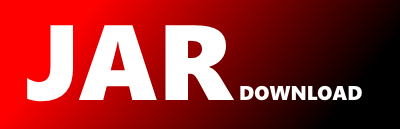
de.learnlib.algorithms.lstargeneric.mealy.ExtensibleLStarMealy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-lstar-generic Show documentation
Show all versions of learnlib-lstar-generic Show documentation
A flexible, optimized version of Dana Angluin's L* algorithm
/* Copyright (C) 2013 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* LearnLib is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License version 3.0 as published by the Free Software Foundation.
*
* LearnLib is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with LearnLib; if not, see
* .
*/
package de.learnlib.algorithms.lstargeneric.mealy;
import java.util.ArrayList;
import java.util.List;
import net.automatalib.automata.concepts.SuffixOutput;
import net.automatalib.automata.transout.MealyMachine;
import net.automatalib.automata.transout.impl.compact.CompactMealy;
import net.automatalib.automata.transout.impl.compact.CompactMealyTransition;
import net.automatalib.words.Alphabet;
import net.automatalib.words.Word;
import de.learnlib.algorithms.lstargeneric.ExtensibleAutomatonLStar;
import de.learnlib.algorithms.lstargeneric.ce.ObservationTableCEXHandler;
import de.learnlib.algorithms.lstargeneric.closing.ClosingStrategy;
import de.learnlib.algorithms.lstargeneric.table.Row;
import de.learnlib.api.LearningAlgorithm.MealyLearner;
import de.learnlib.api.MembershipOracle;
import de.learnlib.oracles.DefaultQuery;
public class ExtensibleLStarMealy extends
ExtensibleAutomatonLStar, I, Word, Integer, CompactMealyTransition, Void, O, CompactMealy>
implements MealyLearner {
private final List outputTable
= new ArrayList();
public ExtensibleLStarMealy(Alphabet alphabet,
MembershipOracle> oracle,
List> initialSuffixes,
ObservationTableCEXHandler super I, ? super Word> cexHandler,
ClosingStrategy super I, ? super Word> closingStrategy) {
super(alphabet, oracle, new CompactMealy(alphabet),
LStarMealyUtil.ensureSuffixCompliancy(initialSuffixes, alphabet, cexHandler.needsConsistencyCheck()),
cexHandler,
closingStrategy);
}
@Override
protected Void stateProperty(Row stateRow) {
return null;
}
@Override
protected O transitionProperty(Row stateRow, int inputIdx) {
updateOutputs();
Row transRow = stateRow.getSuccessor(inputIdx);
return outputTable.get(transRow.getRowId() - 1);
}
@Override
protected List> initialSuffixes() {
return initialSuffixes;
}
protected void updateOutputs() {
int numOutputs = outputTable.size();
int numTransRows = table.numTotalRows() - 1;
int newOutputs = numTransRows - numOutputs;
if(newOutputs == 0)
return;
List>> outputQueries
= new ArrayList>>(numOutputs);
for(int i = numOutputs+1; i <= numTransRows; i++) {
Row row = table.getRow(i);
Word rowPrefix = row.getPrefix();
int prefixLen = rowPrefix.size();
outputQueries.add(new DefaultQuery>(rowPrefix.prefix(prefixLen - 1),
rowPrefix.suffix(1)));
}
oracle.processQueries(outputQueries);
for(int i = 0; i < newOutputs; i++) {
DefaultQuery> query = outputQueries.get(i);
O outSym = query.getOutput().getSymbol(0);
outputTable.add(outSym);
}
}
@Override
protected MealyMachine, I, ?, O> exposeInternalHypothesis() {
return internalHyp;
}
@Override
protected SuffixOutput> hypothesisOutput() {
return internalHyp;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy