return This builder for chaining.
*/
public Builder clearSyntax() {
bitField0_ = (bitField0_ & ~0x00000004);
syntax_ = 0;
onChanged();
return this;
}
private com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer importer_;
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder> importerBuilder_;
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
* @return Whether the importer field is set.
*/
public boolean hasImporter() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
* @return The importer.
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getImporter() {
if (importerBuilder_ == null) {
return importer_ == null ? com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance() : importer_;
} else {
return importerBuilder_.getMessage();
}
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
public Builder setImporter(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer value) {
if (importerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
importer_ = value;
} else {
importerBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
public Builder setImporter(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder builderForValue) {
if (importerBuilder_ == null) {
importer_ = builderForValue.build();
} else {
importerBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
public Builder mergeImporter(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer value) {
if (importerBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
importer_ != null &&
importer_ != com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance()) {
getImporterBuilder().mergeFrom(value);
} else {
importer_ = value;
}
} else {
importerBuilder_.mergeFrom(value);
}
if (importer_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
public Builder clearImporter() {
bitField0_ = (bitField0_ & ~0x00000008);
importer_ = null;
if (importerBuilder_ != null) {
importerBuilder_.dispose();
importerBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder getImporterBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getImporterFieldBuilder().getBuilder();
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder getImporterOrBuilder() {
if (importerBuilder_ != null) {
return importerBuilder_.getMessageOrBuilder();
} else {
return importer_ == null ?
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance() : importer_;
}
}
/**
*
* The importer to use to resolve imports relative to `url`.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importer = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder>
getImporterFieldBuilder() {
if (importerBuilder_ == null) {
importerBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder>(
getImporter(),
getParentForChildren(),
isClean());
importer_ = null;
}
return importerBuilder_;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.CompileRequest.StringInput)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.CompileRequest.StringInput)
private static final com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput();
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StringInput parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ImporterOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.CompileRequest.Importer)
com.google.protobuf.MessageOrBuilder {
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return Whether the path field is set.
*/
boolean hasPath();
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return The path.
*/
java.lang.String getPath();
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @return Whether the importerId field is set.
*/
boolean hasImporterId();
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @return The importerId.
*/
int getImporterId();
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @return Whether the fileImporterId field is set.
*/
boolean hasFileImporterId();
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @return The fileImporterId.
*/
int getFileImporterId();
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
* @return Whether the nodePackageImporter field is set.
*/
boolean hasNodePackageImporter();
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
* @return The nodePackageImporter.
*/
com.sass_lang.embedded_protocol.NodePackageImporter getNodePackageImporter();
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
com.sass_lang.embedded_protocol.NodePackageImporterOrBuilder getNodePackageImporterOrBuilder();
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @return A list containing the nonCanonicalScheme.
*/
java.util.List
getNonCanonicalSchemeList();
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @return The count of nonCanonicalScheme.
*/
int getNonCanonicalSchemeCount();
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param index The index of the element to return.
* @return The nonCanonicalScheme at the given index.
*/
java.lang.String getNonCanonicalScheme(int index);
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param index The index of the value to return.
* @return The bytes of the nonCanonicalScheme at the given index.
*/
com.google.protobuf.ByteString
getNonCanonicalSchemeBytes(int index);
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.ImporterCase getImporterCase();
}
/**
*
* A wrapper message that represents either a user-defined importer or a
* load path on disk. This must be a wrapper because `oneof` types can't be
* `repeated`.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CompileRequest.Importer}
*/
public static final class Importer extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.CompileRequest.Importer)
ImporterOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Importer.class.getName());
}
// Use Importer.newBuilder() to construct.
private Importer(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Importer() {
nonCanonicalScheme_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_Importer_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_Importer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.class, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder.class);
}
private int importerCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object importer_;
public enum ImporterCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PATH(1),
IMPORTER_ID(2),
FILE_IMPORTER_ID(3),
NODE_PACKAGE_IMPORTER(5),
IMPORTER_NOT_SET(0);
private final int value;
private ImporterCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ImporterCase valueOf(int value) {
return forNumber(value);
}
public static ImporterCase forNumber(int value) {
switch (value) {
case 1: return PATH;
case 2: return IMPORTER_ID;
case 3: return FILE_IMPORTER_ID;
case 5: return NODE_PACKAGE_IMPORTER;
case 0: return IMPORTER_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ImporterCase
getImporterCase() {
return ImporterCase.forNumber(
importerCase_);
}
public static final int PATH_FIELD_NUMBER = 1;
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return Whether the path field is set.
*/
public boolean hasPath() {
return importerCase_ == 1;
}
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = "";
if (importerCase_ == 1) {
ref = importer_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (importerCase_ == 1) {
importer_ = s;
}
return s;
}
}
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = "";
if (importerCase_ == 1) {
ref = importer_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (importerCase_ == 1) {
importer_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IMPORTER_ID_FIELD_NUMBER = 2;
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @return Whether the importerId field is set.
*/
@java.lang.Override
public boolean hasImporterId() {
return importerCase_ == 2;
}
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @return The importerId.
*/
@java.lang.Override
public int getImporterId() {
if (importerCase_ == 2) {
return (java.lang.Integer) importer_;
}
return 0;
}
public static final int FILE_IMPORTER_ID_FIELD_NUMBER = 3;
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @return Whether the fileImporterId field is set.
*/
@java.lang.Override
public boolean hasFileImporterId() {
return importerCase_ == 3;
}
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @return The fileImporterId.
*/
@java.lang.Override
public int getFileImporterId() {
if (importerCase_ == 3) {
return (java.lang.Integer) importer_;
}
return 0;
}
public static final int NODE_PACKAGE_IMPORTER_FIELD_NUMBER = 5;
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
* @return Whether the nodePackageImporter field is set.
*/
@java.lang.Override
public boolean hasNodePackageImporter() {
return importerCase_ == 5;
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
* @return The nodePackageImporter.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.NodePackageImporter getNodePackageImporter() {
if (importerCase_ == 5) {
return (com.sass_lang.embedded_protocol.NodePackageImporter) importer_;
}
return com.sass_lang.embedded_protocol.NodePackageImporter.getDefaultInstance();
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.NodePackageImporterOrBuilder getNodePackageImporterOrBuilder() {
if (importerCase_ == 5) {
return (com.sass_lang.embedded_protocol.NodePackageImporter) importer_;
}
return com.sass_lang.embedded_protocol.NodePackageImporter.getDefaultInstance();
}
public static final int NON_CANONICAL_SCHEME_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList nonCanonicalScheme_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @return A list containing the nonCanonicalScheme.
*/
public com.google.protobuf.ProtocolStringList
getNonCanonicalSchemeList() {
return nonCanonicalScheme_;
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @return The count of nonCanonicalScheme.
*/
public int getNonCanonicalSchemeCount() {
return nonCanonicalScheme_.size();
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param index The index of the element to return.
* @return The nonCanonicalScheme at the given index.
*/
public java.lang.String getNonCanonicalScheme(int index) {
return nonCanonicalScheme_.get(index);
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param index The index of the value to return.
* @return The bytes of the nonCanonicalScheme at the given index.
*/
public com.google.protobuf.ByteString
getNonCanonicalSchemeBytes(int index) {
return nonCanonicalScheme_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (importerCase_ == 1) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, importer_);
}
if (importerCase_ == 2) {
output.writeUInt32(
2, (int)((java.lang.Integer) importer_));
}
if (importerCase_ == 3) {
output.writeUInt32(
3, (int)((java.lang.Integer) importer_));
}
for (int i = 0; i < nonCanonicalScheme_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, nonCanonicalScheme_.getRaw(i));
}
if (importerCase_ == 5) {
output.writeMessage(5, (com.sass_lang.embedded_protocol.NodePackageImporter) importer_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (importerCase_ == 1) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, importer_);
}
if (importerCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(
2, (int)((java.lang.Integer) importer_));
}
if (importerCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(
3, (int)((java.lang.Integer) importer_));
}
{
int dataSize = 0;
for (int i = 0; i < nonCanonicalScheme_.size(); i++) {
dataSize += computeStringSizeNoTag(nonCanonicalScheme_.getRaw(i));
}
size += dataSize;
size += 1 * getNonCanonicalSchemeList().size();
}
if (importerCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (com.sass_lang.embedded_protocol.NodePackageImporter) importer_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer other = (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer) obj;
if (!getNonCanonicalSchemeList()
.equals(other.getNonCanonicalSchemeList())) return false;
if (!getImporterCase().equals(other.getImporterCase())) return false;
switch (importerCase_) {
case 1:
if (!getPath()
.equals(other.getPath())) return false;
break;
case 2:
if (getImporterId()
!= other.getImporterId()) return false;
break;
case 3:
if (getFileImporterId()
!= other.getFileImporterId()) return false;
break;
case 5:
if (!getNodePackageImporter()
.equals(other.getNodePackageImporter())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNonCanonicalSchemeCount() > 0) {
hash = (37 * hash) + NON_CANONICAL_SCHEME_FIELD_NUMBER;
hash = (53 * hash) + getNonCanonicalSchemeList().hashCode();
}
switch (importerCase_) {
case 1:
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
break;
case 2:
hash = (37 * hash) + IMPORTER_ID_FIELD_NUMBER;
hash = (53 * hash) + getImporterId();
break;
case 3:
hash = (37 * hash) + FILE_IMPORTER_ID_FIELD_NUMBER;
hash = (53 * hash) + getFileImporterId();
break;
case 5:
hash = (37 * hash) + NODE_PACKAGE_IMPORTER_FIELD_NUMBER;
hash = (53 * hash) + getNodePackageImporter().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A wrapper message that represents either a user-defined importer or a
* load path on disk. This must be a wrapper because `oneof` types can't be
* `repeated`.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CompileRequest.Importer}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.CompileRequest.Importer)
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_Importer_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_Importer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.class, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (nodePackageImporterBuilder_ != null) {
nodePackageImporterBuilder_.clear();
}
nonCanonicalScheme_ =
com.google.protobuf.LazyStringArrayList.emptyList();
importerCase_ = 0;
importer_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_Importer_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer build() {
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer result = new com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000010) != 0)) {
nonCanonicalScheme_.makeImmutable();
result.nonCanonicalScheme_ = nonCanonicalScheme_;
}
}
private void buildPartialOneofs(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer result) {
result.importerCase_ = importerCase_;
result.importer_ = this.importer_;
if (importerCase_ == 5 &&
nodePackageImporterBuilder_ != null) {
result.importer_ = nodePackageImporterBuilder_.build();
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance()) return this;
if (!other.nonCanonicalScheme_.isEmpty()) {
if (nonCanonicalScheme_.isEmpty()) {
nonCanonicalScheme_ = other.nonCanonicalScheme_;
bitField0_ |= 0x00000010;
} else {
ensureNonCanonicalSchemeIsMutable();
nonCanonicalScheme_.addAll(other.nonCanonicalScheme_);
}
onChanged();
}
switch (other.getImporterCase()) {
case PATH: {
importerCase_ = 1;
importer_ = other.importer_;
onChanged();
break;
}
case IMPORTER_ID: {
setImporterId(other.getImporterId());
break;
}
case FILE_IMPORTER_ID: {
setFileImporterId(other.getFileImporterId());
break;
}
case NODE_PACKAGE_IMPORTER: {
mergeNodePackageImporter(other.getNodePackageImporter());
break;
}
case IMPORTER_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
importerCase_ = 1;
importer_ = s;
break;
} // case 10
case 16: {
importer_ = input.readUInt32();
importerCase_ = 2;
break;
} // case 16
case 24: {
importer_ = input.readUInt32();
importerCase_ = 3;
break;
} // case 24
case 34: {
java.lang.String s = input.readStringRequireUtf8();
ensureNonCanonicalSchemeIsMutable();
nonCanonicalScheme_.add(s);
break;
} // case 34
case 42: {
input.readMessage(
getNodePackageImporterFieldBuilder().getBuilder(),
extensionRegistry);
importerCase_ = 5;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int importerCase_ = 0;
private java.lang.Object importer_;
public ImporterCase
getImporterCase() {
return ImporterCase.forNumber(
importerCase_);
}
public Builder clearImporter() {
importerCase_ = 0;
importer_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return Whether the path field is set.
*/
@java.lang.Override
public boolean hasPath() {
return importerCase_ == 1;
}
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = "";
if (importerCase_ == 1) {
ref = importer_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (importerCase_ == 1) {
importer_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = "";
if (importerCase_ == 1) {
ref = importer_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (importerCase_ == 1) {
importer_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
importerCase_ = 1;
importer_ = value;
onChanged();
return this;
}
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @return This builder for chaining.
*/
public Builder clearPath() {
if (importerCase_ == 1) {
importerCase_ = 0;
importer_ = null;
onChanged();
}
return this;
}
/**
*
* A built-in importer that loads Sass files within the given directory
* on disk.
*
*
* string path = 1;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
importerCase_ = 1;
importer_ = value;
onChanged();
return this;
}
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @return Whether the importerId field is set.
*/
public boolean hasImporterId() {
return importerCase_ == 2;
}
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @return The importerId.
*/
public int getImporterId() {
if (importerCase_ == 2) {
return (java.lang.Integer) importer_;
}
return 0;
}
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @param value The importerId to set.
* @return This builder for chaining.
*/
public Builder setImporterId(int value) {
importerCase_ = 2;
importer_ = value;
onChanged();
return this;
}
/**
*
* A unique ID for a user-defined importer. This ID will be included in
* outbound `CanonicalizeRequest` and `ImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all
* importers registered for this compilation.
*
*
* uint32 importer_id = 2;
* @return This builder for chaining.
*/
public Builder clearImporterId() {
if (importerCase_ == 2) {
importerCase_ = 0;
importer_ = null;
onChanged();
}
return this;
}
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @return Whether the fileImporterId field is set.
*/
public boolean hasFileImporterId() {
return importerCase_ == 3;
}
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @return The fileImporterId.
*/
public int getFileImporterId() {
if (importerCase_ == 3) {
return (java.lang.Integer) importer_;
}
return 0;
}
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @param value The fileImporterId to set.
* @return This builder for chaining.
*/
public Builder setFileImporterId(int value) {
importerCase_ = 3;
importer_ = value;
onChanged();
return this;
}
/**
*
* A unique ID for a special kind of user-defined importer that tells
* the compiler where to look for files on the physical filesystem, but
* leaves the details of resolving partials and extensions and loading
* the file from disk up to the compiler itself.
*
* This ID will be included in outbound `FileImportRequest` messages to
* indicate which importer is being called. The host is responsible for
* generating this ID and ensuring that it's unique across all importers
* registered for this compilation.
*
*
* uint32 file_importer_id = 3;
* @return This builder for chaining.
*/
public Builder clearFileImporterId() {
if (importerCase_ == 3) {
importerCase_ = 0;
importer_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.NodePackageImporter, com.sass_lang.embedded_protocol.NodePackageImporter.Builder, com.sass_lang.embedded_protocol.NodePackageImporterOrBuilder> nodePackageImporterBuilder_;
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
* @return Whether the nodePackageImporter field is set.
*/
@java.lang.Override
public boolean hasNodePackageImporter() {
return importerCase_ == 5;
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
* @return The nodePackageImporter.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.NodePackageImporter getNodePackageImporter() {
if (nodePackageImporterBuilder_ == null) {
if (importerCase_ == 5) {
return (com.sass_lang.embedded_protocol.NodePackageImporter) importer_;
}
return com.sass_lang.embedded_protocol.NodePackageImporter.getDefaultInstance();
} else {
if (importerCase_ == 5) {
return nodePackageImporterBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.NodePackageImporter.getDefaultInstance();
}
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
public Builder setNodePackageImporter(com.sass_lang.embedded_protocol.NodePackageImporter value) {
if (nodePackageImporterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
importer_ = value;
onChanged();
} else {
nodePackageImporterBuilder_.setMessage(value);
}
importerCase_ = 5;
return this;
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
public Builder setNodePackageImporter(
com.sass_lang.embedded_protocol.NodePackageImporter.Builder builderForValue) {
if (nodePackageImporterBuilder_ == null) {
importer_ = builderForValue.build();
onChanged();
} else {
nodePackageImporterBuilder_.setMessage(builderForValue.build());
}
importerCase_ = 5;
return this;
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
public Builder mergeNodePackageImporter(com.sass_lang.embedded_protocol.NodePackageImporter value) {
if (nodePackageImporterBuilder_ == null) {
if (importerCase_ == 5 &&
importer_ != com.sass_lang.embedded_protocol.NodePackageImporter.getDefaultInstance()) {
importer_ = com.sass_lang.embedded_protocol.NodePackageImporter.newBuilder((com.sass_lang.embedded_protocol.NodePackageImporter) importer_)
.mergeFrom(value).buildPartial();
} else {
importer_ = value;
}
onChanged();
} else {
if (importerCase_ == 5) {
nodePackageImporterBuilder_.mergeFrom(value);
} else {
nodePackageImporterBuilder_.setMessage(value);
}
}
importerCase_ = 5;
return this;
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
public Builder clearNodePackageImporter() {
if (nodePackageImporterBuilder_ == null) {
if (importerCase_ == 5) {
importerCase_ = 0;
importer_ = null;
onChanged();
}
} else {
if (importerCase_ == 5) {
importerCase_ = 0;
importer_ = null;
}
nodePackageImporterBuilder_.clear();
}
return this;
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
public com.sass_lang.embedded_protocol.NodePackageImporter.Builder getNodePackageImporterBuilder() {
return getNodePackageImporterFieldBuilder().getBuilder();
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.NodePackageImporterOrBuilder getNodePackageImporterOrBuilder() {
if ((importerCase_ == 5) && (nodePackageImporterBuilder_ != null)) {
return nodePackageImporterBuilder_.getMessageOrBuilder();
} else {
if (importerCase_ == 5) {
return (com.sass_lang.embedded_protocol.NodePackageImporter) importer_;
}
return com.sass_lang.embedded_protocol.NodePackageImporter.getDefaultInstance();
}
}
/**
*
* The [Node.js package importer], which is a built-in Package Importer
* with an associated `entry_point_directory` that resolves `pkg:` URLs
* using the standards and conventions of the Node ecosystem.
*
* [Node.js package importer]: https://github.com/sass/sass/tree/main/spec/modules.md#node-package-importer
*
*
* .sass.embedded_protocol.NodePackageImporter node_package_importer = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.NodePackageImporter, com.sass_lang.embedded_protocol.NodePackageImporter.Builder, com.sass_lang.embedded_protocol.NodePackageImporterOrBuilder>
getNodePackageImporterFieldBuilder() {
if (nodePackageImporterBuilder_ == null) {
if (!(importerCase_ == 5)) {
importer_ = com.sass_lang.embedded_protocol.NodePackageImporter.getDefaultInstance();
}
nodePackageImporterBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.NodePackageImporter, com.sass_lang.embedded_protocol.NodePackageImporter.Builder, com.sass_lang.embedded_protocol.NodePackageImporterOrBuilder>(
(com.sass_lang.embedded_protocol.NodePackageImporter) importer_,
getParentForChildren(),
isClean());
importer_ = null;
}
importerCase_ = 5;
onChanged();
return nodePackageImporterBuilder_;
}
private com.google.protobuf.LazyStringArrayList nonCanonicalScheme_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureNonCanonicalSchemeIsMutable() {
if (!nonCanonicalScheme_.isModifiable()) {
nonCanonicalScheme_ = new com.google.protobuf.LazyStringArrayList(nonCanonicalScheme_);
}
bitField0_ |= 0x00000010;
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @return A list containing the nonCanonicalScheme.
*/
public com.google.protobuf.ProtocolStringList
getNonCanonicalSchemeList() {
nonCanonicalScheme_.makeImmutable();
return nonCanonicalScheme_;
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @return The count of nonCanonicalScheme.
*/
public int getNonCanonicalSchemeCount() {
return nonCanonicalScheme_.size();
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param index The index of the element to return.
* @return The nonCanonicalScheme at the given index.
*/
public java.lang.String getNonCanonicalScheme(int index) {
return nonCanonicalScheme_.get(index);
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param index The index of the value to return.
* @return The bytes of the nonCanonicalScheme at the given index.
*/
public com.google.protobuf.ByteString
getNonCanonicalSchemeBytes(int index) {
return nonCanonicalScheme_.getByteString(index);
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param index The index to set the value at.
* @param value The nonCanonicalScheme to set.
* @return This builder for chaining.
*/
public Builder setNonCanonicalScheme(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureNonCanonicalSchemeIsMutable();
nonCanonicalScheme_.set(index, value);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param value The nonCanonicalScheme to add.
* @return This builder for chaining.
*/
public Builder addNonCanonicalScheme(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureNonCanonicalSchemeIsMutable();
nonCanonicalScheme_.add(value);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param values The nonCanonicalScheme to add.
* @return This builder for chaining.
*/
public Builder addAllNonCanonicalScheme(
java.lang.Iterable values) {
ensureNonCanonicalSchemeIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nonCanonicalScheme_);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @return This builder for chaining.
*/
public Builder clearNonCanonicalScheme() {
nonCanonicalScheme_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);;
onChanged();
return this;
}
/**
*
* The set of URL schemes that are considered *non-canonical* for this
* importer. This must be empty unless `importer.importer_id` is set.
*
* If any element of this contains a character other than a lowercase
* ASCII letter, an ASCII numeral, U+002B (`+`), U+002D (`-`), or U+002E
* (`.`), the compiler must treat the compilation as failed.
*
*
* repeated string non_canonical_scheme = 4;
* @param value The bytes of the nonCanonicalScheme to add.
* @return This builder for chaining.
*/
public Builder addNonCanonicalSchemeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureNonCanonicalSchemeIsMutable();
nonCanonicalScheme_.add(value);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.CompileRequest.Importer)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.CompileRequest.Importer)
private static final com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer();
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Importer parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int inputCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object input_;
public enum InputCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
STRING(2),
PATH(3),
INPUT_NOT_SET(0);
private final int value;
private InputCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static InputCase valueOf(int value) {
return forNumber(value);
}
public static InputCase forNumber(int value) {
switch (value) {
case 2: return STRING;
case 3: return PATH;
case 0: return INPUT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public InputCase
getInputCase() {
return InputCase.forNumber(
inputCase_);
}
public static final int STRING_FIELD_NUMBER = 2;
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
* @return Whether the string field is set.
*/
@java.lang.Override
public boolean hasString() {
return inputCase_ == 2;
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
* @return The string.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput getString() {
if (inputCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance();
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInputOrBuilder getStringOrBuilder() {
if (inputCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance();
}
public static final int PATH_FIELD_NUMBER = 3;
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return Whether the path field is set.
*/
public boolean hasPath() {
return inputCase_ == 3;
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = "";
if (inputCase_ == 3) {
ref = input_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (inputCase_ == 3) {
input_ = s;
}
return s;
}
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = "";
if (inputCase_ == 3) {
ref = input_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (inputCase_ == 3) {
input_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STYLE_FIELD_NUMBER = 4;
private int style_ = 0;
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @return The enum numeric value on the wire for style.
*/
@java.lang.Override public int getStyleValue() {
return style_;
}
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @return The style.
*/
@java.lang.Override public com.sass_lang.embedded_protocol.OutputStyle getStyle() {
com.sass_lang.embedded_protocol.OutputStyle result = com.sass_lang.embedded_protocol.OutputStyle.forNumber(style_);
return result == null ? com.sass_lang.embedded_protocol.OutputStyle.UNRECOGNIZED : result;
}
public static final int SOURCE_MAP_FIELD_NUMBER = 5;
private boolean sourceMap_ = false;
/**
*
* Whether to generate a source map. Note that this will *not* add a source
* map comment to the stylesheet; that's up to the host or its users.
*
*
* bool source_map = 5;
* @return The sourceMap.
*/
@java.lang.Override
public boolean getSourceMap() {
return sourceMap_;
}
public static final int IMPORTERS_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List importers_;
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
@java.lang.Override
public java.util.List getImportersList() {
return importers_;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
@java.lang.Override
public java.util.List extends com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder>
getImportersOrBuilderList() {
return importers_;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
@java.lang.Override
public int getImportersCount() {
return importers_.size();
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getImporters(int index) {
return importers_.get(index);
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder getImportersOrBuilder(
int index) {
return importers_.get(index);
}
public static final int GLOBAL_FUNCTIONS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList globalFunctions_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @return A list containing the globalFunctions.
*/
public com.google.protobuf.ProtocolStringList
getGlobalFunctionsList() {
return globalFunctions_;
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @return The count of globalFunctions.
*/
public int getGlobalFunctionsCount() {
return globalFunctions_.size();
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param index The index of the element to return.
* @return The globalFunctions at the given index.
*/
public java.lang.String getGlobalFunctions(int index) {
return globalFunctions_.get(index);
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param index The index of the value to return.
* @return The bytes of the globalFunctions at the given index.
*/
public com.google.protobuf.ByteString
getGlobalFunctionsBytes(int index) {
return globalFunctions_.getByteString(index);
}
public static final int ALERT_COLOR_FIELD_NUMBER = 8;
private boolean alertColor_ = false;
/**
*
* Whether to use terminal colors in the formatted message of errors and
* logs.
*
*
* bool alert_color = 8;
* @return The alertColor.
*/
@java.lang.Override
public boolean getAlertColor() {
return alertColor_;
}
public static final int ALERT_ASCII_FIELD_NUMBER = 9;
private boolean alertAscii_ = false;
/**
*
* Whether to encode the formatted message of errors and logs in ASCII.
*
*
* bool alert_ascii = 9;
* @return The alertAscii.
*/
@java.lang.Override
public boolean getAlertAscii() {
return alertAscii_;
}
public static final int VERBOSE_FIELD_NUMBER = 10;
private boolean verbose_ = false;
/**
*
* Whether to report all deprecation warnings or only the first few ones.
* If this is `false`, the compiler may choose not to send events for
* repeated deprecation warnings. If this is `true`, the compiler must emit
* an event for every deprecation warning it encounters.
*
*
* bool verbose = 10;
* @return The verbose.
*/
@java.lang.Override
public boolean getVerbose() {
return verbose_;
}
public static final int QUIET_DEPS_FIELD_NUMBER = 11;
private boolean quietDeps_ = false;
/**
*
* Whether to omit events for deprecation warnings coming from dependencies
* (files loaded from a different importer than the input).
*
*
* bool quiet_deps = 11;
* @return The quietDeps.
*/
@java.lang.Override
public boolean getQuietDeps() {
return quietDeps_;
}
public static final int SOURCE_MAP_INCLUDE_SOURCES_FIELD_NUMBER = 12;
private boolean sourceMapIncludeSources_ = false;
/**
*
* Whether to include sources in the generated sourcemap
*
*
* bool source_map_include_sources = 12;
* @return The sourceMapIncludeSources.
*/
@java.lang.Override
public boolean getSourceMapIncludeSources() {
return sourceMapIncludeSources_;
}
public static final int CHARSET_FIELD_NUMBER = 13;
private boolean charset_ = false;
/**
*
* Whether to emit a `@charset`/BOM for non-ASCII stylesheets.
*
*
* bool charset = 13;
* @return The charset.
*/
@java.lang.Override
public boolean getCharset() {
return charset_;
}
public static final int SILENT_FIELD_NUMBER = 14;
private boolean silent_ = false;
/**
*
* Whether to silently suppresses all `LogEvent`s.
*
*
* bool silent = 14;
* @return The silent.
*/
@java.lang.Override
public boolean getSilent() {
return silent_;
}
public static final int FATAL_DEPRECATION_FIELD_NUMBER = 15;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList fatalDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @return A list containing the fatalDeprecation.
*/
public com.google.protobuf.ProtocolStringList
getFatalDeprecationList() {
return fatalDeprecation_;
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @return The count of fatalDeprecation.
*/
public int getFatalDeprecationCount() {
return fatalDeprecation_.size();
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param index The index of the element to return.
* @return The fatalDeprecation at the given index.
*/
public java.lang.String getFatalDeprecation(int index) {
return fatalDeprecation_.get(index);
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param index The index of the value to return.
* @return The bytes of the fatalDeprecation at the given index.
*/
public com.google.protobuf.ByteString
getFatalDeprecationBytes(int index) {
return fatalDeprecation_.getByteString(index);
}
public static final int SILENCE_DEPRECATION_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList silenceDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @return A list containing the silenceDeprecation.
*/
public com.google.protobuf.ProtocolStringList
getSilenceDeprecationList() {
return silenceDeprecation_;
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @return The count of silenceDeprecation.
*/
public int getSilenceDeprecationCount() {
return silenceDeprecation_.size();
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param index The index of the element to return.
* @return The silenceDeprecation at the given index.
*/
public java.lang.String getSilenceDeprecation(int index) {
return silenceDeprecation_.get(index);
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param index The index of the value to return.
* @return The bytes of the silenceDeprecation at the given index.
*/
public com.google.protobuf.ByteString
getSilenceDeprecationBytes(int index) {
return silenceDeprecation_.getByteString(index);
}
public static final int FUTURE_DEPRECATION_FIELD_NUMBER = 17;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList futureDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @return A list containing the futureDeprecation.
*/
public com.google.protobuf.ProtocolStringList
getFutureDeprecationList() {
return futureDeprecation_;
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @return The count of futureDeprecation.
*/
public int getFutureDeprecationCount() {
return futureDeprecation_.size();
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param index The index of the element to return.
* @return The futureDeprecation at the given index.
*/
public java.lang.String getFutureDeprecation(int index) {
return futureDeprecation_.get(index);
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param index The index of the value to return.
* @return The bytes of the futureDeprecation at the given index.
*/
public com.google.protobuf.ByteString
getFutureDeprecationBytes(int index) {
return futureDeprecation_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (inputCase_ == 2) {
output.writeMessage(2, (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_);
}
if (inputCase_ == 3) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, input_);
}
if (style_ != com.sass_lang.embedded_protocol.OutputStyle.EXPANDED.getNumber()) {
output.writeEnum(4, style_);
}
if (sourceMap_ != false) {
output.writeBool(5, sourceMap_);
}
for (int i = 0; i < importers_.size(); i++) {
output.writeMessage(6, importers_.get(i));
}
for (int i = 0; i < globalFunctions_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 7, globalFunctions_.getRaw(i));
}
if (alertColor_ != false) {
output.writeBool(8, alertColor_);
}
if (alertAscii_ != false) {
output.writeBool(9, alertAscii_);
}
if (verbose_ != false) {
output.writeBool(10, verbose_);
}
if (quietDeps_ != false) {
output.writeBool(11, quietDeps_);
}
if (sourceMapIncludeSources_ != false) {
output.writeBool(12, sourceMapIncludeSources_);
}
if (charset_ != false) {
output.writeBool(13, charset_);
}
if (silent_ != false) {
output.writeBool(14, silent_);
}
for (int i = 0; i < fatalDeprecation_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 15, fatalDeprecation_.getRaw(i));
}
for (int i = 0; i < silenceDeprecation_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 16, silenceDeprecation_.getRaw(i));
}
for (int i = 0; i < futureDeprecation_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 17, futureDeprecation_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (inputCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_);
}
if (inputCase_ == 3) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, input_);
}
if (style_ != com.sass_lang.embedded_protocol.OutputStyle.EXPANDED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, style_);
}
if (sourceMap_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, sourceMap_);
}
for (int i = 0; i < importers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, importers_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < globalFunctions_.size(); i++) {
dataSize += computeStringSizeNoTag(globalFunctions_.getRaw(i));
}
size += dataSize;
size += 1 * getGlobalFunctionsList().size();
}
if (alertColor_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, alertColor_);
}
if (alertAscii_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, alertAscii_);
}
if (verbose_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, verbose_);
}
if (quietDeps_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, quietDeps_);
}
if (sourceMapIncludeSources_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, sourceMapIncludeSources_);
}
if (charset_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, charset_);
}
if (silent_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(14, silent_);
}
{
int dataSize = 0;
for (int i = 0; i < fatalDeprecation_.size(); i++) {
dataSize += computeStringSizeNoTag(fatalDeprecation_.getRaw(i));
}
size += dataSize;
size += 1 * getFatalDeprecationList().size();
}
{
int dataSize = 0;
for (int i = 0; i < silenceDeprecation_.size(); i++) {
dataSize += computeStringSizeNoTag(silenceDeprecation_.getRaw(i));
}
size += dataSize;
size += 2 * getSilenceDeprecationList().size();
}
{
int dataSize = 0;
for (int i = 0; i < futureDeprecation_.size(); i++) {
dataSize += computeStringSizeNoTag(futureDeprecation_.getRaw(i));
}
size += dataSize;
size += 2 * getFutureDeprecationList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.CompileRequest)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest other = (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) obj;
if (style_ != other.style_) return false;
if (getSourceMap()
!= other.getSourceMap()) return false;
if (!getImportersList()
.equals(other.getImportersList())) return false;
if (!getGlobalFunctionsList()
.equals(other.getGlobalFunctionsList())) return false;
if (getAlertColor()
!= other.getAlertColor()) return false;
if (getAlertAscii()
!= other.getAlertAscii()) return false;
if (getVerbose()
!= other.getVerbose()) return false;
if (getQuietDeps()
!= other.getQuietDeps()) return false;
if (getSourceMapIncludeSources()
!= other.getSourceMapIncludeSources()) return false;
if (getCharset()
!= other.getCharset()) return false;
if (getSilent()
!= other.getSilent()) return false;
if (!getFatalDeprecationList()
.equals(other.getFatalDeprecationList())) return false;
if (!getSilenceDeprecationList()
.equals(other.getSilenceDeprecationList())) return false;
if (!getFutureDeprecationList()
.equals(other.getFutureDeprecationList())) return false;
if (!getInputCase().equals(other.getInputCase())) return false;
switch (inputCase_) {
case 2:
if (!getString()
.equals(other.getString())) return false;
break;
case 3:
if (!getPath()
.equals(other.getPath())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STYLE_FIELD_NUMBER;
hash = (53 * hash) + style_;
hash = (37 * hash) + SOURCE_MAP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSourceMap());
if (getImportersCount() > 0) {
hash = (37 * hash) + IMPORTERS_FIELD_NUMBER;
hash = (53 * hash) + getImportersList().hashCode();
}
if (getGlobalFunctionsCount() > 0) {
hash = (37 * hash) + GLOBAL_FUNCTIONS_FIELD_NUMBER;
hash = (53 * hash) + getGlobalFunctionsList().hashCode();
}
hash = (37 * hash) + ALERT_COLOR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAlertColor());
hash = (37 * hash) + ALERT_ASCII_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAlertAscii());
hash = (37 * hash) + VERBOSE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getVerbose());
hash = (37 * hash) + QUIET_DEPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getQuietDeps());
hash = (37 * hash) + SOURCE_MAP_INCLUDE_SOURCES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSourceMapIncludeSources());
hash = (37 * hash) + CHARSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCharset());
hash = (37 * hash) + SILENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSilent());
if (getFatalDeprecationCount() > 0) {
hash = (37 * hash) + FATAL_DEPRECATION_FIELD_NUMBER;
hash = (53 * hash) + getFatalDeprecationList().hashCode();
}
if (getSilenceDeprecationCount() > 0) {
hash = (37 * hash) + SILENCE_DEPRECATION_FIELD_NUMBER;
hash = (53 * hash) + getSilenceDeprecationList().hashCode();
}
if (getFutureDeprecationCount() > 0) {
hash = (37 * hash) + FUTURE_DEPRECATION_FIELD_NUMBER;
hash = (53 * hash) + getFutureDeprecationList().hashCode();
}
switch (inputCase_) {
case 2:
hash = (37 * hash) + STRING_FIELD_NUMBER;
hash = (53 * hash) + getString().hashCode();
break;
case 3:
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A request that compiles an entrypoint to CSS.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CompileRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.CompileRequest)
com.sass_lang.embedded_protocol.InboundMessage.CompileRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.class, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (stringBuilder_ != null) {
stringBuilder_.clear();
}
style_ = 0;
sourceMap_ = false;
if (importersBuilder_ == null) {
importers_ = java.util.Collections.emptyList();
} else {
importers_ = null;
importersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
globalFunctions_ =
com.google.protobuf.LazyStringArrayList.emptyList();
alertColor_ = false;
alertAscii_ = false;
verbose_ = false;
quietDeps_ = false;
sourceMapIncludeSources_ = false;
charset_ = false;
silent_ = false;
fatalDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
silenceDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
futureDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
inputCase_ = 0;
input_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CompileRequest_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest build() {
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest result = new com.sass_lang.embedded_protocol.InboundMessage.CompileRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest result) {
if (importersBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
importers_ = java.util.Collections.unmodifiableList(importers_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.importers_ = importers_;
} else {
result.importers_ = importersBuilder_.build();
}
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.style_ = style_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.sourceMap_ = sourceMap_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
globalFunctions_.makeImmutable();
result.globalFunctions_ = globalFunctions_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.alertColor_ = alertColor_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.alertAscii_ = alertAscii_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.verbose_ = verbose_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.quietDeps_ = quietDeps_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.sourceMapIncludeSources_ = sourceMapIncludeSources_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.charset_ = charset_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.silent_ = silent_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
fatalDeprecation_.makeImmutable();
result.fatalDeprecation_ = fatalDeprecation_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
silenceDeprecation_.makeImmutable();
result.silenceDeprecation_ = silenceDeprecation_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
futureDeprecation_.makeImmutable();
result.futureDeprecation_ = futureDeprecation_;
}
}
private void buildPartialOneofs(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest result) {
result.inputCase_ = inputCase_;
result.input_ = this.input_;
if (inputCase_ == 2 &&
stringBuilder_ != null) {
result.input_ = stringBuilder_.build();
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.CompileRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance()) return this;
if (other.style_ != 0) {
setStyleValue(other.getStyleValue());
}
if (other.getSourceMap() != false) {
setSourceMap(other.getSourceMap());
}
if (importersBuilder_ == null) {
if (!other.importers_.isEmpty()) {
if (importers_.isEmpty()) {
importers_ = other.importers_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureImportersIsMutable();
importers_.addAll(other.importers_);
}
onChanged();
}
} else {
if (!other.importers_.isEmpty()) {
if (importersBuilder_.isEmpty()) {
importersBuilder_.dispose();
importersBuilder_ = null;
importers_ = other.importers_;
bitField0_ = (bitField0_ & ~0x00000010);
importersBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getImportersFieldBuilder() : null;
} else {
importersBuilder_.addAllMessages(other.importers_);
}
}
}
if (!other.globalFunctions_.isEmpty()) {
if (globalFunctions_.isEmpty()) {
globalFunctions_ = other.globalFunctions_;
bitField0_ |= 0x00000020;
} else {
ensureGlobalFunctionsIsMutable();
globalFunctions_.addAll(other.globalFunctions_);
}
onChanged();
}
if (other.getAlertColor() != false) {
setAlertColor(other.getAlertColor());
}
if (other.getAlertAscii() != false) {
setAlertAscii(other.getAlertAscii());
}
if (other.getVerbose() != false) {
setVerbose(other.getVerbose());
}
if (other.getQuietDeps() != false) {
setQuietDeps(other.getQuietDeps());
}
if (other.getSourceMapIncludeSources() != false) {
setSourceMapIncludeSources(other.getSourceMapIncludeSources());
}
if (other.getCharset() != false) {
setCharset(other.getCharset());
}
if (other.getSilent() != false) {
setSilent(other.getSilent());
}
if (!other.fatalDeprecation_.isEmpty()) {
if (fatalDeprecation_.isEmpty()) {
fatalDeprecation_ = other.fatalDeprecation_;
bitField0_ |= 0x00002000;
} else {
ensureFatalDeprecationIsMutable();
fatalDeprecation_.addAll(other.fatalDeprecation_);
}
onChanged();
}
if (!other.silenceDeprecation_.isEmpty()) {
if (silenceDeprecation_.isEmpty()) {
silenceDeprecation_ = other.silenceDeprecation_;
bitField0_ |= 0x00004000;
} else {
ensureSilenceDeprecationIsMutable();
silenceDeprecation_.addAll(other.silenceDeprecation_);
}
onChanged();
}
if (!other.futureDeprecation_.isEmpty()) {
if (futureDeprecation_.isEmpty()) {
futureDeprecation_ = other.futureDeprecation_;
bitField0_ |= 0x00008000;
} else {
ensureFutureDeprecationIsMutable();
futureDeprecation_.addAll(other.futureDeprecation_);
}
onChanged();
}
switch (other.getInputCase()) {
case STRING: {
mergeString(other.getString());
break;
}
case PATH: {
inputCase_ = 3;
input_ = other.input_;
onChanged();
break;
}
case INPUT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
input.readMessage(
getStringFieldBuilder().getBuilder(),
extensionRegistry);
inputCase_ = 2;
break;
} // case 18
case 26: {
java.lang.String s = input.readStringRequireUtf8();
inputCase_ = 3;
input_ = s;
break;
} // case 26
case 32: {
style_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 32
case 40: {
sourceMap_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 40
case 50: {
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer m =
input.readMessage(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.parser(),
extensionRegistry);
if (importersBuilder_ == null) {
ensureImportersIsMutable();
importers_.add(m);
} else {
importersBuilder_.addMessage(m);
}
break;
} // case 50
case 58: {
java.lang.String s = input.readStringRequireUtf8();
ensureGlobalFunctionsIsMutable();
globalFunctions_.add(s);
break;
} // case 58
case 64: {
alertColor_ = input.readBool();
bitField0_ |= 0x00000040;
break;
} // case 64
case 72: {
alertAscii_ = input.readBool();
bitField0_ |= 0x00000080;
break;
} // case 72
case 80: {
verbose_ = input.readBool();
bitField0_ |= 0x00000100;
break;
} // case 80
case 88: {
quietDeps_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case 88
case 96: {
sourceMapIncludeSources_ = input.readBool();
bitField0_ |= 0x00000400;
break;
} // case 96
case 104: {
charset_ = input.readBool();
bitField0_ |= 0x00000800;
break;
} // case 104
case 112: {
silent_ = input.readBool();
bitField0_ |= 0x00001000;
break;
} // case 112
case 122: {
java.lang.String s = input.readStringRequireUtf8();
ensureFatalDeprecationIsMutable();
fatalDeprecation_.add(s);
break;
} // case 122
case 130: {
java.lang.String s = input.readStringRequireUtf8();
ensureSilenceDeprecationIsMutable();
silenceDeprecation_.add(s);
break;
} // case 130
case 138: {
java.lang.String s = input.readStringRequireUtf8();
ensureFutureDeprecationIsMutable();
futureDeprecation_.add(s);
break;
} // case 138
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int inputCase_ = 0;
private java.lang.Object input_;
public InputCase
getInputCase() {
return InputCase.forNumber(
inputCase_);
}
public Builder clearInput() {
inputCase_ = 0;
input_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInputOrBuilder> stringBuilder_;
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
* @return Whether the string field is set.
*/
@java.lang.Override
public boolean hasString() {
return inputCase_ == 2;
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
* @return The string.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput getString() {
if (stringBuilder_ == null) {
if (inputCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance();
} else {
if (inputCase_ == 2) {
return stringBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance();
}
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
public Builder setString(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput value) {
if (stringBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
input_ = value;
onChanged();
} else {
stringBuilder_.setMessage(value);
}
inputCase_ = 2;
return this;
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
public Builder setString(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.Builder builderForValue) {
if (stringBuilder_ == null) {
input_ = builderForValue.build();
onChanged();
} else {
stringBuilder_.setMessage(builderForValue.build());
}
inputCase_ = 2;
return this;
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
public Builder mergeString(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput value) {
if (stringBuilder_ == null) {
if (inputCase_ == 2 &&
input_ != com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance()) {
input_ = com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_)
.mergeFrom(value).buildPartial();
} else {
input_ = value;
}
onChanged();
} else {
if (inputCase_ == 2) {
stringBuilder_.mergeFrom(value);
} else {
stringBuilder_.setMessage(value);
}
}
inputCase_ = 2;
return this;
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
public Builder clearString() {
if (stringBuilder_ == null) {
if (inputCase_ == 2) {
inputCase_ = 0;
input_ = null;
onChanged();
}
} else {
if (inputCase_ == 2) {
inputCase_ = 0;
input_ = null;
}
stringBuilder_.clear();
}
return this;
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.Builder getStringBuilder() {
return getStringFieldBuilder().getBuilder();
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInputOrBuilder getStringOrBuilder() {
if ((inputCase_ == 2) && (stringBuilder_ != null)) {
return stringBuilder_.getMessageOrBuilder();
} else {
if (inputCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance();
}
}
/**
*
* A stylesheet loaded from its contents.
*
*
* .sass.embedded_protocol.InboundMessage.CompileRequest.StringInput string = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInputOrBuilder>
getStringFieldBuilder() {
if (stringBuilder_ == null) {
if (!(inputCase_ == 2)) {
input_ = com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.getDefaultInstance();
}
stringBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInputOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.StringInput) input_,
getParentForChildren(),
isClean());
input_ = null;
}
inputCase_ = 2;
onChanged();
return stringBuilder_;
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return Whether the path field is set.
*/
@java.lang.Override
public boolean hasPath() {
return inputCase_ == 3;
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = "";
if (inputCase_ == 3) {
ref = input_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (inputCase_ == 3) {
input_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = "";
if (inputCase_ == 3) {
ref = input_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (inputCase_ == 3) {
input_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
inputCase_ = 3;
input_ = value;
onChanged();
return this;
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @return This builder for chaining.
*/
public Builder clearPath() {
if (inputCase_ == 3) {
inputCase_ = 0;
input_ = null;
onChanged();
}
return this;
}
/**
*
* A stylesheet loaded from the given path on the filesystem.
*
*
* string path = 3;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
inputCase_ = 3;
input_ = value;
onChanged();
return this;
}
private int style_ = 0;
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @return The enum numeric value on the wire for style.
*/
@java.lang.Override public int getStyleValue() {
return style_;
}
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @param value The enum numeric value on the wire for style to set.
* @return This builder for chaining.
*/
public Builder setStyleValue(int value) {
style_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @return The style.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.OutputStyle getStyle() {
com.sass_lang.embedded_protocol.OutputStyle result = com.sass_lang.embedded_protocol.OutputStyle.forNumber(style_);
return result == null ? com.sass_lang.embedded_protocol.OutputStyle.UNRECOGNIZED : result;
}
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @param value The style to set.
* @return This builder for chaining.
*/
public Builder setStyle(com.sass_lang.embedded_protocol.OutputStyle value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
style_ = value.getNumber();
onChanged();
return this;
}
/**
*
* How to format the CSS output.
*
*
* .sass.embedded_protocol.OutputStyle style = 4;
* @return This builder for chaining.
*/
public Builder clearStyle() {
bitField0_ = (bitField0_ & ~0x00000004);
style_ = 0;
onChanged();
return this;
}
private boolean sourceMap_ ;
/**
*
* Whether to generate a source map. Note that this will *not* add a source
* map comment to the stylesheet; that's up to the host or its users.
*
*
* bool source_map = 5;
* @return The sourceMap.
*/
@java.lang.Override
public boolean getSourceMap() {
return sourceMap_;
}
/**
*
* Whether to generate a source map. Note that this will *not* add a source
* map comment to the stylesheet; that's up to the host or its users.
*
*
* bool source_map = 5;
* @param value The sourceMap to set.
* @return This builder for chaining.
*/
public Builder setSourceMap(boolean value) {
sourceMap_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Whether to generate a source map. Note that this will *not* add a source
* map comment to the stylesheet; that's up to the host or its users.
*
*
* bool source_map = 5;
* @return This builder for chaining.
*/
public Builder clearSourceMap() {
bitField0_ = (bitField0_ & ~0x00000008);
sourceMap_ = false;
onChanged();
return this;
}
private java.util.List importers_ =
java.util.Collections.emptyList();
private void ensureImportersIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
importers_ = new java.util.ArrayList(importers_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder> importersBuilder_;
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public java.util.List getImportersList() {
if (importersBuilder_ == null) {
return java.util.Collections.unmodifiableList(importers_);
} else {
return importersBuilder_.getMessageList();
}
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public int getImportersCount() {
if (importersBuilder_ == null) {
return importers_.size();
} else {
return importersBuilder_.getCount();
}
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer getImporters(int index) {
if (importersBuilder_ == null) {
return importers_.get(index);
} else {
return importersBuilder_.getMessage(index);
}
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder setImporters(
int index, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer value) {
if (importersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureImportersIsMutable();
importers_.set(index, value);
onChanged();
} else {
importersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder setImporters(
int index, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder builderForValue) {
if (importersBuilder_ == null) {
ensureImportersIsMutable();
importers_.set(index, builderForValue.build());
onChanged();
} else {
importersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder addImporters(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer value) {
if (importersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureImportersIsMutable();
importers_.add(value);
onChanged();
} else {
importersBuilder_.addMessage(value);
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder addImporters(
int index, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer value) {
if (importersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureImportersIsMutable();
importers_.add(index, value);
onChanged();
} else {
importersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder addImporters(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder builderForValue) {
if (importersBuilder_ == null) {
ensureImportersIsMutable();
importers_.add(builderForValue.build());
onChanged();
} else {
importersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder addImporters(
int index, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder builderForValue) {
if (importersBuilder_ == null) {
ensureImportersIsMutable();
importers_.add(index, builderForValue.build());
onChanged();
} else {
importersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder addAllImporters(
java.lang.Iterable extends com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer> values) {
if (importersBuilder_ == null) {
ensureImportersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, importers_);
onChanged();
} else {
importersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder clearImporters() {
if (importersBuilder_ == null) {
importers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
importersBuilder_.clear();
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public Builder removeImporters(int index) {
if (importersBuilder_ == null) {
ensureImportersIsMutable();
importers_.remove(index);
onChanged();
} else {
importersBuilder_.remove(index);
}
return this;
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder getImportersBuilder(
int index) {
return getImportersFieldBuilder().getBuilder(index);
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder getImportersOrBuilder(
int index) {
if (importersBuilder_ == null) {
return importers_.get(index); } else {
return importersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public java.util.List extends com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder>
getImportersOrBuilderList() {
if (importersBuilder_ != null) {
return importersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(importers_);
}
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder addImportersBuilder() {
return getImportersFieldBuilder().addBuilder(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance());
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder addImportersBuilder(
int index) {
return getImportersFieldBuilder().addBuilder(
index, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.getDefaultInstance());
}
/**
*
* Importers (including load paths on the filesystem) to use when resolving
* imports that can't be resolved relative to the file that contains it. Each
* importer is checked in order until one recognizes the imported URL.
*
*
* repeated .sass.embedded_protocol.InboundMessage.CompileRequest.Importer importers = 6;
*/
public java.util.List
getImportersBuilderList() {
return getImportersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder>
getImportersFieldBuilder() {
if (importersBuilder_ == null) {
importersBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Importer.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.ImporterOrBuilder>(
importers_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
importers_ = null;
}
return importersBuilder_;
}
private com.google.protobuf.LazyStringArrayList globalFunctions_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureGlobalFunctionsIsMutable() {
if (!globalFunctions_.isModifiable()) {
globalFunctions_ = new com.google.protobuf.LazyStringArrayList(globalFunctions_);
}
bitField0_ |= 0x00000020;
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @return A list containing the globalFunctions.
*/
public com.google.protobuf.ProtocolStringList
getGlobalFunctionsList() {
globalFunctions_.makeImmutable();
return globalFunctions_;
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @return The count of globalFunctions.
*/
public int getGlobalFunctionsCount() {
return globalFunctions_.size();
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param index The index of the element to return.
* @return The globalFunctions at the given index.
*/
public java.lang.String getGlobalFunctions(int index) {
return globalFunctions_.get(index);
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param index The index of the value to return.
* @return The bytes of the globalFunctions at the given index.
*/
public com.google.protobuf.ByteString
getGlobalFunctionsBytes(int index) {
return globalFunctions_.getByteString(index);
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param index The index to set the value at.
* @param value The globalFunctions to set.
* @return This builder for chaining.
*/
public Builder setGlobalFunctions(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureGlobalFunctionsIsMutable();
globalFunctions_.set(index, value);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param value The globalFunctions to add.
* @return This builder for chaining.
*/
public Builder addGlobalFunctions(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureGlobalFunctionsIsMutable();
globalFunctions_.add(value);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param values The globalFunctions to add.
* @return This builder for chaining.
*/
public Builder addAllGlobalFunctions(
java.lang.Iterable values) {
ensureGlobalFunctionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, globalFunctions_);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @return This builder for chaining.
*/
public Builder clearGlobalFunctions() {
globalFunctions_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);;
onChanged();
return this;
}
/**
*
* Signatures for custom global functions whose behavior is defined by the
* host.
*
* If this is not a valid Sass function signature that could appear after
* `@function` in a Sass stylesheet (such as `mix($color1, $color2, $weight:
* 50%)`), or if it conflicts with a function name that's built into the
* Sass language, the compiler must treat the compilation as failed.
*
* Compilers must ensure that pure-Sass functions take precedence over
* custom global functions.
*
*
* repeated string global_functions = 7;
* @param value The bytes of the globalFunctions to add.
* @return This builder for chaining.
*/
public Builder addGlobalFunctionsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureGlobalFunctionsIsMutable();
globalFunctions_.add(value);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private boolean alertColor_ ;
/**
*
* Whether to use terminal colors in the formatted message of errors and
* logs.
*
*
* bool alert_color = 8;
* @return The alertColor.
*/
@java.lang.Override
public boolean getAlertColor() {
return alertColor_;
}
/**
*
* Whether to use terminal colors in the formatted message of errors and
* logs.
*
*
* bool alert_color = 8;
* @param value The alertColor to set.
* @return This builder for chaining.
*/
public Builder setAlertColor(boolean value) {
alertColor_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Whether to use terminal colors in the formatted message of errors and
* logs.
*
*
* bool alert_color = 8;
* @return This builder for chaining.
*/
public Builder clearAlertColor() {
bitField0_ = (bitField0_ & ~0x00000040);
alertColor_ = false;
onChanged();
return this;
}
private boolean alertAscii_ ;
/**
*
* Whether to encode the formatted message of errors and logs in ASCII.
*
*
* bool alert_ascii = 9;
* @return The alertAscii.
*/
@java.lang.Override
public boolean getAlertAscii() {
return alertAscii_;
}
/**
*
* Whether to encode the formatted message of errors and logs in ASCII.
*
*
* bool alert_ascii = 9;
* @param value The alertAscii to set.
* @return This builder for chaining.
*/
public Builder setAlertAscii(boolean value) {
alertAscii_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Whether to encode the formatted message of errors and logs in ASCII.
*
*
* bool alert_ascii = 9;
* @return This builder for chaining.
*/
public Builder clearAlertAscii() {
bitField0_ = (bitField0_ & ~0x00000080);
alertAscii_ = false;
onChanged();
return this;
}
private boolean verbose_ ;
/**
*
* Whether to report all deprecation warnings or only the first few ones.
* If this is `false`, the compiler may choose not to send events for
* repeated deprecation warnings. If this is `true`, the compiler must emit
* an event for every deprecation warning it encounters.
*
*
* bool verbose = 10;
* @return The verbose.
*/
@java.lang.Override
public boolean getVerbose() {
return verbose_;
}
/**
*
* Whether to report all deprecation warnings or only the first few ones.
* If this is `false`, the compiler may choose not to send events for
* repeated deprecation warnings. If this is `true`, the compiler must emit
* an event for every deprecation warning it encounters.
*
*
* bool verbose = 10;
* @param value The verbose to set.
* @return This builder for chaining.
*/
public Builder setVerbose(boolean value) {
verbose_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Whether to report all deprecation warnings or only the first few ones.
* If this is `false`, the compiler may choose not to send events for
* repeated deprecation warnings. If this is `true`, the compiler must emit
* an event for every deprecation warning it encounters.
*
*
* bool verbose = 10;
* @return This builder for chaining.
*/
public Builder clearVerbose() {
bitField0_ = (bitField0_ & ~0x00000100);
verbose_ = false;
onChanged();
return this;
}
private boolean quietDeps_ ;
/**
*
* Whether to omit events for deprecation warnings coming from dependencies
* (files loaded from a different importer than the input).
*
*
* bool quiet_deps = 11;
* @return The quietDeps.
*/
@java.lang.Override
public boolean getQuietDeps() {
return quietDeps_;
}
/**
*
* Whether to omit events for deprecation warnings coming from dependencies
* (files loaded from a different importer than the input).
*
*
* bool quiet_deps = 11;
* @param value The quietDeps to set.
* @return This builder for chaining.
*/
public Builder setQuietDeps(boolean value) {
quietDeps_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Whether to omit events for deprecation warnings coming from dependencies
* (files loaded from a different importer than the input).
*
*
* bool quiet_deps = 11;
* @return This builder for chaining.
*/
public Builder clearQuietDeps() {
bitField0_ = (bitField0_ & ~0x00000200);
quietDeps_ = false;
onChanged();
return this;
}
private boolean sourceMapIncludeSources_ ;
/**
*
* Whether to include sources in the generated sourcemap
*
*
* bool source_map_include_sources = 12;
* @return The sourceMapIncludeSources.
*/
@java.lang.Override
public boolean getSourceMapIncludeSources() {
return sourceMapIncludeSources_;
}
/**
*
* Whether to include sources in the generated sourcemap
*
*
* bool source_map_include_sources = 12;
* @param value The sourceMapIncludeSources to set.
* @return This builder for chaining.
*/
public Builder setSourceMapIncludeSources(boolean value) {
sourceMapIncludeSources_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* Whether to include sources in the generated sourcemap
*
*
* bool source_map_include_sources = 12;
* @return This builder for chaining.
*/
public Builder clearSourceMapIncludeSources() {
bitField0_ = (bitField0_ & ~0x00000400);
sourceMapIncludeSources_ = false;
onChanged();
return this;
}
private boolean charset_ ;
/**
*
* Whether to emit a `@charset`/BOM for non-ASCII stylesheets.
*
*
* bool charset = 13;
* @return The charset.
*/
@java.lang.Override
public boolean getCharset() {
return charset_;
}
/**
*
* Whether to emit a `@charset`/BOM for non-ASCII stylesheets.
*
*
* bool charset = 13;
* @param value The charset to set.
* @return This builder for chaining.
*/
public Builder setCharset(boolean value) {
charset_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* Whether to emit a `@charset`/BOM for non-ASCII stylesheets.
*
*
* bool charset = 13;
* @return This builder for chaining.
*/
public Builder clearCharset() {
bitField0_ = (bitField0_ & ~0x00000800);
charset_ = false;
onChanged();
return this;
}
private boolean silent_ ;
/**
*
* Whether to silently suppresses all `LogEvent`s.
*
*
* bool silent = 14;
* @return The silent.
*/
@java.lang.Override
public boolean getSilent() {
return silent_;
}
/**
*
* Whether to silently suppresses all `LogEvent`s.
*
*
* bool silent = 14;
* @param value The silent to set.
* @return This builder for chaining.
*/
public Builder setSilent(boolean value) {
silent_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* Whether to silently suppresses all `LogEvent`s.
*
*
* bool silent = 14;
* @return This builder for chaining.
*/
public Builder clearSilent() {
bitField0_ = (bitField0_ & ~0x00001000);
silent_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList fatalDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureFatalDeprecationIsMutable() {
if (!fatalDeprecation_.isModifiable()) {
fatalDeprecation_ = new com.google.protobuf.LazyStringArrayList(fatalDeprecation_);
}
bitField0_ |= 0x00002000;
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @return A list containing the fatalDeprecation.
*/
public com.google.protobuf.ProtocolStringList
getFatalDeprecationList() {
fatalDeprecation_.makeImmutable();
return fatalDeprecation_;
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @return The count of fatalDeprecation.
*/
public int getFatalDeprecationCount() {
return fatalDeprecation_.size();
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param index The index of the element to return.
* @return The fatalDeprecation at the given index.
*/
public java.lang.String getFatalDeprecation(int index) {
return fatalDeprecation_.get(index);
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param index The index of the value to return.
* @return The bytes of the fatalDeprecation at the given index.
*/
public com.google.protobuf.ByteString
getFatalDeprecationBytes(int index) {
return fatalDeprecation_.getByteString(index);
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param index The index to set the value at.
* @param value The fatalDeprecation to set.
* @return This builder for chaining.
*/
public Builder setFatalDeprecation(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureFatalDeprecationIsMutable();
fatalDeprecation_.set(index, value);
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param value The fatalDeprecation to add.
* @return This builder for chaining.
*/
public Builder addFatalDeprecation(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureFatalDeprecationIsMutable();
fatalDeprecation_.add(value);
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param values The fatalDeprecation to add.
* @return This builder for chaining.
*/
public Builder addAllFatalDeprecation(
java.lang.Iterable values) {
ensureFatalDeprecationIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fatalDeprecation_);
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @return This builder for chaining.
*/
public Builder clearFatalDeprecation() {
fatalDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00002000);;
onChanged();
return this;
}
/**
*
* Deprecation IDs or versions to treat as fatal.
*
*
* repeated string fatal_deprecation = 15;
* @param value The bytes of the fatalDeprecation to add.
* @return This builder for chaining.
*/
public Builder addFatalDeprecationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureFatalDeprecationIsMutable();
fatalDeprecation_.add(value);
bitField0_ |= 0x00002000;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList silenceDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSilenceDeprecationIsMutable() {
if (!silenceDeprecation_.isModifiable()) {
silenceDeprecation_ = new com.google.protobuf.LazyStringArrayList(silenceDeprecation_);
}
bitField0_ |= 0x00004000;
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @return A list containing the silenceDeprecation.
*/
public com.google.protobuf.ProtocolStringList
getSilenceDeprecationList() {
silenceDeprecation_.makeImmutable();
return silenceDeprecation_;
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @return The count of silenceDeprecation.
*/
public int getSilenceDeprecationCount() {
return silenceDeprecation_.size();
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param index The index of the element to return.
* @return The silenceDeprecation at the given index.
*/
public java.lang.String getSilenceDeprecation(int index) {
return silenceDeprecation_.get(index);
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param index The index of the value to return.
* @return The bytes of the silenceDeprecation at the given index.
*/
public com.google.protobuf.ByteString
getSilenceDeprecationBytes(int index) {
return silenceDeprecation_.getByteString(index);
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param index The index to set the value at.
* @param value The silenceDeprecation to set.
* @return This builder for chaining.
*/
public Builder setSilenceDeprecation(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureSilenceDeprecationIsMutable();
silenceDeprecation_.set(index, value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param value The silenceDeprecation to add.
* @return This builder for chaining.
*/
public Builder addSilenceDeprecation(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureSilenceDeprecationIsMutable();
silenceDeprecation_.add(value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param values The silenceDeprecation to add.
* @return This builder for chaining.
*/
public Builder addAllSilenceDeprecation(
java.lang.Iterable values) {
ensureSilenceDeprecationIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, silenceDeprecation_);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @return This builder for chaining.
*/
public Builder clearSilenceDeprecation() {
silenceDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00004000);;
onChanged();
return this;
}
/**
*
* Deprecation IDs to ignore.
*
*
* repeated string silence_deprecation = 16;
* @param value The bytes of the silenceDeprecation to add.
* @return This builder for chaining.
*/
public Builder addSilenceDeprecationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureSilenceDeprecationIsMutable();
silenceDeprecation_.add(value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList futureDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureFutureDeprecationIsMutable() {
if (!futureDeprecation_.isModifiable()) {
futureDeprecation_ = new com.google.protobuf.LazyStringArrayList(futureDeprecation_);
}
bitField0_ |= 0x00008000;
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @return A list containing the futureDeprecation.
*/
public com.google.protobuf.ProtocolStringList
getFutureDeprecationList() {
futureDeprecation_.makeImmutable();
return futureDeprecation_;
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @return The count of futureDeprecation.
*/
public int getFutureDeprecationCount() {
return futureDeprecation_.size();
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param index The index of the element to return.
* @return The futureDeprecation at the given index.
*/
public java.lang.String getFutureDeprecation(int index) {
return futureDeprecation_.get(index);
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param index The index of the value to return.
* @return The bytes of the futureDeprecation at the given index.
*/
public com.google.protobuf.ByteString
getFutureDeprecationBytes(int index) {
return futureDeprecation_.getByteString(index);
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param index The index to set the value at.
* @param value The futureDeprecation to set.
* @return This builder for chaining.
*/
public Builder setFutureDeprecation(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureFutureDeprecationIsMutable();
futureDeprecation_.set(index, value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param value The futureDeprecation to add.
* @return This builder for chaining.
*/
public Builder addFutureDeprecation(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureFutureDeprecationIsMutable();
futureDeprecation_.add(value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param values The futureDeprecation to add.
* @return This builder for chaining.
*/
public Builder addAllFutureDeprecation(
java.lang.Iterable values) {
ensureFutureDeprecationIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, futureDeprecation_);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @return This builder for chaining.
*/
public Builder clearFutureDeprecation() {
futureDeprecation_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00008000);;
onChanged();
return this;
}
/**
*
* Deprecation IDs to opt into early.
*
*
* repeated string future_deprecation = 17;
* @param value The bytes of the futureDeprecation to add.
* @return This builder for chaining.
*/
public Builder addFutureDeprecationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureFutureDeprecationIsMutable();
futureDeprecation_.add(value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.CompileRequest)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.CompileRequest)
private static final com.sass_lang.embedded_protocol.InboundMessage.CompileRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.CompileRequest();
}
public static com.sass_lang.embedded_protocol.InboundMessage.CompileRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CompileRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CanonicalizeResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.CanonicalizeResponse)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 id = 1;
* @return The id.
*/
int getId();
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return Whether the url field is set.
*/
boolean hasUrl();
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return The url.
*/
java.lang.String getUrl();
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return The bytes for url.
*/
com.google.protobuf.ByteString
getUrlBytes();
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
boolean hasError();
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return The error.
*/
java.lang.String getError();
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return The bytes for error.
*/
com.google.protobuf.ByteString
getErrorBytes();
/**
*
* Whether `containing_url` in `CanonicalizeRequest` is unused.
*
* The compiler can cache the `CanonicalizeResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `CanonicalizeResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return The containingUrlUnused.
*/
boolean getContainingUrlUnused();
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.ResultCase getResultCase();
}
/**
*
* A response indicating the result of canonicalizing an imported URL.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CanonicalizeResponse}
*/
public static final class CanonicalizeResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.CanonicalizeResponse)
CanonicalizeResponseOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
CanonicalizeResponse.class.getName());
}
// Use CanonicalizeResponse.newBuilder() to construct.
private CanonicalizeResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CanonicalizeResponse() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CanonicalizeResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CanonicalizeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.class, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.Builder.class);
}
private int resultCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object result_;
public enum ResultCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
URL(2),
ERROR(3),
RESULT_NOT_SET(0);
private final int value;
private ResultCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResultCase valueOf(int value) {
return forNumber(value);
}
public static ResultCase forNumber(int value) {
switch (value) {
case 2: return URL;
case 3: return ERROR;
case 0: return RESULT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public static final int ID_FIELD_NUMBER = 1;
private int id_ = 0;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
public static final int URL_FIELD_NUMBER = 2;
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return Whether the url field is set.
*/
public boolean hasUrl() {
return resultCase_ == 2;
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return The url.
*/
public java.lang.String getUrl() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 2) {
result_ = s;
}
return s;
}
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return The bytes for url.
*/
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 2) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ERROR_FIELD_NUMBER = 3;
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return The error.
*/
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
}
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return The bytes for error.
*/
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTAINING_URL_UNUSED_FIELD_NUMBER = 4;
private boolean containingUrlUnused_ = false;
/**
*
* Whether `containing_url` in `CanonicalizeRequest` is unused.
*
* The compiler can cache the `CanonicalizeResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `CanonicalizeResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return The containingUrlUnused.
*/
@java.lang.Override
public boolean getContainingUrlUnused() {
return containingUrlUnused_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0) {
output.writeUInt32(1, id_);
}
if (resultCase_ == 2) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, result_);
}
if (resultCase_ == 3) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, result_);
}
if (containingUrlUnused_ != false) {
output.writeBool(4, containingUrlUnused_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (resultCase_ == 2) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, result_);
}
if (resultCase_ == 3) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, result_);
}
if (containingUrlUnused_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, containingUrlUnused_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse other = (com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) obj;
if (getId()
!= other.getId()) return false;
if (getContainingUrlUnused()
!= other.getContainingUrlUnused()) return false;
if (!getResultCase().equals(other.getResultCase())) return false;
switch (resultCase_) {
case 2:
if (!getUrl()
.equals(other.getUrl())) return false;
break;
case 3:
if (!getError()
.equals(other.getError())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
hash = (37 * hash) + CONTAINING_URL_UNUSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getContainingUrlUnused());
switch (resultCase_) {
case 2:
hash = (37 * hash) + URL_FIELD_NUMBER;
hash = (53 * hash) + getUrl().hashCode();
break;
case 3:
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A response indicating the result of canonicalizing an imported URL.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.CanonicalizeResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.CanonicalizeResponse)
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CanonicalizeResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CanonicalizeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.class, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = 0;
containingUrlUnused_ = false;
resultCase_ = 0;
result_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_CanonicalizeResponse_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse build() {
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse result = new com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.containingUrlUnused_ = containingUrlUnused_;
}
}
private void buildPartialOneofs(com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse result) {
result.resultCase_ = resultCase_;
result.result_ = this.result_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance()) return this;
if (other.getId() != 0) {
setId(other.getId());
}
if (other.getContainingUrlUnused() != false) {
setContainingUrlUnused(other.getContainingUrlUnused());
}
switch (other.getResultCase()) {
case URL: {
resultCase_ = 2;
result_ = other.result_;
onChanged();
break;
}
case ERROR: {
resultCase_ = 3;
result_ = other.result_;
onChanged();
break;
}
case RESULT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
id_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
java.lang.String s = input.readStringRequireUtf8();
resultCase_ = 2;
result_ = s;
break;
} // case 18
case 26: {
java.lang.String s = input.readStringRequireUtf8();
resultCase_ = 3;
result_ = s;
break;
} // case 26
case 32: {
containingUrlUnused_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int resultCase_ = 0;
private java.lang.Object result_;
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public Builder clearResult() {
resultCase_ = 0;
result_ = null;
onChanged();
return this;
}
private int bitField0_;
private int id_ ;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
* uint32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* uint32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return resultCase_ == 2;
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return The url.
*/
@java.lang.Override
public java.lang.String getUrl() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 2) {
result_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return The bytes for url.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 2) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @param value The url to set.
* @return This builder for chaining.
*/
public Builder setUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resultCase_ = 2;
result_ = value;
onChanged();
return this;
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @return This builder for chaining.
*/
public Builder clearUrl() {
if (resultCase_ == 2) {
resultCase_ = 0;
result_ = null;
onChanged();
}
return this;
}
/**
*
* The successfully canonicalized URL.
*
* If this is not an absolute URL (including scheme), the compiler must
* treat that as an error thrown by the importer. If this URL's scheme is
* an `Importer.non_canonical_scheme` for the importer being invoked, the
* compiler must treat that as an error thrown by the importer.
*
*
* string url = 2;
* @param value The bytes for url to set.
* @return This builder for chaining.
*/
public Builder setUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resultCase_ = 2;
result_ = value;
onChanged();
return this;
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
@java.lang.Override
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return The error.
*/
@java.lang.Override
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return The bytes for error.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @param value The error to set.
* @return This builder for chaining.
*/
public Builder setError(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @return This builder for chaining.
*/
public Builder clearError() {
if (resultCase_ == 3) {
resultCase_ = 0;
result_ = null;
onChanged();
}
return this;
}
/**
*
* An error message explaining why canonicalization failed.
*
* This indicates that a stylesheet was found, but a canonical URL for it
* could not be determined. If no stylesheet was found, `result` should be
* `null` instead.
*
*
* string error = 3;
* @param value The bytes for error to set.
* @return This builder for chaining.
*/
public Builder setErrorBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
private boolean containingUrlUnused_ ;
/**
*
* Whether `containing_url` in `CanonicalizeRequest` is unused.
*
* The compiler can cache the `CanonicalizeResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `CanonicalizeResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return The containingUrlUnused.
*/
@java.lang.Override
public boolean getContainingUrlUnused() {
return containingUrlUnused_;
}
/**
*
* Whether `containing_url` in `CanonicalizeRequest` is unused.
*
* The compiler can cache the `CanonicalizeResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `CanonicalizeResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @param value The containingUrlUnused to set.
* @return This builder for chaining.
*/
public Builder setContainingUrlUnused(boolean value) {
containingUrlUnused_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Whether `containing_url` in `CanonicalizeRequest` is unused.
*
* The compiler can cache the `CanonicalizeResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `CanonicalizeResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return This builder for chaining.
*/
public Builder clearContainingUrlUnused() {
bitField0_ = (bitField0_ & ~0x00000008);
containingUrlUnused_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.CanonicalizeResponse)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.CanonicalizeResponse)
private static final com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse();
}
public static com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CanonicalizeResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ImportResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.ImportResponse)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 id = 1;
* @return The id.
*/
int getId();
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
* @return Whether the success field is set.
*/
boolean hasSuccess();
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
* @return The success.
*/
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess getSuccess();
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccessOrBuilder getSuccessOrBuilder();
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
boolean hasError();
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The error.
*/
java.lang.String getError();
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The bytes for error.
*/
com.google.protobuf.ByteString
getErrorBytes();
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ResultCase getResultCase();
}
/**
*
* A response indicating the result of importing a canonical URL.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.ImportResponse}
*/
public static final class ImportResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.ImportResponse)
ImportResponseOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ImportResponse.class.getName());
}
// Use ImportResponse.newBuilder() to construct.
private ImportResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ImportResponse() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.class, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.Builder.class);
}
public interface ImportSuccessOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess)
com.google.protobuf.MessageOrBuilder {
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @return The contents.
*/
java.lang.String getContents();
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @return The bytes for contents.
*/
com.google.protobuf.ByteString
getContentsBytes();
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @return The enum numeric value on the wire for syntax.
*/
int getSyntaxValue();
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @return The syntax.
*/
com.sass_lang.embedded_protocol.Syntax getSyntax();
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return Whether the sourceMapUrl field is set.
*/
boolean hasSourceMapUrl();
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return The sourceMapUrl.
*/
java.lang.String getSourceMapUrl();
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return The bytes for sourceMapUrl.
*/
com.google.protobuf.ByteString
getSourceMapUrlBytes();
}
/**
*
* The stylesheet's contents were loaded successfully.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess}
*/
public static final class ImportSuccess extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess)
ImportSuccessOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ImportSuccess.class.getName());
}
// Use ImportSuccess.newBuilder() to construct.
private ImportSuccess(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ImportSuccess() {
contents_ = "";
syntax_ = 0;
sourceMapUrl_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_ImportSuccess_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_ImportSuccess_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.class, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.Builder.class);
}
private int bitField0_;
public static final int CONTENTS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object contents_ = "";
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @return The contents.
*/
@java.lang.Override
public java.lang.String getContents() {
java.lang.Object ref = contents_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contents_ = s;
return s;
}
}
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @return The bytes for contents.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContentsBytes() {
java.lang.Object ref = contents_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contents_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SYNTAX_FIELD_NUMBER = 2;
private int syntax_ = 0;
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @return The enum numeric value on the wire for syntax.
*/
@java.lang.Override public int getSyntaxValue() {
return syntax_;
}
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @return The syntax.
*/
@java.lang.Override public com.sass_lang.embedded_protocol.Syntax getSyntax() {
com.sass_lang.embedded_protocol.Syntax result = com.sass_lang.embedded_protocol.Syntax.forNumber(syntax_);
return result == null ? com.sass_lang.embedded_protocol.Syntax.UNRECOGNIZED : result;
}
public static final int SOURCE_MAP_URL_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceMapUrl_ = "";
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return Whether the sourceMapUrl field is set.
*/
@java.lang.Override
public boolean hasSourceMapUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return The sourceMapUrl.
*/
@java.lang.Override
public java.lang.String getSourceMapUrl() {
java.lang.Object ref = sourceMapUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceMapUrl_ = s;
return s;
}
}
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return The bytes for sourceMapUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSourceMapUrlBytes() {
java.lang.Object ref = sourceMapUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceMapUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(contents_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, contents_);
}
if (syntax_ != com.sass_lang.embedded_protocol.Syntax.SCSS.getNumber()) {
output.writeEnum(2, syntax_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, sourceMapUrl_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(contents_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, contents_);
}
if (syntax_ != com.sass_lang.embedded_protocol.Syntax.SCSS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, syntax_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, sourceMapUrl_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess other = (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) obj;
if (!getContents()
.equals(other.getContents())) return false;
if (syntax_ != other.syntax_) return false;
if (hasSourceMapUrl() != other.hasSourceMapUrl()) return false;
if (hasSourceMapUrl()) {
if (!getSourceMapUrl()
.equals(other.getSourceMapUrl())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTENTS_FIELD_NUMBER;
hash = (53 * hash) + getContents().hashCode();
hash = (37 * hash) + SYNTAX_FIELD_NUMBER;
hash = (53 * hash) + syntax_;
if (hasSourceMapUrl()) {
hash = (37 * hash) + SOURCE_MAP_URL_FIELD_NUMBER;
hash = (53 * hash) + getSourceMapUrl().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The stylesheet's contents were loaded successfully.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess)
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccessOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_ImportSuccess_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_ImportSuccess_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.class, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
contents_ = "";
syntax_ = 0;
sourceMapUrl_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_ImportSuccess_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess build() {
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess result = new com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.contents_ = contents_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.syntax_ = syntax_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.sourceMapUrl_ = sourceMapUrl_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance()) return this;
if (!other.getContents().isEmpty()) {
contents_ = other.contents_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.syntax_ != 0) {
setSyntaxValue(other.getSyntaxValue());
}
if (other.hasSourceMapUrl()) {
sourceMapUrl_ = other.sourceMapUrl_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
contents_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
syntax_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
sourceMapUrl_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object contents_ = "";
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @return The contents.
*/
public java.lang.String getContents() {
java.lang.Object ref = contents_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contents_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @return The bytes for contents.
*/
public com.google.protobuf.ByteString
getContentsBytes() {
java.lang.Object ref = contents_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contents_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @param value The contents to set.
* @return This builder for chaining.
*/
public Builder setContents(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
contents_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @return This builder for chaining.
*/
public Builder clearContents() {
contents_ = getDefaultInstance().getContents();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The text of the stylesheet.
*
*
* string contents = 1;
* @param value The bytes for contents to set.
* @return This builder for chaining.
*/
public Builder setContentsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
contents_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int syntax_ = 0;
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @return The enum numeric value on the wire for syntax.
*/
@java.lang.Override public int getSyntaxValue() {
return syntax_;
}
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @param value The enum numeric value on the wire for syntax to set.
* @return This builder for chaining.
*/
public Builder setSyntaxValue(int value) {
syntax_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @return The syntax.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.Syntax getSyntax() {
com.sass_lang.embedded_protocol.Syntax result = com.sass_lang.embedded_protocol.Syntax.forNumber(syntax_);
return result == null ? com.sass_lang.embedded_protocol.Syntax.UNRECOGNIZED : result;
}
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @param value The syntax to set.
* @return This builder for chaining.
*/
public Builder setSyntax(com.sass_lang.embedded_protocol.Syntax value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
syntax_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The syntax of `contents`.
*
*
* .sass.embedded_protocol.Syntax syntax = 2;
* @return This builder for chaining.
*/
public Builder clearSyntax() {
bitField0_ = (bitField0_ & ~0x00000002);
syntax_ = 0;
onChanged();
return this;
}
private java.lang.Object sourceMapUrl_ = "";
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return Whether the sourceMapUrl field is set.
*/
public boolean hasSourceMapUrl() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return The sourceMapUrl.
*/
public java.lang.String getSourceMapUrl() {
java.lang.Object ref = sourceMapUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceMapUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return The bytes for sourceMapUrl.
*/
public com.google.protobuf.ByteString
getSourceMapUrlBytes() {
java.lang.Object ref = sourceMapUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceMapUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @param value The sourceMapUrl to set.
* @return This builder for chaining.
*/
public Builder setSourceMapUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
sourceMapUrl_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @return This builder for chaining.
*/
public Builder clearSourceMapUrl() {
sourceMapUrl_ = getDefaultInstance().getSourceMapUrl();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* An absolute, browser-accessible URL indicating the resolved location of
* the imported stylesheet.
*
* This should be a `file:` URL if one is available, but an `http:` URL is
* acceptable as well. If no URL is supplied, a `data:` URL is generated
* automatically from `contents`.
*
* If this is provided and is not an absolute URL (including scheme) the
* compiler must treat that as an error thrown by the importer.
*
*
* optional string source_map_url = 3;
* @param value The bytes for sourceMapUrl to set.
* @return This builder for chaining.
*/
public Builder setSourceMapUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
sourceMapUrl_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess)
private static final com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess();
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ImportSuccess parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int resultCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object result_;
public enum ResultCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
SUCCESS(2),
ERROR(3),
RESULT_NOT_SET(0);
private final int value;
private ResultCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResultCase valueOf(int value) {
return forNumber(value);
}
public static ResultCase forNumber(int value) {
switch (value) {
case 2: return SUCCESS;
case 3: return ERROR;
case 0: return RESULT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public static final int ID_FIELD_NUMBER = 1;
private int id_ = 0;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
public static final int SUCCESS_FIELD_NUMBER = 2;
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
* @return Whether the success field is set.
*/
@java.lang.Override
public boolean hasSuccess() {
return resultCase_ == 2;
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
* @return The success.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess getSuccess() {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance();
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccessOrBuilder getSuccessOrBuilder() {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance();
}
public static final int ERROR_FIELD_NUMBER = 3;
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The error.
*/
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
}
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The bytes for error.
*/
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0) {
output.writeUInt32(1, id_);
}
if (resultCase_ == 2) {
output.writeMessage(2, (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_);
}
if (resultCase_ == 3) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, result_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (resultCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_);
}
if (resultCase_ == 3) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, result_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.ImportResponse)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse other = (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) obj;
if (getId()
!= other.getId()) return false;
if (!getResultCase().equals(other.getResultCase())) return false;
switch (resultCase_) {
case 2:
if (!getSuccess()
.equals(other.getSuccess())) return false;
break;
case 3:
if (!getError()
.equals(other.getError())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
switch (resultCase_) {
case 2:
hash = (37 * hash) + SUCCESS_FIELD_NUMBER;
hash = (53 * hash) + getSuccess().hashCode();
break;
case 3:
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A response indicating the result of importing a canonical URL.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.ImportResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.ImportResponse)
com.sass_lang.embedded_protocol.InboundMessage.ImportResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.class, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = 0;
if (successBuilder_ != null) {
successBuilder_.clear();
}
resultCase_ = 0;
result_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_ImportResponse_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse build() {
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse result = new com.sass_lang.embedded_protocol.InboundMessage.ImportResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
}
}
private void buildPartialOneofs(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse result) {
result.resultCase_ = resultCase_;
result.result_ = this.result_;
if (resultCase_ == 2 &&
successBuilder_ != null) {
result.result_ = successBuilder_.build();
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.ImportResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance()) return this;
if (other.getId() != 0) {
setId(other.getId());
}
switch (other.getResultCase()) {
case SUCCESS: {
mergeSuccess(other.getSuccess());
break;
}
case ERROR: {
resultCase_ = 3;
result_ = other.result_;
onChanged();
break;
}
case RESULT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
id_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getSuccessFieldBuilder().getBuilder(),
extensionRegistry);
resultCase_ = 2;
break;
} // case 18
case 26: {
java.lang.String s = input.readStringRequireUtf8();
resultCase_ = 3;
result_ = s;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int resultCase_ = 0;
private java.lang.Object result_;
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public Builder clearResult() {
resultCase_ = 0;
result_ = null;
onChanged();
return this;
}
private int bitField0_;
private int id_ ;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
* uint32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* uint32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.Builder, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccessOrBuilder> successBuilder_;
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
* @return Whether the success field is set.
*/
@java.lang.Override
public boolean hasSuccess() {
return resultCase_ == 2;
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
* @return The success.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess getSuccess() {
if (successBuilder_ == null) {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance();
} else {
if (resultCase_ == 2) {
return successBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance();
}
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
public Builder setSuccess(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess value) {
if (successBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
result_ = value;
onChanged();
} else {
successBuilder_.setMessage(value);
}
resultCase_ = 2;
return this;
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
public Builder setSuccess(
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.Builder builderForValue) {
if (successBuilder_ == null) {
result_ = builderForValue.build();
onChanged();
} else {
successBuilder_.setMessage(builderForValue.build());
}
resultCase_ = 2;
return this;
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
public Builder mergeSuccess(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess value) {
if (successBuilder_ == null) {
if (resultCase_ == 2 &&
result_ != com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance()) {
result_ = com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_)
.mergeFrom(value).buildPartial();
} else {
result_ = value;
}
onChanged();
} else {
if (resultCase_ == 2) {
successBuilder_.mergeFrom(value);
} else {
successBuilder_.setMessage(value);
}
}
resultCase_ = 2;
return this;
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
public Builder clearSuccess() {
if (successBuilder_ == null) {
if (resultCase_ == 2) {
resultCase_ = 0;
result_ = null;
onChanged();
}
} else {
if (resultCase_ == 2) {
resultCase_ = 0;
result_ = null;
}
successBuilder_.clear();
}
return this;
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.Builder getSuccessBuilder() {
return getSuccessFieldBuilder().getBuilder();
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccessOrBuilder getSuccessOrBuilder() {
if ((resultCase_ == 2) && (successBuilder_ != null)) {
return successBuilder_.getMessageOrBuilder();
} else {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance();
}
}
/**
*
* The contents of the loaded stylesheet.
*
*
* .sass.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess success = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.Builder, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccessOrBuilder>
getSuccessFieldBuilder() {
if (successBuilder_ == null) {
if (!(resultCase_ == 2)) {
result_ = com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.getDefaultInstance();
}
successBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess.Builder, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccessOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.ImportSuccess) result_,
getParentForChildren(),
isClean());
result_ = null;
}
resultCase_ = 2;
onChanged();
return successBuilder_;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
@java.lang.Override
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The error.
*/
@java.lang.Override
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The bytes for error.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @param value The error to set.
* @return This builder for chaining.
*/
public Builder setError(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return This builder for chaining.
*/
public Builder clearError() {
if (resultCase_ == 3) {
resultCase_ = 0;
result_ = null;
onChanged();
}
return this;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @param value The bytes for error to set.
* @return This builder for chaining.
*/
public Builder setErrorBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.ImportResponse)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.ImportResponse)
private static final com.sass_lang.embedded_protocol.InboundMessage.ImportResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.ImportResponse();
}
public static com.sass_lang.embedded_protocol.InboundMessage.ImportResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ImportResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FileImportResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.FileImportResponse)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 id = 1;
* @return The id.
*/
int getId();
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return Whether the fileUrl field is set.
*/
boolean hasFileUrl();
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return The fileUrl.
*/
java.lang.String getFileUrl();
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return The bytes for fileUrl.
*/
com.google.protobuf.ByteString
getFileUrlBytes();
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
boolean hasError();
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The error.
*/
java.lang.String getError();
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The bytes for error.
*/
com.google.protobuf.ByteString
getErrorBytes();
/**
*
* Whether `containing_url` in `FileImportRequest` is unused.
*
* The compiler can cache the `FileImportResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `FileImportResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return The containingUrlUnused.
*/
boolean getContainingUrlUnused();
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.ResultCase getResultCase();
}
/**
*
* A response indicating the result of redirecting a URL to the filesystem.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.FileImportResponse}
*/
public static final class FileImportResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.FileImportResponse)
FileImportResponseOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
FileImportResponse.class.getName());
}
// Use FileImportResponse.newBuilder() to construct.
private FileImportResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private FileImportResponse() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FileImportResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FileImportResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.class, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.Builder.class);
}
private int resultCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object result_;
public enum ResultCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
FILE_URL(2),
ERROR(3),
RESULT_NOT_SET(0);
private final int value;
private ResultCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResultCase valueOf(int value) {
return forNumber(value);
}
public static ResultCase forNumber(int value) {
switch (value) {
case 2: return FILE_URL;
case 3: return ERROR;
case 0: return RESULT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public static final int ID_FIELD_NUMBER = 1;
private int id_ = 0;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
public static final int FILE_URL_FIELD_NUMBER = 2;
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return Whether the fileUrl field is set.
*/
public boolean hasFileUrl() {
return resultCase_ == 2;
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return The fileUrl.
*/
public java.lang.String getFileUrl() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 2) {
result_ = s;
}
return s;
}
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return The bytes for fileUrl.
*/
public com.google.protobuf.ByteString
getFileUrlBytes() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 2) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ERROR_FIELD_NUMBER = 3;
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The error.
*/
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
}
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The bytes for error.
*/
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTAINING_URL_UNUSED_FIELD_NUMBER = 4;
private boolean containingUrlUnused_ = false;
/**
*
* Whether `containing_url` in `FileImportRequest` is unused.
*
* The compiler can cache the `FileImportResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `FileImportResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return The containingUrlUnused.
*/
@java.lang.Override
public boolean getContainingUrlUnused() {
return containingUrlUnused_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0) {
output.writeUInt32(1, id_);
}
if (resultCase_ == 2) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, result_);
}
if (resultCase_ == 3) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, result_);
}
if (containingUrlUnused_ != false) {
output.writeBool(4, containingUrlUnused_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (resultCase_ == 2) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, result_);
}
if (resultCase_ == 3) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, result_);
}
if (containingUrlUnused_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, containingUrlUnused_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse other = (com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) obj;
if (getId()
!= other.getId()) return false;
if (getContainingUrlUnused()
!= other.getContainingUrlUnused()) return false;
if (!getResultCase().equals(other.getResultCase())) return false;
switch (resultCase_) {
case 2:
if (!getFileUrl()
.equals(other.getFileUrl())) return false;
break;
case 3:
if (!getError()
.equals(other.getError())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
hash = (37 * hash) + CONTAINING_URL_UNUSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getContainingUrlUnused());
switch (resultCase_) {
case 2:
hash = (37 * hash) + FILE_URL_FIELD_NUMBER;
hash = (53 * hash) + getFileUrl().hashCode();
break;
case 3:
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A response indicating the result of redirecting a URL to the filesystem.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.FileImportResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.FileImportResponse)
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FileImportResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FileImportResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.class, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = 0;
containingUrlUnused_ = false;
resultCase_ = 0;
result_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FileImportResponse_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse build() {
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse result = new com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.containingUrlUnused_ = containingUrlUnused_;
}
}
private void buildPartialOneofs(com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse result) {
result.resultCase_ = resultCase_;
result.result_ = this.result_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance()) return this;
if (other.getId() != 0) {
setId(other.getId());
}
if (other.getContainingUrlUnused() != false) {
setContainingUrlUnused(other.getContainingUrlUnused());
}
switch (other.getResultCase()) {
case FILE_URL: {
resultCase_ = 2;
result_ = other.result_;
onChanged();
break;
}
case ERROR: {
resultCase_ = 3;
result_ = other.result_;
onChanged();
break;
}
case RESULT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
id_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
java.lang.String s = input.readStringRequireUtf8();
resultCase_ = 2;
result_ = s;
break;
} // case 18
case 26: {
java.lang.String s = input.readStringRequireUtf8();
resultCase_ = 3;
result_ = s;
break;
} // case 26
case 32: {
containingUrlUnused_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int resultCase_ = 0;
private java.lang.Object result_;
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public Builder clearResult() {
resultCase_ = 0;
result_ = null;
onChanged();
return this;
}
private int bitField0_;
private int id_ ;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
* uint32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* uint32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return Whether the fileUrl field is set.
*/
@java.lang.Override
public boolean hasFileUrl() {
return resultCase_ == 2;
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return The fileUrl.
*/
@java.lang.Override
public java.lang.String getFileUrl() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 2) {
result_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return The bytes for fileUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFileUrlBytes() {
java.lang.Object ref = "";
if (resultCase_ == 2) {
ref = result_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 2) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @param value The fileUrl to set.
* @return This builder for chaining.
*/
public Builder setFileUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resultCase_ = 2;
result_ = value;
onChanged();
return this;
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @return This builder for chaining.
*/
public Builder clearFileUrl() {
if (resultCase_ == 2) {
resultCase_ = 0;
result_ = null;
onChanged();
}
return this;
}
/**
*
* The absolute `file:` URL to look for the file on the physical
* filesystem.
*
* The compiler must verify to the best of its ability that this URL
* follows the format for an absolute `file:` URL on the current operating
* system without a hostname. If it doesn't, the compiler must treat that
* as an error thrown by the importer. See
* https://en.wikipedia.org/wiki/File_URI_scheme for details on the
* format.
*
* The compiler must handle turning this into a canonical URL by resolving
* it for partials, file extensions, and index files. The compiler must
* then loading the contents of the resulting canonical URL from the
* filesystem.
*
*
* string file_url = 2;
* @param value The bytes for fileUrl to set.
* @return This builder for chaining.
*/
public Builder setFileUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resultCase_ = 2;
result_ = value;
onChanged();
return this;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
@java.lang.Override
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The error.
*/
@java.lang.Override
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return The bytes for error.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @param value The error to set.
* @return This builder for chaining.
*/
public Builder setError(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @return This builder for chaining.
*/
public Builder clearError() {
if (resultCase_ == 3) {
resultCase_ = 0;
result_ = null;
onChanged();
}
return this;
}
/**
*
* An error message explaining why the URL could not be loaded.
*
*
* string error = 3;
* @param value The bytes for error to set.
* @return This builder for chaining.
*/
public Builder setErrorBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
private boolean containingUrlUnused_ ;
/**
*
* Whether `containing_url` in `FileImportRequest` is unused.
*
* The compiler can cache the `FileImportResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `FileImportResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return The containingUrlUnused.
*/
@java.lang.Override
public boolean getContainingUrlUnused() {
return containingUrlUnused_;
}
/**
*
* Whether `containing_url` in `FileImportRequest` is unused.
*
* The compiler can cache the `FileImportResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `FileImportResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @param value The containingUrlUnused to set.
* @return This builder for chaining.
*/
public Builder setContainingUrlUnused(boolean value) {
containingUrlUnused_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Whether `containing_url` in `FileImportRequest` is unused.
*
* The compiler can cache the `FileImportResponse` if the `containing_url`
* is unused.
*
* The default value is `false`, thus when the value is not set by the host,
* the `FileImportResponse` will not be cached by the compiler.
*
*
* bool containing_url_unused = 4;
* @return This builder for chaining.
*/
public Builder clearContainingUrlUnused() {
bitField0_ = (bitField0_ & ~0x00000008);
containingUrlUnused_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.FileImportResponse)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.FileImportResponse)
private static final com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse();
}
public static com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FileImportResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FunctionCallResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sass.embedded_protocol.InboundMessage.FunctionCallResponse)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 id = 1;
* @return The id.
*/
int getId();
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
* @return Whether the success field is set.
*/
boolean hasSuccess();
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
* @return The success.
*/
com.sass_lang.embedded_protocol.Value getSuccess();
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
com.sass_lang.embedded_protocol.ValueOrBuilder getSuccessOrBuilder();
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
boolean hasError();
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return The error.
*/
java.lang.String getError();
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return The bytes for error.
*/
com.google.protobuf.ByteString
getErrorBytes();
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @return A list containing the accessedArgumentLists.
*/
java.util.List getAccessedArgumentListsList();
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @return The count of accessedArgumentLists.
*/
int getAccessedArgumentListsCount();
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @param index The index of the element to return.
* @return The accessedArgumentLists at the given index.
*/
int getAccessedArgumentLists(int index);
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.ResultCase getResultCase();
}
/**
*
* A response indicating the result of calling a custom Sass function defined
* in the host.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.FunctionCallResponse}
*/
public static final class FunctionCallResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sass.embedded_protocol.InboundMessage.FunctionCallResponse)
FunctionCallResponseOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
FunctionCallResponse.class.getName());
}
// Use FunctionCallResponse.newBuilder() to construct.
private FunctionCallResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private FunctionCallResponse() {
accessedArgumentLists_ = emptyIntList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FunctionCallResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FunctionCallResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.class, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.Builder.class);
}
private int resultCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object result_;
public enum ResultCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
SUCCESS(2),
ERROR(3),
RESULT_NOT_SET(0);
private final int value;
private ResultCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResultCase valueOf(int value) {
return forNumber(value);
}
public static ResultCase forNumber(int value) {
switch (value) {
case 2: return SUCCESS;
case 3: return ERROR;
case 0: return RESULT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public static final int ID_FIELD_NUMBER = 1;
private int id_ = 0;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
public static final int SUCCESS_FIELD_NUMBER = 2;
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
* @return Whether the success field is set.
*/
@java.lang.Override
public boolean hasSuccess() {
return resultCase_ == 2;
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
* @return The success.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.Value getSuccess() {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.Value) result_;
}
return com.sass_lang.embedded_protocol.Value.getDefaultInstance();
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.ValueOrBuilder getSuccessOrBuilder() {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.Value) result_;
}
return com.sass_lang.embedded_protocol.Value.getDefaultInstance();
}
public static final int ERROR_FIELD_NUMBER = 3;
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return The error.
*/
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
}
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return The bytes for error.
*/
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCESSED_ARGUMENT_LISTS_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.IntList accessedArgumentLists_ =
emptyIntList();
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @return A list containing the accessedArgumentLists.
*/
@java.lang.Override
public java.util.List
getAccessedArgumentListsList() {
return accessedArgumentLists_;
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @return The count of accessedArgumentLists.
*/
public int getAccessedArgumentListsCount() {
return accessedArgumentLists_.size();
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @param index The index of the element to return.
* @return The accessedArgumentLists at the given index.
*/
public int getAccessedArgumentLists(int index) {
return accessedArgumentLists_.getInt(index);
}
private int accessedArgumentListsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (id_ != 0) {
output.writeUInt32(1, id_);
}
if (resultCase_ == 2) {
output.writeMessage(2, (com.sass_lang.embedded_protocol.Value) result_);
}
if (resultCase_ == 3) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, result_);
}
if (getAccessedArgumentListsList().size() > 0) {
output.writeUInt32NoTag(34);
output.writeUInt32NoTag(accessedArgumentListsMemoizedSerializedSize);
}
for (int i = 0; i < accessedArgumentLists_.size(); i++) {
output.writeUInt32NoTag(accessedArgumentLists_.getInt(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (resultCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.sass_lang.embedded_protocol.Value) result_);
}
if (resultCase_ == 3) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, result_);
}
{
int dataSize = 0;
for (int i = 0; i < accessedArgumentLists_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(accessedArgumentLists_.getInt(i));
}
size += dataSize;
if (!getAccessedArgumentListsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
accessedArgumentListsMemoizedSerializedSize = dataSize;
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse other = (com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) obj;
if (getId()
!= other.getId()) return false;
if (!getAccessedArgumentListsList()
.equals(other.getAccessedArgumentListsList())) return false;
if (!getResultCase().equals(other.getResultCase())) return false;
switch (resultCase_) {
case 2:
if (!getSuccess()
.equals(other.getSuccess())) return false;
break;
case 3:
if (!getError()
.equals(other.getError())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
if (getAccessedArgumentListsCount() > 0) {
hash = (37 * hash) + ACCESSED_ARGUMENT_LISTS_FIELD_NUMBER;
hash = (53 * hash) + getAccessedArgumentListsList().hashCode();
}
switch (resultCase_) {
case 2:
hash = (37 * hash) + SUCCESS_FIELD_NUMBER;
hash = (53 * hash) + getSuccess().hashCode();
break;
case 3:
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A response indicating the result of calling a custom Sass function defined
* in the host.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage.FunctionCallResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage.FunctionCallResponse)
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FunctionCallResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FunctionCallResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.class, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = 0;
if (successBuilder_ != null) {
successBuilder_.clear();
}
accessedArgumentLists_ = emptyIntList();
resultCase_ = 0;
result_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_FunctionCallResponse_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse build() {
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse result = new com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
accessedArgumentLists_.makeImmutable();
result.accessedArgumentLists_ = accessedArgumentLists_;
}
}
private void buildPartialOneofs(com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse result) {
result.resultCase_ = resultCase_;
result.result_ = this.result_;
if (resultCase_ == 2 &&
successBuilder_ != null) {
result.result_ = successBuilder_.build();
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance()) return this;
if (other.getId() != 0) {
setId(other.getId());
}
if (!other.accessedArgumentLists_.isEmpty()) {
if (accessedArgumentLists_.isEmpty()) {
accessedArgumentLists_ = other.accessedArgumentLists_;
accessedArgumentLists_.makeImmutable();
bitField0_ |= 0x00000008;
} else {
ensureAccessedArgumentListsIsMutable();
accessedArgumentLists_.addAll(other.accessedArgumentLists_);
}
onChanged();
}
switch (other.getResultCase()) {
case SUCCESS: {
mergeSuccess(other.getSuccess());
break;
}
case ERROR: {
resultCase_ = 3;
result_ = other.result_;
onChanged();
break;
}
case RESULT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
id_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getSuccessFieldBuilder().getBuilder(),
extensionRegistry);
resultCase_ = 2;
break;
} // case 18
case 26: {
java.lang.String s = input.readStringRequireUtf8();
resultCase_ = 3;
result_ = s;
break;
} // case 26
case 32: {
int v = input.readUInt32();
ensureAccessedArgumentListsIsMutable();
accessedArgumentLists_.addInt(v);
break;
} // case 32
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureAccessedArgumentListsIsMutable();
while (input.getBytesUntilLimit() > 0) {
accessedArgumentLists_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int resultCase_ = 0;
private java.lang.Object result_;
public ResultCase
getResultCase() {
return ResultCase.forNumber(
resultCase_);
}
public Builder clearResult() {
resultCase_ = 0;
result_ = null;
onChanged();
return this;
}
private int bitField0_;
private int id_ ;
/**
* uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
* uint32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* uint32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.Value, com.sass_lang.embedded_protocol.Value.Builder, com.sass_lang.embedded_protocol.ValueOrBuilder> successBuilder_;
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
* @return Whether the success field is set.
*/
@java.lang.Override
public boolean hasSuccess() {
return resultCase_ == 2;
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
* @return The success.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.Value getSuccess() {
if (successBuilder_ == null) {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.Value) result_;
}
return com.sass_lang.embedded_protocol.Value.getDefaultInstance();
} else {
if (resultCase_ == 2) {
return successBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.Value.getDefaultInstance();
}
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
public Builder setSuccess(com.sass_lang.embedded_protocol.Value value) {
if (successBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
result_ = value;
onChanged();
} else {
successBuilder_.setMessage(value);
}
resultCase_ = 2;
return this;
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
public Builder setSuccess(
com.sass_lang.embedded_protocol.Value.Builder builderForValue) {
if (successBuilder_ == null) {
result_ = builderForValue.build();
onChanged();
} else {
successBuilder_.setMessage(builderForValue.build());
}
resultCase_ = 2;
return this;
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
public Builder mergeSuccess(com.sass_lang.embedded_protocol.Value value) {
if (successBuilder_ == null) {
if (resultCase_ == 2 &&
result_ != com.sass_lang.embedded_protocol.Value.getDefaultInstance()) {
result_ = com.sass_lang.embedded_protocol.Value.newBuilder((com.sass_lang.embedded_protocol.Value) result_)
.mergeFrom(value).buildPartial();
} else {
result_ = value;
}
onChanged();
} else {
if (resultCase_ == 2) {
successBuilder_.mergeFrom(value);
} else {
successBuilder_.setMessage(value);
}
}
resultCase_ = 2;
return this;
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
public Builder clearSuccess() {
if (successBuilder_ == null) {
if (resultCase_ == 2) {
resultCase_ = 0;
result_ = null;
onChanged();
}
} else {
if (resultCase_ == 2) {
resultCase_ = 0;
result_ = null;
}
successBuilder_.clear();
}
return this;
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
public com.sass_lang.embedded_protocol.Value.Builder getSuccessBuilder() {
return getSuccessFieldBuilder().getBuilder();
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.ValueOrBuilder getSuccessOrBuilder() {
if ((resultCase_ == 2) && (successBuilder_ != null)) {
return successBuilder_.getMessageOrBuilder();
} else {
if (resultCase_ == 2) {
return (com.sass_lang.embedded_protocol.Value) result_;
}
return com.sass_lang.embedded_protocol.Value.getDefaultInstance();
}
}
/**
*
* The return value of a successful function call.
*
*
* .sass.embedded_protocol.Value success = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.Value, com.sass_lang.embedded_protocol.Value.Builder, com.sass_lang.embedded_protocol.ValueOrBuilder>
getSuccessFieldBuilder() {
if (successBuilder_ == null) {
if (!(resultCase_ == 2)) {
result_ = com.sass_lang.embedded_protocol.Value.getDefaultInstance();
}
successBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.Value, com.sass_lang.embedded_protocol.Value.Builder, com.sass_lang.embedded_protocol.ValueOrBuilder>(
(com.sass_lang.embedded_protocol.Value) result_,
getParentForChildren(),
isClean());
result_ = null;
}
resultCase_ = 2;
onChanged();
return successBuilder_;
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return Whether the error field is set.
*/
@java.lang.Override
public boolean hasError() {
return resultCase_ == 3;
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return The error.
*/
@java.lang.Override
public java.lang.String getError() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (resultCase_ == 3) {
result_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return The bytes for error.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorBytes() {
java.lang.Object ref = "";
if (resultCase_ == 3) {
ref = result_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (resultCase_ == 3) {
result_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @param value The error to set.
* @return This builder for chaining.
*/
public Builder setError(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @return This builder for chaining.
*/
public Builder clearError() {
if (resultCase_ == 3) {
resultCase_ = 0;
result_ = null;
onChanged();
}
return this;
}
/**
*
* An error message explaining why the function call failed.
*
*
* string error = 3;
* @param value The bytes for error to set.
* @return This builder for chaining.
*/
public Builder setErrorBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resultCase_ = 3;
result_ = value;
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList accessedArgumentLists_ = emptyIntList();
private void ensureAccessedArgumentListsIsMutable() {
if (!accessedArgumentLists_.isModifiable()) {
accessedArgumentLists_ = makeMutableCopy(accessedArgumentLists_);
}
bitField0_ |= 0x00000008;
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @return A list containing the accessedArgumentLists.
*/
public java.util.List
getAccessedArgumentListsList() {
accessedArgumentLists_.makeImmutable();
return accessedArgumentLists_;
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @return The count of accessedArgumentLists.
*/
public int getAccessedArgumentListsCount() {
return accessedArgumentLists_.size();
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @param index The index of the element to return.
* @return The accessedArgumentLists at the given index.
*/
public int getAccessedArgumentLists(int index) {
return accessedArgumentLists_.getInt(index);
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @param index The index to set the value at.
* @param value The accessedArgumentLists to set.
* @return This builder for chaining.
*/
public Builder setAccessedArgumentLists(
int index, int value) {
ensureAccessedArgumentListsIsMutable();
accessedArgumentLists_.setInt(index, value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @param value The accessedArgumentLists to add.
* @return This builder for chaining.
*/
public Builder addAccessedArgumentLists(int value) {
ensureAccessedArgumentListsIsMutable();
accessedArgumentLists_.addInt(value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @param values The accessedArgumentLists to add.
* @return This builder for chaining.
*/
public Builder addAllAccessedArgumentLists(
java.lang.Iterable extends java.lang.Integer> values) {
ensureAccessedArgumentListsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, accessedArgumentLists_);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The IDs of all `Value.ArgumentList`s in `FunctionCallRequest.arguments`
* whose keywords were accessed. See `Value.ArgumentList` for details. This
* may not include the special value `0` and it may not include multiple
* instances of the same ID.
*
*
* repeated uint32 accessed_argument_lists = 4;
* @return This builder for chaining.
*/
public Builder clearAccessedArgumentLists() {
accessedArgumentLists_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage.FunctionCallResponse)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage.FunctionCallResponse)
private static final com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse();
}
public static com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FunctionCallResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int messageCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object message_;
public enum MessageCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
COMPILE_REQUEST(2),
CANONICALIZE_RESPONSE(3),
IMPORT_RESPONSE(4),
FILE_IMPORT_RESPONSE(5),
FUNCTION_CALL_RESPONSE(6),
VERSION_REQUEST(7),
MESSAGE_NOT_SET(0);
private final int value;
private MessageCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MessageCase valueOf(int value) {
return forNumber(value);
}
public static MessageCase forNumber(int value) {
switch (value) {
case 2: return COMPILE_REQUEST;
case 3: return CANONICALIZE_RESPONSE;
case 4: return IMPORT_RESPONSE;
case 5: return FILE_IMPORT_RESPONSE;
case 6: return FUNCTION_CALL_RESPONSE;
case 7: return VERSION_REQUEST;
case 0: return MESSAGE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public MessageCase
getMessageCase() {
return MessageCase.forNumber(
messageCase_);
}
public static final int COMPILE_REQUEST_FIELD_NUMBER = 2;
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
* @return Whether the compileRequest field is set.
*/
@java.lang.Override
public boolean hasCompileRequest() {
return messageCase_ == 2;
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
* @return The compileRequest.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest getCompileRequest() {
if (messageCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance();
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequestOrBuilder getCompileRequestOrBuilder() {
if (messageCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance();
}
public static final int CANONICALIZE_RESPONSE_FIELD_NUMBER = 3;
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
* @return Whether the canonicalizeResponse field is set.
*/
@java.lang.Override
public boolean hasCanonicalizeResponse() {
return messageCase_ == 3;
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
* @return The canonicalizeResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse getCanonicalizeResponse() {
if (messageCase_ == 3) {
return (com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance();
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponseOrBuilder getCanonicalizeResponseOrBuilder() {
if (messageCase_ == 3) {
return (com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance();
}
public static final int IMPORT_RESPONSE_FIELD_NUMBER = 4;
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
* @return Whether the importResponse field is set.
*/
@java.lang.Override
public boolean hasImportResponse() {
return messageCase_ == 4;
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
* @return The importResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse getImportResponse() {
if (messageCase_ == 4) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance();
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponseOrBuilder getImportResponseOrBuilder() {
if (messageCase_ == 4) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance();
}
public static final int FILE_IMPORT_RESPONSE_FIELD_NUMBER = 5;
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
* @return Whether the fileImportResponse field is set.
*/
@java.lang.Override
public boolean hasFileImportResponse() {
return messageCase_ == 5;
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
* @return The fileImportResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse getFileImportResponse() {
if (messageCase_ == 5) {
return (com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance();
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponseOrBuilder getFileImportResponseOrBuilder() {
if (messageCase_ == 5) {
return (com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance();
}
public static final int FUNCTION_CALL_RESPONSE_FIELD_NUMBER = 6;
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
* @return Whether the functionCallResponse field is set.
*/
@java.lang.Override
public boolean hasFunctionCallResponse() {
return messageCase_ == 6;
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
* @return The functionCallResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse getFunctionCallResponse() {
if (messageCase_ == 6) {
return (com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance();
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponseOrBuilder getFunctionCallResponseOrBuilder() {
if (messageCase_ == 6) {
return (com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance();
}
public static final int VERSION_REQUEST_FIELD_NUMBER = 7;
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
* @return Whether the versionRequest field is set.
*/
@java.lang.Override
public boolean hasVersionRequest() {
return messageCase_ == 7;
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
* @return The versionRequest.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequest getVersionRequest() {
if (messageCase_ == 7) {
return (com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance();
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequestOrBuilder getVersionRequestOrBuilder() {
if (messageCase_ == 7) {
return (com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (messageCase_ == 2) {
output.writeMessage(2, (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_);
}
if (messageCase_ == 3) {
output.writeMessage(3, (com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_);
}
if (messageCase_ == 4) {
output.writeMessage(4, (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_);
}
if (messageCase_ == 5) {
output.writeMessage(5, (com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_);
}
if (messageCase_ == 6) {
output.writeMessage(6, (com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_);
}
if (messageCase_ == 7) {
output.writeMessage(7, (com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (messageCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_);
}
if (messageCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_);
}
if (messageCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_);
}
if (messageCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_);
}
if (messageCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_);
}
if (messageCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sass_lang.embedded_protocol.InboundMessage)) {
return super.equals(obj);
}
com.sass_lang.embedded_protocol.InboundMessage other = (com.sass_lang.embedded_protocol.InboundMessage) obj;
if (!getMessageCase().equals(other.getMessageCase())) return false;
switch (messageCase_) {
case 2:
if (!getCompileRequest()
.equals(other.getCompileRequest())) return false;
break;
case 3:
if (!getCanonicalizeResponse()
.equals(other.getCanonicalizeResponse())) return false;
break;
case 4:
if (!getImportResponse()
.equals(other.getImportResponse())) return false;
break;
case 5:
if (!getFileImportResponse()
.equals(other.getFileImportResponse())) return false;
break;
case 6:
if (!getFunctionCallResponse()
.equals(other.getFunctionCallResponse())) return false;
break;
case 7:
if (!getVersionRequest()
.equals(other.getVersionRequest())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (messageCase_) {
case 2:
hash = (37 * hash) + COMPILE_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getCompileRequest().hashCode();
break;
case 3:
hash = (37 * hash) + CANONICALIZE_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getCanonicalizeResponse().hashCode();
break;
case 4:
hash = (37 * hash) + IMPORT_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getImportResponse().hashCode();
break;
case 5:
hash = (37 * hash) + FILE_IMPORT_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getFileImportResponse().hashCode();
break;
case 6:
hash = (37 * hash) + FUNCTION_CALL_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getFunctionCallResponse().hashCode();
break;
case 7:
hash = (37 * hash) + VERSION_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getVersionRequest().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.sass_lang.embedded_protocol.InboundMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sass_lang.embedded_protocol.InboundMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The wrapper type for all messages sent from the host to the compiler. This
* provides a `oneof` that makes it possible to determine the type of each
* inbound message.
*
*
* Protobuf type {@code sass.embedded_protocol.InboundMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sass.embedded_protocol.InboundMessage)
com.sass_lang.embedded_protocol.InboundMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sass_lang.embedded_protocol.InboundMessage.class, com.sass_lang.embedded_protocol.InboundMessage.Builder.class);
}
// Construct using com.sass_lang.embedded_protocol.InboundMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (compileRequestBuilder_ != null) {
compileRequestBuilder_.clear();
}
if (canonicalizeResponseBuilder_ != null) {
canonicalizeResponseBuilder_.clear();
}
if (importResponseBuilder_ != null) {
importResponseBuilder_.clear();
}
if (fileImportResponseBuilder_ != null) {
fileImportResponseBuilder_.clear();
}
if (functionCallResponseBuilder_ != null) {
functionCallResponseBuilder_.clear();
}
if (versionRequestBuilder_ != null) {
versionRequestBuilder_.clear();
}
messageCase_ = 0;
message_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sass_lang.embedded_protocol.EmbeddedSass.internal_static_sass_embedded_protocol_InboundMessage_descriptor;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage getDefaultInstanceForType() {
return com.sass_lang.embedded_protocol.InboundMessage.getDefaultInstance();
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage build() {
com.sass_lang.embedded_protocol.InboundMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage buildPartial() {
com.sass_lang.embedded_protocol.InboundMessage result = new com.sass_lang.embedded_protocol.InboundMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.sass_lang.embedded_protocol.InboundMessage result) {
int from_bitField0_ = bitField0_;
}
private void buildPartialOneofs(com.sass_lang.embedded_protocol.InboundMessage result) {
result.messageCase_ = messageCase_;
result.message_ = this.message_;
if (messageCase_ == 2 &&
compileRequestBuilder_ != null) {
result.message_ = compileRequestBuilder_.build();
}
if (messageCase_ == 3 &&
canonicalizeResponseBuilder_ != null) {
result.message_ = canonicalizeResponseBuilder_.build();
}
if (messageCase_ == 4 &&
importResponseBuilder_ != null) {
result.message_ = importResponseBuilder_.build();
}
if (messageCase_ == 5 &&
fileImportResponseBuilder_ != null) {
result.message_ = fileImportResponseBuilder_.build();
}
if (messageCase_ == 6 &&
functionCallResponseBuilder_ != null) {
result.message_ = functionCallResponseBuilder_.build();
}
if (messageCase_ == 7 &&
versionRequestBuilder_ != null) {
result.message_ = versionRequestBuilder_.build();
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sass_lang.embedded_protocol.InboundMessage) {
return mergeFrom((com.sass_lang.embedded_protocol.InboundMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sass_lang.embedded_protocol.InboundMessage other) {
if (other == com.sass_lang.embedded_protocol.InboundMessage.getDefaultInstance()) return this;
switch (other.getMessageCase()) {
case COMPILE_REQUEST: {
mergeCompileRequest(other.getCompileRequest());
break;
}
case CANONICALIZE_RESPONSE: {
mergeCanonicalizeResponse(other.getCanonicalizeResponse());
break;
}
case IMPORT_RESPONSE: {
mergeImportResponse(other.getImportResponse());
break;
}
case FILE_IMPORT_RESPONSE: {
mergeFileImportResponse(other.getFileImportResponse());
break;
}
case FUNCTION_CALL_RESPONSE: {
mergeFunctionCallResponse(other.getFunctionCallResponse());
break;
}
case VERSION_REQUEST: {
mergeVersionRequest(other.getVersionRequest());
break;
}
case MESSAGE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
input.readMessage(
getCompileRequestFieldBuilder().getBuilder(),
extensionRegistry);
messageCase_ = 2;
break;
} // case 18
case 26: {
input.readMessage(
getCanonicalizeResponseFieldBuilder().getBuilder(),
extensionRegistry);
messageCase_ = 3;
break;
} // case 26
case 34: {
input.readMessage(
getImportResponseFieldBuilder().getBuilder(),
extensionRegistry);
messageCase_ = 4;
break;
} // case 34
case 42: {
input.readMessage(
getFileImportResponseFieldBuilder().getBuilder(),
extensionRegistry);
messageCase_ = 5;
break;
} // case 42
case 50: {
input.readMessage(
getFunctionCallResponseFieldBuilder().getBuilder(),
extensionRegistry);
messageCase_ = 6;
break;
} // case 50
case 58: {
input.readMessage(
getVersionRequestFieldBuilder().getBuilder(),
extensionRegistry);
messageCase_ = 7;
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int messageCase_ = 0;
private java.lang.Object message_;
public MessageCase
getMessageCase() {
return MessageCase.forNumber(
messageCase_);
}
public Builder clearMessage() {
messageCase_ = 0;
message_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequestOrBuilder> compileRequestBuilder_;
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
* @return Whether the compileRequest field is set.
*/
@java.lang.Override
public boolean hasCompileRequest() {
return messageCase_ == 2;
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
* @return The compileRequest.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest getCompileRequest() {
if (compileRequestBuilder_ == null) {
if (messageCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance();
} else {
if (messageCase_ == 2) {
return compileRequestBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
public Builder setCompileRequest(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest value) {
if (compileRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
compileRequestBuilder_.setMessage(value);
}
messageCase_ = 2;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
public Builder setCompileRequest(
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Builder builderForValue) {
if (compileRequestBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
compileRequestBuilder_.setMessage(builderForValue.build());
}
messageCase_ = 2;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
public Builder mergeCompileRequest(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest value) {
if (compileRequestBuilder_ == null) {
if (messageCase_ == 2 &&
message_ != com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance()) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_)
.mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
if (messageCase_ == 2) {
compileRequestBuilder_.mergeFrom(value);
} else {
compileRequestBuilder_.setMessage(value);
}
}
messageCase_ = 2;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
public Builder clearCompileRequest() {
if (compileRequestBuilder_ == null) {
if (messageCase_ == 2) {
messageCase_ = 0;
message_ = null;
onChanged();
}
} else {
if (messageCase_ == 2) {
messageCase_ = 0;
message_ = null;
}
compileRequestBuilder_.clear();
}
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Builder getCompileRequestBuilder() {
return getCompileRequestFieldBuilder().getBuilder();
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CompileRequestOrBuilder getCompileRequestOrBuilder() {
if ((messageCase_ == 2) && (compileRequestBuilder_ != null)) {
return compileRequestBuilder_.getMessageOrBuilder();
} else {
if (messageCase_ == 2) {
return (com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.CompileRequest compile_request = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequestOrBuilder>
getCompileRequestFieldBuilder() {
if (compileRequestBuilder_ == null) {
if (!(messageCase_ == 2)) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.getDefaultInstance();
}
compileRequestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CompileRequest, com.sass_lang.embedded_protocol.InboundMessage.CompileRequest.Builder, com.sass_lang.embedded_protocol.InboundMessage.CompileRequestOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.CompileRequest) message_,
getParentForChildren(),
isClean());
message_ = null;
}
messageCase_ = 2;
onChanged();
return compileRequestBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponseOrBuilder> canonicalizeResponseBuilder_;
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
* @return Whether the canonicalizeResponse field is set.
*/
@java.lang.Override
public boolean hasCanonicalizeResponse() {
return messageCase_ == 3;
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
* @return The canonicalizeResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse getCanonicalizeResponse() {
if (canonicalizeResponseBuilder_ == null) {
if (messageCase_ == 3) {
return (com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance();
} else {
if (messageCase_ == 3) {
return canonicalizeResponseBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
public Builder setCanonicalizeResponse(com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse value) {
if (canonicalizeResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
canonicalizeResponseBuilder_.setMessage(value);
}
messageCase_ = 3;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
public Builder setCanonicalizeResponse(
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.Builder builderForValue) {
if (canonicalizeResponseBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
canonicalizeResponseBuilder_.setMessage(builderForValue.build());
}
messageCase_ = 3;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
public Builder mergeCanonicalizeResponse(com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse value) {
if (canonicalizeResponseBuilder_ == null) {
if (messageCase_ == 3 &&
message_ != com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance()) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_)
.mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
if (messageCase_ == 3) {
canonicalizeResponseBuilder_.mergeFrom(value);
} else {
canonicalizeResponseBuilder_.setMessage(value);
}
}
messageCase_ = 3;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
public Builder clearCanonicalizeResponse() {
if (canonicalizeResponseBuilder_ == null) {
if (messageCase_ == 3) {
messageCase_ = 0;
message_ = null;
onChanged();
}
} else {
if (messageCase_ == 3) {
messageCase_ = 0;
message_ = null;
}
canonicalizeResponseBuilder_.clear();
}
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.Builder getCanonicalizeResponseBuilder() {
return getCanonicalizeResponseFieldBuilder().getBuilder();
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponseOrBuilder getCanonicalizeResponseOrBuilder() {
if ((messageCase_ == 3) && (canonicalizeResponseBuilder_ != null)) {
return canonicalizeResponseBuilder_.getMessageOrBuilder();
} else {
if (messageCase_ == 3) {
return (com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.CanonicalizeResponse canonicalize_response = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponseOrBuilder>
getCanonicalizeResponseFieldBuilder() {
if (canonicalizeResponseBuilder_ == null) {
if (!(messageCase_ == 3)) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.getDefaultInstance();
}
canonicalizeResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponseOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.CanonicalizeResponse) message_,
getParentForChildren(),
isClean());
message_ = null;
}
messageCase_ = 3;
onChanged();
return canonicalizeResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.ImportResponseOrBuilder> importResponseBuilder_;
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
* @return Whether the importResponse field is set.
*/
@java.lang.Override
public boolean hasImportResponse() {
return messageCase_ == 4;
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
* @return The importResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse getImportResponse() {
if (importResponseBuilder_ == null) {
if (messageCase_ == 4) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance();
} else {
if (messageCase_ == 4) {
return importResponseBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
public Builder setImportResponse(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse value) {
if (importResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
importResponseBuilder_.setMessage(value);
}
messageCase_ = 4;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
public Builder setImportResponse(
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.Builder builderForValue) {
if (importResponseBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
importResponseBuilder_.setMessage(builderForValue.build());
}
messageCase_ = 4;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
public Builder mergeImportResponse(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse value) {
if (importResponseBuilder_ == null) {
if (messageCase_ == 4 &&
message_ != com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance()) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_)
.mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
if (messageCase_ == 4) {
importResponseBuilder_.mergeFrom(value);
} else {
importResponseBuilder_.setMessage(value);
}
}
messageCase_ = 4;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
public Builder clearImportResponse() {
if (importResponseBuilder_ == null) {
if (messageCase_ == 4) {
messageCase_ = 0;
message_ = null;
onChanged();
}
} else {
if (messageCase_ == 4) {
messageCase_ = 0;
message_ = null;
}
importResponseBuilder_.clear();
}
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.Builder getImportResponseBuilder() {
return getImportResponseFieldBuilder().getBuilder();
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.ImportResponseOrBuilder getImportResponseOrBuilder() {
if ((messageCase_ == 4) && (importResponseBuilder_ != null)) {
return importResponseBuilder_.getMessageOrBuilder();
} else {
if (messageCase_ == 4) {
return (com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.ImportResponse import_response = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.ImportResponseOrBuilder>
getImportResponseFieldBuilder() {
if (importResponseBuilder_ == null) {
if (!(messageCase_ == 4)) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.getDefaultInstance();
}
importResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.ImportResponse, com.sass_lang.embedded_protocol.InboundMessage.ImportResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.ImportResponseOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.ImportResponse) message_,
getParentForChildren(),
isClean());
message_ = null;
}
messageCase_ = 4;
onChanged();
return importResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponseOrBuilder> fileImportResponseBuilder_;
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
* @return Whether the fileImportResponse field is set.
*/
@java.lang.Override
public boolean hasFileImportResponse() {
return messageCase_ == 5;
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
* @return The fileImportResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse getFileImportResponse() {
if (fileImportResponseBuilder_ == null) {
if (messageCase_ == 5) {
return (com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance();
} else {
if (messageCase_ == 5) {
return fileImportResponseBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
public Builder setFileImportResponse(com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse value) {
if (fileImportResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
fileImportResponseBuilder_.setMessage(value);
}
messageCase_ = 5;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
public Builder setFileImportResponse(
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.Builder builderForValue) {
if (fileImportResponseBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
fileImportResponseBuilder_.setMessage(builderForValue.build());
}
messageCase_ = 5;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
public Builder mergeFileImportResponse(com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse value) {
if (fileImportResponseBuilder_ == null) {
if (messageCase_ == 5 &&
message_ != com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance()) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_)
.mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
if (messageCase_ == 5) {
fileImportResponseBuilder_.mergeFrom(value);
} else {
fileImportResponseBuilder_.setMessage(value);
}
}
messageCase_ = 5;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
public Builder clearFileImportResponse() {
if (fileImportResponseBuilder_ == null) {
if (messageCase_ == 5) {
messageCase_ = 0;
message_ = null;
onChanged();
}
} else {
if (messageCase_ == 5) {
messageCase_ = 0;
message_ = null;
}
fileImportResponseBuilder_.clear();
}
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.Builder getFileImportResponseBuilder() {
return getFileImportResponseFieldBuilder().getBuilder();
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FileImportResponseOrBuilder getFileImportResponseOrBuilder() {
if ((messageCase_ == 5) && (fileImportResponseBuilder_ != null)) {
return fileImportResponseBuilder_.getMessageOrBuilder();
} else {
if (messageCase_ == 5) {
return (com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.FileImportResponse file_import_response = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponseOrBuilder>
getFileImportResponseFieldBuilder() {
if (fileImportResponseBuilder_ == null) {
if (!(messageCase_ == 5)) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.getDefaultInstance();
}
fileImportResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.FileImportResponseOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.FileImportResponse) message_,
getParentForChildren(),
isClean());
message_ = null;
}
messageCase_ = 5;
onChanged();
return fileImportResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponseOrBuilder> functionCallResponseBuilder_;
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
* @return Whether the functionCallResponse field is set.
*/
@java.lang.Override
public boolean hasFunctionCallResponse() {
return messageCase_ == 6;
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
* @return The functionCallResponse.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse getFunctionCallResponse() {
if (functionCallResponseBuilder_ == null) {
if (messageCase_ == 6) {
return (com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance();
} else {
if (messageCase_ == 6) {
return functionCallResponseBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
public Builder setFunctionCallResponse(com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse value) {
if (functionCallResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
functionCallResponseBuilder_.setMessage(value);
}
messageCase_ = 6;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
public Builder setFunctionCallResponse(
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.Builder builderForValue) {
if (functionCallResponseBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
functionCallResponseBuilder_.setMessage(builderForValue.build());
}
messageCase_ = 6;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
public Builder mergeFunctionCallResponse(com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse value) {
if (functionCallResponseBuilder_ == null) {
if (messageCase_ == 6 &&
message_ != com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance()) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_)
.mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
if (messageCase_ == 6) {
functionCallResponseBuilder_.mergeFrom(value);
} else {
functionCallResponseBuilder_.setMessage(value);
}
}
messageCase_ = 6;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
public Builder clearFunctionCallResponse() {
if (functionCallResponseBuilder_ == null) {
if (messageCase_ == 6) {
messageCase_ = 0;
message_ = null;
onChanged();
}
} else {
if (messageCase_ == 6) {
messageCase_ = 0;
message_ = null;
}
functionCallResponseBuilder_.clear();
}
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.Builder getFunctionCallResponseBuilder() {
return getFunctionCallResponseFieldBuilder().getBuilder();
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponseOrBuilder getFunctionCallResponseOrBuilder() {
if ((messageCase_ == 6) && (functionCallResponseBuilder_ != null)) {
return functionCallResponseBuilder_.getMessageOrBuilder();
} else {
if (messageCase_ == 6) {
return (com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.FunctionCallResponse function_call_response = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponseOrBuilder>
getFunctionCallResponseFieldBuilder() {
if (functionCallResponseBuilder_ == null) {
if (!(messageCase_ == 6)) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.getDefaultInstance();
}
functionCallResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse.Builder, com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponseOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.FunctionCallResponse) message_,
getParentForChildren(),
isClean());
message_ = null;
}
messageCase_ = 6;
onChanged();
return functionCallResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest, com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.Builder, com.sass_lang.embedded_protocol.InboundMessage.VersionRequestOrBuilder> versionRequestBuilder_;
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
* @return Whether the versionRequest field is set.
*/
@java.lang.Override
public boolean hasVersionRequest() {
return messageCase_ == 7;
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
* @return The versionRequest.
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequest getVersionRequest() {
if (versionRequestBuilder_ == null) {
if (messageCase_ == 7) {
return (com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance();
} else {
if (messageCase_ == 7) {
return versionRequestBuilder_.getMessage();
}
return com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
public Builder setVersionRequest(com.sass_lang.embedded_protocol.InboundMessage.VersionRequest value) {
if (versionRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
versionRequestBuilder_.setMessage(value);
}
messageCase_ = 7;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
public Builder setVersionRequest(
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.Builder builderForValue) {
if (versionRequestBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
versionRequestBuilder_.setMessage(builderForValue.build());
}
messageCase_ = 7;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
public Builder mergeVersionRequest(com.sass_lang.embedded_protocol.InboundMessage.VersionRequest value) {
if (versionRequestBuilder_ == null) {
if (messageCase_ == 7 &&
message_ != com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance()) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.newBuilder((com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_)
.mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
if (messageCase_ == 7) {
versionRequestBuilder_.mergeFrom(value);
} else {
versionRequestBuilder_.setMessage(value);
}
}
messageCase_ = 7;
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
public Builder clearVersionRequest() {
if (versionRequestBuilder_ == null) {
if (messageCase_ == 7) {
messageCase_ = 0;
message_ = null;
onChanged();
}
} else {
if (messageCase_ == 7) {
messageCase_ = 0;
message_ = null;
}
versionRequestBuilder_.clear();
}
return this;
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.Builder getVersionRequestBuilder() {
return getVersionRequestFieldBuilder().getBuilder();
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage.VersionRequestOrBuilder getVersionRequestOrBuilder() {
if ((messageCase_ == 7) && (versionRequestBuilder_ != null)) {
return versionRequestBuilder_.getMessageOrBuilder();
} else {
if (messageCase_ == 7) {
return (com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_;
}
return com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance();
}
}
/**
* .sass.embedded_protocol.InboundMessage.VersionRequest version_request = 7;
*/
private com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest, com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.Builder, com.sass_lang.embedded_protocol.InboundMessage.VersionRequestOrBuilder>
getVersionRequestFieldBuilder() {
if (versionRequestBuilder_ == null) {
if (!(messageCase_ == 7)) {
message_ = com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.getDefaultInstance();
}
versionRequestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.sass_lang.embedded_protocol.InboundMessage.VersionRequest, com.sass_lang.embedded_protocol.InboundMessage.VersionRequest.Builder, com.sass_lang.embedded_protocol.InboundMessage.VersionRequestOrBuilder>(
(com.sass_lang.embedded_protocol.InboundMessage.VersionRequest) message_,
getParentForChildren(),
isClean());
message_ = null;
}
messageCase_ = 7;
onChanged();
return versionRequestBuilder_;
}
// @@protoc_insertion_point(builder_scope:sass.embedded_protocol.InboundMessage)
}
// @@protoc_insertion_point(class_scope:sass.embedded_protocol.InboundMessage)
private static final com.sass_lang.embedded_protocol.InboundMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sass_lang.embedded_protocol.InboundMessage();
}
public static com.sass_lang.embedded_protocol.InboundMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InboundMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sass_lang.embedded_protocol.InboundMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy