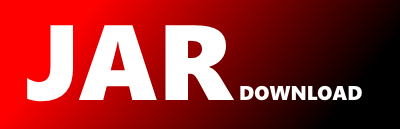
de.matrixweb.smaller.config.Processor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smaller-config Show documentation
Show all versions of smaller-config Show documentation
smaller-config is a unified config file format for smaller and smaller-dev-server.
The file format could be json or yaml.
package de.matrixweb.smaller.config;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
/**
* @author markusw
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class Processor {
private String src;
private String dest;
private Map options;
/**
* @return the src
*/
public String getSrc() {
return this.src;
}
/**
* @param src
* the src to set
*/
public void setSrc(final String src) {
this.src = src;
}
/**
* @return the dest
*/
public String getDest() {
return this.dest;
}
/**
* @param dest
* the dest to set
*/
public void setDest(final String dest) {
this.dest = dest;
}
/**
* @return the options
*/
public Map getOptions() {
final Map map = new HashMap();
if (this.options != null) {
for (final Entry entry : this.options.entrySet()) {
map.put(entry.getKey(), new Option(entry.getValue()));
}
}
return map;
}
/**
* @return Returns the plain objects
*/
public Map getPlainOptions() {
if (this.options == null) {
this.options = new HashMap();
}
return this.options;
}
/**
* @param options
* the options to set
*/
public void setOptions(final Map options) {
this.options = options;
}
/** */
public static class Option {
private Object value;
/**
*
* @param value
*/
public Option(final String value) {
this.value = value;
}
/**
* @param value
*/
public Option(final Boolean value) {
this.value = value;
}
/**
* @param value
*/
public Option(final Integer value) {
this.value = value;
}
/**
* @param value
*/
public Option(final Double value) {
this.value = value;
}
/**
* @param value
*/
public Option(final Map value) {
this.value = value;
}
/**
* @param value
*/
private Option(final Object value) {
this.value = value;
}
/**
* @return the value
*/
public Object getValue() {
return this.value;
}
/**
* @param value
* the value to set
*/
public void setValue(final Object value) {
this.value = value;
}
/**
* @return The string
*/
@JsonIgnore
public String getString() {
return this.value != null ? this.value.toString() : null;
}
/**
* @return The boolean
*/
@JsonIgnore
public Boolean getBoolean() {
return this.value != null ? Boolean.valueOf(this.value.toString()) : null;
}
/**
* @return The integer
*/
@JsonIgnore
public Integer getInteger() {
return this.value != null ? Integer.valueOf(this.value.toString()) : null;
}
/**
* @return The double
*/
@JsonIgnore
public Double getDouble() {
return this.value != null ? Double.valueOf(this.value.toString()) : null;
}
/**
* @return The map
*/
@JsonIgnore
@SuppressWarnings("unchecked")
public Map getMap() {
return (Map) this.value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy