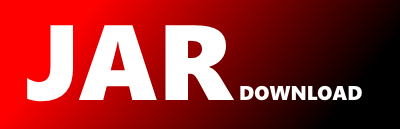
de.learnlib.algorithms.adt.learner.ADTLearner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-adt Show documentation
Show all versions of learnlib-adt Show documentation
This artifact provides the implementation of the ADT learning algorithm as described in the Master thesis
"Active Automata Learning with Adaptive Distinguishing Sequences" (http://arxiv.org/abs/1902.01139) by Markus
Frohme.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy