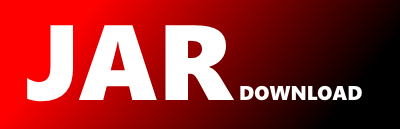
mServer.crawler.sender.orf.parser.OrfEpisodeDeserializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MServer Show documentation
Show all versions of MServer Show documentation
The crawler for mediathekview/MediathekView
package mServer.crawler.sender.orf.parser;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import java.lang.reflect.Type;
import java.time.Duration;
import java.util.Optional;
import mServer.crawler.sender.orf.JsonUtils;
import mServer.crawler.sender.orf.OrfEpisodeInfoDTO;
import mServer.crawler.sender.orf.OrfVideoInfoDTO;
public class OrfEpisodeDeserializer implements JsonDeserializer> {
private static final String ELEMENT_VIDEO = "video";
private static final String ATTRIBUTE_TITLE = "title";
private static final String ATTRIBUTE_DESCRIPTION = "description";
private static final String ATTRIBUTE_DURATION = "duration";
@Override
public Optional deserialize(JsonElement aJsonElement, Type aType, JsonDeserializationContext aContext) throws JsonParseException {
if (!aJsonElement.getAsJsonObject().has(ELEMENT_VIDEO)) {
return Optional.empty();
}
JsonObject videoObject = aJsonElement.getAsJsonObject().get(ELEMENT_VIDEO).getAsJsonObject();
final Optional title = JsonUtils.getAttributeAsString(videoObject, ATTRIBUTE_TITLE);
final Optional description = JsonUtils.getAttributeAsString(videoObject, ATTRIBUTE_DESCRIPTION);
final Optional duration = parseDuration(videoObject);
final Optional videoInfoOptional = parseUrls(videoObject);
if (videoInfoOptional.isPresent()) {
OrfEpisodeInfoDTO episode = new OrfEpisodeInfoDTO(videoInfoOptional.get(), title, description, duration);
return Optional.of(episode);
}
return Optional.empty();
}
private Optional parseUrls(final JsonObject aVideoObject) {
OrfVideoDetailDeserializer deserializer = new OrfVideoDetailDeserializer();
return deserializer.deserializeVideoObject(aVideoObject);
}
private static Optional parseDuration(final JsonObject aVideoObject) {
if (aVideoObject.has(ATTRIBUTE_DURATION)) {
Long durationValue = aVideoObject.get(ATTRIBUTE_DURATION).getAsLong();
// Duration ist in Millisekunden angegeben, diese interessieren aber nicht
return Optional.of(Duration.ofSeconds(durationValue / 1000));
}
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy