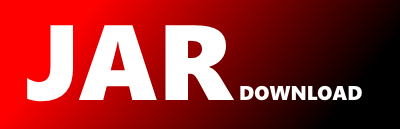
de.learnlib.eqtests.basic.SimulatorEQOracle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-basic-eqtests Show documentation
Show all versions of learnlib-basic-eqtests Show documentation
A collection of basic algorithm for approximating equivalence queries
/* Copyright (C) 2013 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* LearnLib is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License version 3.0 as published by the Free Software Foundation.
*
* LearnLib is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with LearnLib; if not, see
* .
*/
package de.learnlib.eqtests.basic;
import java.util.Collection;
import de.learnlib.api.EquivalenceOracle;
import de.learnlib.oracles.DefaultQuery;
import net.automatalib.automata.UniversalDeterministicAutomaton;
import net.automatalib.automata.concepts.Output;
import net.automatalib.automata.fsa.DFA;
import net.automatalib.automata.transout.MealyMachine;
import net.automatalib.util.automata.Automata;
import net.automatalib.words.Word;
public class SimulatorEQOracle
implements EquivalenceOracle, I, D> {
public static class DFASimulatorEQOracle implements DFAEquivalenceOracle {
private final SimulatorEQOracle delegate;
public DFASimulatorEQOracle(DFA,I> dfa) {
this.delegate = new SimulatorEQOracle<>(dfa);
}
@Override
public DefaultQuery findCounterExample(
DFA, I> hypothesis, Collection extends I> inputs) {
return delegate.findCounterExample(hypothesis, inputs);
}
}
public static class MealySimulatorEQOracle implements MealyEquivalenceOracle {
private final SimulatorEQOracle> delegate;
public MealySimulatorEQOracle(MealyMachine, I, ?, O> mealy) {
this.delegate = new SimulatorEQOracle<>(mealy);
}
@Override
public DefaultQuery> findCounterExample(
MealyMachine, I, ?, O> hypothesis,
Collection extends I> inputs) {
return delegate.findCounterExample(hypothesis, inputs);
}
}
private final UniversalDeterministicAutomaton, I, ?, ?, ?> reference;
private final Output output;
public & Output>
SimulatorEQOracle(R reference) {
this.reference = reference;
this.output = reference;
}
@Override
public DefaultQuery findCounterExample(UniversalDeterministicAutomaton, I, ?, ?, ?> hypothesis, Collection extends I> inputs) {
Word sep = Automata.findSeparatingWord(reference, hypothesis, inputs);
if(sep == null) {
return null;
}
D out = output.computeOutput(sep);
DefaultQuery qry = new DefaultQuery<>(sep);
qry.answer(out);
return qry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy