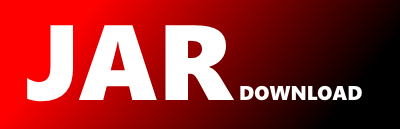
de.learnlib.algorithm.ttt.base.TTTState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-ttt Show documentation
Show all versions of learnlib-ttt Show documentation
This artifact provides the implementation of the TTT algorithm as described in the paper "The TTT Algorithm: A
Redundancy-Free Approach to Active Automata Learning" (https://doi.org/10.1007/978-3-319-11164-3_26) by Malte
Isberner, Falk Howar, and Bernhard Steffen.
The newest version!
/* Copyright (C) 2013-2023 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.learnlib.algorithm.ttt.base;
import de.learnlib.AccessSequenceProvider;
import net.automatalib.common.smartcollection.ResizingArrayStorage;
import net.automatalib.word.Word;
/**
* A state in a {@link AbstractTTTHypothesis}.
*
* @param
* input symbol
*/
public class TTTState implements AccessSequenceProvider {
final int id;
private final ResizingArrayStorage> transitions;
private final TTTTransition parentTransition;
AbstractBaseDTNode dtLeaf;
public TTTState(int initialAlphabetSize, TTTTransition parentTransition, int id) {
this.id = id;
this.parentTransition = parentTransition;
this.transitions = new ResizingArrayStorage<>(TTTTransition.class, initialAlphabetSize);
}
/**
* Checks whether this state is the initial state (i.e., the root of the spanning tree).
*
* @return {@code true} if this state is the initial state, {@code false} otherwise
*/
public boolean isRoot() {
return getParentTransition() == null;
}
public TTTTransition getParentTransition() {
return parentTransition;
}
/**
* Retrieves the discrimination tree leaf associated with this state.
*
* @return the discrimination tree leaf associated with this state
*/
public AbstractBaseDTNode getDTLeaf() {
return dtLeaf;
}
@Override
public Word getAccessSequence() {
if (getParentTransition() != null) {
return getParentTransition().getAccessSequence();
}
return Word.epsilon(); // root
}
@Override
public String toString() {
return "s" + id;
}
public void setTransition(int idx, TTTTransition transition) {
transitions.array[idx] = transition;
}
public TTTTransition getTransition(int idx) {
return transitions.array[idx];
}
public TTTTransition[] getTransitions() {
return transitions.array;
}
/**
* See {@link ResizingArrayStorage#ensureCapacity(int)}.
*/
public boolean ensureInputCapacity(int capacity) {
return this.transitions.ensureCapacity(capacity);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy