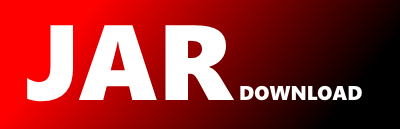
de.learnlib.oracle.parallelism.ParallelOracleBuilders Maven / Gradle / Ivy
/* Copyright (C) 2013-2018 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.learnlib.oracle.parallelism;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.function.Supplier;
import javax.annotation.Nonnull;
import javax.annotation.ParametersAreNonnullByDefault;
import com.google.common.base.Suppliers;
import de.learnlib.api.oracle.MembershipOracle;
/**
* Builders for (static and dynamic) parallel oracles.
*
* Using the methods defined in this class is the preferred way of instantiating parallel oracles.
*
* Usage examples
*
* Creating a static parallel oracle with a minimum batch size of 20 and a fixed thread pool, using a membership oracle
* shared by 4 threads:
*
* ParallelOracleBuilders.newStaticParallelOracle(membershipOracle)
* .withMinBatchSize(20)
* .withNumInstances(4)
* .withPoolPolicy(PoolPolicy.FIXED)
* .create();
*
*
* Creating a dynamic parallel oracle with a custom executor, and a batch size of 5, using a shared membership oracle:
*
* ParallelOracleBuilders.newDynamicParallelOracle(membershipOracle)
* .withBatchSize(5)
* .withCustomExecutor(myExecutor)
* .create();
*
*
* Creating a dynamic parallel oracle with a cached thread pool of maximum size 4, a batch size of 5, using an oracle
* supplier:
*
* ParallelOracleBuilders.newDynamicParallelOracle(oracleSupplier)
* .withBatchSize(5)
* .withPoolSize(4)
* .withPoolPolicy(PoolPolicy.CACHED)
* .create();
*
*
* @author Malte Isberner
*/
@ParametersAreNonnullByDefault
public final class ParallelOracleBuilders {
private ParallelOracleBuilders() {
throw new AssertionError("Constructor should not be invoked");
}
@Nonnull
public static DynamicParallelOracleBuilder newDynamicParallelOracle(MembershipOracle sharedOracle) {
return newDynamicParallelOracle(() -> sharedOracle);
}
@Nonnull
public static DynamicParallelOracleBuilder newDynamicParallelOracle(Supplier extends MembershipOracle> oracleSupplier) {
return new DynamicParallelOracleBuilder<>(oracleSupplier);
}
@Nonnull
public static StaticParallelOracleBuilder newStaticParallelOracle(MembershipOracle sharedOracle) {
return newStaticParallelOracle(Suppliers.ofInstance(sharedOracle));
}
@Nonnull
public static StaticParallelOracleBuilder newStaticParallelOracle(Supplier extends MembershipOracle> oracleSupplier) {
return new StaticParallelOracleBuilder<>(oracleSupplier);
}
@Nonnull
@SafeVarargs
public static StaticParallelOracleBuilder newStaticParallelOracle(MembershipOracle firstOracle,
MembershipOracle... otherOracles) {
List> oracles = new ArrayList<>(otherOracles.length + 1);
oracles.add(firstOracle);
Collections.addAll(oracles, otherOracles);
return newStaticParallelOracle(oracles);
}
@Nonnull
public static StaticParallelOracleBuilder newStaticParallelOracle(Collection extends MembershipOracle> oracles) {
return new StaticParallelOracleBuilder<>(oracles);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy