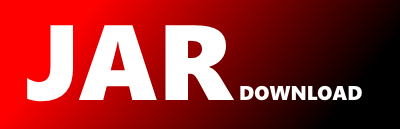
de.learnlib.algorithm.ttt.moore.TTTHypothesisMoore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-ttt Show documentation
Show all versions of learnlib-ttt Show documentation
This artifact provides the implementation of the TTT algorithm as described in the paper "The TTT Algorithm: A
Redundancy-Free Approach to Active Automata Learning" (https://doi.org/10.1007/978-3-319-11164-3_26) by Malte
Isberner, Falk Howar, and Bernhard Steffen.
The newest version!
/* Copyright (C) 2013-2023 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.learnlib.algorithm.ttt.moore;
import de.learnlib.algorithm.ttt.base.AbstractTTTHypothesis;
import de.learnlib.algorithm.ttt.base.TTTTransition;
import net.automatalib.alphabet.Alphabet;
import net.automatalib.automaton.UniversalDeterministicAutomaton;
import net.automatalib.automaton.UniversalDeterministicAutomaton.FullIntAbstraction;
import net.automatalib.automaton.transducer.MooreMachine;
import net.automatalib.word.Word;
/**
* A {@link MooreMachine}-based specialization of the TTT hypothesis.
*
* @param
* input symbol type
* @param
* output symbol type
*/
public class TTTHypothesisMoore
extends AbstractTTTHypothesis, I, Word, TTTStateMoore>
implements MooreMachine, I, TTTStateMoore, O>,
FullIntAbstraction, O, Void> {
/**
* Constructor.
*
* @param alphabet
* the input alphabet
*/
public TTTHypothesisMoore(Alphabet alphabet) {
super(alphabet);
}
@Override
protected TTTStateMoore mapTransition(TTTTransition> internalTransition) {
return (TTTStateMoore) internalTransition.getTarget();
}
@Override
public O getStateProperty(int state) {
TTTStateMoore mooreState = states.get(state);
return mooreState.getOutput();
}
@Override
protected TTTStateMoore newState(int alphabetSize, TTTTransition> parent, int id) {
return new TTTStateMoore<>(alphabetSize, parent, id);
}
@Override
public UniversalDeterministicAutomaton.FullIntAbstraction, O, Void> fullIntAbstraction(Alphabet alphabet) {
if (alphabet.equals(getInputAlphabet())) {
return this;
}
return MooreMachine.super.fullIntAbstraction(alphabet);
}
@Override
public O getStateOutput(TTTStateMoore state) {
return state.getOutput();
}
@Override
public TTTStateMoore getSuccessor(TTTStateMoore transition) {
return transition;
}
@Override
public Void getTransitionProperty(TTTStateMoore state) {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy