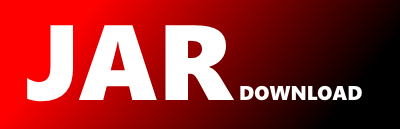
de.learnlib.algorithms.lstargeneric.closing.ClosingStrategies Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-lstar-generic Show documentation
Show all versions of learnlib-lstar-generic Show documentation
A flexible, optimized version of Dana Angluin's L* algorithm
/* Copyright (C) 2013 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* LearnLib is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License version 3.0 as published by the Free Software Foundation.
*
* LearnLib is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with LearnLib; if not, see
* .
*/
package de.learnlib.algorithms.lstargeneric.closing;
import java.util.ArrayList;
import java.util.List;
import net.automatalib.commons.util.comparison.CmpUtil;
import net.automatalib.words.Alphabet;
import de.learnlib.algorithms.lstargeneric.table.ObservationTable;
import de.learnlib.algorithms.lstargeneric.table.Row;
import de.learnlib.api.MembershipOracle;
/**
* Collection of predefined observation table closing strategies.
*
* @see ClosingStrategy
*
* @author Malte Isberner
*
*/
public class ClosingStrategies {
/**
* Closing strategy that randomly selects one representative row to close from each equivalence
* class.
*/
public static final ClosingStrategy
© 2015 - 2025 Weber Informatics LLC | Privacy Policy