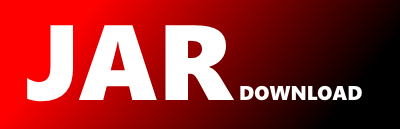
de.learnlib.algorithm.ttt.moore.TTTLearnerMoore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of learnlib-ttt Show documentation
Show all versions of learnlib-ttt Show documentation
This artifact provides the implementation of the TTT algorithm as described in the paper "The TTT Algorithm: A
Redundancy-Free Approach to Active Automata Learning" (https://doi.org/10.1007/978-3-319-11164-3_26) by Malte
Isberner, Falk Howar, and Bernhard Steffen.
The newest version!
/* Copyright (C) 2013-2023 TU Dortmund
* This file is part of LearnLib, http://www.learnlib.de/.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.learnlib.algorithm.ttt.moore;
import com.github.misberner.buildergen.annotations.GenerateBuilder;
import de.learnlib.acex.AcexAnalyzer;
import de.learnlib.acex.MooreOutInconsPrefixTransformAcex;
import de.learnlib.acex.OutInconsPrefixTransformAcex;
import de.learnlib.algorithm.LearningAlgorithm.MooreLearner;
import de.learnlib.algorithm.ttt.base.AbstractBaseDTNode;
import de.learnlib.algorithm.ttt.base.AbstractTTTLearner;
import de.learnlib.algorithm.ttt.base.BaseTTTDiscriminationTree;
import de.learnlib.algorithm.ttt.base.OutputInconsistency;
import de.learnlib.algorithm.ttt.base.TTTState;
import de.learnlib.algorithm.ttt.base.TTTTransition;
import de.learnlib.oracle.MembershipOracle;
import de.learnlib.query.DefaultQuery;
import de.learnlib.util.moore.MooreUtil;
import net.automatalib.alphabet.Alphabet;
import net.automatalib.automaton.transducer.MooreMachine;
import net.automatalib.word.Word;
import net.automatalib.word.WordBuilder;
/**
* A {@link MooreMachine}-based specialization of the TTT learner.
*
* @param
* input symbol type
* @param
* output symbols type
*/
public class TTTLearnerMoore extends AbstractTTTLearner, I, Word>
implements MooreLearner {
@GenerateBuilder(defaults = AbstractTTTLearner.BuilderDefaults.class)
public TTTLearnerMoore(Alphabet alphabet, MembershipOracle> oracle, AcexAnalyzer analyzer) {
super(alphabet,
oracle,
new TTTHypothesisMoore<>(alphabet),
new BaseTTTDiscriminationTree<>(oracle, TTTDTNodeMoore::new),
analyzer);
dtree.getRoot().split(Word.epsilon(), oracle.answerQuery(Word.epsilon()));
}
@Override
protected Word succEffect(Word effect) {
return effect.subWord(1);
}
@Override
protected Word predictSuccOutcome(TTTTransition> trans,
AbstractBaseDTNode> succSeparator) {
TTTStateMoore curr = (TTTStateMoore) trans.getSource();
final Word label = succSeparator.subtreeLabel(trans.getDTTarget());
assert label != null;
return label.prepend(curr.getOutput());
}
@Override
protected void initializeState(TTTState> state) {
super.initializeState(state);
TTTStateMoore mooreState = (TTTStateMoore) state;
final Word label = dtree.getRoot().subtreeLabel(mooreState.getDTLeaf());
assert label != null && !label.isEmpty();
mooreState.setOutput(label.firstSymbol());
}
@Override
@SuppressWarnings("unchecked")
protected boolean refineHypothesisSingle(DefaultQuery> ceQuery) {
DefaultQuery> shortenedCeQuery =
MooreUtil.shortenCounterExample((TTTHypothesisMoore) hypothesis, ceQuery);
return shortenedCeQuery != null && super.refineHypothesisSingle(shortenedCeQuery);
}
@Override
protected OutInconsPrefixTransformAcex> deriveAcex(OutputInconsistency> outIncons) {
TTTState> source = outIncons.srcState;
Word suffix = outIncons.suffix;
OutInconsPrefixTransformAcex> acex = new MooreOutInconsPrefixTransformAcex<>(suffix,
oracle,
w -> getDeterministicState(
source,
w).getAccessSequence());
acex.setEffect(0, outIncons.targetOut);
Word lastHypOut = computeHypothesisOutput(getAnySuccessor(source, suffix), Word.epsilon());
acex.setEffect(suffix.length(), lastHypOut);
return acex;
}
@Override
protected Word computeHypothesisOutput(TTTState> state, Word suffix) {
TTTStateMoore curr = (TTTStateMoore) state;
WordBuilder wb = new WordBuilder<>(suffix.length());
wb.append(curr.output);
if (suffix.isEmpty()) {
return wb.toWord();
}
for (I sym : suffix) {
curr = (TTTStateMoore) getAnySuccessor(curr, sym);
wb.append(curr.output);
}
return wb.toWord();
}
@Override
protected AbstractBaseDTNode> createNewNode(AbstractBaseDTNode> parent,
Word parentOutput) {
return new TTTDTNodeMoore<>(parent, parentOutput);
}
@Override
@SuppressWarnings("unchecked") // parent class uses the same instance that we pass in the constructor
public TTTHypothesisMoore getHypothesisModel() {
return (TTTHypothesisMoore) hypothesis;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy