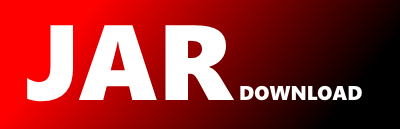
de.learnlib.algorithm.ttt.base.BaseTTTDiscriminationTree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-ttt Show documentation
Show all versions of learnlib-ttt Show documentation
This artifact provides the implementation of the TTT algorithm as described in the paper "The TTT Algorithm: A
Redundancy-Free Approach to Active Automata Learning" (https://doi.org/10.1007/978-3-319-11164-3_26) by Malte
Isberner, Falk Howar, and Bernhard Steffen.
The newest version!
/* Copyright (C) 2013-2023 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.learnlib.algorithm.ttt.base;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.function.Predicate;
import java.util.function.Supplier;
import de.learnlib.datastructure.discriminationtree.model.AbstractDiscriminationTree;
import de.learnlib.oracle.MembershipOracle;
import de.learnlib.query.DefaultQuery;
import net.automatalib.visualization.VisualizationHelper;
import net.automatalib.word.Word;
/**
* The discrimination tree data structure.
*
* @param
* input symbol type
*/
public class BaseTTTDiscriminationTree
extends AbstractDiscriminationTree, I, D, TTTState, AbstractBaseDTNode> {
public BaseTTTDiscriminationTree(MembershipOracle oracle,
Supplier extends AbstractBaseDTNode> supplier) {
this(oracle, supplier.get());
}
public BaseTTTDiscriminationTree(MembershipOracle oracle, AbstractBaseDTNode root) {
super(root, oracle);
}
/**
* Sifts an access sequence provided by an object into the tree, starting at the root. This can either be a "soft"
* sift, which stops either at the leaf or at the first temporary node, or a "hard" sift, stopping only at a
* leaf.
*
* @param word
* the object providing the access sequence
* @param hard
* flag, whether this should be a soft or a hard sift
*
* @return the leaf resulting from the sift operation
*/
public AbstractBaseDTNode sift(Word word, boolean hard) {
return sift(getRoot(), word, hard);
}
public AbstractBaseDTNode sift(AbstractBaseDTNode start, Word prefix, boolean hard) {
return super.sift(start, prefix, getSiftPredicate(hard));
}
public List> sift(List> starts,
List> prefixes,
boolean hard) {
return super.sift(starts, prefixes, getSiftPredicate(hard));
}
@Override
public AbstractBaseDTNode sift(AbstractBaseDTNode start, Word prefix) {
return sift(start, prefix, true);
}
@Override
public List> sift(List> starts, List> prefixes) {
return sift(starts, prefixes, true);
}
@Override
protected DefaultQuery buildQuery(AbstractBaseDTNode node, Word prefix) {
return new DefaultQuery<>(prefix, node.getDiscriminator());
}
private static Predicate> getSiftPredicate(boolean hard) {
return n -> !n.isLeaf() && (hard || !n.isTemp());
}
@Override
public VisualizationHelper, Entry>> getVisualizationHelper() {
return new VisualizationHelper, Entry>>() {
@Override
public boolean getNodeProperties(AbstractBaseDTNode node, Map properties) {
if (node.isLeaf()) {
properties.put(NodeAttrs.SHAPE, NodeShapes.BOX);
properties.put(NodeAttrs.LABEL, String.valueOf(node.getData()));
} else {
properties.put(NodeAttrs.LABEL, node.getDiscriminator().toString());
if (!node.isTemp()) {
properties.put(NodeAttrs.SHAPE, NodeShapes.OVAL);
} else if (node.isBlockRoot()) {
properties.put(NodeAttrs.SHAPE, NodeShapes.DOUBLEOCTAGON);
} else {
properties.put(NodeAttrs.SHAPE, NodeShapes.OCTAGON);
}
}
return true;
}
@Override
public boolean getEdgeProperties(AbstractBaseDTNode src,
Map.Entry> edge,
AbstractBaseDTNode tgt,
Map properties) {
properties.put(EdgeAttrs.LABEL, String.valueOf(edge.getValue().getParentOutcome()));
return true;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy