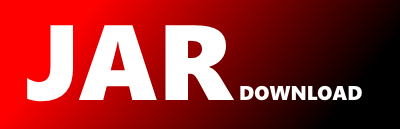
de.learnlib.algorithm.ttt.mealy.TTTLearnerMealy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-ttt Show documentation
Show all versions of learnlib-ttt Show documentation
This artifact provides the implementation of the TTT algorithm as described in the paper "The TTT Algorithm: A
Redundancy-Free Approach to Active Automata Learning" (https://doi.org/10.1007/978-3-319-11164-3_26) by Malte
Isberner, Falk Howar, and Bernhard Steffen.
The newest version!
/* Copyright (C) 2013-2023 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.learnlib.algorithm.ttt.mealy;
import com.github.misberner.buildergen.annotations.GenerateBuilder;
import de.learnlib.acex.AcexAnalyzer;
import de.learnlib.acex.MealyOutInconsPrefixTransformAcex;
import de.learnlib.acex.OutInconsPrefixTransformAcex;
import de.learnlib.algorithm.LearningAlgorithm;
import de.learnlib.algorithm.ttt.base.AbstractBaseDTNode;
import de.learnlib.algorithm.ttt.base.AbstractTTTLearner;
import de.learnlib.algorithm.ttt.base.BaseTTTDiscriminationTree;
import de.learnlib.algorithm.ttt.base.OutputInconsistency;
import de.learnlib.algorithm.ttt.base.TTTState;
import de.learnlib.algorithm.ttt.base.TTTTransition;
import de.learnlib.oracle.MembershipOracle;
import de.learnlib.query.DefaultQuery;
import de.learnlib.util.mealy.MealyUtil;
import net.automatalib.alphabet.Alphabet;
import net.automatalib.automaton.transducer.MealyMachine;
import net.automatalib.word.Word;
import net.automatalib.word.WordBuilder;
public class TTTLearnerMealy extends AbstractTTTLearner, I, Word>
implements LearningAlgorithm.MealyLearner {
@GenerateBuilder(defaults = AbstractTTTLearner.BuilderDefaults.class)
public TTTLearnerMealy(Alphabet alphabet, MembershipOracle> oracle, AcexAnalyzer analyzer) {
super(alphabet,
oracle,
new TTTHypothesisMealy<>(alphabet),
new BaseTTTDiscriminationTree<>(oracle, TTTDTNodeMealy::new),
analyzer);
}
@Override
@SuppressWarnings("unchecked")
public MealyMachine, I, ?, O> getHypothesisModel() {
return (TTTHypothesisMealy) hypothesis;
}
@Override
protected TTTTransition> createTransition(TTTState> state, I sym) {
TTTTransitionMealy trans = new TTTTransitionMealy<>(state, sym);
trans.output = query(state, Word.fromLetter(sym)).firstSymbol();
return trans;
}
@Override
@SuppressWarnings("unchecked")
protected boolean refineHypothesisSingle(DefaultQuery> ceQuery) {
DefaultQuery> shortenedCeQuery =
MealyUtil.shortenCounterExample((TTTHypothesisMealy) hypothesis, ceQuery);
return shortenedCeQuery != null && super.refineHypothesisSingle(shortenedCeQuery);
}
@Override
protected OutInconsPrefixTransformAcex> deriveAcex(OutputInconsistency> outIncons) {
TTTState> source = outIncons.srcState;
Word suffix = outIncons.suffix;
OutInconsPrefixTransformAcex> acex = new MealyOutInconsPrefixTransformAcex<>(suffix,
oracle,
w -> getDeterministicState(
source,
w).getAccessSequence());
acex.setEffect(0, outIncons.targetOut);
Word lastHypOut = computeHypothesisOutput(getAnySuccessor(source, suffix.prefix(-1)), suffix.suffix(1));
acex.setEffect(suffix.length() - 1, lastHypOut);
return acex;
}
@Override
protected Word succEffect(Word effect) {
return effect.subWord(1);
}
@Override
protected OutputInconsistency> findOutputInconsistency() {
OutputInconsistency> best = null;
for (TTTState> state : hypothesis.getStates()) {
AbstractBaseDTNode> node = state.getDTLeaf();
while (!node.isRoot()) {
Word expectedOut = node.getParentOutcome();
node = node.getParent();
Word suffix = node.getDiscriminator();
Word hypOut = computeHypothesisOutput(state, suffix);
int mismatchIdx = MealyUtil.findMismatch(expectedOut, hypOut);
if (mismatchIdx != MealyUtil.NO_MISMATCH && (best == null || mismatchIdx <= best.suffix.length())) {
best = new OutputInconsistency<>(state,
suffix.prefix(mismatchIdx + 1),
expectedOut.prefix(mismatchIdx + 1));
}
}
}
return best;
}
@Override
protected Word predictSuccOutcome(TTTTransition> trans,
AbstractBaseDTNode> succSeparator) {
TTTTransitionMealy mtrans = (TTTTransitionMealy) trans;
if (succSeparator == null) {
return Word.fromLetter(mtrans.output);
}
Word subtreeLabel = succSeparator.subtreeLabel(trans.getDTTarget());
assert subtreeLabel != null;
return subtreeLabel.prepend(mtrans.output);
}
@Override
protected Word computeHypothesisOutput(TTTState> state, Word suffix) {
TTTState> curr = state;
WordBuilder wb = new WordBuilder<>(suffix.length());
for (I sym : suffix) {
TTTTransitionMealy trans = (TTTTransitionMealy) hypothesis.getInternalTransition(curr, sym);
wb.append(trans.output);
curr = getAnyTarget(trans);
}
return wb.toWord();
}
@Override
protected AbstractBaseDTNode> createNewNode(AbstractBaseDTNode> parent,
Word parentOutput) {
return new TTTDTNodeMealy<>(parent, parentOutput);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy